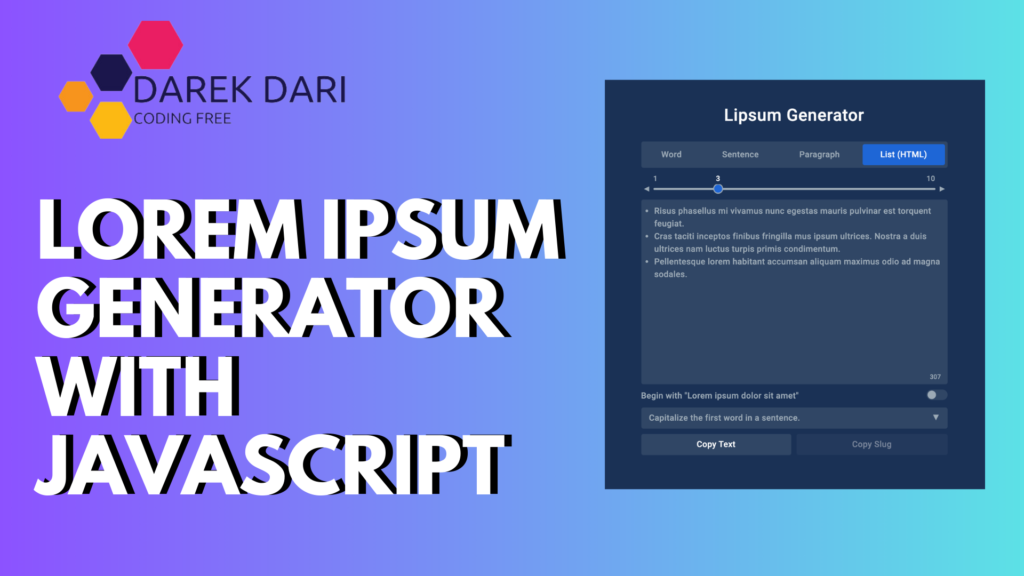
Table of Contents
Introduction
Welcome to our JavaScript journey where we’ll explore the creation of an amazing Lorem Ipsum generator!
Whether you’re a beginner in coding or searching for an exciting project to enhance your skills, you’ve come to the perfect spot.
By the conclusion of this guide, you’ll be producing Lorem Ipsum paragraphs like an expert.
javascript exercises
javascript exercises and solutions
javascript exercises for beginners
javascript function exercises
javascript practice exercises with solutions
javascript tasks for practice
javascript variables exercises
js exercises
What is the lorem ipsum function in JavaScript?
Lorem Ipsum has been utilized in the printing and typesetting industry since the 1500s as a placeholder text. It serves the purpose of filling spaces in designs until real content is added.
The nonsensical nature of Lorem Ipsum, derived from sections of a Latin text by Cicero, helps to keep the focus on the design rather than the content itself.
Why Lorem Ipsum?
Prior to delving into the coding part, let’s take a quick look at the reasons behind the widespread use of Lorem Ipsum text in design and development.
Lorem Ipsum has served as the standard placeholder text in the industry for many years, enabling designers and developers to concentrate on layout and visual elements without the need for meaningful content.
Let’s Start Coding
Step 1: Getting Started
To begin, let’s set up our project environment. We’ll create a simple HTML file (index.html
) and link it to our JavaScript file (script.js
). This setup will allow us to see our generator in action directly in the browser.
Setting Up index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Let's Generate Lorem Ipsum!</title>
</head>
<body>
<h1>Let's Generate Lorem Ipsum!</h1>
<div id="generatedLoremIpsum">
<!-- Our generated Lorem Ipsum text will appear here -->
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Writing the JavaScript Magic
Now, let’s bring our generator to life with JavaScript! We’ll write a function that dynamically generates Lorem Ipsum text based on user-defined parameters.
Explaining the Code
// Function to generate Lorem Ipsum text
function generateLoremIpsum(paragraphs, wordsPerParagraph) {
// Our beloved Lorem Ipsum text
const loremIpsum = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.";
// Splitting Lorem Ipsum into an array of words
const words = loremIpsum.split(' ');
let result = ''; // To hold our generated text
// Generating specified number of paragraphs
for (let i = 0; i < paragraphs; i++) {
// Generating each paragraph with specified number of words
for (let j = 0; j < wordsPerParagraph; j++) {
// Picking words randomly from Lorem Ipsum text
const randomIndex = Math.floor(Math.random() * words.length);
result += words[randomIndex] + ' ';
}
result += '\n\n'; // Adding double line breaks to separate paragraphs
}
return result;
}
// Example usage: Generate 3 paragraphs with 5 words each
const lorem = generateLoremIpsum(3, 5);
console.log(lorem); // Displaying generated Lorem Ipsum in the browser console
Step 3: Bringing It All Together
Let’s integrate our JavaScript function with our HTML to see the magic unfold on the webpage!
Enhancing script.js
// Function to display Lorem Ipsum text on the webpage
function displayLoremIpsum(paragraphs, wordsPerParagraph) {
const lorem = generateLoremIpsum(paragraphs, wordsPerParagraph);
const generatedLoremIpsumElement = document.getElementById('generatedLoremIpsum');
generatedLoremIpsumElement.textContent = lorem;
}
// Example usage: Display 3 paragraphs with 5 words each on the webpage
displayLoremIpsum(3, 5);
Step 4: Enjoying the Results
Voilà! Now, when you open index.html
in your browser, you’ll see beautifully generated Lorem Ipsum text waiting for you. Experiment with different numbers of paragraphs and words per paragraph to create exactly the length and style of text you need.
Code Enhancements
HTML Update (index.html
)
Custom Text Input: Allow users to input their own text to generate a mixed Lorem Ipsum output.
Add an input field for users to enter custom text:
<div>
<label for="customText">Enter Custom Text:</label><br>
<textarea id="customText" rows="4" cols="50"></textarea><br>
<button onclick="generateCustomLoremIpsum()">Generate Custom Lorem Ipsum</button>
</div>
JavaScript Update (script.js
)
Update the JavaScript code to incorporate user-provided text:
// Function to generate Lorem Ipsum text with custom input
function generateCustomLoremIpsum(paragraphs, wordsPerParagraph, customText) {
const loremIpsum = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.";
// Split Lorem Ipsum into an array of words
const loremWords = loremIpsum.split(' ');
const customWords = customText.split(' ');
let result = '';
for (let i = 0; i < paragraphs; i++) {
for (let j = 0; j < wordsPerParagraph; j++) {
const useCustomText = Math.random() > 0.5; // Randomly decide to use custom text or lorem ipsum
if (useCustomText && customWords.length > 0) {
const randomIndex = Math.floor(Math.random() * customWords.length);
result += customWords[randomIndex] + ' ';
} else {
const randomIndex = Math.floor(Math.random() * loremWords.length);
result += loremWords[randomIndex] + ' ';
}
}
result += '\n\n';
}
return result;
}
// Function to display custom Lorem Ipsum text on the webpage
function displayCustomLoremIpsum() {
const paragraphs = 3; // Example: Generate 3 paragraphs
const wordsPerParagraph = 5; // Example: 5 words per paragraph
const customText = document.getElementById('customText').value;
const lorem = generateCustomLoremIpsum(paragraphs, wordsPerParagraph, customText);
const generatedLoremIpsumElement = document.getElementById('generatedLoremIpsum');
generatedLoremIpsumElement.textContent = lorem;
}
// Example usage: Display custom Lorem Ipsum based on user input
function generateCustomLoremIpsum() {
displayCustomLoremIpsum();
}
CSS Update (styles.css
)
Styling and Formatting: Enhance the visual appearance of the generated Lorem Ipsum text using CSS.
Create a CSS file and style the generated Lorem Ipsum text:
#generatedLoremIpsum {
font-family: 'Arial', sans-serif;
font-size: 16px;
line-height: 1.6;
margin-top: 20px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #f9f9f9;
}
Link this CSS file in your index.html
:
<head>
<!-- Other meta tags and title -->
<link rel="stylesheet" href="styles.css">
</head>
Update (script.js
)
Lorem Ipsum Variations: Explore different variations of Lorem Ipsum text to add diversity to your generator.
Consider creating arrays of different Lorem Ipsum variations and randomly selecting from them. Here’s a basic example:
// Array of different Lorem Ipsum variations
const loremVariations = [
"Lorem ipsum dolor sit amet, consectetur adipiscing elit.",
"Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.",
"Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.",
// Add more variations as needed
];
// Function to generate Lorem Ipsum text with variations
function generateLoremIpsumWithVariations(paragraphs, wordsPerParagraph) {
let result = '';
for (let i = 0; i < paragraphs; i++) {
for (let j = 0; j < wordsPerParagraph; j++) {
const randomIndex = Math.floor(Math.random() * loremVariations.length);
result += loremVariations[randomIndex] + ' ';
}
result += '\n\n';
}
return result;
}
// Example usage: Generate Lorem Ipsum with variations
const loremWithVariations = generateLoremIpsumWithVariations(3, 5);
console.log(loremWithVariations); // Display generated Lorem Ipsum with variations
Using Lorem Ipsum Generators
You can create a function that generates random Lorem Ipsum text:
function generateLoremIpsum(paragraphs, wordsPerParagraph) {
const loremIpsum = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.";
const words = loremIpsum.split(' ');
let result = '';
for (let i = 0; i < paragraphs; i++) {
for (let j = 0; j < wordsPerParagraph; j++) {
const randomIndex = Math.floor(Math.random() * words.length);
result += words[randomIndex] + ' ';
}
result += '\n\n';
}
return result.trim(); // Trim to remove any extra whitespace
}
// Example usage: Generate 2 paragraphs with 10 words each
const lorem = generateLoremIpsum(2, 10);
console.log(lorem);
Using Predefined Text
You can also define specific dummy text directly in your JavaScript code:
const dummyText = "This is a placeholder text. It can be used for testing layouts and designs.";
console.log(dummyText);
Combining Custom and Lorem Ipsum Text
Mixing predefined text with Lorem Ipsum for varied content:
function generateMixedText(paragraphs, wordsPerParagraph, customText) {
const loremIpsum = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
const words = loremIpsum.split(' ');
let result = '';
for (let i = 0; i < paragraphs; i++) {
for (let j = 0; j < wordsPerParagraph; j++) {
const useCustomText = Math.random() > 0.5;
if (useCustomText && customText) {
result += customText + ' ';
} else {
const randomIndex = Math.floor(Math.random() * words.length);
result += words[randomIndex] + ' ';
}
}
result += '\n\n';
}
return result.trim();
}
// Example usage: Generate 2 paragraphs with a mix of custom and Lorem Ipsum text
const mixedText = generateMixedText(2, 8, "Custom text for testing purposes.");
console.log(mixedText);
Usage
These methods allow you to easily add dummy text in JavaScript for testing layouts, designs, or generating content. Customize the functions according to your specific needs and project requirements.
How do I automatically type lorem ipsum?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Auto Typing Lorem Ipsum</title>
<style>
#autoType {
font-family: 'Arial', sans-serif;
font-size: 18px;
line-height: 1.6;
padding: 20px;
border: 1px solid #ccc;
white-space: pre-wrap; /* Preserve line breaks */
}
</style>
</head>
<body>
<div id="autoType"></div>
<script>
// Function to generate Lorem Ipsum text
function generateLoremIpsum(paragraphs, wordsPerParagraph) {
const loremIpsum = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.";
const words = loremIpsum.split(' ');
let result = '';
for (let i = 0; i < paragraphs; i++) {
for (let j = 0; j < wordsPerParagraph; j++) {
const randomIndex = Math.floor(Math.random() * words.length);
result += words[randomIndex] + ' ';
}
result += '\n\n';
}
return result.trim(); // Trim to remove any extra whitespace
}
// Function to simulate typing effect
function autoTypeLoremIpsum(targetElement, paragraphs, wordsPerParagraph, typingSpeed) {
const loremIpsum = generateLoremIpsum(paragraphs, wordsPerParagraph);
const element = document.getElementById(targetElement);
let index = 0;
function type() {
if (index < loremIpsum.length) {
element.textContent += loremIpsum.charAt(index);
index++;
setTimeout(type, typingSpeed); // Adjust typing speed (in milliseconds)
}
}
type();
}
// Example usage: Auto type Lorem Ipsum into 'autoType' element
autoTypeLoremIpsum('autoType', 3, 10, 50); // Typing speed 50ms per character
</script>
</body>
</html>
Explanation
The HTML structure defines a <div>
element with the ID autoType
, where the dynamically generated Lorem Ipsum text will be displayed. The CSS styles applied to this element (#autoType
) control its appearance, including the font family, size, line height, padding, border, and preservation of line breaks using white-space: pre-wrap
.
Within the JavaScript section of the code, two main functions are defined:
generateLoremIpsum(paragraphs, wordsPerParagraph)
: This function generates Lorem Ipsum text based on the specified number of paragraphs and words per paragraph. It splits a predefined Lorem Ipsum text into an array of words, randomly selects words to form paragraphs, and returns the generated text after trimming any extra whitespace.autoTypeLoremIpsum(targetElement, paragraphs, wordsPerParagraph, typingSpeed)
: This function simulates a typing effect by progressively appending characters from the generated Lorem Ipsum text to the specifiedtargetElement
on the webpage. It usessetTimeout
to control the typing speed, which is specified in milliseconds (typingSpeed
parameter).
An example usage of autoTypeLoremIpsum
is demonstrated at the bottom of the script, where it is called with parameters to type out 3 paragraphs of Lorem Ipsum text, each containing approximately 10 words, at a speed of 50 milliseconds per character.
This setup provides a straightforward approach to dynamically generate and display Lorem Ipsum text with a typing effect on a webpage using HTML, CSS, and JavaScript.
How to generate lorem text in VS Code?
To generate Lorem Ipsum text directly within Visual Studio Code (VS Code), you can use an extension that provides lorem ipsum generation functionality. Here’s how you can do it:
Using Lorem Ipsum Generator Extension
Install an Extension:
- Open VS Code.
- Go to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window (or by pressing
Ctrl+Shift+X
). - In the Extensions Marketplace (the search bar at the top), type “Lorem Ipsum” or “Lorem Ipsum generator”.
- Choose an extension that suits your needs. A popular one is the “Lorem Ipsum” extension by Daniel Imms.
Install the Extension:
- Click on the extension you want to install.
- Click on the “Install” button.
Generate Lorem Ipsum Text:
- After installing the extension, open a file in VS Code where you want to insert Lorem Ipsum text.
- Use the extension’s commands to insert Lorem Ipsum text. Typically, this is done by opening the Command Palette (
Ctrl+Shift+P
orCmd+Shift+P
on macOS), typing “Lorem Ipsum”, and selecting the appropriate command to insert Lorem Ipsum text.
Customize the Lorem Ipsum:
- Some extensions allow you to customize the generated Lorem Ipsum text, such as specifying the number of paragraphs or words per paragraph.
- Follow the extension’s documentation or prompts in VS Code to customize the text according to your needs.
Example with “Lorem Ipsum” Extension
Here’s a brief example using the “Lorem Ipsum” extension:
Install the extension by Daniel Imms (or any similar extension):
- Open VS Code.
- Go to Extensions (Ctrl+Shift+X).
- Search for “Lorem Ipsum” and install the extension.
Usage:
- Open a file in VS Code.
- Open the Command Palette (Ctrl+Shift+P).
- Type “Lorem Ipsum” and select the command to generate Lorem Ipsum text.
- The Lorem Ipsum text will be inserted at the cursor position in your file.
This method allows you to quickly generate Lorem Ipsum text directly within VS Code, saving you time and effort, especially when drafting content or testing layouts.
Conclusion
Congratulations on building your very own Lorem Ipsum generator using JavaScript! This project not only strengthens your coding skills but also adds a bit of playful creativity to your toolkit. Feel free to explore further by adding more features or customizing the generator to suit your preferences.
Thanks for joining this coding adventure with us! Happy generating! 🌟
0 Comments