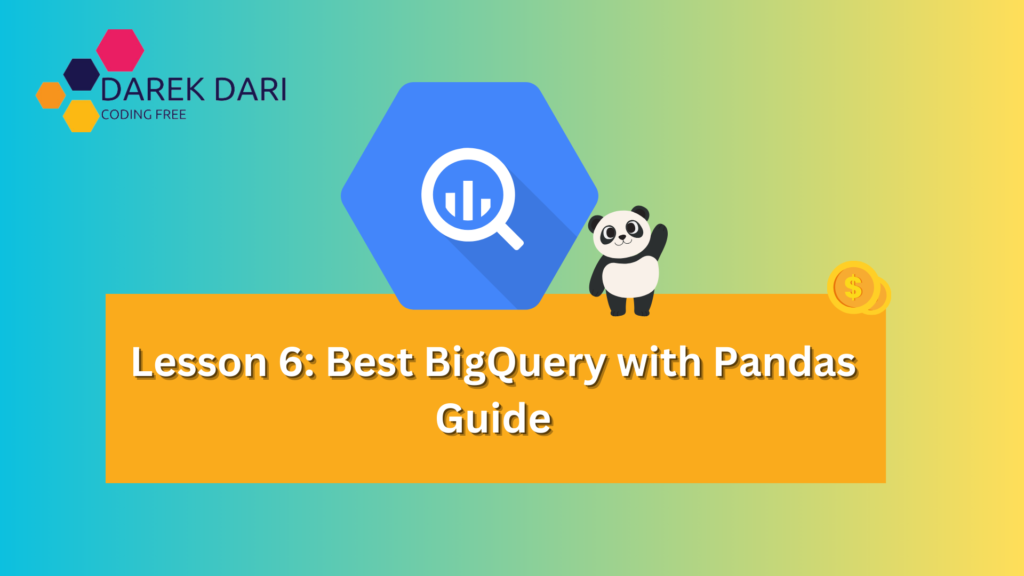
Table of Contents
Introduction
Hello, data enthusiast! 🌟 Are you prepared to elevate your data analytics skills? Welcome to Lesson 6, where we explore the fascinating realm of Google BigQuery and discover how you can leverage its capabilities with Pandas in Python and the versatile R language.
Also, check:
- Lesson 1: What are pandas used for? Pandas Tips and Tricks
- Lesson 2: What does pandas DataFrame mean?
- Lesson 3: Charts and Diagrams in Colab with Matplotlib
- Lesson 4: Interactive Forms in Google Colab with Python vs. R
- Lesson 5: Working with Local Files, Google Drive, Google Sheets, and Google Cloud Storage in Google Colab
- Lesson 6: Best BigQuery with Pandas Python and R Guide
This comprehensive guide will assist you in seamlessly integrating these tools, empowering you to efficiently handle, analyze, and visualize extensive datasets like a true professional.
Well, buckle up, ’cause we’re gonna take a ride through the clouds. No, not the fluffy kind – the Google Cloud kind. Let’s get started!
Getting Set Up
First things first, we need a Cloud Platform project. Head over to the Cloud Resource Manager to set one up. Got it? Sweet! Next, make sure you enable billing so we can actually do stuff. Finally, flip the switch for BigQuery by enabling its APIs here.
Authenticate and Import
Time to let Google know it’s us. Authenticate yourself with:
from google.colab import auth
auth.authenticate_user()
Let’s Play with Pandas and BigQuery
Alright, now let’s get our hands dirty with some code. First, we need to import Pandas. Then, we’ll set up our project ID and the number of samples we want.
import pandas as pd
project_id = '[your Cloud Platform project ID]'
sample_count = 2000
Counting Rows and Sampling Data
Let’s find out how many rows we’re dealing with in the gsod
table:
row_count = pd.io.gbq.read_gbq('''
SELECT
COUNT(*) as total
FROM `bigquery-public-data.samples.gsod`
''', project_id=project_id).total[0]
Now, let’s grab a sample of the data:
df = pd.io.gbq.read_gbq(f'''
SELECT
*
FROM
`bigquery-public-data.samples.gsod`
WHERE RAND() < {sample_count}/{row_count}
''', project_id=project_id)
And print out the total rows:
print(f'Full dataset has {row_count} rows')
Describe the Data
Get a quick overview of our sampled data:
df.describe()
Cool Facts
Did you know the GSOD sample table has weather data collected by NOAA from late 1929 to early 2010? That’s like, almost a century of weather!
Also, here’s a handy link to the Pandas GBQ Documentation for all your geeky needs.
Diving Deeper with BigQuery and Cloud API
Ready for round two? Let’s see how we can work with BigQuery directly using the Cloud API.
Authenticate Again (Just to be Safe)
from google.colab import auth
auth.authenticate_user()
Import BigQuery and List Datasets
Let’s bring in BigQuery and see what datasets we have:
from google.cloud import bigquery
project_id = '[your Cloud Platform project ID]'
client = bigquery.Client(project=project_id)
for dataset in client.list_datasets():
print(dataset.dataset_id)
Interactive Tables with Colab
Want to make your tables interactive? Of course you do! Load the google.colab.data_table
extension:
%load_ext google.colab.data_table
Now, when you display dataframes, they’ll be all fancy and interactive:
from vega_datasets import data
data.cars()
Want to switch back to plain old tables? No problem:
%unload_ext google.colab.data_table
data.cars()
BigQuery DataFrames
Let’s play with bigframes
, which makes BigQuery feel more like Pandas. First, authenticate:
from google.colab import auth
auth.authenticate_user()
Set Up
Set your project ID and import bigframes
:
project_id = '[your project ID]'
import bigframes.pandas as bpd
from google.cloud import bigquery
bpd.options.bigquery.project = project_id
bpd.options.bigquery.location = "US"
Load and Query Data
Create a query and load data with BigFrames:
from google.colab import syntax
query = syntax.sql('''
SELECT *
FROM `bigquery-public-data.ml_datasets.penguins`
LIMIT 20
''')
bq_df = bpd.read_gbq(query)
Describe and View Data
Get a quick summary and peek at the first 10 rows:
bq_df.describe()
bq_df.head(10)
And there you have it, folks! You’ve just conquered BigQuery using both Pandas and the Cloud API. Now go forth and analyze all the data! 🕵️♀️📊
Advanced BigQuery with Pandas and Cloud API
Hey there, data wizard! Ready to level up your BigQuery game? Let’s dive deeper with more tricks and tips.
Authenticate and Import
First, the usual drill: authenticate and import the necessary libraries.
from google.colab import auth
auth.authenticate_user()
import pandas as pd
import bigframes.pandas as bpd
from google.cloud import bigquery
Set up your project ID:
project_id = '[your Cloud Platform project ID]'
bpd.options.bigquery.project = project_id
bpd.options.bigquery.location = "US"
Running More Complex Queries
Let’s run a more complex query on a different dataset. We’ll use the natality
dataset from bigquery-public-data.samples
.
query = '''
SELECT
year,
COUNT(*) as birth_count
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
GROUP BY year
ORDER BY year
'''
df = pd.io.gbq.read_gbq(query, project_id=project_id)
print(df)
Visualizing the Data
Let’s visualize the birth counts over the years using Matplotlib.
import matplotlib.pyplot as plt
df.plot(kind='bar', x='year', y='birth_count', legend=False)
plt.title('Birth Counts by Year')
plt.xlabel('Year')
plt.ylabel('Birth Count')
plt.show()
Loading Data Directly from a Table
If you just want to load data directly from a table without running a query, you can do that too.
df_direct = pd.io.gbq.read_gbq('''
SELECT
*
FROM `bigquery-public-data.samples.natality`
LIMIT 100
''', project_id=project_id)
print(df_direct.head())
Using BigQuery Client for More Control
Sometimes, you might need more control over your queries and results. Let’s use the BigQuery Client for that.
client = bigquery.Client(project=project_id)
query_job = client.query('''
SELECT
weight_pounds,
state,
year
FROM `bigquery-public-data.samples.natality`
WHERE year = 2005
ORDER BY weight_pounds DESC
LIMIT 10
''')
results = query_job.result()
for row in results:
print(f'Baby weight: {row.weight_pounds} lbs, State: {row.state}, Year: {row.year}')
Writing Data to BigQuery
You can also write data back to BigQuery. Let’s create a new dataset and table, and insert some sample data.
dataset_id = 'my_new_dataset'
dataset = bigquery.Dataset(f'{project_id}.{dataset_id}')
dataset = client.create_dataset(dataset, exists_ok=True)
table_id = 'my_new_table'
schema = [
bigquery.SchemaField('name', 'STRING'),
bigquery.SchemaField('age', 'INTEGER'),
]
table = bigquery.Table(f'{project_id}.{dataset_id}.{table_id}', schema=schema)
table = client.create_table(table, exists_ok=True)
rows_to_insert = [
{"name": "John", "age": 30},
{"name": "Jane", "age": 25},
]
errors = client.insert_rows_json(table, rows_to_insert)
if not errors:
print('New rows have been added.')
else:
print('Errors occurred while inserting rows: {}'.format(errors))
Using BigFrames for Larger Data
BigFrames can be super helpful for handling larger datasets efficiently.
query = '''
SELECT
year,
month,
day,
MAX(mean_temp) as max_temp
FROM `bigquery-public-data.samples.gsod`
GROUP BY year, month, day
ORDER BY max_temp DESC
LIMIT 100
'''
bq_df = bpd.read_gbq(query)
print(bq_df.head())
Aggregating and Summarizing Data
Let’s perform some aggregation and summarization on the gsod
dataset.
query = '''
SELECT
station_number,
COUNT(*) as observation_count,
AVG(mean_temp) as avg_temp
FROM `bigquery-public-data.samples.gsod`
GROUP BY station_number
ORDER BY observation_count DESC
LIMIT 10
'''
agg_df = pd.io.gbq.read_gbq(query, project_id=project_id)
print(agg_df)
Joining Multiple Tables
You can join multiple tables in your queries too. Let’s join the natality
dataset with another table.
query = '''
SELECT
n.year,
n.weight_pounds,
s.state_name
FROM `bigquery-public-data.samples.natality` as n
JOIN `bigquery-public-data.utility_us.states` as s
ON n.state = s.state_abbreviation
WHERE n.year = 2000
LIMIT 10
'''
join_df = pd.io.gbq.read_gbq(query, project_id=project_id)
print(join_df)
Advanced BigQuery Techniques with Pandas and Cloud API
Hey data enthusiast! Ready to kick things up a notch? Let’s explore some advanced BigQuery techniques to make your data analysis even more powerful.
Authenticate and Import Libraries
First, let’s authenticate and import the necessary libraries as usual:
from google.colab import auth
auth.authenticate_user()
import pandas as pd
import bigframes.pandas as bpd
from google.cloud import bigquery
project_id = '[your Cloud Platform project ID]'
bpd.options.bigquery.project = project_id
bpd.options.bigquery.location = "US"
client = bigquery.Client(project=project_id)
Window Functions
Window functions are great for performing operations across a set of table rows related to the current row. Let’s use a window function to calculate a running total of birth counts by year.
query = '''
SELECT
year,
COUNT(*) OVER (ORDER BY year) as running_total
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
GROUP BY year
ORDER BY year
'''
df_window = pd.io.gbq.read_gbq(query, project_id=project_id)
print(df_window)
Handling Large Datasets Efficiently
For large datasets, using BigQuery’s storage and processing power efficiently is crucial. Use BigQuery’s LIMIT
and pagination to handle large results.
query = '''
SELECT
*
FROM `bigquery-public-data.samples.gsod`
LIMIT 10000
'''
df_large = pd.io.gbq.read_gbq(query, project_id=project_id)
print(df_large.head())
User-Defined Functions (UDFs)
UDFs allow you to write custom JavaScript functions for more complex operations. Let’s create a simple UDF to categorize birth weights.
query = '''
CREATE TEMP FUNCTION categorize_weight(weight FLOAT64)
RETURNS STRING
LANGUAGE js AS """
if (weight < 5) return 'Low';
else if (weight >= 5 && weight <= 8) return 'Normal';
else return 'High';
""";
SELECT
weight_pounds,
categorize_weight(weight_pounds) as weight_category
FROM `bigquery-public-data.samples.natality`
LIMIT 100
'''
df_udf = pd.io.gbq.read_gbq(query, project_id=project_id)
print(df_udf)
BigQuery ML for Machine Learning
BigQuery ML lets you build and deploy machine learning models using SQL. Let’s create a simple linear regression model to predict birth weights.
query = '''
CREATE OR REPLACE MODEL `my_project.my_dataset.birth_weight_model`
OPTIONS(model_type='linear_regression') AS
SELECT
weight_pounds,
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
'''
client.query(query).result()
# Evaluate the model
eval_query = '''
SELECT
*
FROM
ML.EVALUATE(MODEL `my_project.my_dataset.birth_weight_model`,
(
SELECT
weight_pounds,
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
))
'''
df_eval = pd.io.gbq.read_gbq(eval_query, project_id=project_id)
print(df_eval)
Optimizing Queries with Partitioning and Clustering
Partitioning and clustering can significantly speed up queries on large datasets. Let’s create a partitioned and clustered table for the natality
dataset.
# Create a partitioned table
create_table_query = '''
CREATE TABLE `my_project.my_dataset.natality_partitioned`
PARTITION BY DATE(_PARTITIONTIME)
CLUSTER BY state
AS
SELECT * FROM `bigquery-public-data.samples.natality`
'''
client.query(create_table_query).result()
# Query the partitioned table
partition_query = '''
SELECT
state,
COUNT(*) as birth_count
FROM `my_project.my_dataset.natality_partitioned`
WHERE _PARTITIONTIME BETWEEN '2000-01-01' AND '2010-12-31'
GROUP BY state
ORDER BY birth_count DESC
'''
df_partition = pd.io.gbq.read_gbq(partition_query, project_id=project_id)
print(df_partition)
Time Travel Queries
BigQuery’s time travel feature lets you query historical data from up to 7 days ago. This is useful for data recovery and auditing.
# Query data from 1 day ago
time_travel_query = '''
SELECT
*
FROM `my_project.my_dataset.natality_partitioned`
FOR SYSTEM_TIME AS OF TIMESTAMP_SUB(CURRENT_TIMESTAMP(), INTERVAL 1 DAY)
LIMIT 10
'''
df_time_travel = pd.io.gbq.read_gbq(time_travel_query, project_id=project_id)
print(df_time_travel)
Using External Data Sources
BigQuery can also query external data sources like Google Sheets. Let’s query a Google Sheet from BigQuery.
# Set up the external data source (assuming the Google Sheet is accessible)
external_query = '''
SELECT
*
FROM EXTERNAL_QUERY(
"my_project.my_dataset",
"SELECT * FROM `spreadsheet_id.sheet_name`"
)
'''
df_external = pd.io.gbq.read_gbq(external_query, project_id=project_id)
print(df_external)
And there you have it! These advanced techniques should help you take full advantage of BigQuery’s capabilities, from efficient data handling and custom functions to machine learning and more.
Advanced BigQuery Techniques in Python
Hey there, data aficionado! Ready to push your BigQuery skills to the max? Let’s dive into some advanced features and tricks to make your data analysis even more powerful and efficient.
Authenticate and Import Libraries
First, the usual setup: authenticate and import the necessary libraries.
from google.colab import auth
auth.authenticate_user()
from google.cloud import bigquery
import pandas as pd
project_id = '[your Cloud Platform project ID]'
client = bigquery.Client(project=project_id)
Parameterized Queries
Parameterized queries help prevent SQL injection and make your queries more dynamic.
query = '''
SELECT
name,
birth_year
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = @state
AND gender = @gender
LIMIT 100
'''
job_config = bigquery.QueryJobConfig(
query_parameters=[
bigquery.ScalarQueryParameter("state", "STRING", "TX"),
bigquery.ScalarQueryParameter("gender", "STRING", "F"),
]
)
df_param = client.query(query, job_config=job_config).to_dataframe()
print(df_param)
Streaming Inserts
BigQuery supports streaming inserts, allowing real-time data ingestion.
table_id = 'my_dataset.my_table'
rows_to_insert = [
{"name": "Alice", "age": 30},
{"name": "Bob", "age": 25},
]
errors = client.insert_rows_json(table_id, rows_to_insert)
if errors:
print(f'Errors occurred while inserting rows: {errors}')
else:
print('Rows successfully inserted.')
Google Cloud Storage Integration
Load data from Google Cloud Storage (GCS) directly into BigQuery.
dataset_id = 'my_dataset'
table_id = 'my_table'
uri = 'gs://my-bucket/my-file.csv'
job_config = bigquery.LoadJobConfig(
source_format=bigquery.SourceFormat.CSV,
skip_leading_rows=1,
autodetect=True,
)
load_job = client.load_table_from_uri(
uri, f'{project_id}.{dataset_id}.{table_id}', job_config=job_config
)
load_job.result() # Waits for the job to complete.
print('Data loaded successfully.')
Geospatial Analysis
BigQuery has robust support for geospatial data. Let’s perform some geospatial analysis.
query = '''
SELECT
state_name,
ST_CENTROID(geo) AS center
FROM `bigquery-public-data.geo_us_boundaries.states`
'''
df_geo = client.query(query).to_dataframe()
print(df_geo)
Managing Jobs and Datasets
Use the BigQuery client library to manage jobs and datasets programmatically.
Listing Datasets
datasets = list(client.list_datasets())
if datasets:
print('Datasets in project {}:'.format(project_id))
for dataset in datasets:
print('\t{}'.format(dataset.dataset_id))
else:
print('{} project does not contain any datasets.'.format(project_id))
Listing Tables in a Dataset
dataset_id = 'my_dataset'
tables = list(client.list_tables(dataset_id))
if tables:
print('Tables in dataset {}:'.format(dataset_id))
for table in tables:
print('\t{}'.format(table.table_id))
else:
print('Dataset {} does not contain any tables.'.format(dataset_id))
Exporting Query Results to GCS
query = '''
SELECT
name,
birth_year
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = 'TX'
LIMIT 100
'''
job_config = bigquery.QueryJobConfig(
destination=f'gs://my-bucket/query_results.csv',
write_disposition=bigquery.WriteDisposition.WRITE_TRUNCATE
)
query_job = client.query(query, job_config=job_config)
query_job.result() # Wait for the job to complete.
print('Query results exported to GCS.')
Scheduling Queries with Cloud Scheduler
Use Google Cloud Scheduler to automate query execution. First, create a Cloud Function to run your query, then use Cloud Scheduler to trigger it.
Cloud Function Code (example)
# This code should be deployed as a Google Cloud Function.
def run_query(request):
from google.cloud import bigquery
client = bigquery.Client()
query = '''
SELECT
COUNT(*)
FROM `bigquery-public-data.samples.gsod`
'''
query_job = client.query(query)
results = query_job.result()
for row in results:
print(f'Total rows: {row[0]}')
return 'Query executed successfully.'
Using BigQuery ML for Machine Learning
Train and use machine learning models directly within BigQuery.
Train a Model
query = '''
CREATE OR REPLACE MODEL `my_dataset.birth_weight_model`
OPTIONS(model_type='linear_regression') AS
SELECT
weight_pounds,
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
'''
client.query(query).result()
print('Model trained successfully.')
Predict with the Model
query = '''
SELECT
predicted_weight_pounds
FROM
ML.PREDICT(MODEL `my_dataset.birth_weight_model`,
(
SELECT
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year = 2008
))
'''
df_predictions = client.query(query).to_dataframe()
print(df_predictions.head())
Advanced BigQuery Techniques in R
Hey there, data explorer! 🌟 Are you ready to level up your data analysis skills using R and Google BigQuery?
If you’re already familiar with the basics, it’s time to dive into some advanced techniques that will supercharge your data projects.
Setup: Authenticate and Load Libraries
First, you need to authenticate and load the necessary libraries.
# Install necessary packages
install.packages("bigrquery")
install.packages("dplyr")
# Load libraries
library(bigrquery)
library(dplyr)
# Authenticate with Google Cloud
bq_auth()
# Set your project ID
project_id <- "[your Cloud Platform project ID]"
Parameterized Queries
Parameterized queries help prevent SQL injection and make your queries more dynamic.
query <- "
SELECT
name,
birth_year
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = @state
AND gender = @gender
LIMIT 100
"
params <- list(
state = "TX",
gender = "F"
)
df_param <- bq_project_query(project_id, query, parameters = params) %>%
bq_table_download()
print(df_param)
Handling Large Datasets Efficiently
For large datasets, use LIMIT
and pagination to handle large results efficiently.
query <- "
SELECT
*
FROM `bigquery-public-data.samples.gsod`
LIMIT 10000
"
df_large <- bq_project_query(project_id, query) %>%
bq_table_download()
print(head(df_large))
User-Defined Functions (UDFs)
UDFs allow you to write custom JavaScript functions for more complex operations. Here’s how to use a UDF to categorize birth weights.
query <- "
CREATE TEMP FUNCTION categorize_weight(weight FLOAT64)
RETURNS STRING
LANGUAGE js AS \"
if (weight < 5) return 'Low';
else if (weight >= 5 && weight <= 8) return 'Normal';
else return 'High';
\";
SELECT
weight_pounds,
categorize_weight(weight_pounds) as weight_category
FROM `bigquery-public-data.samples.natality`
LIMIT 100
"
df_udf <- bq_project_query(project_id, query) %>%
bq_table_download()
print(df_udf)
BigQuery ML for Machine Learning
BigQuery ML allows you to build and deploy machine learning models using SQL. Let’s create a simple linear regression model to predict birth weights.
# Train the model
query <- "
CREATE OR REPLACE MODEL `my_project.my_dataset.birth_weight_model`
OPTIONS(model_type='linear_regression') AS
SELECT
weight_pounds,
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
"
bq_project_query(project_id, query)
# Evaluate the model
eval_query <- "
SELECT
*
FROM
ML.EVALUATE(MODEL `my_project.my_dataset.birth_weight_model`,
(
SELECT
weight_pounds,
gestation_weeks
FROM `bigquery-public-data.samples.natality`
WHERE year > 2000
))
"
df_eval <- bq_project_query(project_id, eval_query) %>%
bq_table_download()
print(df_eval)
Google Cloud Storage Integration
Load data from Google Cloud Storage (GCS) directly into BigQuery.
# Load data from GCS
dataset_id <- 'my_dataset'
table_id <- 'my_table'
uri <- 'gs://my-bucket/my-file.csv'
load_job <- bq_table_load(paste(project_id, dataset_id, table_id, sep = "."),
source_uris = uri,
source_format = "CSV",
skip_leading_rows = 1,
autodetect = TRUE)
bq_job_wait(load_job)
print('Data loaded successfully.')
Geospatial Analysis
BigQuery supports geospatial data analysis. Let’s perform some geospatial queries.
query <- "
SELECT
state_name,
ST_CENTROID(geo) AS center
FROM `bigquery-public-data.geo_us_boundaries.states`
"
df_geo <- bq_project_query(project_id, query) %>%
bq_table_download()
print(df_geo)
Managing Jobs and Datasets
Use the BigQuery client library to manage jobs and datasets programmatically.
Listing Datasets
datasets <- bq_project_datasets(project_id)
print(datasets)
Listing Tables in a Dataset
dataset_id <- 'my_dataset'
tables <- bq_dataset_tables(bq_dataset(project_id, dataset_id))
print(tables)
Exporting Query Results to GCS
query <- "
SELECT
name,
birth_year
FROM `bigquery-public-data.usa_names.usa_1910_2013`
WHERE state = 'TX'
LIMIT 100
"
export_uri <- "gs://my-bucket/query_results.csv"
export_job <- bq_table_save(
x = bq_project_query(project_id, query),
destination_uri = export_uri,
destination_format = "CSV"
)
bq_job_wait(export_job)
print('Query results exported to GCS.')
Scheduling Queries with Cloud Scheduler
Use Google Cloud Scheduler to automate query execution. First, create a Cloud Function to run your query, then use Cloud Scheduler to trigger it.
Cloud Function Code (example)
# This code should be deployed as a Google Cloud Function.
def run_query(request):
from google.cloud import bigquery
client = bigquery.Client()
query = '''
SELECT
COUNT(*)
FROM `bigquery-public-data.samples.gsod`
'''
query_job = client.query(query)
results = query_job.result()
for row in results:
print(f'Total rows: {row[0]}')
return 'Query executed successfully.'
What is BigQuery?
Google BigQuery is a fully-managed, serverless data warehouse that allows you to analyze petabytes of data with high-speed SQL queries. It’s part of the Google Cloud Platform (GCP) and is designed to handle very large datasets efficiently. Whether you’re dealing with structured or semi-structured data, BigQuery provides a fast, scalable, and cost-effective solution.
Why Use R with BigQuery?
R is a powerful statistical programming language that is widely used for data analysis, visualization, and machine learning. By combining R with BigQuery, you get the best of both worlds: the advanced analytical capabilities of R and the scalability and speed of BigQuery.
This integration allows you to handle large datasets, perform complex queries, and leverage machine learning directly within your R environment.
Setting Up BigQuery in R
Before we dive into advanced techniques, let’s set up our environment. You’ll need a Google Cloud project and the bigrquery
package in R to get started.
Step-by-Step Setup
- Create a Google Cloud Project: If you don’t already have one, use the Cloud Resource Manager to create a new project.
- Enable Billing: Make sure billing is enabled for your project.
- Enable BigQuery API: Enable the BigQuery API for your project from the API Library.
- Install and Load the BigQuery Library in R:
install.packages("bigrquery")
library(bigrquery)
- Authenticate:
# Authenticate with Google Cloud
bq_auth()
- Set Your Project ID:
project_id <- "[your Cloud Platform project ID]"
Advanced Techniques
With your environment set up, you’re ready to explore some advanced BigQuery techniques using R. Here’s what we’ll cover:
1. Parameterized Queries
Parameterized queries help prevent SQL injection and make your queries more dynamic. We’ll see how to run queries with parameters in BigQuery using R.
2. Handling Large Datasets Efficiently
BigQuery is designed to handle massive datasets. Learn how to efficiently manage large data queries and pagination in R.
3. User-Defined Functions (UDFs)
UDFs allow you to write custom JavaScript functions for more complex operations. We’ll create a UDF to categorize data within your queries.
4. BigQuery ML for Machine Learning
BigQuery ML lets you build and deploy machine learning models directly within BigQuery using SQL. We’ll create a simple model and make predictions.
5. Google Cloud Storage Integration
Load data from Google Cloud Storage (GCS) directly into BigQuery. This section will guide you through integrating GCS with BigQuery in R.
6. Geospatial Analysis
BigQuery supports robust geospatial data analysis. We’ll perform geospatial queries to analyze location-based data.
7. Managing Jobs and Datasets
Use the BigQuery client library to manage jobs and datasets programmatically in R. This includes listing datasets and tables, and managing BigQuery jobs.
8. Scheduling Queries with Cloud Scheduler
Automate your queries using Google Cloud Scheduler and Cloud Functions to ensure your data is always up to date.
Why Use BigQuery with Pandas, Python, and R?
- Pandas and Python: Pandas is a fast, powerful, and flexible data analysis library in Python. Combining Pandas with BigQuery allows you to leverage Python’s extensive ecosystem for data manipulation, analysis, and machine learning, while BigQuery handles the heavy lifting of large-scale data processing.
- R: R is a language and environment specifically designed for statistical computing and graphics. It’s widely used for data analysis and visualization. Integrating R with BigQuery gives you the ability to perform advanced statistical analyses and create detailed visualizations on massive datasets.
Certainly! Let’s expand on each question with more detailed explanations, tables, lists, and paragraphs.
Is BigQuery a SQL or NoSQL?
When talking about BigQuery, it’s crucial to grasp its underlying architecture and the kind of data it deals with. BigQuery functions primarily as a SQL database, meaning it’s designed for querying and analyzing structured data using SQL syntax.
Structured data is data organized into tables with predefined schemas, making it ideal for relational databases.
While BigQuery is great at handling structured data and running complex SQL queries, it’s important to mention that it also supports semi-structured and unstructured data to some extent.
Nevertheless, its main focus is on structured data analysis, aligning it more closely with SQL databases rather than NoSQL databases.
To illustrate this point further, let’s compare the characteristics of BigQuery with typical features associated with SQL and NoSQL databases:
BigQuery | SQL Databases | NoSQL Databases |
---|---|---|
Optimized for querying and analyzing structured data using SQL syntax. | Ideal for structured data storage and manipulation, with support for transactions and relational integrity. | Suited for flexible schema design and scalability, commonly used for handling large volumes of unstructured or semi-structured data. |
Provides scalability, efficiency, and advanced analytics capabilities for big data analysis. | Offers ACID (Atomicity, Consistency, Isolation, Durability) properties to ensure data integrity and transactional consistency. | Designed for eventual consistency and horizontal scalability, allowing for distributed data storage and processing. |
Structured data is organized into tables with predefined schemas, facilitating efficient querying and analysis. | Data is stored in tables with well-defined schemas, enabling efficient querying and indexing for performance optimization. | Supports flexible schema design, allowing for dynamic changes to the data model without requiring predefined schemas. |
While BigQuery’s architecture and capabilities align it more closely with SQL databases, it’s essential to recognize that it also supports some aspects of NoSQL databases, particularly in handling semi-structured and unstructured data formats. However, its primary focus remains on structured data analysis using SQL queries. |
What is BigQuery vs SQL?
To gain a deeper understanding of BigQuery and its relationship to SQL, let’s explore the characteristics and functionalities of each:
BigQuery
- Definition: BigQuery is a fully managed, serverless data warehouse provided by Google Cloud Platform. It is designed for analyzing large datasets using SQL queries and offers scalability, efficiency, and advanced analytics capabilities for big data analysis.
- Features:
- Serverless: No infrastructure management required; Google Cloud handles the infrastructure provisioning, scaling, and maintenance.
- Scalable: Can handle petabyte-scale datasets with high performance and reliability.
- SQL-based: Uses SQL syntax for querying and analyzing data, making it accessible to users familiar with SQL.
- Integration: Seamlessly integrates with other Google Cloud services and third-party tools for data ingestion, processing, and visualization.
- Advanced Analytics: Supports advanced analytics features such as machine learning (BigQuery ML), geospatial analysis, and data visualization.
- Use Cases: Commonly used for business intelligence, data warehousing, ad hoc analysis, and machine learning applications.
SQL (Structured Query Language)
- Definition: SQL is a standardized programming language used for managing and manipulating relational databases. It provides a set of commands and syntax for querying, inserting, updating, and deleting data within tables.
- Features:
- Data Manipulation: Enables users to perform various operations on relational databases, including selecting, filtering, aggregating, joining, and sorting data.
- Data Definition: Supports defining the structure of databases, tables, and indexes, specifying constraints, and managing schema changes.
- Data Control: Provides mechanisms for controlling access to data through user permissions, roles, and privileges.
- Transaction Management: Offers support for transactions to ensure data integrity and consistency during database operations.
- Use Cases: Widely used in various industries and applications for data management, reporting, analytics, and transaction processing.
While BigQuery and SQL are closely related, it’s important to recognize that BigQuery utilizes SQL as its primary language for querying and analysis. BigQuery abstracts away the complexities of managing infrastructure, allowing users to focus on querying and analyzing data using familiar SQL syntax.
Is BigQuery a SQL language?
BigQuery utilizes SQL (Structured Query Language) as its main language for querying and analyzing data. Users can interact with BigQuery by using SQL syntax to carry out operations like selecting, filtering, aggregating, and joining data in tables.
- SQL offers a user-friendly and robust interface for querying structured data, catering to a wide range of users from data analysts to data scientists.
- By utilizing SQL as its query language, BigQuery provides a familiar and robust platform for analyzing large datasets.
Users can create intricate queries to uncover valuable insights from their data, whether it’s for business intelligence reporting, ad hoc analysis, or machine learning applications.
Hence, BigQuery can be accurately described as a SQL-centric platform, with SQL serving as the foundation of its querying capabilities.
In essence, BigQuery’s integration of SQL empowers users to leverage the potential of SQL queries for analyzing structured data on a large scale, making it a versatile and potent tool for data analytics and exploration.
Conclusion
Congratulations on completing Lesson 6! 🎉 You’ve delved into advanced BigQuery techniques using Pandas in Python and R, equipping yourself with powerful tools for data analysis. Here’s a quick recap:
- Environment Setup: You’ve learned to set up your environment, authenticate, and import necessary libraries in both Python and R.
- Advanced Techniques: From parameterized queries to geospatial analysis, you’ve explored a range of advanced techniques to handle large datasets, perform complex operations, and leverage machine learning directly within BigQuery.
- Why This Matters: Combining BigQuery with Pandas, Python, and R provides scalability, efficiency, flexibility, and advanced analytics capabilities, empowering you to derive deeper insights from your data.
- Next Steps: Put your new skills into practice by building data pipelines, analyzing real-world datasets, deploying machine learning models, or creating interactive dashboards.
Congratulations again, and happy querying! 🚀
1 Comment
Machine Learning Project 1: Honda Motor Stocks Best Prices · May 23, 2024 at 6:59 pm
[…] Lesson 6: Best BigQuery with Pandas Python and R Guide […]