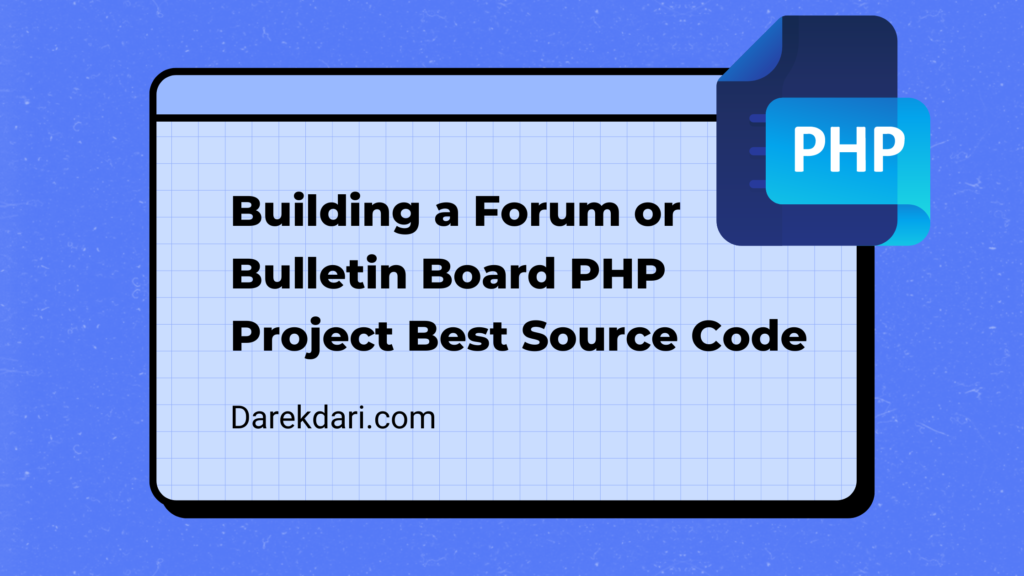
Table of Contents
So, you’re thinking about diving into the world of web development and creating your very own forum or bulletin board, eh?
PHP Projects:
- Quiz Application in PHP
- PHP Image Gallery Project Source Code
- Best 200 Questions Game in PHP
- Bulletin Board PHP Project
- Content Management System (CMS) PhP Project
- Event Management System Project in PHP
- Personal Blog with PHP
- Chatbot in PHP
- URL Shortenee in php
Well, you’re in for an exciting ride! Forums have been the backbone of online communities for decades, serving as hubs for discussions, information sharing, and community building.
In this guide, we’ll walk you through the process of building a forum or bulletin board using PHP, one of the most versatile and widely used programming languages for web development.
Understand the Importance of Forums and Bulletin Boards π
Forums and bulletin boards play a crucial role in fostering online communities. Whether it’s discussing the latest tech trends, sharing cooking recipes, or seeking advice on pet care, forums provide a platform for like-minded individuals to connect and engage in meaningful conversations.
From troubleshooting technical issues to debating hot topics, forums facilitate interaction and knowledge exchange on a global scale.
Why Choose PHP for Your Project? π‘
PHP (Hypertext Preprocessor) is a server-side scripting language that’s tailor-made for web development. Its simplicity, versatility, and extensive community support make it an ideal choice for building dynamic websites and web applications. With PHP, you can seamlessly integrate with databases, handle user authentication, and create interactive web interfaces. Plus, there’s a plethora of frameworks and libraries available to expedite development and enhance functionality.
photo gallery php
php exercises
php exercises and solutions
php exercises and solutions pdf
php exercises online
php lab exercises
php mvc framework from scratch
php mvc from scratch
Source Code of Forum or Bulletin Board Project π οΈ
So, how do you kickstart your journey into forum development? Let’s break it down into manageable steps:
Step 1: Database Design π
First things first, you’ll need to design the database schema to store user information, forum categories, topics, posts, and more. Think of it as laying the foundation for your virtual community. Here’s a glimpse of what your database structure might look like:
- Users table: Store user details such as username, email, password (encrypted, of course!), and registration date.
- Categories table: Define different categories for organizing discussions, e.g., Technology, Sports, Food & Drink.
- Topics table: Keep track of individual discussion topics within each category.
- Posts table: Store the actual messages posted by users, along with timestamps and topic associations.
CREATE TABLE users (
user_id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
password VARCHAR(255) NOT NULL,
registration_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE categories (
category_id INT AUTO_INCREMENT PRIMARY KEY,
category_name VARCHAR(100) NOT NULL
);
CREATE TABLE topics (
topic_id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
content TEXT NOT NULL,
category_id INT,
user_id INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (category_id) REFERENCES categories(category_id),
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
CREATE TABLE posts (
post_id INT AUTO_INCREMENT PRIMARY KEY,
content TEXT NOT NULL,
topic_id INT,
user_id INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (topic_id) REFERENCES topics(topic_id),
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
When it comes to creating a bulletin board, a well-structured database is essential to manage user data, categories, topics, and posts effectively. Let’s see how you can design your tables to accommodate the requirements of a bulletin board.
Step 2: User Authentication and Management π
Next up, implement user authentication to ensure that only registered users can participate in forum discussions. You’ll need to create registration and login forms, encrypt passwords before storing them in the database, and set up sessions to keep track of logged-in users.
Registration (register.php)
<?php
include_once 'db_connect.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = $_POST['username'];
$email = $_POST['email'];
$password = password_hash($_POST['password'], PASSWORD_DEFAULT);
$sql = "INSERT INTO users (username, email, password) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sss", $username, $email, $password);
if ($stmt->execute()) {
echo "Registration successful!";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
?>
Security is paramount here, so make sure to follow best practices to safeguard user credentials and sensitive information.
Login (login.php)
<?php
session_start();
include_once 'db_connect.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$email = $_POST['email'];
$password = $_POST['password'];
$sql = "SELECT * FROM users WHERE email = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $email);
$stmt->execute();
$result = $stmt->get_result();
$user = $result->fetch_assoc();
if ($user && password_verify($password, $user['password'])) {
$_SESSION['user_id'] = $user['user_id'];
echo "Login successful!";
} else {
echo "Invalid email or password!";
}
}
?>
Step 3: Forum Structure and Functionality π
Now comes the fun part β building the actual forum structure and functionality. This involves creating CRUD (Create, Read, Update, Delete) operations for managing forum data, designing user interfaces for browsing categories and topics, and allowing users to create new topics and post replies.
Creating a New Topic (create_topic.php)
<?php
session_start();
include_once 'db_connect.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$title = $_POST['title'];
$content = $_POST['content'];
$category_id = $_POST['category_id'];
$user_id = $_SESSION['user_id'];
$sql = "INSERT INTO topics (title, content, category_id, user_id) VALUES (?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("ssii", $title, $content, $category_id, $user_id);
if ($stmt->execute()) {
echo "Topic created successfully!";
} else {
echo "Error creating topic!";
}
}
?>
Don’t forget to incorporate features like pagination, search functionality, and user profiles to enhance the user experience.
Posting a Reply (post_reply.php)
<?php
session_start();
include_once 'db_connect.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$content = $_POST['content'];
$topic_id = $_POST['topic_id'];
$user_id = $_SESSION['user_id'];
$sql = "INSERT INTO posts (content, topic_id, user_id) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sii", $content, $topic_id, $user_id);
if ($stmt->execute()) {
echo "Reply posted successfully!";
} else {
echo "Error posting reply!";
}
}
?>
Step 4: Frontend Interface Design and Styling π¨
A visually appealing and user-friendly interface is key to keeping users engaged on your forum. Utilize HTML, CSS, and JavaScript to design responsive and intuitive frontend components. Whether it’s designing sleek discussion threads or crafting interactive forms for posting replies, put yourself in the shoes of your users and prioritize usability and aesthetics.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Bulletin Board</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>Welcome to My Bulletin Board</h1>
<nav>
<ul>
<li><a href="index.php">Home</a></li>
<li><a href="create_topic.php">New Topic</a></li>
<li><a href="logout.php">Logout</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>Categories</h2>
<ul>
<li><a href="category.php?id=1">Technology</a></li>
<li><a href="category.php?id=2">Sports</a></li>
<li><a href="category.php?id=3">Food & Drink</a></li>
</ul>
</section>
<section>
<h2>Recent Topics</h2>
<ul>
<li><a href="topic.php?id=1">Topic 1</a></li>
<li><a href="topic.php?id=2">Topic 2</a></li>
<li><a href="topic.php?id=3">Topic 3</a></li>
</ul>
</section>
</main>
<footer>
<p>© 2024 My Bulletin Board</p>
</footer>
</body>
</html>

Step 5: Backend Logic and Security Measures π‘οΈ
Underneath the hood, your forum application needs robust backend logic to handle user actions, process form submissions, and interact with the database.
Backend Logic for User Actions (backend.php)
<?php
session_start();
include_once 'db_connect.php';
// Code for handling various user actions (e.g., creating topics, posting replies) goes here
?>
Implement server-side validation to sanitize user input, prevent SQL injection and cross-site scripting (XSS) attacks, and ensure data integrity at all times. Remember, security should never be an afterthought β bake it into your code from the get-go.
In this backend script, you can handle user actions such as creating topics, posting replies, editing/deleting posts, etc. Ensure that each action is properly validated and sanitized to prevent security vulnerabilities.
Security Measures (security.php)
<?php
// Function to sanitize user input
function sanitize_input($input) {
$input = trim($input);
$input = stripslashes($input);
$input = htmlspecialchars($input);
return $input;
}
// Function to verify user authentication
function verify_user($conn, $email, $password) {
$sql = "SELECT * FROM users WHERE email = ? AND password = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("ss", $email, $password);
$stmt->execute();
$result = $stmt->get_result();
return $result->fetch_assoc();
}
// Code for other security measures (e.g., CSRF protection, session management) goes here
?>
In this security script, you can implement functions for sanitizing user input, verifying user authentication, and other security measures like Cross-Site Request Forgery (CSRF) protection and session management.
Step 6: Testing, Debugging, and Deployment π
Last but not least, it’s time to put your forum through its paces! Thoroughly test each aspect of your application to identify and squash any bugs or issues that may arise. Leverage debugging tools like Xdebug for PHP to streamline the debugging process and ensure smooth operation.
Test Cases for Validation (test_debug.php)
<?php
// Test Case 1: Registration Functionality
// Simulate user registration and verify if the user is added to the database
function test_registration() {
// Your test code here
}
// Test Case 2: Login Functionality
// Simulate user login and verify if the correct user credentials are accepted
function test_login() {
// Your test code here
}
// Test Case 3: Posting a New Topic
// Simulate posting a new topic and verify if it appears in the topic list
function test_posting_topic() {
// Your test code here
}
// Invoke test cases
test_registration();
test_login();
test_posting_topic();
?>
Deployment Instructions (deployment.php)
<?php
// Step 1: Choose a Web Hosting Provider
// Select a reliable web hosting provider that meets your requirements and supports PHP and MySQL databases.
// Step 2: Upload Files
// Transfer your bulletin board files (PHP scripts, HTML/CSS files, images, etc.) to the web server using FTP or file manager provided by your hosting provider.
// Step 3: Import Database
// If you're using a MySQL database, import your database schema and data to the web server using phpMyAdmin or similar tools.
// Step 4: Configure Server Settings
// Adjust server settings (e.g., PHP configuration, file permissions) as necessary to ensure compatibility and security.
// Step 5: Test Deployment
// Verify that your bulletin board is functioning correctly on the live server by accessing it through a web browser and performing various actions.
// Step 6: Monitor Performance
// Monitor the performance of your bulletin board on the live server and address any issues or optimizations as needed to ensure optimal user experience.
// Step 7: Regular Maintenance
// Perform regular maintenance tasks such as updating software, backing up data, and monitoring security to keep your bulletin board running smoothly.
// Congratulations! Your bulletin board is now deployed and ready for users to engage with.
?>
In this script, you can provide step-by-step instructions for deploying your bulletin board to a web server, including choosing a hosting provider, uploading files, importing the database, configuring server settings, testing deployment, and ongoing maintenance.
Once you’re confident that everything is shipshape, deploy your forum application to a web server and make it accessible to the world. Don’t forget to configure server settings, set up backups, and monitor performance to keep your forum running smoothly.
By following these deployment instructions, you can make your bulletin board accessible to users and create a thriving online community. Remember to regularly monitor and maintain your bulletin board to ensure its continued success.
php mvc project source code
php photo database
php photo gallery
php project source code
php projects source code
php projects with source code
php real time application
php real time chat application
php realtime chat
Detailed Structure
Certainly! Let’s break down the detailed structure for a Forum or Bulletin Board web application using PHP:
Project Structure
- Database Design:
- Create tables for users, topics, posts, categories, etc.
- Define relationships between tables using foreign keys.
- User Authentication:
- Implement registration and login functionality.
- Encrypt passwords before storing them in the database.
- Use sessions for user authentication.
- Forum Structure:
- Design database schema to handle categories, topics, and posts.
- Implement CRUD operations for managing forum data.
- Frontend Interface:
- Create HTML templates for displaying forum categories, topics, and posts.
- Design forms for creating new topics and posting replies.
- Use CSS for styling the frontend components.
- Backend Logic:
- Implement PHP scripts to handle user actions such as posting new topics or replies.
- Validate user input to prevent SQL injection and XSS attacks.
- Use PHP functions to interact with the database.
- User Profile:
- Allow users to customize their profiles.
- Display user activity such as the number of posts and topics created.
- Search Functionality:
- Implement a search feature to allow users to search for topics or posts by keywords.
- Use SQL queries with LIKE operator for searching.
- Moderation Tools:
- Implement moderation tools for administrators.
- Allow moderators to delete inappropriate content and ban users.
- Security Measures:
- Use prepared statements and parameterized queries to prevent SQL injection.
- Use htmlspecialchars() or htmlentities() to escape user-generated content.
- Responsive Design:
- Make the forum interface responsive using CSS media queries.
- Ensure that the forum layout adjusts well on different devices and screen sizes.
- Error Handling:
- Implement error handling to display meaningful error messages to users.
- Log errors for debugging purposes.
- Testing and Debugging:
- Test the application thoroughly to identify and fix any bugs.
- Use debugging tools like Xdebug for PHP debugging.
- Deployment:
- Deploy the forum application to a web server.
- Configure server settings and permissions.
- Set up backups and monitoring for the deployed application.
Certainly! Let’s improve the code snippet to make it more robust and secure. We’ll also include error handling for better feedback:
<?php
// Assuming database connection is already established
// Function to create a new topic
function createTopic($conn, $title, $content, $categoryId, $userId) {
// Prepare the SQL statement
$sql = "INSERT INTO topics (title, content, category_id, user_id) VALUES (?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
// Bind parameters
$stmt->bind_param("ssii", $title, $content, $categoryId,
Bulletin Board Features Overview π
Let’s provide an overview of the key features of your bulletin board application using a table to present the information clearly:
Feature | Description |
---|---|
User Registration | Allow users to create accounts and access the bulletin board with unique usernames and emails |
User Authentication | Secure user authentication process using hashed passwords to protect user accounts |
Topic Creation | Enable users to create new discussion topics on various categories |
Post Replies | Allow users to post replies to existing topics, fostering engaging discussions |
Category Management | Organize topics into different categories for easy navigation and content organization |
User Profiles | Provide user profiles where users can view their activity, such as topics and posts |
Search Functionality | Implement a search feature to allow users to search for topics or posts by keywords |
Moderation Tools | Equip administrators with tools to manage and moderate content, including post deletion and user banning |
Responsive Design | Ensure the bulletin board interface adapts seamlessly to different devices and screen sizes |
Error Handling | Implement error handling to provide meaningful error messages to users in case of issues |
In this table, is talking about various features of your bulletin board application along with their descriptions. This overview will help users understand the functionality and capabilities of your bulletin board at a glance.
9 Comments
Creating Best PHP MVC Framework From Scratch 2025 · July 3, 2024 at 11:06 am
[…] Bulletin Board PHP Project […]
Best Booking System In PHP With Source Code 2024 · September 18, 2024 at 5:21 pm
[…] Bulletin Board PHP Project […]
How To Create A Chatbot In PHP 2024? Quick And Easy · September 18, 2024 at 5:26 pm
[…] Bulletin Board PHP Project […]
Event Management System Project In PHP 2 Best Source Code · September 18, 2024 at 5:28 pm
[…] Bulletin Board PHP Project […]
Build The Best Real Time Chat Application In PHP 2024 · October 9, 2024 at 7:50 pm
[…] Bulletin Board PHP Project […]
How To Build The Best Job Board With PHP 2024? · October 9, 2024 at 7:55 pm
[…] Bulletin Board PHP Project […]
Building The Best Quiz Application In PHP 2024 · October 9, 2024 at 7:57 pm
[…] Bulletin Board PHP Project […]
Best Social Networking Platform With PHP And React.js 2024 · October 9, 2024 at 7:58 pm
[…] Bulletin Board PHP Project […]
BesT Content Management System (CMS) PHP Project 2024 · October 9, 2024 at 7:59 pm
[…] Bulletin Board PHP Project […]