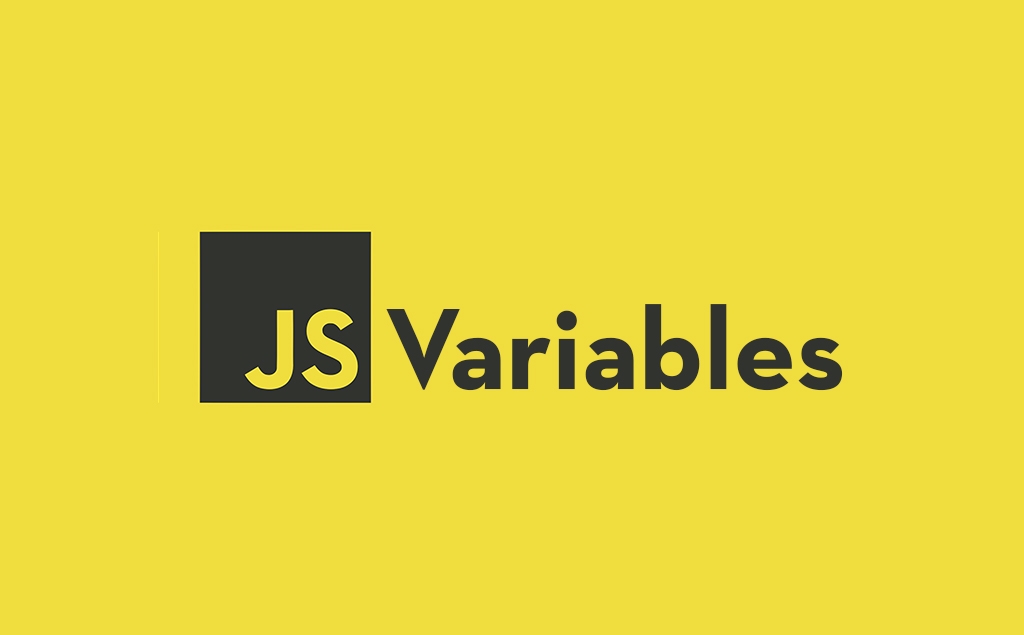
Introduction to Javascript variable :
In this blog, we’ll take you on a journey through ten practical exercises that cover a wide range of variable-related tasks. You’ll learn how to create, manipulate, and utilize variables effectively, giving you the skills you need to excel in JavaScript programming.
Whether you’re a newcomer to JavaScript or looking to brush up on your skills, these exercises and solutions will provide you with valuable insights and hands-on experience. So, let’s dive in and explore the world of JavaScript variables together!
Exercise 1: Understanding Javascript variables
let num1 = 5;
let num2 = 10;
if (num1 > num2) {
console.log("num1 is greater than num2.");
} else {
console.log("num2 is greater than or equal to num1.");
}
In this exercise, we create two variables, num1
and num2
, and use an if statement to compare them. If you’re not sure how this works, don’t worry; we’ll explain it in detail below.
Solution:
- We declare two variables,
num1
andnum2
, with numeric values. - An if statement is used to check if
num1
is greater thannum2
. - Depending on the comparison, we output the result using
console.log
.
Exercise 2: Looping Through Arrays
let myArray = [1, 2, 3, 4, 5];
for (let i = 0; i < myArray.length; i++) {
console.log(myArray[i]);
}
Arrays are essential in JavaScript, and this exercise of javascript variable will help you iterate through them and print their values to the console.
Solution:
- We create an array,
myArray
, with a list of numbers. - A for loop iterates through the array, and each value is printed using
console.log
.
Exercise 3: String Manipulation
let myString = "hello world";
let uppercaseString = myString.toUpperCase();
console.log(uppercaseString);
String manipulation is a common task in JavaScript, and this exercise javascript variable demonstrates how to convert a string to uppercase.
Solution:
- We create a string,
myString
. - The
toUpperCase()
method is applied to make the entire string uppercase. - The result is then displayed using
console.log
.
Exercise 4: Using Switch Statements
let num = 3;
switch (num) {
case 1:
console.log("One.");
break;
case 2:
console.log("Two.");
break;
case 3:
console.log("Three.");
break;
default:
console.log("Other.");
break;
}
Switch statements are excellent for handling multiple cases. This exercise shows you how to use them effectively.
Solution:
- We declare a variable,
num
, and assign it a numeric value. - A switch statement evaluates the value of
num
and executes the corresponding code block. - The
default
case is used for values that don’t match any defined cases.
Exercise 5: Joining Array Elements
let myArray = ["apple", "banana", "orange"];
let myString = myArray.join(", ");
console.log(myString);
In this exercise, we work with arrays and the .join()
method to concatenate elements into a single string.
Solution:
- We create an array,
myArray
, containing strings. - The
.join()
method combines the array elements with a comma and space, creating a string. - The resulting string is printed to the console.
Exercise 6: Looping with a For Loop
let num = 5;
for (let i = 1; i <= num; i++) {
console.log(i);
}
Looping is a fundamental concept in programming. This exercise of javascript variable demonstrates how to use a for
loop to print numbers.
Solution:
- We initialize a variable,
num
, with a numeric value. - A
for
loop iterates from 1 to the value ofnum
, printing each number to the console.
Exercise 7: Extracting Characters from a String
let myString = "hello world";
console.log(myString.charAt(0));
Learn how to extract individual characters from a string using the .charAt()
method in this exercise.
Solution:
- We define a string,
myString
. - The
.charAt(0)
method retrieves and outputs the first character of the string.
Exercise 8: Calculating the Sum of an Array
let myArray = [1, 2, 3, 4, 5];
let sum = myArray.reduce((acc, val) => acc + val, 0);
console.log(sum);
Arrays are often used to store and manipulate data. This exercise shows how to calculate the sum of all elements in an array using the .reduce()
method.
Solution:
- We create an array,
myArray
, with numeric values. - The
.reduce()
method is employed to accumulate the sum of the array elements. - The result is displayed using
console.log
.
Exercise 9: Iterating Through Object Key-Value Pairs
let myObject = { name: "John", age: 25, city: "New York" };
for (let key in myObject) {
console.log(`${key}: ${myObject[key]}`);
}
Objects are crucial in JavaScript, and this exercise of javascript variable teaches you how to iterate through an object’s key-value pairs using a for-in
loop.
Solution:
- We define an object,
myObject
, with key-value pairs. - A
for-in
loop iterates through the object, and each key-value pair is displayed.
Exercise 10: Printing Even Numbers with a While Loop
let num = 10;
let i = 2;
while (i <= num) {
console.log(i);
i += 2;
}
While loops are another way to control program flow. This exercise focuses on using a while
loop to print even numbers.
Solution:
- We set the value of
num
and initialize a counter,i
. - A
while
loop continues untili
is less than or equal tonum
. - Even numbers are printed, and
i
is incremented by 2 in each iteration.
Learn more
Conclusion of Javascript variable :
In conclusion, this blog has equipped you with the fundamental knowledge and practical skills required to work with JavaScript variables. These exercises and expert solutions serve as an excellent starting point on your journey to becoming a proficient JavaScript developer.
Frequently Asked Questions
1. What are variables in JavaScript?
Variables in JavaScript are containers for storing data values. They play a crucial role in programming, allowing you to work with different types of data such as numbers, strings, and objects.
2. How do I check if one variable is greater than another in JavaScript?
You can use an if
statement to compare two variables in JavaScript. If the condition inside the if
statement is true, you can execute a specific block of code.
3. What is the purpose of a for
loop?
A for
loop is used to repeatedly execute a block of code.
4. Where can I practice my JavaScript skills?
You can practice your JavaScript skills on platforms like Codecademy, freeCodeCamp, and LeetCode.
5. How to practice JavaScript methods?
Practice JavaScript methods through coding challenges, projects, and interactive tutorials.
6. How to practice JavaScript for beginners?
Beginners can start with online courses, tutorials, and coding exercises designed for novices.
7. How can I practice JavaScript daily?
To practice JavaScript daily, set aside dedicated time, work on small projects, and explore coding communities for support and inspiration.
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments