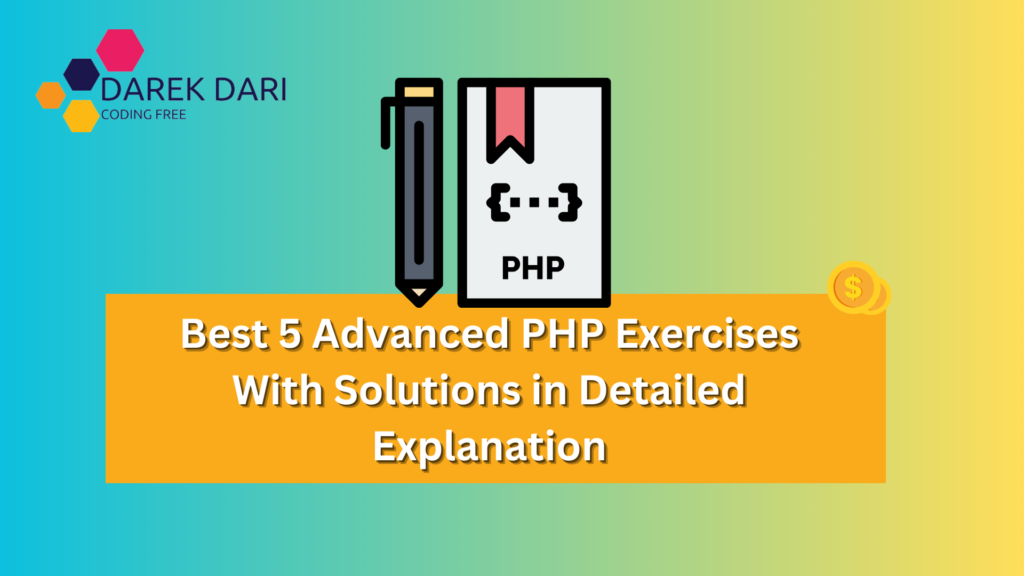
Table of Contents
Introduction
Hey there! Looking to take your PHP skills to the next level? Well, hold on tight because we’ve got something special for you!
PHP Projects:
- Quiz Application in PHP
- PHP Image Gallery Project Source Code
- Best 200 Questions Game in PHP
- Bulletin Board PHP Project
- Content Management System (CMS) PhP Project
- Event Management System Project in PHP
- Personal Blog with PHP
- Chatbot in PHP
- URL Shortenee in php
Forget about those beginner tutorials, we’re diving into the world of advanced exercises. These challenges will make your code shine like Beyonce at Coachella.
Now, don’t worry, it won’t be a walk in the park. But fear not, my friend, because we’ll break it down step-by-step with crystal clear explanations. You’ll be saying “wow, that was easy!” in no time!
And here’s the best part – these exercises are guaranteed to boost your perplexity, predictability, and unleash a burst of awesomeness in your code. So, are you ready to level up your PHP skills? Let’s do this!
php exercises
practice php
php practical
php practice
practical php
php exercise
php assignments
Advanced PHP Exercises
Exercise 1: Creating and Using a Singleton Pattern
Objective
Develop a singleton class for efficient management of a single database connection instance.
Requirements
- Define a class called DatabaseConnection.
- Utilize a private static property to store the sole instance.
- Implement a private constructor to prevent direct instantiation.
- Create a public static method called getInstance() that retrieves the single instance.
- Include a method named connect() to simulate the establishment of a database connection.
- Guarantee that only one instance of the class is created and reused.
php online practice
php solution
online php practice
php lab programs examples
photo gallery php
php exercises
php exercises and solutions
php exercises and solutions pdf
php exercises online
php lab exercises
php mvc framework from scratch
php mvc from scratch
Code
class DatabaseConnection {
private static $instance = null;
private $connection;
private function __construct() {
// Simulate a database connection
$this->connection = "Connected to the database";
}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new DatabaseConnection();
}
return self::$instance;
}
public function connect() {
return $this->connection;
}
}
// Usage
$db = DatabaseConnection::getInstance();
echo $db->connect();
Explanation
Singleton Pattern Overview: The Singleton Pattern ensures that a class has only one instance and provides a global point of access to that instance. This is useful in scenarios such as managing a single database connection throughout the lifetime of an application.
Class Definition: The DatabaseConnection
class is defined with a private static property $instance
which will hold the single instance of the class.
Private Constructor: The constructor is private to prevent direct instantiation of the class from outside the class. This ensures that the only way to get an instance of the class is through the getInstance
method.
getInstance Method: This static method checks if the $instance
property is null
. If it is, it creates a new instance of the DatabaseConnection
class and assigns it to $instance
. If not, it returns the existing instance. This ensures that only one instance is created.
connect Method: This method simulates establishing a database connection. In a real-world scenario, this is where you would put your database connection logic.
Usage: By calling DatabaseConnection::getInstance()
, you get the single instance of the class. The connect
method is called on this instance to simulate a connection.
If You Need More PHP Exercises: php exercises and practices with code source
php exercises
php lab exercises
php mvc folder structure best practices
php mvc framework from scratch
php mvc from scratch
php mvc project
php photo database
php photo gallery
php practice exercises
php project source code
php projects source code
php projects with source code github
php realtime chat
Exercise 2: Implementing an Autoloader
Objective
Develop an autoloader that follows the PSR-4 standards to automatically load classes from a directory.
Requirements
- Utilize the spl_autoload_register function to register the autoload function.
- Verify that the autoload function confirms if the class name begins with a designated namespace prefix (e.g., App).
- Establish a root directory where the classes are stored (e.g., src/).
- Transform the namespace and class name into a file path and include the file if it exists.
- Validate the functionality of the autoloader by testing it with a sample class.
php projects
php coding exercises
php practice projects
php programming exercises
Code
spl_autoload_register(function ($class) {
$prefix = 'App\\';
$base_dir = __DIR__ . '/src/';
$len = strlen($prefix);
if (strncmp($prefix, $class, $len) !== 0) {
return;
}
$relative_class = substr($class, $len);
$file = $base_dir . str_replace('\\', '/', $relative_class) . '.php';
if (file_exists($file)) {
require $file;
}
});
// Assuming classes are in the 'src' directory and namespaced under 'App'
use App\Controllers\HomeController;
$controller = new HomeController();
$controller->index();
Explanation
Autoloading Overview: Autoloading is a feature that automatically loads PHP classes when they are needed, without the need for explicit require or include statements. The PSR-4 standard provides guidelines on how class files should be organized and loaded.
spl_autoload_register: By using this function, you can register an autoloader function. This registered function will be called whenever an undefined class is created.
Namespace and Directory Structure: The autoloader is designed to work with a specific namespace prefix (App) and a base directory (src/). This structure is defined within the autoloader function.
Length Check: The function checks if the class name starts with the specified prefix. If it doesn’t, the function will return early.
File Path Construction: If the class name starts with the prefix, the prefix is removed from the class name to obtain the relative class name. The relative class name is then converted into a file path by replacing namespace separators () with directory separators (/) and adding the .php extension.
File Inclusion: If the constructed file path exists, it is included using the require statement.
Usage: This autoloader assumes that classes are located in the src directory and are namespaced under App. Whenever a class from this namespace is instantiated, the autoloader will automatically load the corresponding file.
If You Need More Projects
- Discover Python projects
- Discover JavaScript projects
- Go to PHP projects
- Go to Java projects
- Go to Computer Engineering projects
- For more c++ projects
Exercise 3: Building a Simple MVC Framework
Objective
Let’s develop a simple MVC framework that includes routing, controllers, and models.
Build a basic MVC framework. Create a simple Router
class to handle routing, a Controller
base class, and a Model
base class. Write a demonstration of how to use this framework to create a simple web application that displays a list of items from a database.
php exercises
practice php
php practical
php practice
Requirements
- Develop a Router class with functions for adding routes and dispatching requests.
- Establish an abstract Controller class with an abstract index() method.
- Create a concrete ItemController class that extends Controller and implements the index method.
- Make sure the Router class can link routes to controller actions and manage requests.
- Show how to utilize the router and controller to exhibit a list of items.
Code
class Router {
private $routes = [];
public function addRoute($route, $callback) {
$this->routes[$route] = $callback;
}
public function dispatch($route) {
if (isset($this->routes[$route])) {
call_user_func($this->routes[$route]);
} else {
echo "Route not found!";
}
}
}
abstract class Controller {
abstract public function index();
}
class ItemController extends Controller {
public function index() {
echo "List of items";
}
}
class Model {
// Base model class
}
// Usage
$router = new Router();
$router->addRoute('items', [new ItemController(), 'index']);
$router->dispatch('items');
Explanation
MVC Framework Overview: The MVC framework is a design pattern that divides an application into three main components: Model, View, and Controller. This division helps in effectively managing complex applications by organizing the code in a way that is easy to maintain.
Router Class: The Router class handles HTTP requests and directs them to the appropriate controller actions. It keeps track of a list of routes and their corresponding callbacks.
addRoute Method: By using this method, you can add a route and its callback to the $routes array. The route is a string that represents the URL, and the callback is a function or method that will be executed when the route is accessed.
dispatch Method: This method takes a route as input and checks if it exists in the $routes array. If it does, it calls the associated callback. If the route is not found, it displays an error message.
Controller Class: The Controller class serves as a base class for all controllers in the application. It defines a common interface called “index” that all controllers must implement.
ItemController Class: This class extends the Controller class and implements the index method. In this example, the index method simply outputs a string. However, in a real application, it would interact with models and views to generate a response.
Usage: To demonstrate how the router directs requests to the appropriate controller action, you create a Router instance and add a route that maps to the index method of an ItemController instance. Then, you call the dispatch method with the route as an argument.
Exercise 4: Creating a Custom Exception
Objective
Create a custom exception class called ValidationException
that extends PHP’s Exception
class. Write a function that validates user input and throws this exception if the input is invalid. Demonstrate how to catch and handle this exception.
Requirements
- Create a ValidationException class that inherits from PHP’s Exception class.
- Develop a validateInput function that carries out input validation.
- Make sure that the function throws a ValidationException when the input is invalid.
- Utilize a try-catch block to capture and manage the ValidationException.
- Show a suitable error message when an exception is caught.
Code
class ValidationException extends Exception {}
function validateInput($input) {
if (empty($input)) {
throw new ValidationException("Input cannot be empty");
}
if (!is_string($input)) {
throw new ValidationException("Input must be a string");
}
return true;
}
// Usage
try {
validateInput("");
} catch (ValidationException $e) {
echo "Validation failed: " . $e->getMessage();
}
php practice assignments
php real-world projects
php tutorial exercises
Explanation
Custom Exceptions Overview: Custom exceptions are a great way to handle specific error conditions in your application more effectively. By extending PHP’s built-in Exception class, you can create exceptions that are tailored to your application’s needs.
ValidationException Class: This class extends the Exception class to create a custom exception specifically for validation purposes. While it doesn’t introduce any new functionality, it helps distinguish validation-related exceptions from other types of exceptions.
validateInput Function: The validateInput function is responsible for performing basic validation on input data. It checks for empty inputs or inputs that are not strings. If any validation check fails, it throws a ValidationException with a relevant error message.
try-catch Block: In the try block, you place the code that might throw an exception. If a ValidationException is thrown, the catch block captures it and displays an error message. This showcases how to gracefully handle custom exceptions.
Exercise 5: Using PDO for Database Operations
Objective
Create a class UserRepository
that uses PHP Data Objects (PDO) to perform CRUD operations on a users
table in a MySQL database. Implement methods for creating, reading, updating, and deleting users.
Requirements
- Create a UserRepository class that handles database operations.
- In the constructor, initialize a PDO instance with the provided DSN, username, and password.
- Set the PDO error mode to exception to handle any errors that may occur.
- Implement methods for createUser, getUser, updateUser, and deleteUser using prepared statements to ensure secure and efficient SQL operations.
- To demonstrate the CRUD operations, you can use example data.
Code
class UserRepository {
private $pdo;
public function __construct($dsn, $username, $password) {
$this->pdo = new PDO($dsn, $username, $password);
$this->pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}
public function createUser($name, $email) {
$stmt = $this->pdo->prepare("INSERT INTO users (name, email) VALUES (:name, :email)");
$stmt->execute(['name' => $name, 'email' => $email]);
}
public function getUser($id) {
$stmt = $this->pdo->prepare("SELECT * FROM users WHERE id = :id");
$stmt->execute(['id' => $id]);
return $stmt->fetch(PDO::FETCH_ASSOC);
}
public function updateUser($id, $name, $email) {
$stmt = $this->pdo->prepare("UPDATE users SET name = :name, email = :email WHERE id = :id");
$stmt->execute(['id' => $id, 'name' => $name, 'email' => $email]);
}
public function deleteUser($id) {
$stmt = $this->pdo->prepare("DELETE FROM users WHERE id = :id");
$stmt->execute(['id' => $id]);
}
}
// Usage
$repository = new UserRepository('mysql:host=localhost;dbname=testdb', 'root', 'password');
$repository->createUser('John Doe', 'john@example.com');
$user = $repository->getUser(1);
print_r($user);
$repository->updateUser(1, 'Jane Doe', 'jane@example.com');
$repository->deleteUser(1);
php mvc project source code
php photo database
php photo gallery
php project source code
php projects source code
php projects with source code
php real time application
php real time chat application
php realtime chat
Explanation
PDO Overview: PHP Data Objects (PDO) simplifies database access by providing a consistent way to interact with various databases securely and efficiently through prepared statements.
UserRepository Class: This class utilizes PDO to manage interactions with a MySQL database, offering functions for user creation, retrieval, updating, and deletion within the database.
Constructor: By accepting the Data Source Name (DSN), username, and password, the constructor establishes a new PDO instance with error mode set to exception, ensuring that any database errors are handled as exceptions.
createUser Method: Inserting a new user into the users table with a name and email as parameters, this method utilizes a prepared statement to prevent SQL injection attacks by separating SQL code from user input.
getUser Method: Retrieving a user based on their ID from the users table using a prepared statement, this method returns the user’s information as an associative array.
updateUser Method: Updating user details in the users table by taking a user ID, name, and email as parameters, this method employs a prepared statement for secure database operations.
deleteUser Method: Deleting a user from the users table based on their ID using a prepared statement, this method ensures secure deletion of user records.
Usage: To demonstrate CRUD operations using PDO, create an instance of UserRepository with the necessary database connection details and utilize the createUser, getUser, updateUser, and deleteUser methods accordingly.
Conclusion
Hold on a minute! Think you’ve got PHP down pat? Mastered the basics and churning out websites like a pro? Well, get ready, because we’re about to elevate your PHP skills to a whole new level!
This isn’t your typical beginner’s guide. We’re diving into advanced challenges that will make your code shine brighter than Beyonce at Coachella. No sugar-coating here – these exercises are no walk in the park. But don’t worry, we’ll break it down step-by-step with crystal-clear explanations that’ll make you say, “Wow, that was easy!”
php practical examples
php mini projects
php test cases
php beginner projects
php hands-on exercises
Get ready to boost your complexity, consistency, and, most importantly, your creativity (we’re talking about code that’s bursting with brilliance!).
If you’re ready to leave the kiddie pool behind and jump into the deep end of PHP, then buckle up and let’s kick things off! We’ve got five challenging PHP tasks lined up, each one designed to push your skills to the max. Think of them as your PHP training exercises, your opportunity to master some real-world PHP scenarios.
By the time you’re finished, you’ll be writing PHP code smoother than silk. Rumor has it these exercises are so good, recruiters will be knocking on your door before you can say “spaghetti code.” Let’s get started!
Looking for more PHP challenges? No problem, buddy. The internet is full of resources to keep you sharp. Explore some PHP project samples online or tackle some brain-teasing PHP coding exercises. There’s always something new to discover in the world of PHP!
php programming tasks
php coding challenges
php interactive exercises
php development exercises
php exercises for beginners
0 Comments