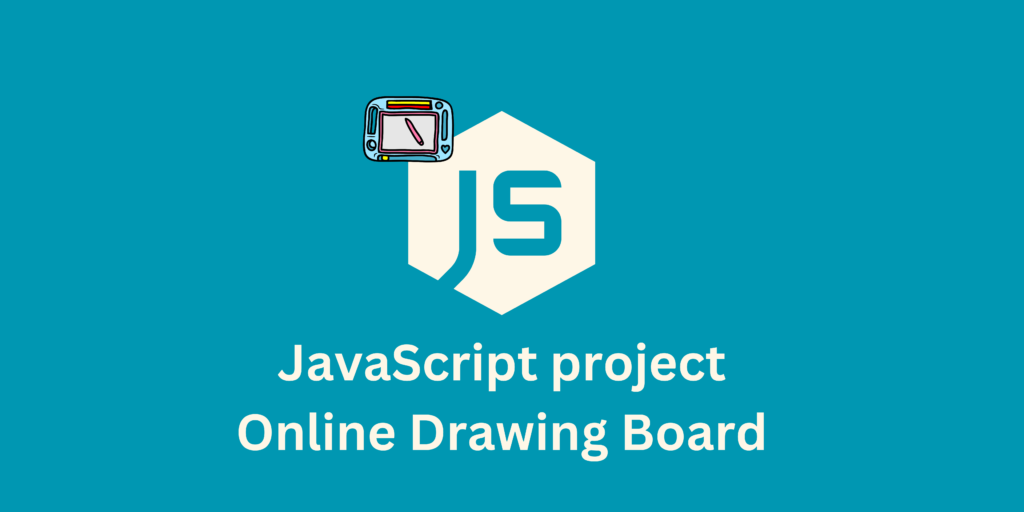
Table of Contents
Introduction to Online Drawing Board in JavaScript
Online Drawing Board: This code will create an online drawing board where users can draw, sketch, or create digital artwork using various tools and color options.
Drawing tablets are an important part of many applications today, such as Google Jamboard, Canva, Sketch.io, and more.
Since there are so many such apps out there, I still haven’t felt the urge to get a custom artboard that I can customize to my liking.
Watch the Video Tutorial
So I thought, why not create a place where I can write the components I want? “How do these components work, you ask?” Do you need a binary tree? Write the nodes in the order ['H', 'E', 'L', 'L', 'O'] and voila! Binary tree sketch.
Do you need neural networks? Enter your configuration and boom! Prepare a neural network! In this article, we will create a drawing board so that you can personalize your own components according to your needs.
The elements will be centered on the page, and the design will include color options and a clear button.
Step 0: Create the Requirements
Make one HTML, one CSS and one JavaScript file, link them.
I will be adding links to code pen in this article at certain points. Everything is in the video.
online whiteboard online whiteboards whiteboard online
Step 1: The CSS Code (The style)
Welcome to the backstage of our Free Drawing Board! Let’s break down the HTML code and see what’s cooking:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Free Drawing Board</title>
<!-- CSS -->
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
text-align: center;
}
.titre{
color: #154415;
font-size: 58px;
}
#drawing-board {
border: 1px solid #ccc;
background-color: #fff;
}
#color-options {
margin-top: 10px;
display: flex;
justify-content: center;
}
.color-option {
display: inline-block;
width: 20px;
height: 20px;
border: 1px solid #ccc;
margin-right: 5px;
cursor: pointer;
border-radius: 15px;
}
#clear-btn {
margin-top: 10px;
background-color: #154415;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
border-radius: 30px;
}
.selected {
border: 2px solid #000;
}
#clear-btn {
margin-top: 10px;
}
</style>
</head>
Here’s a peek behind the curtain:
- We start with the
<!DOCTYPE html>
declaration, setting the document type to HTML5 for modern web browsing. - The
<html>
tag wraps our entire HTML document, specifying the language as English. - Moving on to the
<head>
section, we set character encoding, compatibility, and viewport settings for responsive design. - The page gets a snazzy title with
<title>Free Drawing Board</title>
. Gotta give it some personality, right? - Now, onto the CSS! We embed our styles right in the HTML with
<style>
. It’s like giving our webpage its own fashion statement.
And that’s just the beginning! Stay tuned for more HTML shenanigans as we delve deeper into the interactive world of our Free Drawing Board! 🎨🚀
free online whiteboard whiteboard drawing board drawing online
Step 2: The HTML Code (the body)
Now, let’s dive into the heart of our Online Drawing Board with the <body>
section:
<body>
<h1 class="titre">Online Drawing Board</h1>
<canvas id="drawing-board" width="800" height="600"></canvas>
<br>
<div id="color-options">
<div class="color-option selected" style="background-color: #000000;"></div>
<div class="color-option" style="background-color: #ff0000;"></div>
<div class="color-option" style="background-color: #00ff00;"></div>
<div class="color-option" style="background-color: #0000ff;"></div>
<div class="color-option" style="background-color: #ffff00;"></div>
<div class="color-option" style="background-color: #ff00ff;"></div>
<div class="color-option" style="background-color: #00ffff;"></div>
</div>
<br>
<button id="clear-btn">Clear</button>
Here’s what’s happening:
- We kick things off with a bold
<h1>
heading, declaring to the world that this is our Online Drawing Board. Let’s get creative! - Next up, we introduce the canvas element
<canvas>
with an ID of “drawing-board” and set its width and height to 800 and 600 pixels, respectively. Time to unleash our artistic genius! - Below the canvas, we present a palette of color options with the
<div>
container “color-options”. Each color option is represented by a<div>
with the class “color-option” and a unique background color. - We even start with a selected color to give our users a head start on their masterpieces. Ain’t that thoughtful?
- And last but not least, we add a handy-dandy “Clear” button with the ID “clear-btn”. When clicked, it’ll wipe the canvas clean and give us a fresh canvas to work with. Let the creative chaos begin!
With this setup, our Online Drawing Board is ready to rock and roll! Let the doodling commence! 🎨🚀
So, whether you’re sketching a masterpiece, doodling for fun, or simply exploring the capabilities of web development, remember that the journey is just as enriching as the destination.
With the knowledge gained from this adventure, you’re equipped to embark on your own creative endeavors and continue pushing the boundaries of what’s possible in the digital landscape.
online drawing board together whiteboard online drawing draw board online
Step 3: The JavaScript Code
And now, let’s add some magic with our JavaScript code:
<!-- Javasctript -->
<script>
// Get the canvas element and its context
var canvas = document.getElementById('drawing-board');
var context = canvas.getContext('2d');
// Set initial drawing color and line width
var color = '#000000';
var lineWidth = 5;
// Flag to track drawing state
var isDrawing = false;
// Function to start drawing
function startDrawing(event) {
isDrawing = true;
// Get the coordinates of the mouse pointer
var x = event.pageX - canvas.offsetLeft;
var y = event.pageY - canvas.offsetTop;
// Begin a new drawing path
context.beginPath();
// Set the starting point of the path
context.moveTo(x, y);
}
// Function to continue drawing
function continueDrawing(event) {
if (isDrawing) {
// Get the coordinates of the mouse pointer
var x = event.pageX - canvas.offsetLeft;
var y = event.pageY - canvas.offsetTop;
// Draw a line to the current mouse position
context.lineTo(x, y);
// Set the line color and width
context.strokeStyle = color;
context.lineWidth = lineWidth;
// Stroke the path to display the line
context.stroke();
}
}
// Function to stop drawing
function stopDrawing() {
isDrawing = false;
}
// Function to clear the drawing board
function clearDrawingBoard() {
context.clearRect(0, 0, canvas.width, canvas.height);
}
// Function to set the selected color
function setSelectedColor(selectedColor) {
// Remove the "selected" class from all color options
var colorOptions = document.querySelectorAll('.color-option');
colorOptions.forEach(function(option) {
option.classList.remove('selected');
});
// Add the "selected" class to the clicked color option
selectedColor.classList.add('selected');
// Get the background color of the selected color option
color = selectedColor.style.backgroundColor;
}
// Event listeners for drawing actions
canvas.addEventListener('mousedown', startDrawing);
canvas.addEventListener('mousemove', continueDrawing);
canvas.addEventListener('mouseup', stopDrawing);
canvas.addEventListener('mouseleave', stopDrawing);
// Event listener for clear button
var clearButton = document.getElementById('clear-btn');
clearButton.addEventListener('click', clearDrawingBoard);
// Event listener for color options
var colorOptions = document.querySelectorAll('.color-option');
colorOptions.forEach(function(option) {
option.addEventListener('click', function() {
setSelectedColor(this);
});
});
</script>
</body>
</html>
And now, let’s decode this JavaScript jumble:
- We start by grabbing our canvas element and its context, setting up initial drawing settings like color and line width, and tracking the drawing state.
- We define functions to handle drawing actions like starting, continuing, and stopping drawing, as well as clearing the drawing board and setting the selected color.
- Event listeners are added to capture mouse actions on the canvas, clicks on the clear button, and clicks on color options.
- With this JavaScript sorcery, our Online Drawing Board comes to life, ready to capture your creative genius stroke by stroke! 🎨✨
As we wrap up our exploration, it's clear that the fusion of creativity and technology opens doors to endless possibilities. Whether you're a seasoned coder seeking to expand your skill set or an aspiring artist looking to express yourself in new ways, the Online Drawing Board serves as a canvas for innovation and experimentation.
Conclusion
In conclusion, delving into the realm of Online Drawing Board with JavaScript Source Code offers a captivating journey into the world of digital artistry and interactive web experiences.
By dissecting the intricacies of HTML, CSS, and JavaScript, we’ve uncovered the magic behind creating a dynamic canvas for unleashing creativity.
From setting up the canvas and defining drawing functions to handling user interactions and implementing features like color selection and clearing the board, we’ve witnessed the power of JavaScript in bringing our Online Drawing Board to life.
whiteboard online free board drawing online online whiteboard
In the end, the Online Drawing Board with JavaScript Source Code not only provides a platform for artistic expression but also serves as a testament to the boundless creativity and ingenuity of the human spirit.
So, pick up your virtual brush, embrace the possibilities, and let your imagination soar across the digital canvas. The world is your oyster, and the web is your playground. Happy drawing! 🎨✨
Learn more
online whiteboard
whiteboard online
online whiteboards
free online whiteboard whiteboards online
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments