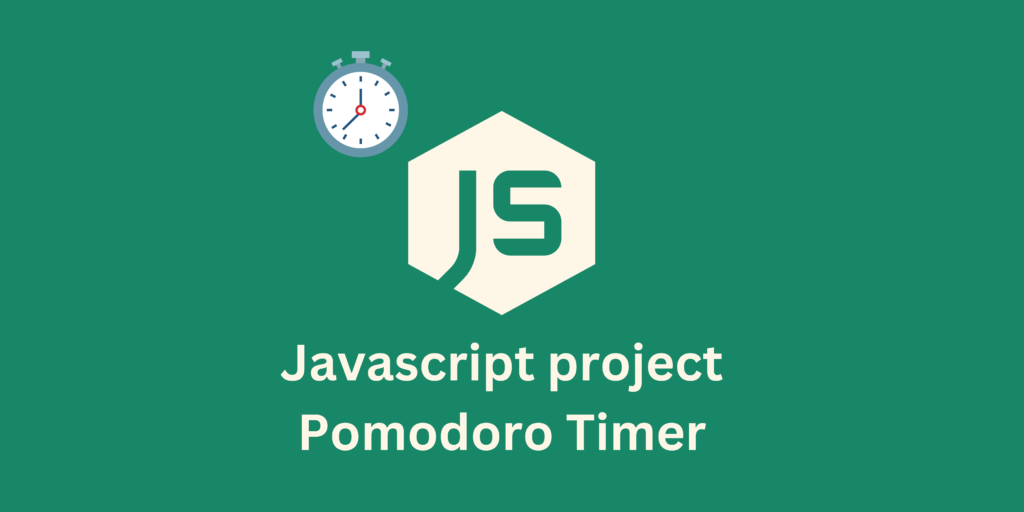
The Javascript project Pomodoro Technique is a tool for helping people concentrate on a task for a predetermined amount of time, usually 25 minutes, followed by a brief break.
The JavaScript implementation consists of an HTML container with start and stop buttons, as well as a timer display. While JavaScript specifies the timer countdown logic and button event listeners, CSS styles the container and its contents.
1. HTML
In the HTML, we have a container div with class timer-container
that contains a heading with class timer
, displaying the time remaining, and two buttons with classes start-button
and stop-button
respectively.
<div class="timer-container">
<h1 class="timer">25:00</h1>
<button class="start-button">Start</button>
<button class="stop-button">Stop</button>
</div>
2. CSS
In the CSS, we style the timer container to be a flexbox with its contents centered. We style the timer heading to be large and the buttons to be smaller.
.timer-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.timer {
font-size: 6rem;
margin-bottom: 2rem;
}
button {
font-size: 1.5rem;
margin: 1rem;
}
3. Javascript
In the JavaScript, we start by defining some variables:
timerDisplay
: a reference to theh1
element that displays the time remaining.startButton
andstopButton
: references to thebutton
elements that start and stop the timer respectively.countdown
: a variable that will hold the ID of the setInterval function that updates the timer every second.isRunning
: a boolean variable that tracks whether the timer is currently running or not.
const timerDisplay = document.querySelector('.timer');
const startButton = document.querySelector('.start-button');
const stopButton = document.querySelector('.stop-button');
let countdown;
let isRunning = false;
function startTimer(duration) {
const startTime = Date.now();
const endTime = startTime + duration * 1000;
updateTimer(duration);
countdown = setInterval(() => {
const remainingTime = Math.round((endTime - Date.now()) / 1000);
if (remainingTime < 0) {
clearInterval(countdown);
timerDisplay.textContent = 'Time is up!';
return;
}
updateTimer(remainingTime);
}, 1000);
}
function updateTimer(duration) {
const minutes = Math.floor(duration / 60).toString().padStart(2, '0');
const seconds = (duration % 60).toString().padStart(2, '0');
timerDisplay.textContent = `${minutes}:${seconds}`;
}
function stopTimer() {
clearInterval(countdown);
isRunning = false;
}
startButton.addEventListener('click', () => {
if (isRunning) {
return;
}
isRunning = true;
startTimer(25 * 60);
});
stopButton.addEventListener('click', () => {
stopTimer();
});
Next, we define two functions:
startTimer(duration)
: this function takes a duration in seconds as an argument and starts the timer countdown. We calculate the end time of the countdown by adding the duration to the current time, and then usesetInterval
to update the timer every second. When the countdown reaches zero, we clear the interval and display a message indicating that time is up.updateTimer(duration)
: this function takes a duration in seconds as an argument and updates the timer display by converting the duration into minutes and seconds, padding them with leading zeroes as necessary, and concatenating them with a colon separator.
Finally, we add event listeners to the start and stop buttons. When the start button is clicked, we check if the timer is already running. If it is, we do nothing. If it isn’t, we set isRunning
to true
and start the timer countdown for 25 minutes (the standard duration for a Pomodoro session). When the stop button is clicked, we call the stopTimer()
function, which clears the interval and sets isRunning
back to false
.
2 Comments
Jamestries · April 20, 2024 at 6:48 am
Thanks
MichaelGlofs · July 26, 2024 at 12:41 pm
Thank you good project