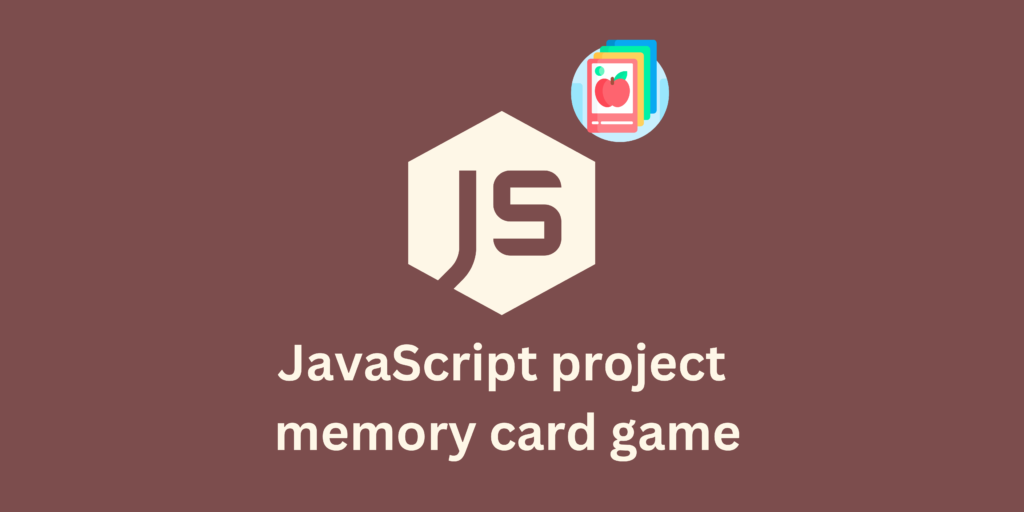
A fun and interactive Javascript project game that puts players’ memory skills to the test is the Memory Card Game project. Matching pairs of cards with similar symbols within a grid is the game’s goal. It’s a timeless game that challenges your memory and attention while also being fun to play.
So let's get started and have fun creating our very own Memory Card Game!
JavaScript code
// Array of card symbols
const cardSymbols = [
'🐶', '🐱', '🐭', '🐹', '🐰', '🦊', '🐻', '🐼',
'🐯', '🦁', '🐮', '🐷', '🐸', '🐵', '🐔', '🐧'
];
// Create a new shuffled deck of cards
const createDeck = () => {
const deck = [...cardSymbols, ...cardSymbols];
for (let i = deck.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[deck[i], deck[j]] = [deck[j], deck[i]];
}
return deck;
};
// Global variables
let deck = [];
let flippedCards = [];
let matchedCards = [];
let isBoardLocked = false;
// Function to handle card click
const handleCardClick = (index) => {
if (isBoardLocked || matchedCards.includes(index) || flippedCards.includes(index)) {
return;
}
const card = document.getElementById(`card-${index}`);
card.classList.add('flipped');
flippedCards.push(index);
if (flippedCards.length === 2) {
isBoardLocked = true;
const [card1, card2] = flippedCards;
if (deck[card1] === deck[card2]) {
matchedCards.push(card1, card2);
if (matchedCards.length === deck.length) {
setTimeout(() => {
alert('Congratulations! You won the game!');
}, 500);
}
} else {
setTimeout(() => {
const card1Elem = document.getElementById(`card-${card1}`);
const card2Elem = document.getElementById(`card-${card2}`);
card1Elem.classList.remove('flipped');
card2Elem.classList.remove('flipped');
flippedCards = [];
isBoardLocked = false;
}, 1000);
}
}
};
// Function to create the game board
const createGameBoard = () => {
const gameBoard = document.getElementById('game-board');
deck = createDeck();
deck.forEach((symbol, index) => {
const card = document.createElement('div');
card.className = 'card';
card.id = `card-${index}`;
card.addEventListener('click', () => handleCardClick(index));
const front = document.createElement('div');
front.className = 'front';
const back = document.createElement('div');
back.className = 'back';
back.innerHTML = symbol;
card.appendChild(front);
card.appendChild(back);
gameBoard.appendChild(card);
});
};
// Initialize the game board
createGameBoard();
HTML code
<!DOCTYPE html>
<html>
<head>
<title>Memory Card Game</title>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="game-board">
<!-- Cards will be dynamically generated here -->
</div>
<script src="script.js"></script>
</body>
</html>
In this HTML code, we have a basic structure for the Memory Card Game. The game board will be dynamically generated inside the <div class="game-board">
element. We link an external CSS file (styles.css
) to style the game and an external JavaScript file (script.js
) to handle the game logic.
CSS code
.game-board {
display: flex;
flex-wrap: wrap;
max-width: 420px;
margin: 0 auto;
}
.card {
width: 100px;
height: 100px;
margin: 5px;
background-color: #ddd;
border-radius: 5px;
display: flex;
justify-content: center;
align-items: center;
font-size: 40px;
cursor: pointer;
}
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments