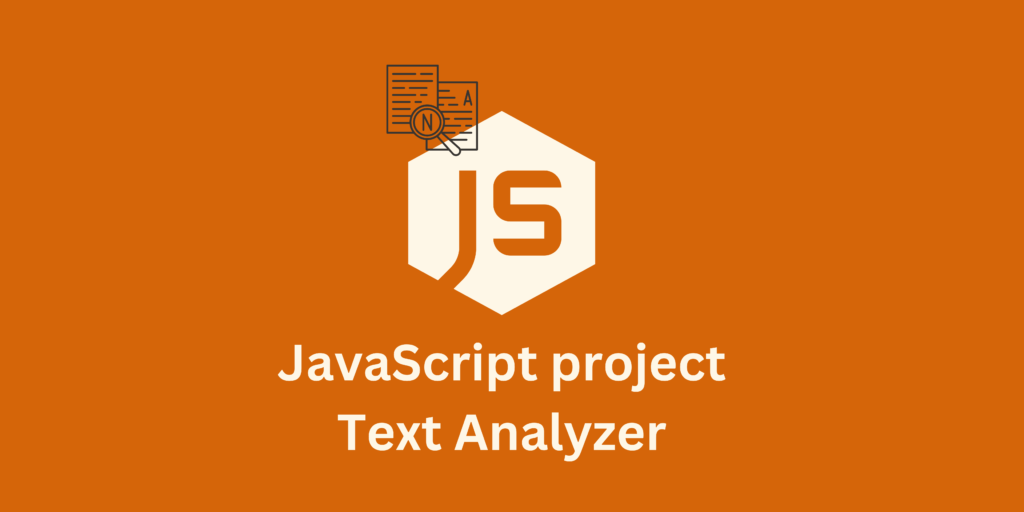
Javascript project ideas: This program analyzes a given text and provides information such as the number of words, characters, and average word length.
The analyzeText() function, which accepts a text string as input, is defined in this code. The text is first cleaned up by the function by removing extra spaces between words and leading and trailing whitespace. The cleaned text is then divided into an array of words, iterated through, and the number of characters, words, and average word length are calculated. The analysis results are then shown in the console.
function analyzeText(text) {
// Remove leading/trailing whitespace and extra spaces between words
const cleanedText = text.trim().replace(/\s+/g, ' ');
// Calculate the number of characters
const characterCount = cleanedText.length;
// Calculate the number of words
const words = cleanedText.split(' ');
const wordCount = words.length;
// Calculate the average word length
let totalWordLength = 0;
for (let i = 0; i < wordCount; i++) {
totalWordLength += words[i].length;
}
const averageWordLength = totalWordLength / wordCount;
// Display the analysis results
console.log('Text:', cleanedText);
console.log('Number of characters:', characterCount);
console.log('Number of words:', wordCount);
console.log('Average word length:', averageWordLength.toFixed(2));
}
// Example usage
const text = 'This is an example text for analysis.';
analyzeText(text);
Any text you want to analyze can be passed to the analyzeText() function. Based on the text input, the program will output the total number of characters, total number of words, and average word length.
Run the program
Save the program’s code in a JavaScript file, such as text_analysis.js, and run the following command at a command prompt or terminal to run it:
node text_analysis.js
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments