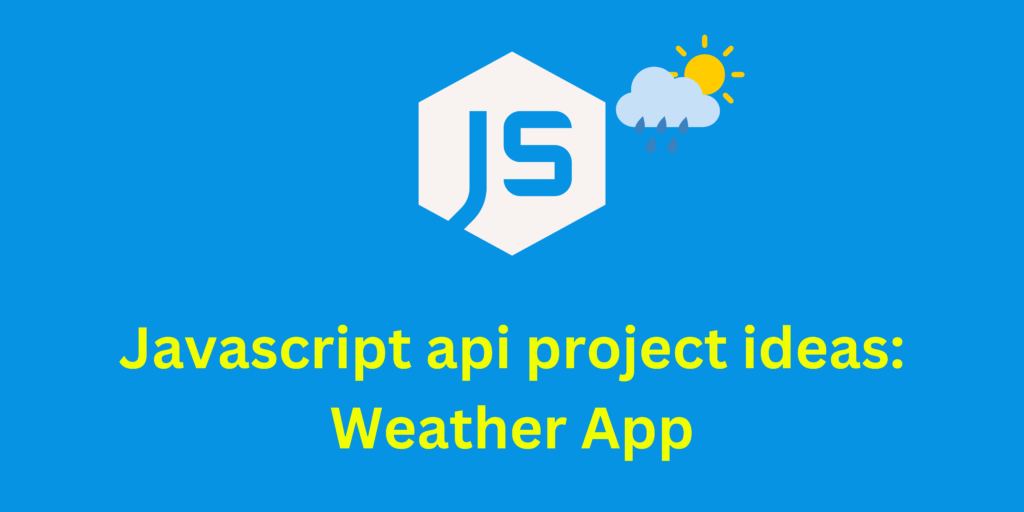
Javascript api project ideas: Weather App: Build a web app that displays a random quote each time the user clicks a button. Use an API to fetch the quotes and display them on the screen.
Description Javascript api project ideas: Weather App
Build a web app that displays the current weather and forecast for a given location. The user should be able to search for a location and see the current weather, as well as a forecast for the next few days.
To build this app, you will need to use a weather API to fetch the current weather and forecast data. You can use HTML, CSS, and JavaScript to build the user interface and handle the API requests.
Steps to build this app
- Create an HTML file with a search box and placeholders for the weather data.
- Add CSS styling to make the app look nice.
- Write JavaScript code to handle user input and fetch the weather data from the API.
- Display the weather data in the appropriate placeholders in the HTML.
Additional Features
- Use geolocation to automatically detect the user’s location and display the weather for that location.
- Allow the user to toggle between Celsius and Fahrenheit units.
- Display additional information, such as wind speed, humidity, and sunrise/sunset times.
- Use icons to represent the weather conditions (e.g., a sun for sunny weather, a cloud for cloudy weather, etc.).
- Add animations to make the app more engaging.
Some resources to help you get started
- OpenWeatherMap API documentation: https://openweathermap.org/api
- AccuWeather API documentation: https://developer.accuweather.com/apis
- Weatherbit API documentation: https://www.weatherbit.io/api
- Fetch API documentation: https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
- Geolocation API documentation: https://developer.mozilla.org/en-US/docs/Web/API/Geolocation_API
- Animate.css animation library: https://animate.style/
- Font Awesome icon library: https://fontawesome.com/
HTML for Javascript api project ideas: Weather App
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Weather App</title>
</head>
<body>
<h1>Weather App</h1>
<form>
<label for="city-input">Enter city name:</label>
<input type="text" id="city-input" required>
<button type="submit">Get Weather</button>
</form>
<div id="weather-info"></div>
<script src="script.js"></script>
</body>
</html>
Javascript code for Javascript api project ideas: Weather App
const apiKey = 'YOUR_API_KEY'; // Replace with your own API key from OpenWeatherMap
const form = document.querySelector('form');
const cityInput = document.querySelector('#city-input');
const weatherInfo = document.querySelector('#weather-info');
form.addEventListener('submit', (e) => {
e.preventDefault();
const city = cityInput.value;
const apiUrl = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const temp = Math.round(data.main.temp - 273.15);
const description = data.weather[0].description;
const country = data.sys.country;
weatherInfo.innerHTML = `The temperature in ${city}, ${country} is ${temp}°C with ${description}.`;
cityInput.value = '';
})
.catch(error => {
console.error(error);
weatherInfo.innerHTML = 'Error: City not found';
});
});
CSS code for Javascript api project ideas: Weather App
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
h1 {
text-align: center;
}
form {
display: flex;
flex-direction: column;
align-items: center;
margin: 20px;
}
label {
margin-bottom: 10px;
}
input {
padding: 5px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc;
margin-bottom: 10px;
}
button {
padding: 10px;
background-color: #0077be;
color: #fff;
font-size: 16px;
border-radius: 5px;
border: none;
cursor: pointer;
}
#weather-info {
margin: 20px;
font-size: 20px;
text-align: center;
}
Note: Remember to replace YOUR_API_KEY
with your own API key from OpenWeatherMap. You can obtain an API key by signing up on their website.
4 Comments
javascript development services · June 19, 2023 at 12:44 pm
Wow that was strange. I just wrote an very long comment but after
I clicked submit my comment didn’t show up. Grrrr…
well I’m not writing all that over again. Anyway, just wanted to say
fantastic blog!
buy ig followers · April 29, 2024 at 7:22 pm
It works and it’s fast, I recommend
ig followers · May 11, 2024 at 3:39 pm
I’m very grateful to have found this site. very useful and helpful with my career.
ig followers · May 13, 2024 at 12:59 am
Awesome. Very clear. They are an awesome website. Will visit and use again!