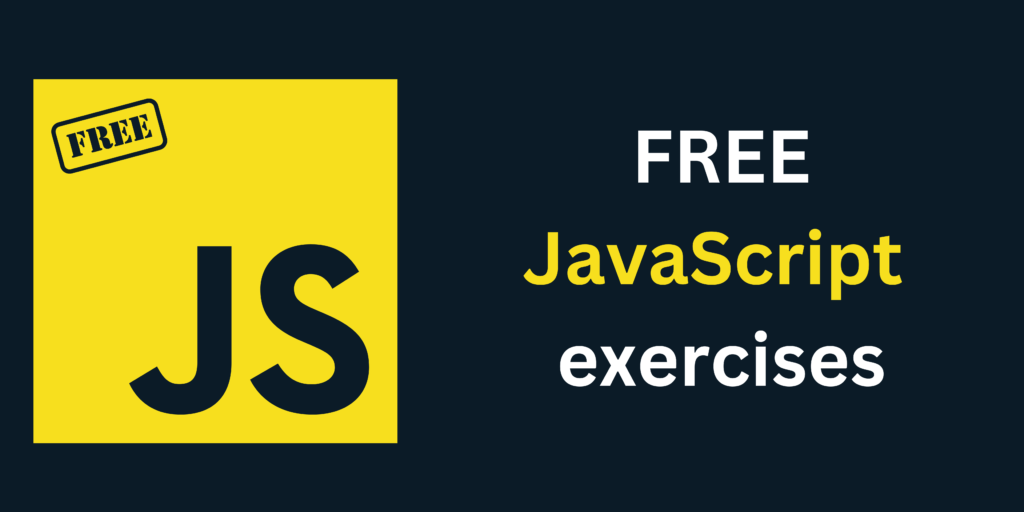
The javascript exercises offered are made to give you practice using JavaScript’s if..else statements to create conditional statements.
Different blocks of code can be executed depending on whether or not certain conditions are met thanks to conditional statements. This is a fundamental idea in programming that is widely applied to the creation of websites, software, and many other things.
JavaScript exercises:
Write a program that takes a string as input and returns the length of the string.
let str = "Hello, world!";
if(str.length > 0) {
console.log("The length of the string is " + str.length);
}
else {
console.log("The string is empty");
}
Write a program that takes three numbers as input and returns the smallest number.
let num1 = 5;
let num2 = 8;
let num3 = 2;
if(num1 <= num2 && num1 <= num3) {
console.log(num1 + " is the smallest number");
}
else if(num2 <= num1 && num2 <= num3) {
console.log(num2 + " is the smallest number");
}
else {
console.log(num3 + " is the smallest number");
}
Write a program that takes a number as input and checks if it is a prime number.
let num = 7;
let isPrime = true;
if(num <= 1) {
isPrime = false;
}
else {
for(let i = 2; i <= Math.sqrt(num); i++) {
if(num % i == 0) {
isPrime = false;
break;
}
}
}
if(isPrime) {
console.log(num + " is a prime number");
}
else {
console.log(num + " is not a prime number");
}
Write a program that takes a number as input and checks if it is a perfect square.
let num = 16;
if(Math.sqrt(num) == Math.floor(Math.sqrt(num))) {
console.log(num + " is a perfect square");
}
else {
console.log(num + " is not a perfect square");
}
Write a program that takes a number as input and checks if it is a multiple of 3 and/or 5.
let num = 15;
if(num % 3 == 0 && num % 5 == 0) {
console.log(num + " is a multiple of both 3 and 5");
}
else if(num % 3 == 0) {
console.log(num + " is a multiple of 3");
}
else if(num % 5 == 0) {
console.log(num + " is a multiple of 5");
}
else {
console.log(num + " is not a multiple of 3 or 5");
}
Write a program that takes two numbers and a mathematical operator (+, -, *, /) as input and returns the result of the operation.
let num1 = 10;
let num2 = 5;
let operator = "+";
let result;
if(operator == "+") {
result = num1 + num2;
}
else if(operator == "-") {
result = num1 - num2;
}
else if(operator == "*") {
result = num1 * num2;
}
else if(operator == "/") {
result = num1 / num2;
}
else {
console.log("Invalid operator");
}
console.log(num1 + " " + operator + " " + num2 + " = " + result);
Write a program that takes a year as input and checks if it is a leap year.
let year = 2024;
let isLeapYear = false;
if(year % 4 == 0) {
if(year % 100 == 0) {
if(year % 400 == 0) {
isLeapYear = true;
}
}
else {
isLeapYear = true;
}
}
if(isLeapYear) {
console.log(year + " is a leap year");
}
else {
console.log(year + " is not a leap year");
}
Write a program that takes a temperature in Celsius as input and converts it to Fahrenheit.
let celsius = 25;
let fahrenheit = (celsius * 9/5) + 32;
console.log(celsius + " degrees Celsius is equal to " + fahrenheit + " degrees Fahrenheit");
Write a program that takes a month and a year as input and returns the number of days in that month.
let month = "February";
let year = 2023;
let daysInMonth;
if(month == "February") {
if(year % 4 == 0) {
if(year % 100 == 0) {
if(year % 400 == 0) {
daysInMonth = 29;
}
else {
daysInMonth = 28;
}
}
else {
daysInMonth = 29;
}
}
else {
daysInMonth = 28;
}
}
else if(month == "April" || month == "June" || month == "September" || month == "November") {
daysInMonth = 30;
}
else {
daysInMonth = 31;
}
console.log("There are " + daysInMonth + " days in " + month + " " + year);
Write a program that takes a number as input and checks if it is positive, negative, or zero.
let num = 0;
if(num > 0) {
console.log(num + " is a positive number");
}
else if(num < 0) {
console.log(num + " is a negative number");
}
else {
console.log(num + " is zero");
}
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments