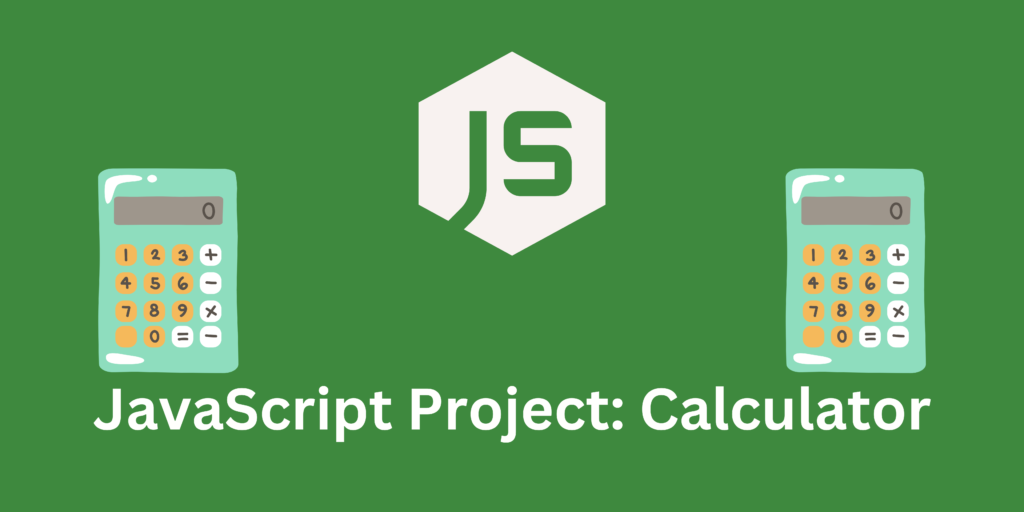
Build a Javascript Calculator using JavaScript that allows users to perform basic arithmetic operations. Use HTML and CSS to create a user interface for the calculator.
HTML:
<div class="calculator">
<input type="text" class="display">
<button class="number" value="1">1</button>
<button class="number" value="2">2</button>
<button class="number" value="3">3</button>
<button class="operator" value="+">+</button>
<button class="number" value="4">4</button>
<button class="number" value="5">5</button>
<button class="number" value="6">6</button>
<button class="operator" value="-">-</button>
<button class="number" value="7">7</button>
<button class="number" value="8">8</button>
<button class="number" value="9">9</button>
<button class="operator" value="*">*</button>
<button class="number" value="0">0</button>
<button class="clear" value="C">C</button>
<button class="equals" value="=">=</button>
<button class="operator" value="/">/</button>
</div>
CSS:
.calculator {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 10px;
background-color: #eee;
}
.display {
grid-column: 1 / -1;
font-size: 2rem;
text-align: right;
padding: 10px;
}
.number, .operator {
font-size: 2rem;
padding: 10px;
border: none;
background-color: #fff;
cursor: pointer;
}
.clear, .equals {
grid-column: 1 / span 2;
}
.clear {
background-color: #f00;
color: #fff;
}
.equals {
background-color: #0f0;
color: #fff;
}
JavaScript:
const calculator = document.querySelector('.calculator');
const display = calculator.querySelector('.display');
const numbers = calculator.querySelectorAll('.number');
const operators = calculator.querySelectorAll('.operator');
const clear = calculator.querySelector('.clear');
const equals = calculator.querySelector('.equals');
let operand1 = null;
let operator = null;
let operand2 = null;
numbers.forEach((number) => {
number.addEventListener('click', () => {
if (operator === null) {
operand1 = operand1 === null ? number.value : operand1 + number.value;
display.value = operand1;
} else {
operand2 = operand2 === null ? number.value : operand2 + number.value;
display.value = operand2;
}
});
});
operators.forEach((op) => {
op.addEventListener('click', () => {
if (operand1 !== null && operand2 !== null) {
const result = operate(parseFloat(operand1), parseFloat(operand2), operator);
operand1 = result.toString();
operand2 = null;
operator = op.value;
display.value = operand1;
} else if (operand1 !== null) {
operator = op.value;
}
});
});
clear.addEventListener('click', () => {
operand1 = null;
operand2 = null;
operator = null;
display.value = '';
});
equals.addEventListener('click', () => {
if (operand1 !== null && operand2 !== null) {
const result
const calculator = document.querySelector('.calculator');
const display = calculator.querySelector('.display');
const numbers = calculator.querySelectorAll('.number');
const operators = calculator.querySelectorAll('.operator');
const clear = calculator.querySelector('.clear');
const equals = calculator.querySelector('.equals');
let operand1 = null;
let operator = null;
let operand2 = null;
function operate(a, b, op) {
switch(op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
}
}
numbers.forEach((number) => {
number.addEventListener('click', () => {
if (operator === null) {
operand1 = operand1 === null ? number.value : operand1 + number.value;
display.value = operand1;
} else {
operand2 = operand2 === null ? number.value : operand2 + number.value;
display.value = operand2;
}
});
});
operators.forEach((op) => {
op.addEventListener('click', () => {
if (operand1 !== null && operand2 !== null) {
const result = operate(parseFloat(operand1), parseFloat(operand2), operator);
operand1 = result.toString();
operand2 = null;
operator = op.value;
display.value = operand1;
} else if (operand1 !== null) {
operator = op.value;
}
});
});
clear.addEventListener('click', () => {
operand1 = null;
operand2 = null;
operator = null;
display.value = '';
});
equals.addEventListener('click', () => {
if (operand1 !== null && operand2 !== null) {
const result = operate(parseFloat(operand1), parseFloat(operand2), operator);
operand1 = result.toString();
operand2 = null;
operator = null;
display.value = result;
}
});
Explaination of the javascript project
The operate function in this code accepts three arguments, a, b, and op, which represent the two operands and the operator, and returns the result of applying the operator to the operands.
The calculator’s main logic is in the event listeners for numbers and operators, which update the values of operand1, operand2, and operator as needed.
The equals event listener invokes the operate function with the current operand and operator values, and the result is displayed in the display element.
The clear event listener clears the display and resets all of the calculator’s values.
1 Comment
ustvarite racun na binance · April 23, 2024 at 8:36 am
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.