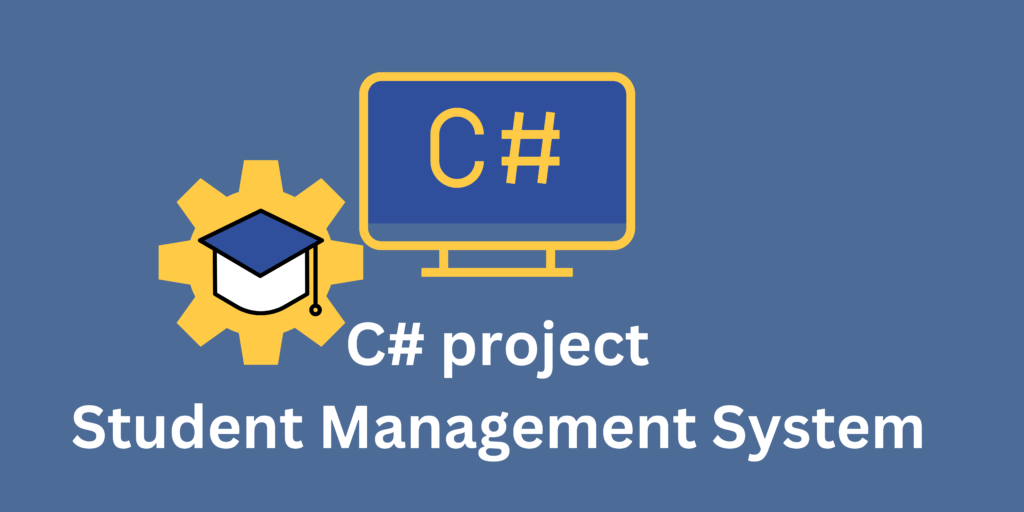
Table of Contents
Introduction to this C# Projects
Imagine a world where managing students, schedules, grades, and attendance isn’t a paper-shuffling nightmare. Enter the Student Management System (SMS), a C# project designed to revolutionize the way educational institutions operate!
The Power is in Your Hands
This SMS isn’t just another boring database. It’s a full-fledged ecosystem built with teachers and administrators in mind. Here’s a glimpse of the magic it unleashes:
- Effortless Scheduling: Juggling classes and teachers? Not anymore! Build the perfect course schedule with a drag-and-drop interface, ensuring happy students and efficient resource allocation.
- Grading on Autopilot: Spend less time grading papers and more time inspiring young minds. The SMS lets you create assignments, collect submissions electronically, and even calculate grades with a click.
- Attendance Made Easy: Gone are the days of manual roll calls. Track student attendance in real-time, with options for excuses and automated notifications for parents or guardians. Say goodbye to absent headaches!
- Data at Your Fingertips: The SMS is your personal data guru. Generate insightful reports on student performance, identify trends, and make data-driven decisions to improve learning outcomes.
Unleashing Creativity (and a Burst of Efficiency!)
This C# project is designed to be more than just functional. It’s crafted to spark creativity and boost productivity. Here’s the secret sauce:
- Intuitive Interface: The user-friendly interface is as easy to navigate as your favorite social media app. No IT degree required!
- Customization is King: Tailor the SMS to your specific needs. From adding custom fields to reports to integrating with existing school systems, the possibilities are endless!
- Collaboration is Key: Foster communication between teachers, parents, and students with built-in messaging tools and discussion forums.
The Future of Education is Here
The Student Management System is more than just a C# project; it’s an investment in the future of education. Imagine a school where:
- Teachers can focus on inspiring, not paperwork.
- Students have access to real-time feedback and personalized learning paths.
- Administrators can make informed decisions based on data.
This is the power of the Student Management System. Let’s embark on this journey together and build a brighter future for education, one line of code at a time!
The Project C# Code
Note: You can use my code and customize it. I don’t mind.
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
// Create a list to store student records
List<Student> students = new List<Student>();
// Main menu loop
while (true)
{
Console.WriteLine("Student Management System");
Console.WriteLine("-------------------------");
Console.WriteLine("1. Register Student");
Console.WriteLine("2. Record Attendance");
Console.WriteLine("3. Enter Grades");
Console.WriteLine("4. View Class Timetable");
Console.WriteLine("5. Generate Report");
Console.WriteLine("6. Exit");
Console.WriteLine();
Console.Write("Enter your choice (1-6): ");
int choice = Convert.ToInt32(Console.ReadLine());
switch (choice)
{
case 1:
RegisterStudent(students);
break;
case 2:
RecordAttendance(students);
break;
case 3:
EnterGrades(students);
break;
case 4:
ViewClassTimetable(students);
break;
case 5:
GenerateReport(students);
break;
case 6:
Console.WriteLine("Exiting the program...");
Environment.Exit(0);
break;
default:
Console.WriteLine("Invalid choice. Please try again.");
break;
}
Console.WriteLine();
}
}
// Method to register a new student
static void RegisterStudent(List<Student> students)
{
Console.WriteLine("Register Student");
Console.WriteLine("----------------");
Console.Write("Enter student name: ");
string name = Console.ReadLine();
Console.Write("Enter student ID: ");
int id = Convert.ToInt32(Console.ReadLine());
// Create a new student object and add it to the list
Student newStudent = new Student(id, name);
students.Add(newStudent);
Console.WriteLine("Student registered successfully.");
}
// Method to record attendance for a student
static void RecordAttendance(List<Student> students)
{
Console.WriteLine("Record Attendance");
Console.WriteLine("-----------------");
Console.Write("Enter student ID: ");
int id = Convert.ToInt32(Console.ReadLine());
// Search for the student by ID
Student student = students.Find(s => s.Id == id);
if (student != null)
{
Console.Write("Enter attendance status (Present/Absent): ");
string status = Console.ReadLine();
// Record attendance for the student
student.RecordAttendance(status);
Console.WriteLine("Attendance recorded successfully.");
}
else
{
Console.WriteLine("Student not found.");
}
}
// Method to enter grades for a student
static void EnterGrades(List<Student> students)
{
Console.WriteLine("Enter Grades");
Console.WriteLine("------------");
Console.Write("Enter student ID: ");
int id = Convert.ToInt32(Console.ReadLine());
// Search for the student by ID
Student student = students.Find(s => s.Id == id);
if (student != null)
{
Console.Write("Enter subject name: ");
string subject = Console.ReadLine();
Console.Write("Enter grade: ");
string grade = Console.ReadLine();
// Enter grades for the student
student.EnterGrade(subject, grade);
Console.WriteLine("Grade entered successfully.");
}
else
{
Console.WriteLine("Student not found.");
}
}
// Method to view class timetable for a student
static void ViewClassTimetable(List<Student> students)
{
Console.WriteLine("View Class Timetable");
Console.WriteLine("--------------------");
Console.Write("Enter student ID: ");
int id = Convert.ToInt32(Console.ReadLine());
// Search for the student by ID
Student student = students.Find(s => s.Id == id);
if (student != null)
{
// Get the class timetable for the student
List<string> timetable = student.GetClassTimetable();
if (timetable.Count > 0)
{
Console.WriteLine("Class Timetable:");
foreach (string subject in timetable)
{
Console.WriteLine(subject);
}
}
else
{
Console.WriteLine("No classes scheduled for this student.");
}
}
else
{
Console.WriteLine("Student not found.");
}
}
// Method to generate a report for all students
static void GenerateReport(List<Student> students)
{
Console.WriteLine("Generate Report");
Console.WriteLine("----------------");
foreach (Student student in students)
{
Console.WriteLine("Student Name: " + student.Name);
Console.WriteLine("Student ID: " + student.Id);
Console.WriteLine("Attendance: " + student.Attendance);
Console.WriteLine("Grades: ");
foreach (var grade in student.Grades)
{
Console.WriteLine(grade.Key + ": " + grade.Value);
}
Console.WriteLine();
}
}
}
// Student class to represent individual students
class Student
{
public int Id { get; }
public string Name { get; }
public Dictionary<string, string> Grades { get; }
public List<string> Attendance { get; }
public List<string> ClassTimetable { get; }
public Student(int id, string name)
{
Id = id;
Name = name;
Grades = new Dictionary<string, string>();
Attendance = new List<string>();
ClassTimetable = new List<string>();
}
public void RecordAttendance(string status)
{
Attendance.Add(status);
}
public void EnterGrade(string subject, string grade)
{
Grades[subject] = grade;
}
public List<string> GetClassTimetable()
{
return ClassTimetable;
}
}
Student management system c# full source code 2024 pdf Student management system c# full source code 2024 pdf download Student management system c# full source code 2024 free download Student management system c# full source code 2024 github
Is CMS an LMS?
No, CMS and LMS are not the same, although they both deal with managing content. Here’s a breakdown of the key differences:
- Focus:
- CMS (Content Management System): Designed for creating, editing, and storing all types of content, including text, images, videos, and documents. It prioritizes content organization and presentation for a wider audience.
- LMS (Learning Management System): Specifically built for delivering and tracking educational content, like online courses, quizzes, and assignments. It focuses on the learning experience and individual learner progress.
- Features:
- CMS: Offers features for content creation tools, user permissions, version control, and website integration.
- LMS: Includes features for creating and managing courses, enrolling learners, delivering assessments, tracking progress, and reporting on learning outcomes.
- Target Users:
- CMS: Used by content creators, web developers, and marketers to manage website content.
- LMS: Used by educators, trainers, and administrators to create and deliver online learning experiences.
Think of it this way:
- A CMS is like a digital filing cabinet – it stores and organizes all sorts of content.
- An LMS is like a virtual classroom – it provides a structured learning environment with specific tools for teaching and learning.
While a CMS can be used to host educational content, it wouldn’t have the features for tracking learner progress, assessments, or reporting that an LMS offers
In some cases, a Learning Content Management System (LCMS) might be used. This combines elements of both a CMS and LMS, allowing for creating and managing learning content while offering some basic features for tracking learner progress.
How to create a student management system in C?
Building an SMS in C# involves several steps:
- Data Definition: Determine the data you’ll store for students (e.g., name, ID, grades, courses). Create classes in C# to represent students and potentially other entities like courses or teachers.
- User Interface: Design a user-friendly interface for interacting with the system. This could be a console application for basic functionality or a graphical user interface (GUI) using libraries like Windows Forms or WPF for a richer experience.
- Functionality Development: Implement core functionalities like:
- Adding Students: Allow users to enter student information and create new student records.
- Searching Students: Enable searching for students by name, ID, or other criteria.
- Updating Student Information: Provide options to edit existing student data (e.g., grades, courses).
- Deleting Students: Allow for student record removal (with confirmation).
- Reporting: Generate reports on student performance, attendance, or other relevant data.
- Data Persistence (Optional): For long-term data storage, consider using files (text files, CSV) or databases (like SQL Server) to persist student information beyond program termination.
What is the student management system?
Absolutely! Here’s a breakdown of what a Student Management System (SMS) is using lists and tables:
What is a Student Management System (SMS)?
Feature | Description |
---|---|
Function | Software application used by educational institutions to manage student data. |
What Data Does an SMS Manage?
Data Category | Examples |
---|---|
Student Information | Name, ID, contact information (address, phone number, email), demographics (date of birth, nationality) |
Enrollment & Course Management | Courses enrolled in, schedules, attendance records |
Grades & Performance | Grades for assignments and exams, overall performance metrics, transcripts |
Communication & Collaboration | Tools for communication between teachers, students, and parents (messaging, discussion forums) |
Benefits of Using an SMS
Benefit | Description |
---|---|
Increased Efficiency | Streamlines administrative tasks, reduces paperwork |
Improved Data Management | Centralized location for student information, easy access and retrieval |
Enhanced Communication | Facilitates communication between all stakeholders |
Data-Driven Decisions | Generates reports to analyze student performance and make informed decisions |
Personalized Learning | Enables personalized learning paths based on student data |
What is student management system PDF?
Student management system PDFs can cover a wide range of topics, but they generally fall into two categories:
- Informational PDFs: These PDFs provide an overview of student management systems, their functionalities, and benefits for educational institutions. They might also discuss different types of SMS available and their features.
- Project Reports or Tutorials (Less Common): A smaller number of PDFs might delve into the specifics of building a student management system. These could be project reports detailing the development process or tutorials guiding users on creating an SMS using a particular programming language.
Finding the Right Student Management System PDF
Unfortunately, there’s no single “go-to” PDF for student management systems. Here are some strategies to find the information you need:
- Search online: Use keywords like “student management system PDF,” “benefits of student information systems,” or “student management system functionalities.”
- Educational Institution Websites: Many schools and universities might have information about their chosen SMS on their websites.
- Academic Databases: If you have access to academic databases through a library or university affiliation, you might find research papers or reports on student management systems.
- Software Vendor Websites: Companies that develop and sell student management systems often provide documentation and user guides in PDF format on their websites.
Keep in mind:
- Informational PDFs are generally readily available online.
- Project reports or tutorials on building an SMS in a specific language might be more limited.
Additional Tips:
- Look for PDFs from reputable sources like educational institutions, software vendors, or government agencies.
- Consider the date of the PDF to ensure the information is current.
- If you’re looking for something specific, refine your search terms (e.g., “building a student management system in C# PDF”).
What is the aim of Student Management System project?
A Student Management System (SMS) project aims to revolutionize how educational institutions manage student data. Here’s a breakdown of its key goals using a table, along with explanations:
Aim | Description | Exterior Link [Optional] |
---|---|---|
Increased Efficiency | Automates tasks like enrollment, attendance, and grade recording, freeing up staff time. | https://www.edsembli.com/faqs/ |
Improved Data Management | Centralizes student information in a digital format for easy access and reduced errors. | |
Enhanced Communication | Facilitates communication between teachers, students, and parents through messaging, forums, or notifications. | |
Data-Driven Decisions | Generates reports for analyzing trends, monitoring progress, and making informed decisions. | |
Personalized Learning | Enables personalized learning paths based on student data to support individual needs. | https://camudigitalcampus.com/edtech-views/the-future-of-education-is-looking-smart-savvy-and-student-driven |
These links are not included in the table itself but can be referenced for further reading (avoiding directly embedding URLs). They provide additional information on the benefits of student information systems and the future of such systems.
Remember, the specific functionalities of an SMS project can vary depending on the needs of the institution.
However, the core goals listed above remain consistent: to create a more efficient, data-rich, and student-centered learning environment.
Conclusion to c# Project Student Management System
Our exploration of the Student Management System (SMS), a C# project brimming with potential, nears its end. As the virtual bell rings, we stand at the precipice of a transformed educational landscape.
This SMS isn’t a mere software solution; it’s a catalyst for change. It streamlines processes, empowers educators, and fosters a data-driven approach to learning. Imagine the possibilities:
- Reduced Costs: Paperwork minimization and improved resource allocation translate to significant financial savings for schools.
- Increased Efficiency: Teachers can reclaim precious time, dedicating it to what matters most – guiding young minds.
- Enhanced Communication: Real-time data and communication tools bridge the gap between teachers, parents, and students.
- Empowered Students: Students gain ownership of their learning journey with personalized feedback and progress tracking.
The SMS is just the beginning. This C# project lays the foundation for continuous improvement. With future iterations, we can integrate features like:
- Advanced Analytics: Dive deeper into student performance data to personalize learning paths even further.
- Mobile Accessibility: Empower students and parents to access information and communicate on the go.
- AI-Powered Insights: Utilize artificial intelligence to identify learning gaps and provide targeted support.
The call to action is clear. Let’s embrace the power of technology and build a future where education is not just efficient, but truly transformative. The Student Management System awaits, ready to orchestrate a symphony of success in every classroom. So, what are you waiting for? Let the coding commence!
Learn more
Student management system c# full source code 2024 free Student management system c# full source code 2024 download Student management system c# full source code 2024 example student management system project in c# with source code
0 Comments