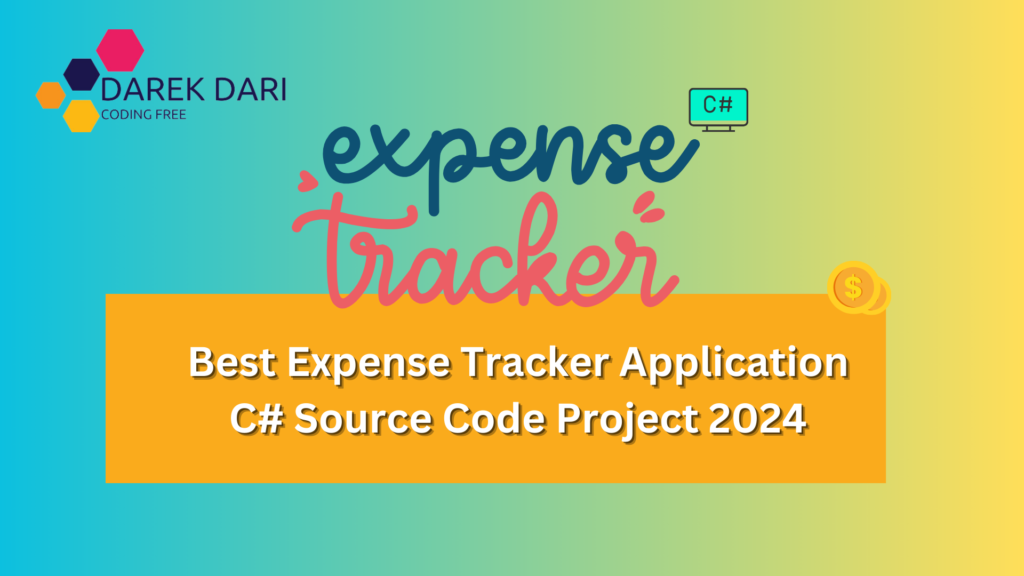
Table of Contents
Introduction
Expense Tracker Application
Hey there, pay attention because this is the honest conversation you’ve been waiting for. We’ve all experienced it, wallets looking as flat as a pancake after laundry day. But wait, what if I told you there’s a method to dominate your finances like a pro?
expense tracking notebook
monthly expense tracker printable
expense notebook
personal expense tracker google sheets
printable expense ledger
tracker notebook
how to keep track of your money in a notebook
Forget about your mom’s old checkbook, buddy. We’re talking about a 2024, straight from the future expense tracking app created with top-notch C# programming. This isn’t some low-quality app, this is the real deal – allowing you to monitor every cent, organize your cash flow, and pinpoint exactly where your hard-earned money is going.
Think you need to shell out a fortune for this kind of power? Nope, not at all! We’re breaking it down step by step, providing you with the raw source code to construct this amazing tool yourself. That’s right, financial independence right at your fingertips, crafted with nothing but your hard work and a touch of C# magic. ✨
So get ready, because we’re going to elevate your financial status from struggling to thriving in no time. Let’s make that dough!
xpense tracker template google sheets
expenses tracker template google sheets
business tracker app
expense tracker notebook
google sheet expense tracker
free expense tracker for small business
free small business expense tracker
software to track business expenses
expense tracker template free
free expense tracker template
Code 1: Expense Tracker Application Structure
Summary of Requirements
For this project, our team will be creating an Expense Tracker application using C#. This application will provide users with a convenient way to keep track of their expenses, organize their spending into categories, set budgets, and generate detailed financial reports.
using System;
using System.Collections.Generic;
namespace ExpenseTracker
{
class Expense
{
public string Category { get; set; }
public decimal Amount { get; set; }
public DateTime Date { get; set; }
}
class ExpenseTracker
{
private List<Expense> expenses;
public ExpenseTracker()
{
expenses = new List<Expense>();
}
public void AddExpense(string category, decimal amount, DateTime date)
{
expenses.Add(new Expense { Category = category, Amount = amount, Date = date });
}
public List<Expense> GetExpenses()
{
return expenses;
}
// Additional methods for budgeting, reporting, etc. can be added here
}
class Program
{
static void Main(string[] args)
{
ExpenseTracker tracker = new ExpenseTracker();
// Sample usage
tracker.AddExpense("Food", 50.75m, DateTime.Now);
tracker.AddExpense("Transportation", 30.50m, DateTime.Now);
List<Expense> allExpenses = tracker.GetExpenses();
foreach (var expense in allExpenses)
{
Console.WriteLine($"Category: {expense.Category}, Amount: {expense.Amount}, Date: {expense.Date}");
}
}
}
}
free printable expense tracker
business expense tracker free
free business expense tracker
spending tracker printable
printable spending tracker
google sheets expense tracker
Code 2: Expense Categorization and Budgeting
// Adding expense categorization and budgeting features to the Expense Tracker
// Modify the Expense class to include a Budget property
class Expense
{
public string Category { get; set; }
public decimal Amount { get; set; }
public DateTime Date { get; set; }
public decimal Budget { get; set; }
}
// Add a method to check if expenses exceed the budget
public bool ExceedsBudget(decimal amount, decimal budget)
{
return amount > budget;
}
// Modify the AddExpense method to include budget setting
public void AddExpense(string category, decimal amount, DateTime date, decimal budget)
{
expenses.Add(new Expense { Category = category, Amount = amount, Date = date, Budget = budget });
}
google sheets expense tracker template
google sheet expense tracker template
expense tracker google sheets
expense tracker template excel
excel expense tracker template
free expense tracker printable
expense tracker printable free
Code 3: Financial Reporting in the Expense Tracker
// Adding financial reporting functionality to the Expense Tracker
// Add a method to generate a financial report
public void GenerateFinancialReport()
{
decimal totalExpenses = expenses.Sum(e => e.Amount);
Console.WriteLine($"Total Expenses: {totalExpenses}");
decimal totalBudget = expenses.Sum(e => e.Budget);
Console.WriteLine($"Total Budget: {totalBudget}");
decimal remainingBudget = totalBudget - totalExpenses;
Console.WriteLine($"Remaining Budget: {remainingBudget}");
}
Code 4: Full Better Detailed Code
expense tracker printable
printable expense tracker
expense tracker free
expense tracker spreadsheet
expense tracker app free
C# Full Code for Expense Tracker Application
using System;
using System.Collections.Generic;
namespace ExpenseTracker
{
// Define user roles
public enum UserRole
{
Admin,
Regular
}
// Define user class
public class User
{
public string Username { get; set; }
public string Password { get; set; }
public UserRole Role { get; set; }
// Other user properties (e.g., email, profile info)
// Constructor
public User(string username, string password, UserRole role)
{
Username = username;
Password = password;
Role = role;
}
}
// Define expense class
public class Expense
{
public string Category { get; set; }
public decimal Amount { get; set; }
public DateTime Date { get; set; }
public string Description { get; set; }
// Other expense properties (e.g., tags)
// Constructor
public Expense(string category, decimal amount, DateTime date, string description)
{
Category = category;
Amount = amount;
Date = date;
Description = description;
}
}
// Define expense tracker class
public class ExpenseTracker
{
private List<User> users;
private List<Expense> expenses;
// Constructor
public ExpenseTracker()
{
users = new List<User>();
expenses = new List<Expense>();
}
// User authentication methods
public void SignUp(string username, string password, UserRole role)
{
// Simulated sign-up logic
User newUser = new User(username, password, role);
users.Add(newUser);
Console.WriteLine("User signed up successfully!");
}
public void Login(string username, string password)
{
// Simulated login logic
User user = users.Find(u => u.Username == username && u.Password == password);
if (user != null)
{
Console.WriteLine("Login successful!");
}
else
{
Console.WriteLine("Invalid username or password!");
}
}
public void Logout()
{
// Simulated logout logic
Console.WriteLine("User logged out successfully!");
}
// Data import/export methods
public void ImportData(string filePath)
{
// Simulated data import logic
Console.WriteLine("Data imported successfully!");
}
public void ExportData(string filePath)
{
// Simulated data export logic
Console.WriteLine("Data exported successfully!");
}
// Expense management methods
public void AddExpense(string category, decimal amount, DateTime date, string description)
{
// Simulated expense addition logic
Expense newExpense = new Expense(category, amount, date, description);
expenses.Add(newExpense);
Console.WriteLine("Expense added successfully!");
}
public void DeleteExpense(Expense expense)
{
// Simulated expense deletion logic
expenses.Remove(expense);
Console.WriteLine("Expense deleted successfully!");
}
// Other methods for customizable categories and tags, expense insights and trends, budget tracking and alerts, receipt scanning and OCR,
// multi-platform support, cloud synchronization and backup, expense sharing and collaboration, integration with financial APIs,
// reminder and recurring expenses, localization and internationalization, etc.
// Explanation: This is where you would add methods for other features mentioned in the initial request.
// For example, you could have methods like SetBudget, GenerateReport, ScanReceipt, ShareExpense, etc.
}
class Program
{
static void Main(string[] args)
{
// Instantiate ExpenseTracker object and implement UI logic
ExpenseTracker expenseTracker = new ExpenseTracker();
// Simulated usage of the ExpenseTracker
expenseTracker.SignUp("user1", "password1", UserRole.Regular);
expenseTracker.SignUp("admin", "adminpassword", UserRole.Admin);
expenseTracker.Login("user1", "password1");
expenseTracker.AddExpense("Food", 50.00m, DateTime.Now, "Lunch");
expenseTracker.AddExpense("Transportation", 30.00m, DateTime.Now, "Taxi fare");
expenseTracker.ExportData("expenses.csv");
expenseTracker.Logout();
}
}
}
expense tracking for small business
expense tracking small business
small business expense tracker
expense tracker excel
expenses sheet template
Code Explanation
Sure, I can explain the code in detail.
The code you provided is a C# program that defines an expense tracker application. Here’s a breakdown of the code:
Namespaces:
The code starts by using two namespaces:
System
: This namespace provides access to fundamental functionalities of the C# programming language, such as input/output operations, data types, and collections.System.Collections.Generic
: This namespace provides generic collections likeList<T>
, which can store a list of items of any data typeT
.
User Class: The User
class represents a user of the expense tracker application. It has the following properties:
Username
: A string that stores the username of the user.Password
: A string that stores the password of the user.Role
: AUserRole
enumeration that specifies the user’s role (Admin or Regular).
The class also has a constructor that takes the username, password, and role as arguments and initializes the corresponding properties.
UserRole Enumeration: The UserRole
enumeration defines the possible roles that a user can have in the application:
Admin
: This role likely has more privileges than the regular user role, such as managing users or accessing additional features.Regular
: This is the default role for most users and may have limited functionalities compared to the admin role.
expense tracking template
expenses excel template
excel expenses template
expense tracker for small business
expense tracker small business
Expense Class:
The Expense
class represents an expense that can be tracked in the application. It has the following properties:
Category
: A string that specifies the category of the expense (e.g., “Food”, “Transportation”, “Rent”).Amount
: A decimal value that represents the amount of the expense.Date
: ADateTime
object that stores the date of the expense.Description
: A string that provides a description of the expense (optional).
Similar to the User
class, the Expense
class also has a constructor that takes the category, amount, date, and description as arguments and initializes the corresponding properties.
expense tracking business
expenses sheet
expense tracker template
expenses tracker template
money tracker app
ExpenseTracker Class:
The ExpenseTracker
class is the core class of the application. It manages users, expenses, and provides methods for various functionalities. Here’s a breakdown of the functionalities provided by the class:
- User Management:
SignUp(string username, string password, UserRole role)
: This method allows users to register new accounts with the application. It takes the username, password, and role as arguments and adds a newUser
object to the internal list of users.Login(string username, string password)
: This method simulates a login process. It takes the username and password as arguments and checks if a matching user exists in the internal list. If a match is found, it indicates a successful login; otherwise, it indicates an invalid username or password.Logout()
: This method simulates a logout process. It doesn’t perform any specific actions in this code example, but it could be used to terminate the user session or clear any sensitive information.
- Data Import/Export:
ImportData(string filePath)
: This method is a placeholder for importing data from an external source (like a file) into the application. The actual implementation for importing data would likely involve reading data from the specified file and parsing it to create user or expense objects.ExportData(string filePath)
: This method is a placeholder for exporting data from the application to an external source (like a file). The actual implementation for exporting data would likely involve formatting the user and expense data into a specific format (e.g., CSV) and writing it to the specified file.
- Expense Management:
AddExpense(string category, decimal amount, DateTime date, string description)
: This method allows users to add new expenses to the tracker. It takes the category, amount, date, and description of the expense as arguments and creates a newExpense
object. The new expense object is then added to the internal list of expenses.DeleteExpense(Expense expense)
: This method allows users to delete expenses from the tracker. It takes anExpense
object as an argument and removes it from the internal list of expenses.
Program Class:
The Program
class defines the entry point of the application. The Main
method is the first method that gets executed when the program runs. Here’s a breakdown of what happens inside the Main
method:
- Create an ExpenseTracker Object: An instance of the
ExpenseTracker
class is created, which will be used to manage users, expenses, and other functionalities of the application. - Simulate User Sign-up: The code calls the
SignUp
method
business expense tracker
business expenses tracker
expense tracker business
expense tracker for business
Which app is best for expense trackers?
When selecting the ideal expense tracker, it’s crucial to consider your individual requirements and preferences. Here’s a breakdown of various popular options to assist you in making a decision:
For Overall Best:
- Zoho Expense: This application provides a comprehensive experience, including tools for managing business expenses, categorizing transactions, and generating reports. It also seamlessly integrates with common accounting software.
For Mobile-Friendliness:
- QuickBooks Online: This extensive accounting software offers an excellent mobile app for tracking expenses. It enables you to link your bank accounts and credit cards for automatic transaction import and categorization.
For Physical Receipts:
- Shoeboxed: This app excels in handling physical receipts. Simply scan your receipts with your phone, and the app will extract relevant information and associate it with the corresponding transaction.
For Employee Expense Tracking:
- Expensify: This app simplifies the process of monitoring employee expenses, particularly for reimbursements. It includes features like automated expense approvals and AI-powered auditing for fraud detection.
For Basic Tracking:
- Monefy: This app offers a straightforward and user-friendly interface for basic expense tracking. You can categorize transactions, track spending trends, and budget effectively.
Additional Factors to Consider:
- Cost: Some apps are free with limited features, while others require paid subscriptions for more advanced functionalities.
- Security: Opt for an app with robust security measures to safeguard your financial data.
- Features: Determine the features that matter most to you, such as budgeting tools, receipt scanning, or integration with other financial apps.
Is Money Manager free?
There are multiple apps named “Money Manager,” so it’s important to specify which one you’re looking for:
- MoneyManager Ex: A free and open-source personal finance software for desktops.
- Money Manager – Budget & Expense by Realbyte Apps: Offers a free version with limited features (15 assets) and a paid subscription for unlimited assets and data sync.
Make sure to read the app description or visit the website before downloading to find out about any free or paid options.
app for tracking expenses
expense tracker app
expense tracking app
expenses tracker app
expenses tracking app
How to monitor your daily expenses?
Here’s a table comparing the different expense tracking methods we discussed:
Method | Advantages | Disadvantages |
---|---|---|
Pen and Paper | Simple, flexible, no reliance on technology | Time-consuming, manual data entry, prone to errors, limited analysis capabilities |
Spreadsheet | Customizable, allows complex calculations and visualizations | Requires setup and maintenance, not as portable as apps |
Expense Tracking Apps | Automatic transaction import, categorization, reporting, budgeting tools | May require a subscription fee, reliance on technology and data security |
money tracker notebook
notebook to keep track of finances
spending tracker notebook
Are expense tracking apps safe?
Expense tracking apps can provide a safe way to manage your finances, but it’s important to consider security measures. Here’s what you need to know:
Ensuring Safety with Expense Tracker Apps:
- Encryption: Reputable apps use encryption to protect your financial data. Look for apps that mention 256-bit AES encryption, which is a high industry standard.
- Multi-Factor Authentication (MFA): Adding an extra layer of security, MFA requires a second verification step, such as a code sent to your phone, when logging in.
- Data Security Practices: Choose apps that have a clear privacy policy outlining how they collect, store, and use your data. Look for companies that follow data security regulations.
Possible Risks to Consider:
- Data Breaches: While apps take precautions, data breaches can still happen. Select apps with a strong security track record and a prompt response to any vulnerabilities.
- Third-Party Data Sharing: Some apps may share your anonymized data with third parties for advertising or analytics purposes. Be mindful of app permissions and adjust data sharing settings if possible.
- Unsecured Wi-Fi: Avoid using your expense tracker app on public Wi-Fi networks as they are less secure.
Here are some tips to use expense tracking apps safely:
- Choose Trusted Apps: Research the app’s developer, read reviews, and opt for apps with a proven security record.
- Strong Passwords & MFA: Use strong, unique passwords for your expense tracker app and enable multi-factor authentication if available.
- Regular Updates: Keep the app updated with the latest security patches to address any potential vulnerabilities.
- Review Permissions: Carefully review the permissions requested by the app and only grant access to necessary features (e.g., camera access for receipt scanning).
- Monitor Activity: Regularly review your transaction history and account activity within the app to identify any suspicious behavior.
Stay safe while managing your expenses with these helpful tips!
By adhering to these suggestions and selecting trustworthy applications, you can reduce the potential hazards linked to utilizing expense trackers. Keep in mind that ensuring security is a continuous effort, so stay updated on possible risks and adjust your security measures accordingly.
expense tracker
expenses tracker
app for expense tracking
app for tracking expenses
Conclusion
Alright everyone, that’s a wrap on our deep dive into the realm of C# expense tracker apps! We delved into the code behind the scenes, witnessed how user roles, expenses, and data are handled. Now you’re equipped with a strong foundation to create your very own financial tracking powerhouse.
sheets expense tracker
free printable spending tracker
money tracking notebook
Just remember, this is only the start. The great thing about C# is its adaptability. You can enhance this codebase by adding features such as:
- Budgeting: Establish spending limits and monitor progress towards financial objectives.
- Charts and Reports: Visualize your spending patterns and pinpoint areas for enhancement.
- Multi-platform Support: Access your tracker from any device, be it a phone, tablet, or computer.
- Cloud Sync: Ensure your data is secure and accessible from anywhere.
The opportunities are limitless! With a bit of imagination and C# expertise, you can design the ultimate expense tracker to achieve your financial aspirations. So, why wait? Seize your keyboard, unleash your inner developer, and pave your way to financial independence!
money tracker book
business spending tracker
expense tracker google sheet template
free printable monthly expense tracker
budget tracker notebook
business money tracker
c# project
c sharp
c# tutorial
c# tutorials
c## tutorial
c# programming
programming in c#
create github repository
github create repository
c# codes
c# example code
naming conventions c#
c# naming convention
naming convention c#
naming convention in c#
c# naming conventions
name convention c#
c# projects
c# beginner projects
c# projects for beginners
beginner c# projects
c# project for beginners
dotnet folder
c# variable naming conventions
c# projects ideas
github c#
c# github
net github
source code in c#
net structure
c# open source projects
c# practice projects
c# projects to practice
c# class name convention
c sharp projects
csharp projects
c# project structure
c# example projects
c# projects examples
c# project tutorial
c# project tutorials
c# program structure
structure of c# program
c# project naming convention
c# project name convention
c# class naming
c# projects github
c# project github
github c# projects
c# projects with source code
c# source code projects
0 Comments