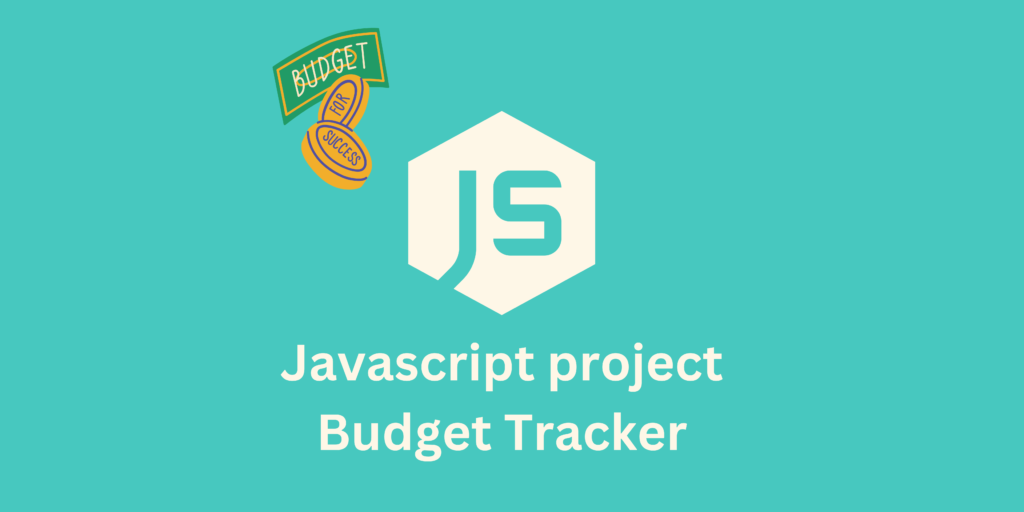
Budget Tracker JavaScript project : We are going to create a budget tracker that enables users to enter their earnings and outgoings and shows their current budget.
1- HTML :
First, we need to create a basic HTML structure for the Budget Tracker application. Here are the steps:
- To add income and expenses, create an HTML form with two input boxes and a submit button.
<form id="budget-form">
<div>
<label for="income-description">Income Description:</label>
<input type="text" id="income-description" placeholder="Enter a description">
<label for="income-amount">Amount:</label>
<input type="number" id="income-amount" placeholder="Enter an amount">
<button type="submit" id="add-income-btn">Add Income</button>
</div>
<div>
<label for="expense-description">Expense Description:</label>
<input type="text" id="expense-description" placeholder="Enter a description">
<label for="expense-amount">Amount:</label>
<input type="number" id="expense-amount" placeholder="Enter an amount">
<button type="submit" id="add-expense-btn">Add Expense</button>
</div>
</form>
To display the list of revenue and expenses, create an HTML table with three columns: Description, Amount, and Date.
<table id="budget-items">
<thead>
<tr>
<th>Description</th>
<th>Amount</th>
<th>Date</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
- Create an HTML element to display the current balance.
<div>
<h3>Balance:</h3>
<p id="balance-amount">0.00</p>
</div>
2- JavaScript :
The Budget Tracker application needs features added, thus we must use JavaScript next. the following steps:
- First, create an empty array to store income and expense objects. Each object should have a description, amount, and date.
let budgetItems = [];
- The variables for the input fields, add button, budget list table, and balance amount display should then be defined.
const incomeDescriptionInput = document.getElementById('income-description');
const incomeAmountInput = document.getElementById('income-amount');
const expenseDescriptionInput = document.getElementById('expense-description');
const expenseAmountInput = document.getElementById('expense-amount');
const addIncomeBtn = document.getElementById('add-income-btn');
const addExpenseBtn = document.getElementById('add-expense-btn');
const budgetList = document.getElementById('budget-items');
const balanceAmount = document.getElementById('balance-amount');
- Make an addition function for income or expenses. The function must accept user input, build a new expense or revenue object, and add it to the array.
function addBudgetItem(description, amount, type) {
const budgetItem = {
description: description,
amount: parseInt(amount),
type: type,
date: new Date().toLocaleDateString()
};
budgetItems.push(budgetItem);
}
- Create a function to display the list of income and expenses in the table. Loop through the array and display each object in the table with the description, amount, and date.
function displayBudgetItems() {
budgetTable.innerHTML = '';
for (let i = 0; i < budgetItems.length; i++) {
const item = budgetItems[i];
const row = document.createElement('tr');
const descriptionCell = document.createElement('td');
const amountCell = document.createElement('td');
const dateCell = document.createElement('td');
descriptionCell.innerText = item.description;
amountCell.innerText = item.amount.toFixed(2);
dateCell.innerText = item.date.toLocaleDateString();
row.appendChild(descriptionCell);
row.appendChild(amountCell);
row.appendChild(dateCell);
if (item.type === 'income') {
row.classList.add('income');
} else {
row.classList.add('expense');
}
budgetTable.appendChild(row);
}
}
- Make a function to figure out the overall revenue and expenditure. Calculate the total income and expense separately using a loop across the array.
function calculateTotal() {
let incomeTotal = 0;
let expenseTotal = 0;
for (let i = 0; i < budgetItems.length; i++) {
const item = budgetItems[i];
if (item.type === 'income') {
incomeTotal += item.amount;
} else {
expenseTotal += item.amount;
}
}
const balance = incomeTotal - expenseTotal;
balanceAmount.innerText = balance.toFixed(2);
}
- Make an event listener for the button that says “Add Income.” Clear the input fields, show the list of revenue and expenses, run the addBudgetItem function with the input values when the button is clicked, compute the total, and display the list of income and expenses.
addIncomeBtn.addEventListener('click', function(event) {
event.preventDefault();
const description = incomeDescriptionInput.value;
const amount = incomeAmountInput.value;
addBudgetItem(description, amount, 'income');
incomeDescriptionInput.value = '';
incomeAmountInput.value = '';
displayBudgetItems();
calculateTotal();
});
- Make an event listener for the button that says “add expense.” Clear the input fields, show the list of revenue and expenses, run the addBudgetItem function with the input values when the button is clicked, compute the total, and display the list of income and expenses.
addExpenseBtn.addEventListener('click', function(event) {
event.preventDefault();
const description = expenseDescriptionInput.value;
const amount = expenseAmountInput.value;
addBudgetItem(description, amount, 'expense');
expenseDescriptionInput.value = '';
expenseAmountInput.value = '';
displayBudgetItems();
calculateTotal();
});
- When the page loads, use the displayBudgetItems and calculateTotal functions to show any existing revenue and cost items as well as the current balance.
displayBudgetItems();
calculateTotal();
3- CSS:
The Budget Tracker program needs some basic CSS to style it, therefore we must add it last. These are a few examples of styles:
#budget-form {
display: flex;
justify-content: space-between;
}
#budget-form div {
display: flex;
flex-direction: column;
align-items: flex-start;
}
#budget-form label {
margin-bottom: 0.5rem;
}
#budget-form input {
margin-bottom: 1rem;
padding: 0.5rem;
border: 1px solid #ccc;
border-radius: 4px;
}
#budget-form button {
padding: 0.5rem 1rem;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
#budget-items {
width: 100%;
margin-top: 2rem;
border-collapse: collapse;
}
#budget-items th {
background-color: #4caf50;
color: #fff;
padding: 0.5rem;
text-align: left;
}
#budget-items td {
border: 1px solid #ccc;
padding: 0.5rem;
}
#budget-items td:first-child {
width: 50%;
}
#balance-amount {
font-size: 2rem;
font-weight: bold;
margin-top: 2rem;
}
I’m done now! Now that you have a working Budget Tracker software, you can let users input their income and expenses and see what their current budget is.
4- JavaScript project: Budget Tracker Conclusion
In summary, the Budget Tracker project is an excellent opportunity to practice JavaScript skills such as working with arrays, DOM manipulation, and event handling. It allows users to track their income and expenses, providing valuable experience in data management and user interface design. The project includes features such as adding income and expenses, displaying a list of items, calculating the total budget, and deleting items from the list. It can be extended and improved in many ways, such as adding sorting and filtering options, implementing data visualization features, or integrating with a database.
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments