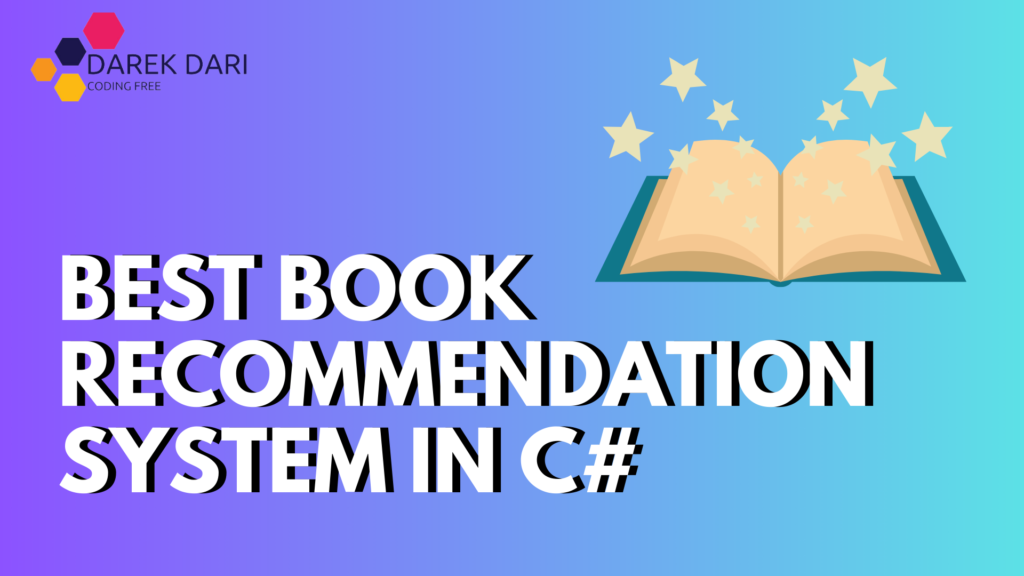
Table of Contents
Hey friends! Today, I want to share with you how I built an Enhanced Book Recommendation System using C#.
It’s a fun little C# project with source code that allows users to get book recommendations based on genres, add new books, and even rate them. Let’s dive into it step by step!
Best C# Pojects With Source Code
Project Setup
Creating the C# Project
First, I opened Visual Studio and created a new Console Application project named EnhancedBookRecommendationSystem
. This will be our main workspace.
Step 1: Define the Book Class
I started by creating a Book
class to represent the books in our system. This class holds important details about each book, including its title, author, genre, and rating.
class Book
{
public string Title { get; }
public string Author { get; }
public string Genre { get; }
public double Rating { get; set; }
public Book(string title, string author, string genre, double rating)
{
Title = title;
Author = author;
Genre = genre;
Rating = rating;
}
public override string ToString()
{
return $"{Title} by {Author} - Genre: {Genre} - Rating: {Rating}";
}
}
Explanation
- Properties: The class has properties for the title, author, genre, and rating.
- Constructor: The constructor initializes these properties when a new book object is created.
- ToString Method: This method formats the book details into a readable string.
Step 2: Implement the Main Program Logic
Next, I set up the main logic of the application. This part includes loading books from a file and providing a menu for users to interact with.
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
namespace EnhancedBookRecommendationSystem
{
class Program
{
static string filePath = "books.txt";
static List<Book> books = new List<Book>();
static void Main(string[] args)
{
LoadBooks();
while (true)
{
Console.WriteLine("1. View Recommendations");
Console.WriteLine("2. Add a Book");
Console.WriteLine("3. Rate a Book");
Console.WriteLine("4. Exit");
Console.Write("Choose an option: ");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
ViewRecommendations();
break;
case "2":
AddBook();
break;
case "3":
RateBook();
break;
case "4":
SaveBooks();
return;
default:
Console.WriteLine("Invalid option. Please try again.");
break;
}
}
}
Explanation
- File Path: The
filePath
variable stores the name of the file where we’ll save our book data. - Book List: A list named
books
holds all the book objects. - Main Loop: The application runs in a loop, presenting users with a menu to choose from.
c# project with source code
c# projects for beginners with source code
c# projects with source code
c# projects with source code and database
Loading and Saving Books
To make the data persistent, I implemented methods to load and save books from/to a text file:
static void LoadBooks()
{
if (File.Exists(filePath))
{
var lines = File.ReadAllLines(filePath);
foreach (var line in lines)
{
var parts = line.Split(',');
if (parts.Length == 4)
{
var book = new Book(parts[0], parts[1], parts[2], double.Parse(parts[3]));
books.Add(book);
}
}
}
}
static void SaveBooks()
{
using (var writer = new StreamWriter(filePath))
{
foreach (var book in books)
{
writer.WriteLine($"{book.Title},{book.Author},{book.Genre},{book.Rating}");
}
}
}
Explanation
- Loading Books: When the program starts, it checks if the file exists and loads the book details into the list.
- Saving Books: Before exiting, the application writes all book details back to the file.
Step 3: Implementing User Features
Now for the fun part! I added methods to view recommendations, add new books, and rate existing ones.
View Recommendations
This method allows users to get book recommendations based on their favorite genre.
static void ViewRecommendations()
{
Console.Write("Enter your favorite genre (e.g., Classic, Dystopian): ");
string genre = Console.ReadLine();
var recommendedBooks = books.Where(b => b.Genre.Equals(genre, StringComparison.OrdinalIgnoreCase)).ToList();
Console.WriteLine("Recommended Books:");
if (recommendedBooks.Count > 0)
{
foreach (var book in recommendedBooks)
{
Console.WriteLine(book);
}
}
else
{
Console.WriteLine("No books found in this genre.");
}
}
Best C# Projects
- Expense Tracker Application C# Source Code
- Fitness Tracker Application C# Source Code
- Hospital Management System C# Source Code
- Inventory Management System C# Source Code
- C# project Shopping Cart with Source Code
- Student Management System C#
- C# Chatbot
Add a Book
Users can add new books using this method:
static void AddBook()
{
Console.Write("Enter the book title: ");
string title = Console.ReadLine();
Console.Write("Enter the author: ");
string author = Console.ReadLine();
Console.Write("Enter the genre: ");
string genre = Console.ReadLine();
Console.Write("Enter the initial rating (0 to 5): ");
double rating = double.Parse(Console.ReadLine());
var newBook = new Book(title, author, genre, rating);
books.Add(newBook);
Console.WriteLine("Book added successfully!");
}
Rate a Book
This method allows users to update the rating of a book they’ve read:
static void RateBook()
{
Console.Write("Enter the title of the book you want to rate: ");
string title = Console.ReadLine();
var book = books.FirstOrDefault(b => b.Title.Equals(title, StringComparison.OrdinalIgnoreCase));
if (book != null)
{
Console.Write("Enter your new rating (0 to 5): ");
double newRating = double.Parse(Console.ReadLine());
book.Rating = newRating;
Console.WriteLine("Rating updated successfully!");
}
else
{
Console.WriteLine("Book not found.");
}
}
Step 4: Running the Application
To run the application, just hit the start button in Visual Studio. You’ll see a menu with options to view recommendations, add books, or rate existing ones. The data will be saved in books.txt
in the project directory, so it persists between sessions!
Future Enhancements
Looking ahead, there are plenty of ways to make this project even better:
- GUI Version: Transition to a graphical user interface using Windows Forms or WPF.
- Database Support: Implement a database like SQLite or SQL Server for more robust data management. This would turn our project into one of those C# projects with source code and database that many beginners seek.
- Advanced Recommendations: Use algorithms to recommend books based on user ratings and preferences.
Conclusion
And that’s it! I hope you found this guide helpful and inspiring for your own projects. Building a C# project with source code like this is a great way to practice C# projects for beginners with source code. Feel free to experiment with the code and make it your own.
Happy coding! If you’re looking for more ideas, check out various C# projects with source code available online. You can find lots of C# sample code to help you along the way!
0 Comments