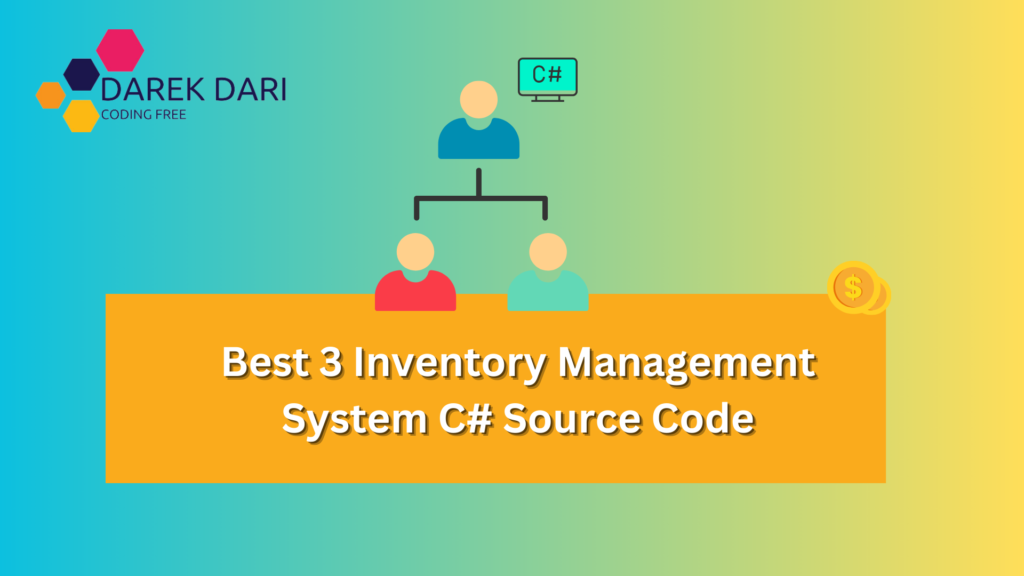
Table of Contents
Introduction
This Inventory Management System project is fully functional and developed using C# Language with MySQL Database. It is specifically designed for first-year and second-year IT students who are working on their college projects.
inventory management systems
inventory manager software
inventory management software
software for inventory management
inventory management system
The project includes all the necessary features for tracking inventories and sales. The system is user-friendly and the desktop application is well-implemented, resembling real-life scenarios.
Code 1: Inventory Management System with Classes
Item Class
- Properties: ItemID, Name, Description, Price, Quantity, etc.
- Methods: Constructors, Getters, Setters, etc.
public class Item
{
public int ItemID { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public int Quantity { get; set; }
// Constructor
public Item(int id, string name, string description, decimal price, int quantity)
{
ItemID = id;
Name = name;
Description = description;
Price = price;
Quantity = quantity;
}
}
Inventory Class
- Methods to add/remove items, update quantities, search items, etc.
using System;
using System.Collections.Generic;
using System.Linq;
public class Inventory
{
private List<Item> items;
public Inventory()
{
items = new List<Item>();
}
public void AddItem(Item item)
{
items.Add(item);
}
public void RemoveItem(Item item)
{
items.Remove(item);
}
public void UpdateItem(int itemId, Item updatedItem)
{
Item itemToUpdate = items.FirstOrDefault(i => i.ItemID == itemId);
if (itemToUpdate != null)
{
itemToUpdate.Name = updatedItem.Name;
itemToUpdate.Description = updatedItem.Description;
itemToUpdate.Price = updatedItem.Price;
itemToUpdate.Quantity = updatedItem.Quantity;
}
else
{
Console.WriteLine("Item not found.");
}
}
public Item SearchItem(int itemId)
{
return items.FirstOrDefault(i => i.ItemID == itemId);
}
public void DisplayInventory()
{
Console.WriteLine("Inventory Items:");
foreach (var item in items)
{
Console.WriteLine($"ID: {item.ItemID}, Name: {item.Name}, Quantity: {item.Quantity}");
}
}
public void RestockItem(int itemId, int quantityToAdd)
{
Item itemToRestock = items.FirstOrDefault(i => i.ItemID == itemId);
if (itemToRestock != null)
{
itemToRestock.Quantity += quantityToAdd;
}
else
{
Console.WriteLine("Item not found.");
}
}
public void SellItem(int itemId, int quantityToSell)
{
Item itemToSell = items.FirstOrDefault(i => i.ItemID == itemId);
if (itemToSell != null)
{
if (itemToSell.Quantity >= quantityToSell)
{
itemToSell.Quantity -= quantityToSell;
}
else
{
Console.WriteLine("Insufficient quantity.");
}
}
else
{
Console.WriteLine("Item not found.");
}
}
}
system inventory management
system for inventory management
inventory system management
inventory software
inventory system
inventory systems
These methods expand the functionality of your Inventory
class:
- UpdateItem: Updates the details of an existing item based on its ID.
- SearchItem: Searches for an item in the inventory based on its ID.
- DisplayInventory: Displays all items currently in the inventory.
- RestockItem: Increases the quantity of a specific item in the inventory.
- SellItem: Decreases the quantity of a specific item in the inventory when sold.
User Interface
- Create a GUI using Windows Forms or WPF to interact with the inventory.
- Include forms for adding/removing items, viewing inventory, generating reports, etc.
Here’s a basic example of how you can create a user interface using Windows Forms for interacting with the inventory management system:
- Open Visual Studio and create a new Windows Forms project.
- Add the necessary controls to your form for adding/removing items, viewing inventory, and generating reports.
- Implement event handlers for these controls to interact with your
Inventory
class.
inventory management small business software
inventory management software small business
inventory software for small business
Below is a simplified example demonstrating how you can design the main form with buttons to perform various inventory management tasks:
using System;
using System.Windows.Forms;
namespace InventoryManagementSystem
{
public partial class MainForm : Form
{
private Inventory inventory;
public MainForm()
{
InitializeComponent();
inventory = new Inventory();
}
private void btnAddItem_Click(object sender, EventArgs e)
{
// Open a new form to add an item
AddItemForm addItemForm = new AddItemForm();
if (addItemForm.ShowDialog() == DialogResult.OK)
{
// Add the item to inventory
inventory.AddItem(addItemForm.Item);
MessageBox.Show("Item added successfully.");
}
}
private void btnRemoveItem_Click(object sender, EventArgs e)
{
// Open a new form to remove an item
RemoveItemForm removeItemForm = new RemoveItemForm(inventory);
if (removeItemForm.ShowDialog() == DialogResult.OK)
{
MessageBox.Show("Item removed successfully.");
}
}
private void btnViewInventory_Click(object sender, EventArgs e)
{
// Open a new form to view inventory
ViewInventoryForm viewInventoryForm = new ViewInventoryForm(inventory);
viewInventoryForm.ShowDialog();
}
private void btnGenerateReport_Click(object sender, EventArgs e)
{
// Generate and display the inventory report
string report = inventory.GenerateInventoryReport();
MessageBox.Show(report, "Inventory Report");
}
}
}
In this scenario, you will need to create placeholder forms called AddItemForm, RemoveItemForm, and ViewInventoryForm. These forms will be responsible for handling the tasks of adding, removing, and viewing items, respectively.
inventory tracking system
system inventory
inventory management software for small businesses
To generate the inventory report, you will also need to implement the GenerateInventoryReport() method in your Inventory class.
Feel free to customize the appearance and functionality of these forms to meet your specific requirements. Furthermore, you can enhance the user experience by adding error handling and validation mechanisms.
Reports Generation
- Implement functionality to generate reports such as current inventory levels, items sold, etc.
- Utilize libraries like Crystal Reports or built-in .NET features.
using System;
using System.Collections.Generic;
using System.IO;
public class Inventory
{
private List<Item> items;
public Inventory()
{
items = new List<Item>();
}
// Other methods (AddItem, RemoveItem, UpdateItem, etc.)
public string GenerateInventoryReport()
{
// Create a StringBuilder to store the report content
var reportBuilder = new System.Text.StringBuilder();
// Append report header
reportBuilder.AppendLine("Inventory Report");
reportBuilder.AppendLine($"Date: {DateTime.Now}");
reportBuilder.AppendLine();
// Append item details
foreach (var item in items)
{
reportBuilder.AppendLine($"Item ID: {item.ItemID}");
reportBuilder.AppendLine($"Name: {item.Name}");
reportBuilder.AppendLine($"Description: {item.Description}");
reportBuilder.AppendLine($"Price: ${item.Price}");
reportBuilder.AppendLine($"Quantity: {item.Quantity}");
reportBuilder.AppendLine();
}
// Return the report as a string
return reportBuilder.ToString();
}
// Other methods...
}
To generate a basic text-based inventory report, we use this method. The report provides the current date and information about each item in the inventory.
To make use of this feature, simply call the GenerateInventoryReport() method from your user interface, like when a button is clicked. For instance:
private void btnGenerateReport_Click(object sender, EventArgs e)
{
// Generate and display the inventory report
string report = inventory.GenerateInventoryReport();
// Save the report to a file
string filePath = "inventory_report.txt";
File.WriteAllText(filePath, report);
MessageBox.Show("Inventory report generated and saved to file: " + filePath, "Report Generated");
}
This piece of code generates the inventory report once the button labeled btnGenerateReport is clicked. It then saves the report to a text file named “inventory_report.txt” in the same folder as the executable. Feel free to modify the file path and format of the report to suit your requirements.
inventory software small business
small business software with inventory
software for small business inventory
inventory management systems software
inventory management software system
inventory management solution
Database Integration (Optional)
- Use a database like SQL Server or SQLite to persist inventory data.
- Implement CRUD operations to interact with the database.
class Program
{
static void Main(string[] args)
{
// Create an instance of Inventory
Inventory inventory = new Inventory();
// Add some items
Item item1 = new Item(1, "Laptop", "High-performance laptop", 999.99m, 10);
Item item2 = new Item(2, "Mouse", "Wireless mouse", 19.99m, 50);
inventory.AddItem(item1);
inventory.AddItem(item2);
// Remove an item
inventory.RemoveItem(item2);
// Display remaining items
foreach (var item in inventory.Items)
{
Console.WriteLine($"{item.Name}: {item.Quantity}");
}
}
}
Keep in mind that this is only the beginning. Based on your individual requirements, you might have to incorporate error handling, authentication, validation, and other advanced functionalities.
Code 2: Inventory Management System with File Handling
Picture yourself as the owner of a mystical shop, in need of a system to keep track of all the amazing items you have in stock. You opt to develop an entertaining program named the “Inventory Management System” using C#.
Within this enchanting system, there are two primary spells (methods) that aid you in managing your inventory:
- AddItemToFile:This spell assists you in adding new items to your inventory list. You simply provide the name of the item and the quantity you possess, and it magically records it in a special book known as “inventory.txt”.
It’s akin to jotting down on a piece of paper, “I have 50 potions of Product A” and “I have 100 enchanted swords of Product B”. - GenerateInventoryReportFromFile: This spell proves to be extremely useful when you wish to view the items available in your store. It reads from the mystical book “inventory.txt” and generates a magical report for you. It’s similar to perusing your inventory list and exclaiming, “Oh look, I have 50 potions of Product A and 100 enchanted swords of Product B.”.
using System;
using System.IO;
public class InventoryManagementSystem
{
public void AddItemToFile(string itemName, int quantity)
{
using (StreamWriter writer = new StreamWriter("inventory.txt", true))
{
writer.WriteLine($"{itemName},{quantity}");
}
}
public void GenerateInventoryReportFromFile()
{
string[] lines = File.ReadAllLines("inventory.txt");
Console.WriteLine("Current Inventory:");
foreach (var line in lines)
{
string[] parts = line.Split(',');
Console.WriteLine($"Item: {parts[0]}, Quantity: {parts[1]}");
}
}
}
class Program
{
static void Main()
{
InventoryManagementSystem inventorySystem = new InventoryManagementSystem();
inventorySystem.AddItemToFile("Product A", 50);
inventorySystem.AddItemToFile("Product B", 100);
inventorySystem.GenerateInventoryReportFromFile();
}
}
As the mystical store owner, you unlock your enchanted book (code) and unleash the spell to conjure your very own Inventory Management System.
inventory management systems for small business
inventory management system for small business
inventory management systems small business
inventory management system small business
inventory management types
Utilizing the “AddItemToFile” incantation, you effortlessly add Product A and Product B to your inventory. Afterwards, you employ the “GenerateInventoryReportFromFile” enchantment to reveal the contents of your stock.
Behold! Like magic, your inventory report materializes on your screen, displaying all the items and quantities available in your store.
And there you have it! With your extraordinary Inventory Management System, you can effortlessly keep tabs on all your enchanting items and ensure the seamless operation of your store.
Code 3: Inventory Management System with Database Integration
Are you ready for a thrilling tech journey? Picture yourself as a wizard in charge of a mystical shop brimming with magical treasures. To ensure you can easily manage your enchanted items, you’ve decided to develop a remarkable “Inventory Management System” using C#.
using System;
using System.Data.SqlClient;
public class InventoryManagementSystem
{
private string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;";
public void AddItemToDatabase(string itemName, int quantity)
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string query = $"INSERT INTO Inventory (ItemName, Quantity) VALUES ('{itemName}', {quantity})";
SqlCommand command = new SqlCommand(query, connection);
command.ExecuteNonQuery();
}
}
public void GenerateInventoryReportFromDatabase()
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string query = "SELECT * FROM Inventory";
SqlCommand command = new SqlCommand(query, connection);
SqlDataReader reader = command.ExecuteReader();
Console.WriteLine("Current Inventory:");
while (reader.Read())
{
Console.WriteLine($"Item: {reader["ItemName"]}, Quantity: {reader["Quantity"]}");
}
}
}
}
class Program
{
static void Main()
{
InventoryManagementSystem inventorySystem = new InventoryManagementSystem();
inventorySystem.AddItemToDatabase("Product A", 50);
inventorySystem.AddItemToDatabase("Product B", 100);
inventorySystem.GenerateInventoryReportFromDatabase();
}
}
Let me explain how it operates:
This code is a simple inventory management system implemented in C# using ADO.NET to interact with a SQL Server database. Let me break it down for you:
- Namespace and Libraries: The code begins by importing necessary namespaces:
System
: The base namespace for fundamental types and core functionalities.System.Data.SqlClient
: This namespace contains classes for accessing a SQL Server database.
- InventoryManagementSystem Class: This class encapsulates methods for managing inventory:
- Fields:
connectionString
: It holds the connection string required to connect to the SQL Server database.
- Methods:
AddItemToDatabase(string itemName, int quantity)
: Adds an item with its quantity to the database. It creates a new SQL connection, opens it, executes an SQL insert query to add the item and quantity to theInventory
table.GenerateInventoryReportFromDatabase()
: Retrieves all items and their quantities from the database and prints them. It opens a connection to the database, executes an SQL select query to retrieve data from theInventory
table, and prints each item and its quantity.
- Fields:
- Program Class:
- Main Method:
- Instantiates an
InventoryManagementSystem
object. - Adds two items to the database using
AddItemToDatabase
method. - Generates an inventory report using
GenerateInventoryReportFromDatabase
method.
- Instantiates an
- Main Method:
- Execution:
- Inside the
Main
method, anInventoryManagementSystem
object is created. - Two items, “Product A” and “Product B”, are added to the database with quantities 50 and 100, respectively.
- Then, the inventory report is generated, which retrieves all items from the database and prints them with their quantities.
- Inside the
types of inventory management
what is inventory management system
what is inventory management systems
inventory management systems examples
example of inventory management system
examples of inventory management systems
inventory management system examples
Yet, there is a major security flaw in this code called SQL injection. By directly combining strings to construct SQL queries, the code becomes vulnerable to SQL injection attacks. To reduce this risk, it is advised to utilize parameterized queries.
What are the 3 inventory control systems?
There is no one standard set of three specific “inventory control systems” that everyone agrees on. Nonetheless, there are three main approaches to managing inventory levels, each offering unique benefits and uses.
Sure, here is a table that compares the three inventory control systems:
System | Concept | Advantages | Disadvantages |
---|---|---|---|
Periodic Inventory | Manual counts at regular intervals | Low initial investment, suitable for small businesses | Time-consuming, data not real-time, prone to errors |
Perpetual Inventory | Real-time tracking with software and technology | Accurate data, reduces stockouts/overstocking, streamlines processes | Requires software/hardware investment, complex to implement |
Just-in-Time (JIT) | Receive goods only when needed for production or sales | Reduces storage costs, minimizes waste, improves efficiency | Requires strong planning/supplier relationships, vulnerable to disruptions |
what is a inventory management system
inventory systems types
types of inventory system
types of inventory systems
inventory management systems types
inventory management system types
Which inventory management system is best?
There isn’t a one-size-fits-all inventory management system that works for every business. The best choice depends on various factors unique to your business:
Business Size and Complexity:
- For large businesses, a perpetual inventory system or dedicated software is likely the most suitable due to the volume and complexity of inventory.
- Small businesses may find that a periodic system or even spreadsheets are sufficient for basic tracking, especially with slow-moving inventory.
Inventory Type:
- Perishable goods or high-demand items require a perpetual system for real-time data and control to prevent stockouts.
- Less volatile inventory may be manageable with a periodic system, but a perpetual system can still offer valuable insights.
types of inventory management systems
types of inventory management system
what is an inventory system
what is inventory system
inventory control system examples
inventory control systems examples
inventory systems examples
Budget:
- If you have a limited budget, consider cloud-based perpetual inventory software solutions for affordability.
- With a larger budget, on-premise systems could be an option, but weigh the upfront cost against potential long-term benefits.
- For a very limited budget, starting with a periodic system or spreadsheets may be necessary, but be aware of potential inefficiencies and inaccuracies.
Desired Level of Control:
- High control: Perpetual systems offer more granular control and visibility.
- Less control: Periodic systems provide a less detailed snapshot.
Additional Suggestions:
- Start with a simpler approach: If you’re unsure about which inventory management system to choose, it’s a good idea to begin with a periodic system or spreadsheets. This will give you a basic understanding of how inventory management works and allow you to gradually transition to a more advanced system as your business grows or your inventory becomes more complex.
- Consider a hybrid approach: Instead of relying solely on one system, you can combine different aspects of multiple systems. For example, you could use a perpetual system for critical items that require real-time tracking, while using a periodic system for less crucial items that don’t need constant monitoring. This way, you can optimize your inventory management based on the specific needs of each item.
- Take advantage of software trials: Many inventory management software solutions offer free trials. This gives you the opportunity to test out different systems and see which one best fits your needs. By experimenting with different software options, you can make an informed decision and choose the most effective inventory management system for your business.
By carefully evaluating your specific business needs and considering these additional tips, you’ll be able to make a well-informed decision about the most suitable inventory management system for your situation.
Which database is best for inventory management?
There isn’t a single “perfect” database for inventory management, as the best choice depends on your specific requirements. Here’s a comparison of the two main types of databases to consider:
- Relational Databases (e.g., MySQL, PostgreSQL):
- Advantages:
- Organized data format: Great for storing and handling well-defined data points like product IDs, descriptions, quantities, prices, suppliers, etc.
- Strong connections: Allows for efficient searching and analysis of interconnected data. For instance, you can easily locate all products from a specific supplier or spot items with low stock levels.
- ACID compliance: Guarantees data integrity and consistency through transactions (e.g., updating stock levels after a sale).
- Established technology: Widely used and backed by a large developer community.
- Disadvantages:
- Schema definition: Requires careful planning to set up the data structure (tables, columns, relationships). Changes may involve complex adjustments later on.
- Scalability limitations: Managing huge datasets or intricate queries can be tough with extensive inventories.
- NoSQL Databases (e.g., MongoDB):
- Advantages:
- Adaptable schema: Fits data that doesn’t neatly fit into predefined tables and columns. This is handy for storing product descriptions, images, or other unstructured information.
- Horizontal scaling: Simple to add more servers to handle increasing data volumes.
- Disadvantages:
- Weaker connections: Might need extra development effort to handle complex data relationships compared to relational databases.
- Eventual consistency: Data updates may not be immediately reflected across all servers, potentially causing temporary inconsistencies.
- Less established technology: Might require more specialized skills for administration and development compared to relational databases.
When selecting the Right Database:
Take into account these factors to decide which type best suits your inventory management needs:
- Data Structure: If your inventory data is clearly defined and has strong relationships (e.g., products linked to suppliers), a relational database may be a good choice.
- Data Volume and Scalability: If you expect a large inventory or complex queries, a NoSQL database’s horizontal scaling could be advantageous.
- Flexibility: If you require storing unstructured data or expect frequent schema changes, a NoSQL database might provide more flexibility.
- Technical Expertise: Think about your team’s familiarity with different database technologies. Relational databases are generally more established, while NoSQL might need specialized skills.
In some instances, you could even consider a hybrid approach:
- Utilize a relational database for core inventory data with well-defined structures.
- Utilize a NoSQL database to store unstructured data such as product descriptions or images.
Ultimately, the optimal approach depends on your specific needs. Exploring specific database solutions within each category (MySQL, PostgreSQL for relational; MongoDB for NoSQL) can assist you in narrowing down the options based on features and functionalities.
Inventory Management Types
Inventory management involves implementing various methods and techniques to optimize the movement of goods in and out of your business.
Consider these different types of inventory management:
- Inventory Control Systems:
- Periodic Inventory System: This manual approach involves conducting physical inventory counts periodically, such as weekly, monthly, or quarterly. Stock levels are determined by subtracting these counts from previous inventory records and recent purchases. It is suitable for small businesses or those with slow-moving inventory, but it can be time-consuming and prone to errors.
- Perpetual Inventory System: This method utilizes software and technology to track inventory levels in real-time. Whenever an item is received, sold, used, or damaged, the system updates the inventory record accordingly. This provides accurate data, reduces stockouts, and streamlines processes. However, it requires an investment in software and hardware.
- Inventory Management Methods:
- Just-in-Time (JIT): This method aims to minimize inventory holding costs by receiving goods only when they are needed for production or sales. It relies on accurate forecasting and strong supplier relationships. However, it can be vulnerable to disruptions in the supply chain.
- ABC Analysis: This method classifies inventory items into categories A (high-value/usage), B (moderate importance), and C (low-value). It helps prioritize inventory control efforts for critical items.
- Economic Order Quantity (EOQ): This approach involves calculating the optimal order quantity to minimize total inventory costs, including ordering and holding costs.
- Minimum Order Quantity (MOQ): This is a predetermined minimum amount to order from a supplier, often used to negotiate better pricing.
- Safety Stock: This involves maintaining a buffer of inventory to avoid stockouts caused by unexpected demand fluctuations or disruptions in the supply chain.
- Days Sales of Inventory (DSI): This metric measures the average time it takes to sell your current inventory. A high DSI might indicate overstocking, while a low DSI could suggest potential stockouts.
These different inventory management methods and systems can help you effectively manage your inventory and improve the overall flow of goods in your business.
Different Inventory Management Practices:
- Demand Forecasting:
Anticipating future inventory demand to effectively plan your purchases. - Warehouse Management:
Streamlining storage and retrieval processes within your warehouse for optimal efficiency. - Dropshipping:
A fulfillment method where you don’t hold inventory, instead, customer orders are forwarded to a third-party supplier who directly ships to the customer. - Vendor Managed Inventory (VMI):
A collaborative approach where the supplier takes charge of managing your inventory levels, ensuring you always have sufficient stock available.
Choosing the right inventory management type for your business depends on various factors, such as:
- Size and complexity of your business
- Type of inventory (perishable, high-demand, etc.)
- Budget constraints
- Desired level of control
By understanding these different practices and considering your specific needs, you can select the most effective inventory management strategies to optimize your stock levels, reduce costs, and enhance overall efficiency.
Conclusion
In the world of inventory management, it’s crucial to focus on efficiency and dependability. When looking for top-notch C# source code for an inventory management system, make sure to choose solutions that prioritize strong functionality, easy customization, and compliance with industry standards.
We’ve carefully selected the top three inventory management systems written in C#, known for their extensive features, smooth integration with SQL databases, and commitment to software development best practices.
inventory management system meaning
inventory management systems definition
inventory management system definition
Whether you’re handling inventory for a small company or a big corporation, these C# source codes offer a reliable base for streamlining your inventory operations and enhancing operational efficiency.
0 Comments