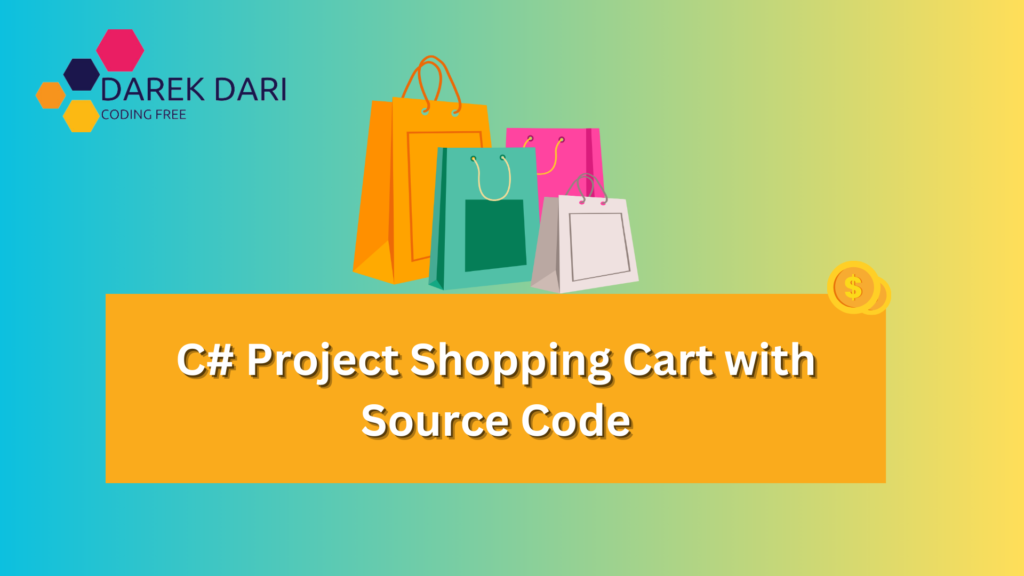
Table of Contents
Introduction
Welcome to our guide on building a shopping cart application using C# project! If you’re looking to dive into the world of software development or enhance your coding skills, you’re in the right place.
In this tutorial, we’ll walk you through the process of creating a fully functional shopping cart system using C#.
Getting Started with C# Shopping Cart
So, you’re ready to embark on your journey to create a shopping cart application in C#. Before we dive into coding, let’s take a quick look at what a shopping cart is and why it’s essential. 🤔
What is a Shopping Cart?
In the realm of e-commerce, a shopping cart is like your virtual basket in a physical store. It allows users to select and store items they intend to purchase while browsing through an online store.
It keeps track of the chosen products, quantities, and prices, enabling customers to review and modify their selections before proceeding to checkout.
Why Build a Shopping Cart in C#?
Now, you might be wondering, why C#? Well, C# project is a powerful and versatile programming language commonly used for building various types of applications, including web applications, desktop software, and games.
c# shopping cart
Its syntax is easy to understand, making it an excellent choice for beginners and seasoned developers alike. Plus, with the .NET framework’s extensive libraries and tools, developing robust and scalable applications becomes a breeze.
Building the Shopping Cart Application
Alright, it’s time to roll up our sleeves and start coding! But first, let’s outline the key components of our shopping cart application.
Classes in C# Project Shopping Cart
Our shopping cart application will consist of two main classes: ShoppingCart
and Product
. These classes will handle the management of products and the shopping cart itself. Here’s a brief overview of each class:
ShoppingCart Class
The ShoppingCart
class represents the shopping cart and manages the list of products. It provides methods to add and remove products, clear the cart, and calculate the total cost of all items in the cart.
List<Product> products
: This private member variable holds the list of products in the shopping cart.AddProduct(Product product)
: Adds a product to the shopping cart.RemoveProduct(Product product)
: Removes a product from the shopping cart.ClearCart()
: Clears the shopping cart by removing all products.CalculateTotal()
: Calculates the total price of all products in the cart.
public class ShoppingCart
{
private List<Product> products;
public ShoppingCart()
{
products = new List<Product>();
}
public void AddProduct(Product product)
{
products.Add(product);
}
public void RemoveProduct(Product product)
{
products.Remove(product);
}
public void ClearCart()
{
products.Clear();
}
public decimal CalculateTotal()
{
decimal total = 0;
foreach (var product in products)
{
total += product.Price;
}
return total;
}
}
Product Class
The Product
class represents a product with a name and price. It simply holds the information about a specific product.
string Name
: Property to get or set the name of the product.decimal Price
: Property to get or set the price of the product.
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
Implementing the ShoppingCart Class
Now that we’ve outlined our classes let’s dive into the implementation of the ShoppingCart
class.
This class will serve as the backbone of our shopping cart application, handling all operations related to managing products.
ShoppingCart Class Implementation Details
The ShoppingCart
class is responsible for managing the list of products added to the cart. Let’s take a closer look at its implementation:
- List of Products: The
products
variable is a private member of theShoppingCart
class, representing the list of products in the cart. - AddProduct Method: This method allows users to add a product to the shopping cart. It takes a
Product
object as a parameter and adds it to theproducts
list. - RemoveProduct Method: Users can remove a product from the shopping cart using this method. It also takes a
Product
object as a parameter and removes it from theproducts
list. - ClearCart Method: If a user wants to start afresh, they can use this method to clear the shopping cart by removing all products from the
products
list. - CalculateTotal Method: This method calculates the total price of all products in the shopping cart. It iterates over the
products
list and accumulates the price of each product, returning the calculated total as a decimal value.
// Insert code snippet for ShoppingCart class implementation here
c# shopping cart
Implementing the Product Class
Next up, let’s implement the Product
class, which represents individual products in our shopping cart.
Product Class
The Product
class is straightforward, holding basic information about a product:
- Name Property: This property gets or sets the name of the product.
- Price Property: This property gets or sets the price of the product.
// Insert code snippet for Product class implementation here
Testing the Shopping Cart Application 🧪
Now that we’ve implemented our shopping cart classes let’s put them to the test! We’ll create a simple console application to demonstrate how the shopping cart works.
Main Method Testing the Application
In the Main
method, we’ll create an instance of the ShoppingCart
class, add sample products to the cart, perform operations like calculating the total price, removing a product, and clearing the cart.
public class Program
{
public static void Main(string[] args)
{
// Create a new shopping cart
ShoppingCart cart = new ShoppingCart();
// Create some sample products
Product product1 = new Product { Name = "Product 1", Price = 10.99m };
Product product2 = new Product { Name = "Product 2", Price = 5.99m };
Product product3 = new Product { Name = "Product 3", Price = 7.99m };
// Add products to the cart
cart.AddProduct(product1);
cart.AddProduct(product2);
cart.AddProduct(product3);
// Calculate and print the total
decimal total = cart.CalculateTotal();
Console.WriteLine("Total: $" + total);
// Remove a product from the cart
cart.RemoveProduct(product2);
// Calculate and print the updated total
total = cart.CalculateTotal();
Console.WriteLine("Updated Total: $" + total);
// Clear the cart
cart.ClearCart();
Console.WriteLine("Cart cleared. Total: $" + cart.CalculateTotal());
}
}
Product Information Table
When building a shopping cart application, it’s essential to display product information clearly for the users. Let’s create a table to showcase the details of each product added to the cart, including their names and prices.
Product Name | Price |
---|---|
Product 1 | $10.99 |
Product 2 | $5.99 |
Product 3 | $7.99 |
This table gives users a quick overview of the products they’ve added to their cart, making it easier for them to review their selections before making a purchase.
Feel free to customize this table further based on your application’s requirements. You can add more columns, such as quantity or product description, to provide additional information to your users. Remember, the goal is to create a user-friendly shopping experience that makes it easy for customers to browse, select, and purchase products.
Learn more
c# shopping cart
Conclusion
Congratulations on completing your C# project! 🎉 You’ve successfully built a functional shopping cart application using C#. By following this tutorial, you’ve learned essential concepts such as class implementation, method creation, and object manipulation.
Now, you can take this C# project further by adding more features and enhancing its functionality.
Remember, coding is all about creativity and problem-solving. Don’t hesitate to experiment and explore new ideas as you continue your journey as a developer. Keep practicing, and you’ll master C# and software development in no time. Happy coding for C# Project! 🚀
Sure, let’s create another section with a table to provide additional information about the products in our shopping cart.
Frequently Asked Questions (FAQs)
Got questions about our shopping cart C# Project? Check out these FAQs for answers!
Q: How do I add products to my shopping cart?
A: To pop goodies into your cart, simply hit the “Add to Cart” button next to the stuff you fancy. Your cart’s just a click away—peek at it by tapping the cart icon up top!
Q: Can I remove items from my shopping cart?
A: Absolutely! If you’re having second thoughts about something in your cart, just give it the boot. Each item’s got a “Remove” button—click it, and poof, it’s gone!
Q: How can I view the total cost of my shopping cart?
A: Easy-peasy! The total bill’s waiting for you at the bottom of your cart. It tallies up everything you’ve tossed in, plus any extra fees like taxes or shipping.
Q: Is it possible to save my shopping cart for later?
A: Right now, we’re not equipped for cart-saving magic. But no worries! Jot down what caught your eye, and swing back whenever you’re ready to seal the deal.
Q: What payment methods do you accept?
A: We’re flexible! Whether you’re flashing plastic or prefer PayPal, we’ve got you covered. Visa, Mastercard, Amex, Discover—you name it, we take it!
Q: How long will items remain in my shopping cart?
A: Your cart’s a holding zone ’til you check out or toss stuff out manually. But remember, cart time doesn’t equal item reservation, so snag what you want before it’s snatched!
Q: Can I apply discount codes or coupons to my order?
A: Heck yeah! Got a nifty discount code or coupon? Punch it in during checkout, and watch those prices drop like confetti! Enjoy the sweet savings!
Q: How do I add products to my shopping cart?
A: Adding products to your shopping cart in our C# project is a breeze! Simply call the AddProduct
method of the ShoppingCart
class and pass in the Product
object you want to add.
Q: Can I remove items from my shopping cart programmatically?
A: Absolutely! With our C# project, removing items from your shopping cart is as simple as calling the RemoveProduct
method of the ShoppingCart
class and specifying the Product
object you want to remove.
Q: How can I calculate the total cost of my shopping cart using C#?
A: Calculating the total cost of your shopping cart is a snap with our C# project! Just call the CalculateTotal
method of the ShoppingCart
class, and it will return the total price of all the products in your cart.
Q: Is it possible to implement discounts or promotions in the shopping cart?
A: Yes, you can! With our C# project, you have the flexibility to implement discounts or promotions in your shopping cart logic. You can adjust the prices of individual products or apply discounts based on certain conditions.
Q: Can I store the shopping cart data in a database using C#?
A: Absolutely! Our C# project allows you to integrate with databases seamlessly. You can store shopping cart data, such as product details and quantities, in a database using SQL commands or through an ORM framework like Entity Framework.
Q: Are there any predefined UI components for displaying the shopping cart in C#?
A: While our C# project focuses on the backend logic of a shopping cart, you can easily integrate it with UI components in various frontend frameworks like WinForms, WPF, or ASP.NET. You have the freedom to design the shopping cart interface according to your preferences and requirements.
Still curious? Shoot us a message, and our crew will steer you in the right direction. Happy shopping with our C# Project! 🛒✨
c# shopping cart
1 Comment
Binance - rejestracja · March 20, 2024 at 3:26 am
Your article helped me a lot, is there any more related content? Thanks!