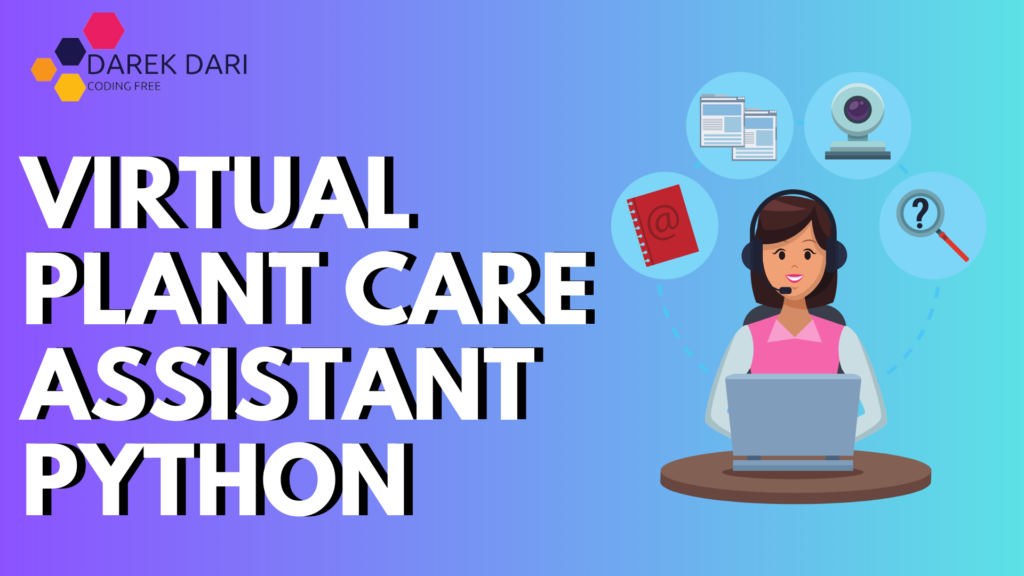
Table of Contents
If you’re a plant lover, you know how important it is to keep track of when to water your plants, how much sunlight they need, and how to keep them healthy.
Well, with a little help from Python, you can build your very own Virtual Plant Care Assistant that will help you manage your plants and remind you when it’s time to give them some attention.
"virtual assistant ai"
100 python projects
100 python projects for beginners
100 python projects for final year
100 python projects github
100 python projects with source code
21 python mini projects with code
In this blog, I’ll guide you through building a simple plant care assistant in Python. You’ll learn how to track watering schedules, set reminders, and keep your plants happy — all from the comfort of your computer!
This Virtual Plant Care Assistant Python tutorial is a great project for beginners and those who want to explore more about integrating AI into practical applications.
Why Build a Plant Care Assistant?
Managing plants can be tricky — different plants need different care. With a Virtual Plant Care Assistant in Python, you can:
- Track Watering Schedules: Easily keep tabs on which plants need water and when.
- Get Reminders: Have your assistant notify you when it’s time to water each plant.
- Store Plant Info: Keep details like plant type, last watering date, and watering frequency, so you always know what your plants need.
And the best part? You don’t need to be a programming expert to get started. Let’s dive in and create your Virtual Plant Care Assistant Python free application!
Step-by-Step Guide: Building Your Virtual Plant Care Assistant
We’ll use Python to create this assistant. It’s simple but powerful, and you can always improve and expand it over time. You can even find the full project code on Virtual Plant Care Assistant Python GitHub if you want to explore more or contribute!
Here’s the code for a basic version of the Virtual Plant Care Assistant:
import datetime
class Plant:
def __init__(self, name, watering_frequency, last_watered):
self.name = name
self.watering_frequency = watering_frequency # in days
self.last_watered = last_watered # last watered date as datetime object
def needs_watering(self):
# Calculate the number of days since the last watering
today = datetime.datetime.today()
days_since_watered = (today - self.last_watered).days
return days_since_watered >= self.watering_frequency
def water(self):
# Update the last watered date to today
self.last_watered = datetime.datetime.today()
print(f"{self.name} has been watered!")
# List to hold all plant objects
plants = []
# Function to add new plants to the list
def add_plant(name, watering_frequency, last_watered):
last_watered_date = datetime.datetime.strptime(last_watered, "%Y-%m-%d")
plant = Plant(name, watering_frequency, last_watered_date)
plants.append(plant)
print(f"{name} has been added to your plant collection!")
# Function to check which plants need watering
def check_plants():
for plant in plants:
if plant.needs_watering():
print(f"{plant.name} needs watering!")
else:
print(f"{plant.name} doesn't need watering yet.")
# Function to water all plants
def water_all_plants():
for plant in plants:
plant.water()
# Example usage
add_plant("Rose", 3, "2024-12-21") # Watering frequency of 3 days, last watered on 21st December 2024
add_plant("Cactus", 7, "2024-12-17") # Watering frequency of 7 days, last watered on 17th December 2024
check_plants() # Check which plants need watering
water_all_plants() # Water all plants, updating their last watered date
check_plants() # Check again after watering
Breaking Down the Code
Let’s walk through the code step by step to make sure you understand how everything works:
- Plant Class:
We start by creating a class calledPlant
to represent each of your plants. The class stores the name, how often it needs to be watered, and the date it was last watered. Theneeds_watering()
method checks if it’s time to water based on the plant’s watering schedule, and thewater()
method updates the plant’s last watered date.
class Plant:
def __init__(self, name, watering_frequency, last_watered):
self.name = name
self.watering_frequency = watering_frequency
self.last_watered = last_watered
def needs_watering(self):
today = datetime.datetime.today()
days_since_watered = (today - self.last_watered).days
return days_since_watered >= self.watering_frequency
def water(self):
self.last_watered = datetime.datetime.today()
print(f"{self.name} has been watered!")
- Adding Plants:
Theadd_plant()
function lets you easily add new plants to your collection. You just need to provide the plant’s name, watering frequency (in days), and the date it was last watered. The last watered date is converted from a string (e.g., “2024-12-21”) into adatetime
object, so it can be used for calculations.
def add_plant(name, watering_frequency, last_watered):
last_watered_date = datetime.datetime.strptime(last_watered, "%Y-%m-%d")
plant = Plant(name, watering_frequency, last_watered_date)
plants.append(plant)
print(f"{name} has been added to your plant collection!")
- Checking Which Plants Need Watering:
Thecheck_plants()
function goes through each of your plants and checks if they need watering by calling theneeds_watering()
method. It then prints out whether each plant needs water or not.
def check_plants():
for plant in plants:
if plant.needs_watering():
print(f"{plant.name} needs watering!")
else:
print(f"{plant.name} doesn't need watering yet.")
- Watering All Plants:
Thewater_all_plants()
function updates thelast_watered
date for all of your plants to today’s date. It’s like giving them a good drink of water all at once!
def water_all_plants():
for plant in plants:
plant.water()
How It Works
- Add Plants:
You can add as many plants as you like using theadd_plant()
function. Just specify the plant name, watering frequency, and last watered date. - Check Watering Needs:
Thecheck_plants()
function will tell you which plants need water. It checks the current date and compares it to the last watered date. - Water Your Plants:
When it’s time to water, use thewater_all_plants()
function to update the last watered date for each plant. - Repeat:
Keep track of your plants, and your assistant will remind you when to water them.
Enhancing the Virtual Plant Care Assistant
Now that you have the basics down, here are some ways you can make your app even better:
- Reminders: You can use Python libraries like schedule or APScheduler to send you reminders to water your plants.
- Tracking Growth: Add more attributes to your plant class like height, sunlight needs, or pot size.
- Interactive GUI: Use Tkinter or another Virtual Assistant using Python with GUI to create a friendly interface where you can easily add, update, and view your plants.
Conclusion
The Virtual Plant Care Assistant is a great way to combine your love of plants with Python programming. Not only does it help you take care of your plants, but it also gives you a chance to practice coding with real-world applications. You can expand it in many ways, like adding notifications, tracking plant health, or even integrating with weather APIs to give personalized care tips based on the weather.
For those of you looking to take this project even further, this is also a great starting point for creating a Virtual Assistant Python code or even a more complex Jarvis AI assistant Python code. If you’re interested in Virtual Assistant AI projects, this is a fantastic way to get your feet wet before diving into more advanced AI tasks.
Have fun building your assistant and taking care of your plants — they’ll thank you for it!
0 Comments