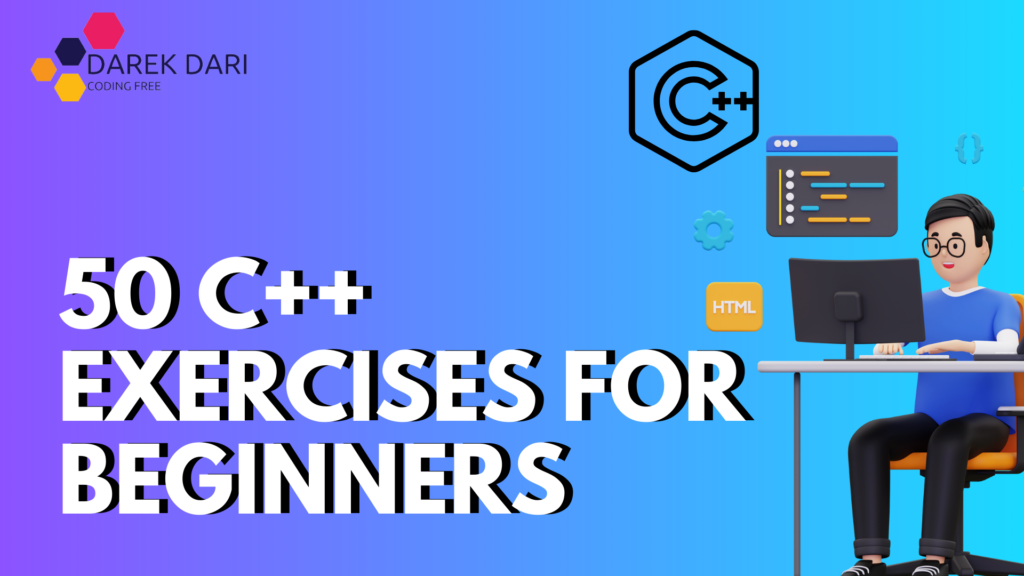
If you’re just starting out with C++ programming, the best way to get comfortable with the language is by practicing coding challenges. While understanding the syntax and theory is important, nothing beats learning by doing. These C++ exercises for beginners will give you the opportunity to tackle real-world problems, get familiar with C++ syntax, and gradually build your coding skills.
Each exercise is designed to introduce a fundamental concept of C++ and will help you apply these concepts in a practical way. With clear explanations and C++ code examples that you can copy-paste and experiment with, you’ll gain confidence in your programming journey.
C++ exercices
C++ exercises
C++ function exercises
C++ practice exercises
C++ basic programs for practice
C++ beginner exercises
C++ beginner practice problems
C++ book with exercises
C++ challenges beginner
C++ challenges for beginners
C++ code practice
C++ coding exercises
C++ coding practice
C++ course with exercises
C++ examples for practice
C++ Exercises for Beginners
1. Hello World: Your First C++ Program
Let’s start with a simple program to print “Hello World” to the screen:
#include <iostream> // Required for input/output operations
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Prints "Hello World"
return 0; // Successful program termination
}
Explanation:
This is the simplest C++ program you can write. It introduces you to the cout
statement, which is used to print data to the console. The endl
outputs a newline after the text, and the return 0;
indicates successful program execution. This helps you get familiar with the basic structure of a C++ program.
2. Input and Output: Greeting the User
Next, let’s write a program that takes the user’s name and age as input and prints a personalized greeting:
#include <iostream>
using namespace std;
int main() {
string name; // Variable for the user's name
int age; // Variable for the user's age
cout << "Enter your name: ";
cin >> name; // User enters their name
cout << "Enter your age: ";
cin >> age; // User enters their age
cout << "Hello, " << name << "! You are " << age << " years old." << endl; // Displays the greeting
return 0;
}
Explanation:
In this program, we use cin
to capture user input. The cin
object is used to read the name and age from the user. After that, cout
displays the greeting message, incorporating the user’s input. This teaches you how to handle input/output in C++ and how to use variables to store data entered by the user.
3. Adding Two Numbers
Now, let’s write a program that adds two numbers entered by the user:
#include <iostream>
using namespace std;
int main() {
int num1, num2; // Variables for two numbers
cout << "Enter two numbers: ";
cin >> num1 >> num2; // User enters two numbers
cout << "The sum is: " << num1 + num2 << endl; // Displays the sum
return 0;
}
Explanation:
This exercise introduces arithmetic operations in C++. We ask the user for two numbers, and then we simply add them using the +
operator. The program then prints the result using cout
. This shows how you can perform basic calculations in C++.
C++ exercices
C++ exercise
C++ exercises
C++ exercises and solutions
C++ exercises for beginners
C++ functions exercises
C++ if-else exercises
C++ library management system project
C++ online exercises
C++ practice
C++ practice exercises
C++ practice problems
C++ practice problems for beginners
C++ practice programs
4. Basic Calculator: Addition, Subtraction, Multiplication, Division
Let’s create a simple calculator that can perform addition, subtraction, multiplication, and division:
#include <iostream>
using namespace std;
int main() {
int num1, num2; // Two numbers for calculation
char op; // Operator for the calculation (+, -, *, /)
cout << "Enter two numbers: ";
cin >> num1 >> num2;
cout << "Enter an operator (+, -, *, /): ";
cin >> op;
if (op == '+') {
cout << "Result: " << num1 + num2 << endl;
} else if (op == '-') {
cout << "Result: " << num1 - num2 << endl;
} else if (op == '*') {
cout << "Result: " << num1 * num2 << endl;
} else if (op == '/') {
if (num2 != 0) {
cout << "Result: " << num1 / num2 << endl; // Prevents division by zero
} else {
cout << "Cannot divide by zero!" << endl; // Handles division by zero
}
} else {
cout << "Invalid operator!" << endl; // Handles invalid operators
}
return 0;
}
Explanation:
This calculator program uses conditional statements (if-else
) to check the operator entered by the user and performs the corresponding arithmetic operation. It also handles the case of division by zero to prevent errors. This exercise helps you understand how to use conditional logic in C++.
Whether you're just starting or looking to level up your C++ programming skills, practicing C++ exercises is essential for growth. Beginners can dive into beginner C++ exercises to grasp the basics, while those with more experience can tackle advanced C++ exercises or dive into C++ exercises for practice to refine their knowledge. Explore a variety of resources like W3Schools C++ exercises or W3Resource C++ exercises to find step-by-step tutorials and challenges. If you're focusing on object-oriented programming (OOP), you'll find dedicated OOP C++ exercises along with C++ function exercises, pointer exercises, and classes and objects in C++ exercises. Whether you're working on recursion C++ exercises, learning how to use arrays with C++ array exercises for beginners, or mastering advanced C++ programming exercises, these challenges will help you build a solid foundation.
For C++ interview coding exercises and solutions, many books and PDFs are available, including C++ exercises and solutions PDF and C++ exercises with solutions. You can also download free resources, such as C++ exercises book pdf and C++ programming exercises and solutions for beginners PDF. If you're specifically looking for algorithmic challenges, there are many C++ algorithm exercises and resources for dynamic memory allocation, exception handling, and C++ data types exercises. And for those diving into more complex topics, C++ debugging exercises, binary tree C++ exercises, and C++ interview coding exercises will challenge even the most seasoned programmers. No matter your skill level, there are C++ exercises available to improve your understanding and mastery of this powerful language.
5. Check if a Number is Even or Odd
In this program, you’ll check if a number is even or odd:
#include <iostream>
using namespace std;
int main() {
int num; // The number to check
cout << "Enter a number: ";
cin >> num; // User inputs the number
if (num % 2 == 0) {
cout << num << " is even." << endl; // Even number check
} else {
cout << num << " is odd." << endl; // Odd number check
}
return 0;
}
Explanation:
This exercise uses the modulus operator (%
) to determine whether a number is divisible by 2. If the remainder is 0, the number is even; otherwise, it is odd. This teaches you how to perform simple arithmetic operations and make decisions in C++.
6. Prime Number Checker
Let’s now write a program to check if a number is prime:
#include <iostream>
using namespace std;
int main() {
int num;
bool isPrime = true; // Flag to indicate if the number is prime
cout << "Enter a number: ";
cin >> num;
for (int i = 2; i <= num / 2; ++i) { // Loop to check divisibility
if (num % i == 0) {
isPrime = false; // Number is divisible, hence not prime
break;
}
}
if (isPrime) {
cout << num << " is a prime number." << endl;
} else {
cout << num << " is not a prime number." << endl;
}
return 0;
}
Explanation:
In this program, we use a for loop to check if the number is divisible by any integer from 2 to num/2. If the number is divisible by any of these integers, it is not a prime number. This teaches you how to use loops and conditional statements in C++ to solve a problem.
C++ practice questions for beginners
C++ program practice
C++ programming exercises
C++ programming exercises and solutions
C++ programming exercises and solutions for beginners
C++ programming exercises and solutions for beginners PDF
C++ programs for practice
C++ training exercises
7. Multiplication Table
Let’s build a program to print the multiplication table of a given number:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
for (int i = 1; i <= 10; ++i) {
cout << num << " x " << i << " = " << num * i << endl; // Print multiplication result
}
return 0;
}
Explanation:
This program uses a for loop to generate the multiplication table of the input number. By multiplying the number with values from 1 to 10, it prints the corresponding results. This exercise helps you practice using loops for repetitive tasks in C++.
8. Fibonacci Sequence
The Fibonacci sequence is a series where each number is the sum of the two preceding ones. Let’s write a program to print the first n terms of the Fibonacci sequence:
#include <iostream>
using namespace std;
int main() {
int n;
cout << "Enter the number of terms: ";
cin >> n;
int first = 0, second = 1, next;
cout << "Fibonacci Sequence: ";
for (int i = 1; i <= n; ++i) {
if (i == 1) {
cout << first << " ";
continue;
}
if (i == 2) {
cout << second << " ";
continue;
}
next = first + second;
first = second;
second = next;
cout << next << " ";
}
cout << endl;
return 0;
}
Explanation:
This program demonstrates how to calculate and print the Fibonacci sequence. We start with the first two terms (0 and 1) and calculate the next terms by adding the two previous terms. This helps you practice iteration and variable updating in C++.
If you’re looking to strengthen your C++ programming skills, practicing C++ exercises is a great way to start. Whether you’re just beginning your journey with beginner C++ exercises or you’re diving into advanced C++ exercises, there’s a wide range of resources to explore. From C++ exercises for beginners to C++ algorithm exercises, and even C++ exercises with solutions in PDF format, you’ll find plenty of opportunities to test your knowledge. If you’re particularly interested in object-oriented programming (POO) in C++, there are POO C++ exercises corrigés to help you grasp the core concepts.
For those aiming for an in-depth understanding, C++ exercises on classes and objects, binary tree C++ exercises, and C++ debugging exercises will take your skills to the next level. Whether you’re learning from books like the best C++ exercises book or accessing free downloadable C++ exercises PDF, you’ll find resources for C++ exercises with solutions, C++ file handling exercises, and C++ exercises for interview preparation. No matter your level, from beginner to advanced C++ exercises, there’s something for everyone. So, if you’re ready to challenge yourself, start practicing with these C++ exercises, and enhance your coding proficiency!
Conclusion
These C++ exercises for beginners will help you get familiar with the basics of the language. As you go through each exercise, you’ll encounter different aspects of C++ programming, including loops, conditional statements, functions, and more. By solving these challenges, you’ll develop a solid foundation in C++ that will prepare you for more advanced topics.
By practicing these exercises, you’re not only learning syntax but also gaining valuable experience in how to structure C++ programs and solve problems efficiently. Keep experimenting with the code, modify it, and create your own projects as you move forward. Let me know if you need any further help or explanations!
0 Comments