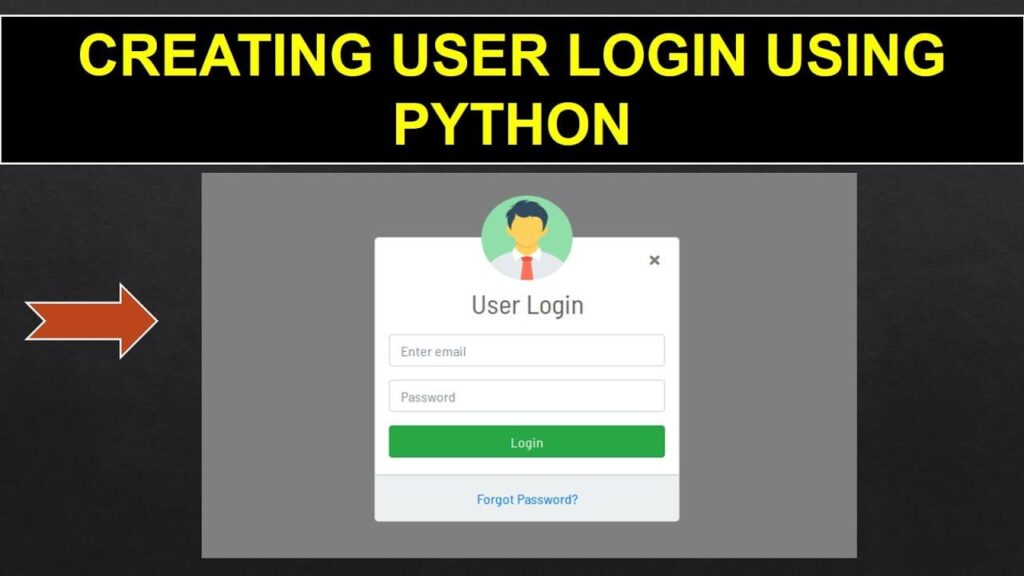
Table of Contents
In this code, the user (student) will be asked to enter his information only once ( for each student). Finally, make the name ‘exit’ to complete this process.
Student login form Python code
First things first, let’s lay the groundwork. Picture a mystical land where students roam freely, their names whispered on the wind. We start our quest by opening the gates to our kingdom – the Python realm – with a magical incantation known as while
loop.
python
students = []
while True:
name = input("Enter student name (or 'exit' to finish): ")
if name.lower() == 'exit':
break
age = input("Enter student age: ")
gender = input("Enter student gender: ")
student = {'name': name, 'age': age, 'gender': gender}
students.append(student)
Here’s the deal: the while True
spell creates an endless loop, echoing the ancient chant, “Enter student name (or ‘exit’ to finish)”.
As brave souls – or students – step forth, their names are etched into our sacred scroll, along with their age and gender.
Student login form
student registration form
But fear not! Should a student utter the sacred word ‘exit’, the spell breaks, and our journey comes to a temporary halt.
Students details code
Now that our scroll brims with names, let’s unveil its secrets! Behold, the Student Details:
python
print("\nStudent Details:")
for student in students:
print("Name:", student['name'])
print("Age:", student['age'])
print("Gender:", student['gender'])
With a flick of our wand – ahem, code – we traverse the realms of our list of students, revealing their identities one by one. Each name, age, and gender shimmers in the candlelight, a testament to our coding prowess.
Code Explanation
But wait, what makes this incantation so special? Let’s break it down:
- The Infinite Loop: Our
while True
spell keeps the gates open indefinitely, welcoming students into our realm until the end of time – or until someone whispers ‘exit’. - The Gathering: As students step forward, their names, ages, and genders are woven into a tapestry of data, forming our sacred scroll of students.
- The Revelation: With a simple
print
spell, we unveil the secrets of our scroll, displaying each student’s details for all to see.
What is the use case of while loop?
Alright, so picture this: you need your computer to do stuff over and over again, but you ain’t sure exactly how many times. That’s where while loops come in, my friend! They’re like the ultimate repeat button for your code.
Here’s the gist: a while loop keeps on pumpin’ out a block of code as long as a certain condition is true. Think of it like this – you keep doin’ somethin’ until somethin’ else happens. Once that somethin’ else flips the switch to “false,” the loop says “aight, I’m outta here!” ✋
students registration form
registration form for students
registration form student
Now, how about some real-life examples to spice things up?
- User Input: Ever gotta beg a user to enter the right info? A while loop can be your knight in shining armor. It keeps askin’ for input until they finally give you somethin’ that works. No more frustration for you!
- Search Party: Imagine you’re lookin’ for a hidden treasure in a giant pile of stuff (like a computer searchin’ data). You don’t know how deep you gotta dig, right? A while loop can help you sift through everything until you unearth that gold!
- Game On!: Video games? Totally use while loops! They keep the game world runnin’ smooth as butter until you, the mighty player, conquer it all (or, you know, accidentally fall off a cliff – happens to the best of us).
So, the next time you need your code to, well, keep on keepin’ on, remember while loops! They’re the dynamic duo of repetition, ready to tackle anything you throw their way.
How does a while () loop work?
A diagram representing the While Loop logic:
Alright listen up! We’re about to dissect this whole “While Loop” thing with a sweet diagram to help you see how it works. Imagine it like a secret mission, but with a flowchart instead of spy gadgets.
Here’s the deal:
- Mission Start: The program bumps into the while loop, which is basically the starting point of our secret operation.
- Intel Check: The loop gotta check a special condition, like a secret code phrase. Is it a “go” (True) or a “nah, hold up” (False)? This condition is like the key to the mission.
- Action Time!: If the code checks out (True!), the loop swings into action, doing whatever it’s programmed to do (those are the statements inside the loop). Think of it as executing the mission tasks.
- Repeat or Escape?: But here’s the twist: this loop ain’t a one-shot deal. It goes back to step 2 and checks the code phrase again. Like a good agent, it keeps confirming the mission status.
- Mission Accomplished!: This loop keeps repeating steps 2 and 3 as long as the code phrase stays true. But once it turns false, the loop knows it’s done and lets the program move on to the next part (like escaping the enemy base after completing the mission).
Here’s the Thing, Though: We gotta make sure this code phrase eventually flips to false. Otherwise, we’re stuck in an infinite loop, which is basically a never-ending mission (no bueno!).
That's why we gotta write our code carefully to update the variables used in the condition, like changing the code phrase after each successful mission.
So, peep the diagram! It’s like a blueprint for the whole operation:
+-------------------+
| | |
| Program Flow |
| v |
+---------+ +---------+ +---------+
| Start | -------> | While | -------> | Loop |
+---------+ | Condition| -------> | Statements |
| (True/False)| +---------+
+---------+ | |
v |
+---------+ |
| Exit | +---------+
| (False) |
+---------+
See how the program flows through the loop, checking the condition and executing the statements until it finally escapes through the exit door? Pretty slick, right? Now you can crack any while loop code like a decoding pro! ️♂️
What is the syntax for while loop?
Alright, so you’ve mastered the while loop mission in theory. Now it’s time to translate that knowledge into some real Python code. Buckle up, because we’re about to break down the while loop syntax like a boss.
Think of it like building a blueprint:
- While (the Keyword Captain): This keyword is like the leader of the loop crew, signaling the start of the operation.
- Condition Check (The Truth Detector): This is the mission’s secret code phrase, written as a condition that evaluates to True or False. It determines if the loop keeps running.
- Colon (The Gateway): This colon acts as a gateway, separating the condition from the loop’s tasks.
- Body of the Loop (The Action Zone): This indented block of code is the heart of the mission. It contains the statements that get executed repeatedly as long as the condition stays true. Imagine it as the specific actions the loop carries out.
Visualize it with this Diagram:
+--------------------+ +--------------------+
| | | | | |
| While | (Space) | | Condition | (True/False) |
| | | | | |
+---------+---------+ +---------+---------+
| | (Colon)
v v
+---------+---------+ +---------+---------+
| | | | | |
| Body of | | | Statements |
| the Loop| (Indented) | | | |
| | | +---------+---------+
+---------+---------+
PEP 8 Tip: Remember, proper indentation is key in Python! Use 4 spaces for each indentation level to keep your code clean and readable. Tabs are a no-no unless the existing code already uses them for consistency.
With this blueprint in mind, you can write some powerful while loops in Python to tackle repetitive tasks with ease!
While Loop in Action: Examples of While Loop with Visuals
Now that you’ve got the while loop basics down, let’s see them come to life with some real-world Python code examples! We’ll break down each example step-by-step and even throw in some snazzy diagrams to visualize the loop’s journey.
Example 1: Printing Numbers Up to 7
This code snippet prints the numbers 4, 5, 6, and 7:
i = 4
while i < 8:
print(i)
i += 1
Behind the Scenes (Diagram):
+---------+ +---------+ +---------+ +---------+
| Start | -------> | While | -------> | i < 8 | | Print(i) |
+---------+ | (i=4) | -------> | (True) | +---------+
+---------+ +---------+ | i += 1 |
| (i becomes 5) |
+---------+
Explanation:
- Initialization: We set
i
to 4. - Condition Check: The loop checks if
i
is less than 8 (True). - Action: The loop prints the current value of
i
(4). - Update: The value of
i
is incremented by 1 (i
becomes 5). - Repeat: The loop jumps back to step 2 and repeats the process.
Iterations 2, 3, and 4: This cycle continues until i
reaches 8. At that point, the condition i < 8
becomes False, and the loop stops.
new student registration form
students registration form template
template for student registration form
Example 2: Guessing Game
Here’s a simple guessing game where the user has to guess a secret number:
secret_number = 7
guess_count = 0
while guess_count < 3:
guess = int(input("Guess a number (1-10): "))
guess_count += 1
if guess == secret_number:
print("You guessed it!")
break # Exit the loop if the guess is correct
else:
print("Sorry, you ran out of guesses. The number was", secret_number)
Imagine the Loop (Flowchart):
- It starts with a box labeled “Start”. The next box is labeled “guesscount < 3”.
- If yes, an arrow leads to a box labeled “Get guess from user”.
- If no, an arrow leads to a box labeled “Out of guesses”. The “Get guess from user” box leads to a decision diamond labeled “Guess == secretnumber”.
- If yes, an arrow leads to a box labeled “Print ‘You guessed it!'”.
- If no, an arrow leads to a box labeled “guesscount += 1”. The “guesscount += 1” box leads back to the decision diamond “guesscount < 3”.]
Explanation:
- Setup: We define the secret number and initialize the guess count.
- Loop Start: The loop checks if the guess count is less than 3 (True).
- Get Guess: The user is prompted to enter a guess.
- Check Guess: The loop checks if the guess matches the secret number.
- If yes, the user wins, and the loop exits with a “break” statement.
- If no, the guess count is incremented.
- Repeat: The loop jumps back to step 2 and continues until the guess count reaches 3 or the user guesses correctly.
- Out of Guesses: If the loop finishes without a correct guess, the user loses.
Example 3: Coin Toss Simulator
This code simulates flipping a coin 5 times and displaying the results (Heads or Tails):
import random
flips = 5
while flips > 0:
coin_side = random.choice(["Heads", "Tails"])
print(coin_side)
flips -= 1
Loop Visualization (Diagram):
“`
+———+ +———+ +———+ +———+ +———+
| Start | ——-> | While | ——-> | flips > 0 | | Coin Toss| | Print(side)|
+———+ | (flips=5)| ——-> | (True) | +———+ +
What are Infinite While Loops?
Alright, so we’ve been rocking these while loops, but there’s a catch: they don’t magically update variables on their own. We gotta be the code whisperers and tell them how to change things up. Here’s the deal:
Infinite Loop Trap in Short!
If we forget to update the variable used in the condition, we’re setting ourselves up for an infinite loop. Imagine a never-ending loop, like a hamster on a wheel that just keeps going and going (not ideal for our programs).
Example: The Unending “Hello, World!”
Let’s see some code that might cause this infinite loop situation:
# Define a variable
i = 5
# Run this loop while i is less than 15
while i < 15:
# Print a message
print("Hello, World!")
Hold on a sec…
What’s missing here? Can you spot the culprit? Yep, the value of i
never changes inside the loop! So, the condition i < 15
will always be True, and our loop gets stuck in a never-ending cycle of printing “Hello, World!”.
How to Escape the Loop-a-Palooza?
- Manual Intervention (CTRL + C): In most cases, you gotta hit CTRL + C on your keyboard to forcefully stop the program. Not exactly elegant, right?
- The Update is Key: The key to avoiding this infinite loop nightmare is to make sure we update the variable used in the condition inside the loop. This way, the condition will eventually become False, and our loop will know it’s time to say goodbye.
student registration form template
student registration form templates
printable student registration form
Intentional Infinity?
Now, there are some rare cases where we might actually want an infinite loop. But these are usually intentional, like creating a never-ending game loop until the player breaks free (by winning or, well, quitting!).
Remember: While loops are powerful tools, but always keep an eye on those variable updates. You don’t want your program stuck in a loop-a-loop for eternity!
How to make three attempts in Python?
Here’s how you can make three attempts in Python using a while loop:
attempts = 3
while attempts > 0:
# Your code to get user input or perform an action here
# For example, get a password guess
guess = input("Enter your guess: ")
# Check if the guess is correct (modify this condition for your specific use case)
if guess == "secret_password":
print("You guessed it!")
break # Exit the loop if the guess is correct
attempts -= 1 # Decrement attempts remaining
if attempts > 0:
print("Incorrect. You have", attempts, "attempts remaining.")
else:
print("Sorry, you ran out of attempts.")
Explanation:
attempts
Variable: We define a variableattempts
set to 3, representing the number of tries the user gets.while
Loop: Thewhile
loop keeps running as long asattempts
is greater than 0.- User Input: Inside the loop, you can replace the placeholder comment with your code to get user input (like a guess or any action you want them to perform).
- Guess Check: The code checks if the user’s input (e.g.,
guess
) matches the desired outcome (e.g.,"secret_password"
).- Correct Guess: If the guess is correct, it prints a success message and uses
break
to exit the loop. - Incorrect Guess: If the guess is wrong, it decrements the
attempts
variable by 1.
- Correct Guess: If the guess is correct, it prints a success message and uses
- Remaining Attempts: If attempts are remaining, it displays a message informing the user how many attempts they have left.
- Out of Attempts: If attempts reach 0, it displays a message indicating the user has run out of tries.
Key Points:
- This code demonstrates a general structure for allowing three attempts. Modify the user input and guess check logic to fit your specific needs.
- The
break
statement is crucial to exit the loop prematurely if the user guesses correctly, preventing unnecessary iterations.
How to do a password while loop in Python?
Here’s how to create a password while loop in Python:
secret_password = "your_secret_password" # Replace with your actual password
attempts = 3
while attempts > 0:
user_guess = input("Enter your password: ")
if user_guess == secret_password:
print("You guessed it! Welcome in.")
break # Exit the loop if the guess is correct
else:
attempts -= 1
if attempts > 0:
print("Incorrect password. You have", attempts, "attempts remaining.")
else:
print("Sorry, you ran out of attempts.")
student registration form pdf
Explanation:
- Secret Password: Define the
secret_password
variable with your actual password (replace"your_secret_password"
). - Attempts: Set the
attempts
variable to 3, representing the number of tries allowed. while
Loop: The loop keeps running as long asattempts
is greater than 0.- User Input: Inside the loop,
input("Enter your password: ")
prompts the user to enter their guess and stores it in theuser_guess
variable. - Guess Check: The
if
statement checks if theuser_guess
matches thesecret_password
.- Correct Guess: If they match, it prints a success message and uses
break
to exit the loop. - Incorrect Guess: If they don’t match, it decrements
attempts
by 1.
- Correct Guess: If they match, it prints a success message and uses
- Remaining Attempts: If attempts are remaining (
attempts > 0
), it displays a message informing the user of remaining tries. - Out of Attempts: If attempts reach 0 (
else
statement), it displays a message indicating the user is out of tries.
Key Points:
- Replace
"your_secret_password"
with your actual password for security reasons. - This code provides a basic structure for password attempts. You can enhance it further by:
- Hiding the user’s password input using
getpass.getpass()
. - Implementing a delay between attempts for security.
- Hiding the user’s password input using
What do you understand by the term iteration in Python?
In Python, iteration refers to the process of executing a block of code repeatedly until a certain condition is met. It’s a fundamental concept for controlling the flow of your program and automating repetitive tasks.
Here’s a breakdown of iteration in Python:
1. Loops:
The primary mechanism for iteration in Python is through loops. These control structures allow you to execute a sequence of statements multiple times. Common loop types include:
while
loop: This loop continues executing its code block as long as a specified condition remains True. It’s useful when you don’t know beforehand how many times you need to repeat the code.for
loop: This loop iterates over a sequence of items (like a list, tuple, or string) and executes the code block for each item in the sequence. It’s ideal when you know the exact collection of elements you need to process.
2. Iterables and Iterators:
- Iterables: These are objects that can be used in a
for
loop. They provide a way to access elements one at a time. Examples include lists, tuples, strings, dictionaries (for keys), and sets. - Iterators: These are special objects that implement the iterator protocol, allowing you to efficiently step through the elements of an iterable object. The
iter()
function is used to create an iterator from an iterable.
Example:
# While loop iterating until i reaches 5
i = 0
while i < 5:
print(i)
i += 1
# For loop iterating over a list
numbers = [1, 2, 3, 4]
for number in numbers:
print(number)
In essence, iteration allows you to write concise and efficient code to handle repetitive tasks. By using loops and iterables effectively, you can make your Python programs more readable and maintainable.
How do you run a program until key press in Python?
There are two main approaches to running a program until a key press in Python:
1. Using the input()
function (for Python 3):
This method is simple but has limitations. The input()
function pauses the program and waits for the user to press a key and optionally enter some text. However, it only captures the entire line of entered text, not just a single key press.
Here’s an example:
print("Press any key to continue...")
input()
print("Program continues!")
This code will print the message, wait for the user to press any key and potentially enter text, and then continue execution.
Limitations:
- Captures the entire line of entered text, not just the key press.
- Not ideal for complex scenarios where you need to distinguish between specific keys.
2. Using external libraries (for more control):
For more advanced scenarios where you need to detect specific key presses, you can use external libraries like msvcrt
(Windows) or getch
(cross-platform). These libraries offer functions to capture single key presses without requiring the user to press Enter.
Example using msvcrt
(Windows):
import msvcrt
print("Press any key to continue...")
key = msvcrt.getch().decode() # Decode the byte value to a character
print("You pressed:", key)
This code imports msvcrt
, waits for a key press, retrieves the pressed key as a byte value, decodes it to a character, and then prints it.
Example using getch
(cross-platform):
from getch import getch
print("Press any key to continue...")
key = getch() # Get the character directly
print("You pressed:", key)
This code uses the getch
library (installation required) to get the pressed character directly, offering a more cross-platform solution.
Choosing the Right Method:
- If you simply need to pause the program until the user interacts (presses any key and potentially enters text), the
input()
function is sufficient. - If you need to capture specific key presses and distinguish between them, using libraries like
msvcrt
orgetch
provides more control.
A Glimpse into the Future
As we bid adieu to our journey, remember this: the magic of Python knows no bounds. With each line of code, we unlock new possibilities, shaping the world around us with the power of imagination.
So, fellow wizards, go forth and create! Let your code be the beacon that guides others through the darkness, illuminating the path to knowledge and discovery.
Until next time, may your loops be infinite and your spells be bug-free. Happy coding, my friends!
✨🐍✨
Related topics to this article
- student registration
- student registration form
- students registration form
- registration form for students
- register at university
- register for university
- register in university
- register to university
- registering at university
- student registration card
- student registration form html template
- Sure, here are the translations:
- formulario de registro de estudiantes
- formulario de registro de estudiantes
- formulario de registro para estudiantes
- formulario de registro estudiantil
- nuevo formulario de registro de estudiantes
- plantilla de formulario de registro de estudiantes
- plantilla para formulario de registro de estudiantes
- plantilla de formulario de registro de estudiantes
- plantillas de formulario de registro de estudiantes
- formulario de registro de estudiantes imprimible
- formulario de registro de estudiantes en formato PDF
1 Comment
Utwórz konto na Binance · April 12, 2024 at 10:28 pm
Your article helped me a lot, is there any more related content? Thanks!