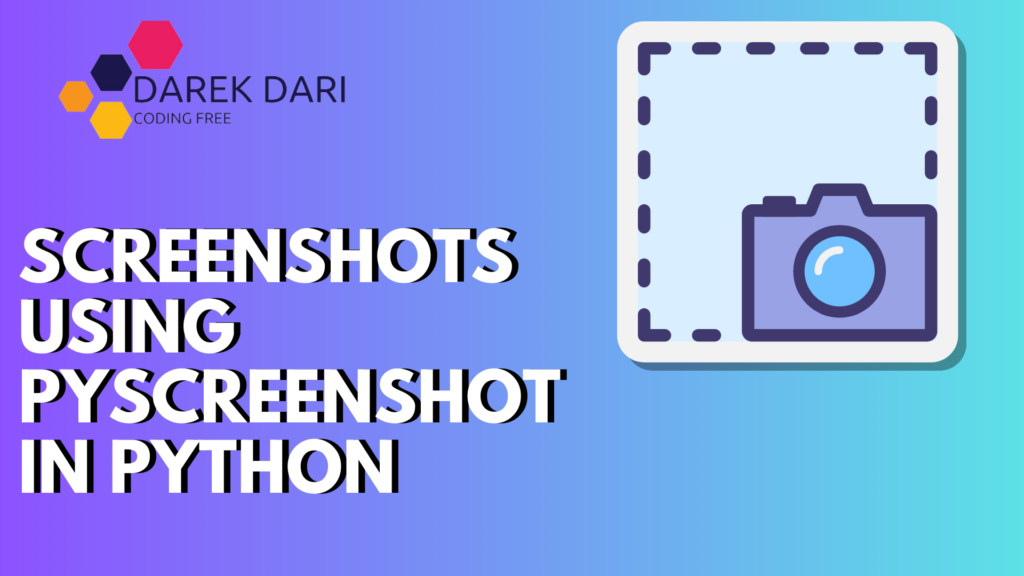
Table of Contents
Screenshots are incredibly useful for capturing the state of a program, documenting bugs, or simply saving visual information for later use.
If you’re a Python developer looking to automate this process, pyscreenshot
is a fantastic library to consider. Let’s dive into how you can use pyscreenshot
to take screenshots in Python, step by step.
What is pyscreenshot
?
pyscreenshot
is a Python library that allows you to capture screenshots in a simple and efficient way. It works across multiple platforms, making it a versatile tool for any project that requires screenshot functionality.
With pyscreenshot
, you can capture the entire screen, a specific region, or even individual windows.
Let’s Start Coding
Installing pyscreenshot
Before you can start taking screenshots, you’ll need to install the pyscreenshot
library. You can do this using pip
:
pip install pyscreenshot
Capturing the Entire Screen
The most basic use of pyscreenshot
is to capture the entire screen. This is useful for capturing the overall state of your desktop or documenting entire processes.
import pyscreenshot as ImageGrab
def capture_entire_screen():
screenshot = ImageGrab.grab()
screenshot.save("entire_screen.png")
capture_entire_screen()
In this example, ImageGrab.grab()
captures the entire screen, and the save
method saves the screenshot to a file named entire_screen.png
.
Capturing a Specific Region
Sometimes, you only need to capture a specific part of the screen. pyscreenshot
allows you to define the coordinates of the region you want to capture.
import pyscreenshot as ImageGrab
def capture_region():
# Define the region to capture: (left, top, right, bottom)
region = (100, 100, 500, 500)
screenshot = ImageGrab.grab(bbox=region)
screenshot.save("region_screen.png")
capture_region()
In this case, bbox
specifies the bounding box of the region to capture, and the screenshot is saved as region_screen.png
.
Capturing an Individual Window
You can also capture screenshots of individual windows. This can be particularly useful for capturing the state of a specific application without including other on-screen elements.
import pyscreenshot as ImageGrab
def capture_window(window_title):
from PIL import ImageChops
import pygetwindow as gw
windows = gw.getWindowsWithTitle(window_title)
if windows:
window = windows[0]
bbox = (window.left, window.top, window.right, window.bottom)
screenshot = ImageGrab.grab(bbox=bbox)
screenshot.save(f"{window_title}.png")
capture_window("Untitled - Notepad")
In this script, pygetwindow
is used to get the coordinates of a window with a specific title. ImageGrab.grab(bbox=bbox)
captures the window, and the screenshot is saved with the window’s title as the file name.
Adding Timestamp to Screenshot
To make your screenshots more informative, you can add a timestamp to the file name. This can help you keep track of when each screenshot was taken.
import pyscreenshot as ImageGrab
from datetime import datetime
def capture_with_timestamp():
timestamp = datetime.now().strftime("%Y-%m-%d_%H-%M-%S")
screenshot = ImageGrab.grab()
screenshot.save(f"screenshot_{timestamp}.png")
capture_with_timestamp()
The timestamp is formatted using strftime
, and it’s included in the file name to ensure each screenshot has a unique identifier.
How to take a screenshot using pyautogui?
Taking screenshots programmatically can be incredibly useful for a variety of applications, from automation scripts to creating documentation. pyautogui
is a powerful library in Python that makes this task simple and efficient. Let’s explore how you can use pyautogui
to capture screenshots, step by step.
pyautogui
is a cross-platform GUI automation library for Python. It allows you to control the mouse and keyboard, as well as capture screenshots of your screen. It’s particularly useful for automating tasks that require visual data.
Installing pyautogui
Before you can start taking screenshots, you’ll need to install the pyautogui
library. You can do this using pip
:
pip install pyautogui
Capturing the Entire Screen
The most straightforward use of pyautogui
for taking screenshots is capturing the entire screen. Here’s how you can do it:
import pyautogui
def capture_entire_screen():
screenshot = pyautogui.screenshot()
screenshot.save("entire_screen.png")
capture_entire_screen()
In this example, pyautogui.screenshot()
captures the entire screen, and the save
method saves the screenshot to a file named entire_screen.png
.
Capturing a Specific Region
Sometimes, you might only need to capture a specific part of the screen. pyautogui
allows you to define the coordinates of the region you want to capture:
import pyautogui
def capture_region():
# Define the region to capture: (left, top, width, height)
region = (100, 100, 400, 400)
screenshot = pyautogui.screenshot(region=region)
screenshot.save("region_screen.png")
capture_region()
In this case, the region
parameter specifies the bounding box of the region to capture, and the screenshot is saved as region_screen.png
.
Adding Timestamp to Screenshot
To make your screenshots more informative, you can add a timestamp to the file name. This can help you keep track of when each screenshot was taken:
import pyautogui
from datetime import datetime
def capture_with_timestamp():
timestamp = datetime.now().strftime("%Y-%m-%d_%H-%M-%S")
screenshot = pyautogui.screenshot()
screenshot.save(f"screenshot_{timestamp}.png")
capture_with_timestamp()
The timestamp is formatted using strftime
, and it’s included in the file name to ensure each screenshot has a unique identifier.
Example Code
Here’s a complete example that combines the above functionalities into a single script:
import pyautogui
from datetime import datetime
def capture_entire_screen():
screenshot = pyautogui.screenshot()
screenshot.save("entire_screen.png")
def capture_region():
region = (100, 100, 400, 400)
screenshot = pyautogui.screenshot(region=region)
screenshot.save("region_screen.png")
def capture_with_timestamp():
timestamp = datetime.now().strftime("%Y-%m-%d_%H-%M-%S")
screenshot = pyautogui.screenshot()
screenshot.save(f"screenshot_{timestamp}.png")
if __name__ == "__main__":
capture_entire_screen()
capture_region()
capture_with_timestamp()
Taking screenshots in Python using pyautogui
is a straightforward and powerful way to capture visual data.
Whether you need to capture the entire screen, a specific region, or add timestamps to your screenshots, pyautogui
provides the tools to do so efficiently.
By incorporating these techniques into your Python projects, you can automate the process of taking screenshots, making your work more efficient and organized. Happy coding!
Conclusion
Taking screenshots in Python is made easy and efficient with the pyscreenshot
library. Whether you need to capture the entire screen, a specific region, or an individual window, pyscreenshot
provides the functionality you need. By automating this process, you can save time and ensure consistency in your screenshots.
By following the steps outlined in this blog, you can integrate screenshot functionality into your Python projects and make your documentation, bug reporting, and visual data capture more effective and automated. Happy screenshotting!
1 Comment
100 Best Python Projects With Source Code: Beginner To Pro · August 23, 2024 at 11:07 pm
[…] 🔹 Screenshots with PyScreeze […]