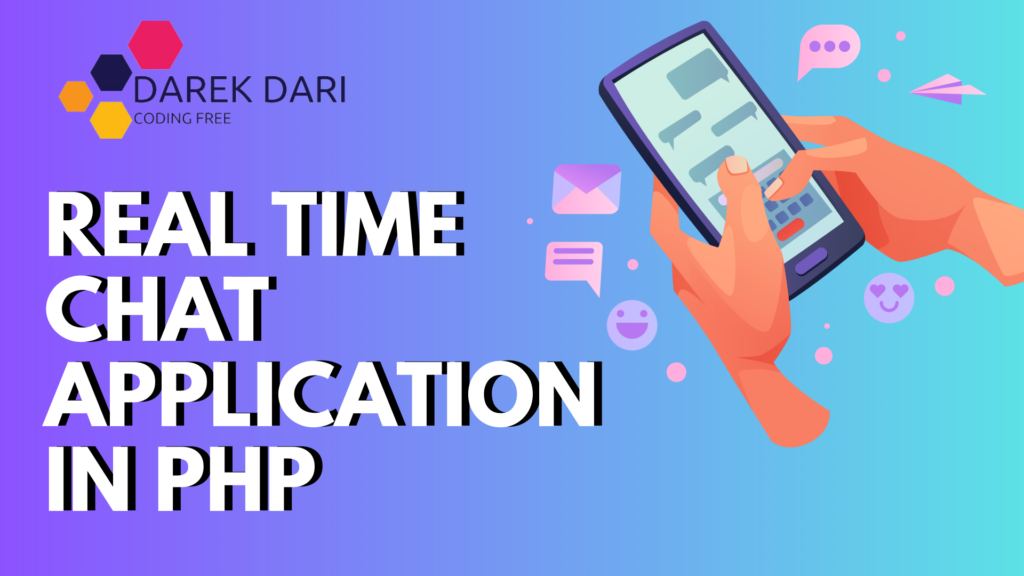
Table of Contents
Introduction to the Best Real Time Chat Application in PHP
Creating a real time chat application can be a fun and rewarding project. We’ll dive into setting up user authentication, enabling private messaging, and creating chat rooms.
PHP Projects
- Quiz Application in PHP
- PHP Image Gallery Project Source Code
- Best 200 Questions Game in PHP
- Bulletin Board PHP Project
- Content Management System (CMS) PhP Project
- Event Management System Project in PHP
- Personal Blog with PHP
- Chatbot in PHP
- URL Shortenee in php
- Creating Best PHP MVC Framework from Scratch
- Recipe Sharing Platform with PHP
- Job Board with PHP
By the end of this guide, you’ll have a fully functional chat app to share with your friends or colleagues!
Let’s embark on this exciting journey to build a chat application that ticks all the boxes: real-time communication, user authentication, private messaging, and chat rooms. We’ll be using PHP for the backend, MySQL for the database, and WebSockets for real-time magic. Get ready to create something awesome! 🚀
photo gallery php
php exercises
php exercises and solutions
php exercises and solutions pdf
php exercises online
php lab exercises
php mvc framework from scratch
php mvc from scratch
What is a Real-Time Chat Application? 🗨️
A real-time chat application allows users to send and receive messages instantly, creating a seamless communication experience. Unlike traditional messaging systems, real-time chat apps update conversations as soon as a message is sent.
Key Features 🚀
Feature | Description |
---|---|
Instant Messaging | Messages are delivered instantly. |
User Authentication | Secure login credentials. |
Private Messaging | Direct messages between users. |
Chat Rooms | Public or private rooms for group conversations. |
Notifications | Alerts for new messages and mentions. |
File Sharing | Share files, images, and media. |
Emojis and Reactions | Adds fun and expressiveness. |
Read Receipts | Shows when messages have been seen. |
How It Works ⚙️
- User Authentication: Secure login.
- Establishing Connection: Persistent connection using WebSockets.
- Sending Messages: User sends a message to the server.
- Broadcasting Messages: Server broadcasts the message to recipients.
- Receiving Messages: Recipient receives the message in real-time.
Example Technologies 🛠️
Technology | Purpose |
---|---|
WebSockets | Real-time communication. |
Node.js | Fast, scalable server-side applications. |
Socket.io | Simplifies WebSocket implementation. |
PHP | Backend logic. |
MySQL or MongoDB | Databases for storing data. |
React.js or Vue.js | Front-end frameworks. |
Benefits 🌟
Benefit | Description |
---|---|
Immediate Communication | Instant interaction. |
Enhanced Collaboration | Quick decision-making and information sharing. |
User Engagement | Keeps users engaged with notifications, emojis, and reactions. |
Efficient Support | Faster customer support for businesses. |
Real-time chat applications make it possible to stay connected instantly, enhancing personal communication and business operations. Happy chatting! 🗨️✨
Setting Up the Environment
Before we jump into the coding fun, let’s make sure our development environment is all set. You’ll need PHP, MySQL, and a WebSocket server like Ratchet for PHP. Tools like XAMPP or MAMP can simplify this setup process.
User Authentication
User authentication is essential for a secure chat application. It ensures only registered users can log in and access the chat features. Here’s how to set it up:
Database Setup
First, create a database and a table for users. Here’s a sample SQL script to get you started:
CREATE DATABASE chat_app;
USE chat_app;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
php exercises
php lab exercises
php mvc folder structure best practices
php mvc framework from scratch
php mvc from scratch
php mvc project
php photo database
php photo gallery
php practice exercises
php project source code
php projects source code
php projects with source code github
php realtime chat
Registration
Create a registration form where users can sign up by providing a username and password. Use PHP to handle form submissions and store the hashed password in the database.
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$username = $_POST['username'];
$password = password_hash($_POST['password'], PASSWORD_BCRYPT);
$stmt = $pdo->prepare('INSERT INTO users (username, password) VALUES (?, ?)');
$stmt->execute([$username, $password]);
echo 'User registered successfully!';
}
?>
<form method="POST">
<input type="text" name="username" required>
<input type="password" name="password" required>
<button type="submit">Register</button>
</form>
Login
Create a login form to authenticate users. Verify the password using password_verify()
.
<?php
session_start();
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$username = $_POST['username'];
$password = $_POST['password'];
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = ?');
$stmt->execute([$username]);
$user = $stmt->fetch();
if ($user && password_verify($password, $user['password'])) {
$_SESSION['user_id'] = $user['id'];
echo 'Login successful!';
} else {
echo 'Invalid credentials!';
}
}
?>
<form method="POST">
<input type="text" name="username" required>
<input type="password" name="password" required>
<button type="submit">Login</button>
</form>
php mvc project source code
php photo database
php photo gallery
php project source code
php projects source code
php projects with source code
php real time application
php real time chat application
php realtime chat
Real-Time Chat Functionality
Let’s bring the chat to life using WebSockets!
Setting Up Ratchet
Install Ratchet using Composer:
composer require cboden/ratchet
Create a WebSocket server script:
<?php
use Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
require dirname(__DIR__) . '/vendor/autoload.php';
class Chat implements MessageComponentInterface {
protected $clients;
public function __construct() {
$this->clients = new \SplObjectStorage;
}
public function onOpen(ConnectionInterface $conn) {
$this->clients->attach($conn);
echo "New connection! ({$conn->resourceId})\n";
}
public function onMessage(ConnectionInterface $from, $msg) {
foreach ($this->clients as $client) {
if ($from !== $client) {
$client->send($msg);
}
}
}
public function onClose(ConnectionInterface $conn) {
$this->clients->detach($conn);
echo "Connection {$conn->resourceId} has disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "An error has occurred: {$e->getMessage()}\n";
$conn->close();
}
}
$server = IoServer::factory(
new HttpServer(
new WsServer(
new Chat()
)
),
8080
);
$server->run();
Client-Side Script
Create a simple HTML page with JavaScript to connect to the WebSocket server.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Chat App</title>
</head>
<body>
<div id="chat"></div>
<input type="text" id="message" placeholder="Type your message here...">
<button onclick="sendMessage()">Send</button>
<script>
const conn = new WebSocket('ws://localhost:8080');
conn.onmessage = function(e) {
document.getElementById('chat').innerHTML += e.data + '<br>';
};
function sendMessage() {
const msg = document.getElementById('message').value;
conn.send(msg);
}
</script>
</body>
</html>
Private Messaging
Let’s add private messaging to our app!
Database Setup
Add a table for messages:
CREATE TABLE messages (
id INT AUTO_INCREMENT PRIMARY KEY,
sender_id INT,
receiver_id INT,
message TEXT,
sent_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (sender_id) REFERENCES users(id),
FOREIGN KEY (receiver_id) REFERENCES users(id)
);
Sending Private Messages
Modify the WebSocket server to handle private messages.
public function onMessage(ConnectionInterface $from, $msg) {
$data = json_decode($msg, true);
if (isset($data['to'])) {
foreach ($this->clients as $client) {
if ($client->resourceId == $data['to']) {
$client->send($data['message']);
break;
}
}
} else {
foreach ($this->clients as $client) {
if ($from !== $client) {
$client->send($msg);
}
}
}
}
Chat Rooms
Create lively chat rooms for users to join and interact.
Database Setup
Add tables for chat rooms and room memberships:
CREATE TABLE chat_rooms (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50) NOT NULL
);
CREATE TABLE room_memberships (
user_id INT,
room_id INT,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (room_id) REFERENCES chat_rooms(id),
PRIMARY KEY (user_id, room_id)
);
WebSocket Server
Modify the server to handle messages for specific rooms.
protected $rooms = [];
public function onMessage(ConnectionInterface $from, $msg) {
$data = json_decode($msg, true);
if (isset($data['room'])) {
$room = $data['room'];
if (!isset($this->rooms[$room])) {
$this->rooms[$room] = new \SplObjectStorage;
}
$this->rooms[$room]->attach($from);
foreach ($this->rooms[$room] as $client) {
if ($from !== $client) {
$client->send($data['message']);
}
}
} else {
foreach ($this->clients as $client) {
if ($from !== $client) {
$client->send($msg);
}
}
}
}
What is a WebSocket Server?
WebSocket is a protocol that provides full-duplex communication channels over a single TCP connection, enabling real-time interaction between a client and a server. A WebSocket server is a server that uses this protocol to communicate with clients.
Here’s a friendly and fun dive into what a WebSocket server is all about!
A WebSocket server allows for bi-directional communication between the server and multiple clients. Unlike traditional HTTP requests, where the client initiates a request and the server responds, WebSockets enable both the server and the client to send and receive messages independently at any time. This makes WebSockets perfect for real-time applications like chat apps, live notifications, and gaming.
Feature | Description |
---|---|
Full-Duplex Communication | Both client and server can send and receive messages simultaneously. |
Persistent Connection | The connection stays open, reducing the overhead of establishing new connections. |
Low Latency | Ideal for real-time applications due to minimal delay in communication. |
Efficient | Uses a single TCP connection, which reduces the bandwidth and resource consumption. |
How Does a WebSocket Server Work?
- Handshake: The connection starts with an HTTP handshake, where the client sends a request to the server to upgrade the connection to WebSocket.
- Establishing Connection: Once the handshake is successful, the server and client establish a persistent WebSocket connection.
- Communication: Both the server and client can now send messages to each other freely without the need for re-establishing the connection.
- Closing Connection: Either the client or the server can close the connection when it’s no longer needed.
Here’s a simple example of how a WebSocket server is set up using Ratchet in PHP:
<?php
use Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
require dirname(__DIR__) . '/vendor/autoload.php';
class ChatServer implements MessageComponentInterface {
protected $clients;
public function __construct() {
$this->clients = new \SplObjectStorage;
}
public function onOpen(ConnectionInterface $conn) {
$this->clients->attach($conn);
echo "New connection! ({$conn->resourceId})\n";
}
public function onMessage(ConnectionInterface $from, $msg) {
foreach ($this->clients as $client) {
if ($from !== $client) {
$client->send($msg);
}
}
}
public function onClose(ConnectionInterface $conn) {
$this->clients->detach($conn);
echo "Connection {$conn->resourceId} has disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "An error has occurred: {$e->getMessage()}\n";
$conn->close();
}
}
$server = \Ratchet\Server\IoServer::factory(
new \Ratchet\Http\HttpServer(
new \Ratchet\WebSocket\WsServer(
new ChatServer()
)
),
8080
);
$server->run();
Why Use a WebSocket Server?
WebSocket servers are incredibly powerful for applications that require real-time communication. Here are some common use cases:
Use Case | Description |
---|---|
Chat Applications | Real-time messaging between users. |
Live Notifications | Instant updates for notifications, such as new messages or alerts. |
Online Gaming | Real-time interaction and communication between players. |
Collaboration Tools | Real-time editing and updates in collaborative applications. |
What is XAMPP? 🤔
XAMPP is an open-source software package that stands for Cross-Platform (X), Apache (A), MariaDB (M), PHP (P), and Perl (P). It provides a convenient and easy-to-use solution for setting up a local web server environment on your computer. Let’s dive into what makes XAMPP so awesome!
Why Use XAMPP? 🌟
XAMPP simplifies the process of setting up a development environment by bundling everything you need into one package. Here are some key features:
Feature | Description |
---|---|
Cross-Platform | Works on Windows, Linux, and MacOS, making it versatile for different operating systems. |
Apache Server | Includes the Apache web server, which is widely used and reliable. |
MariaDB | Comes with MariaDB, a popular fork of MySQL, used for database management. |
PHP and Perl | Supports PHP and Perl, two widely used programming languages for web development. |
Easy Installation | Provides a straightforward installation process, allowing you to get up and running quickly. |
Control Panel | Includes a user-friendly control panel to manage your server components easily. |
Components of XAMPP
Here’s a breakdown of what’s included in XAMPP and what each component does:
Component | Purpose |
---|---|
Apache | Acts as the web server to serve your web pages. |
MariaDB | Database server to store and manage your data. |
PHP | Scripting language for dynamic web pages and server-side scripting. |
Perl | Another scripting language, often used for text processing and CGI scripting. |
phpMyAdmin | Web-based tool to manage MySQL or MariaDB databases easily. |
FileZilla | FTP server to transfer files between your computer and the web server. |
Tomcat | Apache Tomcat server to run Java-based web applications. |
Installing and Using XAMPP 🛠️
- Download: Go to the official XAMPP website and download the appropriate version for your operating system.
- Installation: Run the installer and follow the on-screen instructions. It’s as simple as clicking “Next” a few times!
- Starting XAMPP: Open the XAMPP Control Panel and start the Apache and MariaDB services. Now, your local server is up and running!
- Accessing Your Server: Place your web files in the
htdocs
folder within the XAMPP directory. You can access your site by navigating tohttp://localhost
in your browser.
Practical Uses of XAMPP 🖥️
XAMPP is perfect for:
Use Case | Description |
---|---|
Web Development | Develop and test websites locally before deploying them to a live server. |
Learning PHP/MySQL | Practice and experiment with PHP and MySQL without the need for a remote server. |
Testing CMS | Install and configure content management systems like WordPress, Joomla, or Drupal locally. |
Database Management | Use phpMyAdmin to manage databases conveniently. |
Local Hosting | Host and run web applications locally for development purposes. |
XAMPP is a powerful tool for developers, offering a complete local development environment that is easy to set up and use. Whether you’re a beginner learning to code or an experienced developer testing new projects, XAMPP is a go-to solution for many web development needs.
What is MAMP?
MAMP stands for Macintosh, Apache, MySQL, and PHP/Perl/Python. It’s a free, local server environment that allows you to set up a development environment on your macOS or Windows machine. MAMP is particularly popular among developers who use macOS, as it provides an easy way to create a local server to develop and test web applications. Let’s break it down in a friendly and fun way!
Why Use MAMP? 🌟
MAMP simplifies the process of setting up a development environment by bundling all the necessary components into one package. Here are some key features:
Feature | Description |
---|---|
Cross-Platform | Available for both macOS and Windows, making it versatile for different operating systems. |
Apache Server | Includes the Apache web server, which is widely used and reliable. |
MySQL | Comes with MySQL, one of the most popular database management systems. |
PHP/Perl/Python | Supports multiple scripting languages, allowing flexibility in development. |
Easy Installation | Provides a straightforward installation process, allowing you to get up and running quickly. |
Control Panel | Includes a user-friendly control panel to manage your server components easily. |
Components of MAMP
Here’s a breakdown of what’s included in MAMP and what each component does:
Component | Purpose |
---|---|
Apache | Acts as the web server to serve your web pages. |
MySQL | Database server to store and manage your data. |
PHP | Scripting language for dynamic web pages and server-side scripting. |
Perl | Scripting language, often used for text processing and CGI scripting. |
Python | Another popular scripting language used for web development and other applications. |
phpMyAdmin | Web-based tool to manage MySQL databases easily. |
Installing and Using MAMP 🛠️
- Download: Go to the official MAMP website and download the appropriate version for your operating system.
- Installation: Run the installer and follow the on-screen instructions. It’s a breeze!
- Starting MAMP: Open the MAMP application and start the servers (Apache and MySQL). Your local server is now running!
- Accessing Your Server: Place your web files in the
htdocs
folder within the MAMP directory. You can access your site by navigating tohttp://localhost
in your browser.
Practical Uses of MAMP 🖥️
MAMP is perfect for:
Use Case | Description |
---|---|
Web Development | Develop and test websites locally before deploying them to a live server. |
Learning PHP/MySQL | Practice and experiment with PHP and MySQL without the need for a remote server. |
Testing CMS | Install and configure content management systems like WordPress, Joomla, or Drupal locally. |
Database Management | Use phpMyAdmin to manage databases conveniently. |
Local Hosting | Host and run web applications locally for development purposes. |
MAMP provides a powerful and easy-to-use local server environment for developers, offering all the tools needed to build and test web applications locally. Whether you’re just starting out or you’re an experienced developer, MAMP is a valuable tool for web development on macOS and Windows.
What is MySQL?
MySQL is an open-source relational database management system (RDBMS) that uses Structured Query Language (SQL) to manage and manipulate databases. It’s widely used for web applications and is a core component of the LAMP stack (Linux, Apache, MySQL, PHP/Perl/Python). Let’s dive into the basics of MySQL in a friendly and fun way!
Key Features of MySQL 🌟
MySQL offers a plethora of features that make it a popular choice for developers and businesses alike. Here are some of the standout features:
Feature | Description |
---|---|
Open Source | Free to use and modify, with a strong community and commercial support options available. |
High Performance | Optimized for speed, reliability, and scalability to handle large databases and high-traffic applications. |
Cross-Platform | Compatible with various operating systems including Linux, Windows, and macOS. |
Security | Provides robust security features including user authentication, SSL support, and data encryption. |
Replication | Allows data replication for backup and high availability. |
Scalability | Easily scalable to accommodate growing data needs. |
Stored Procedures | Supports stored procedures, triggers, and views to enhance database functionality. |
How MySQL Works ⚙️
MySQL operates using a client-server model. Here’s a simplified look at the process:
- Client Connection: Users connect to the MySQL server through a client application or command-line interface.
- Query Execution: The client sends SQL queries to the MySQL server to interact with the database.
- Data Processing: The server processes these queries, retrieving or manipulating data as requested.
- Results Return: The server sends the results back to the client.
Here’s an example of a simple SQL query in MySQL:
SELECT * FROM users WHERE age > 30;
This query selects all records from the users
table where the age
column is greater than 30.
Practical Uses of MySQL 🖥️
MySQL is incredibly versatile and can be used in various applications:
Use Case | Description |
---|---|
Web Applications | Powers popular websites and web applications like WordPress, Joomla, and Drupal. |
E-commerce | Used in online stores to manage product information, user data, and transactions. |
Data Warehousing | Utilized for storing and analyzing large volumes of data. |
Content Management | Supports content management systems by handling the backend data operations. |
Social Media | Manages user data, posts, and interactions in social networking platforms. |
Enterprise Solutions | Employed in corporate environments for various business applications, including customer relationship management (CRM) and enterprise resource planning (ERP). |
Benefits of MySQL 🌟
Benefit | Description |
---|---|
Cost-Effective | Being open-source, it reduces licensing costs, making it an economical choice for businesses. |
Community Support | A large, active community provides a wealth of resources, including documentation, forums, and tutorials. |
Reliability | Known for its reliability and uptime, making it suitable for mission-critical applications. |
Ease of Use | User-friendly with a straightforward setup process and easy-to-learn SQL syntax. |
MySQL is a powerful and reliable RDBMS that supports a wide range of applications. Whether you’re developing a small website or a large-scale enterprise application, MySQL provides the tools and features needed to manage and manipulate your data efficiently.
Conclusion
Creating a real-time chat application with user authentication, private messaging, and chat rooms is an exciting project that involves setting up a database, handling user authentication, and using WebSockets for real-time communication.
With these steps, you’ll have a solid foundation for a chat app that you can further expand and customize to fit your needs. Happy coding! 🌟💬🚀
If you need more details or have any questions, feel free to ask! 😊
0 Comments