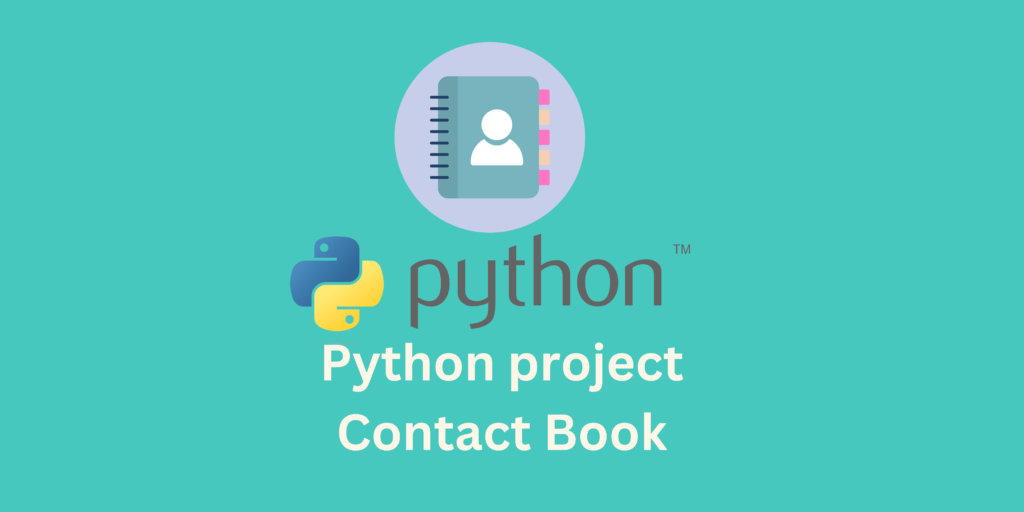
The Contact Book project is a Python project that allows you to store and manage contacts. The program uses a CSV file to store contact information and provides options to add, update, search, and delete contacts.
Steps for python project
Importing Modules
First, we import the required modules – csv
, os
, and sys
.
import csv
import os
import sys
Defining Functions
Next, we define several functions – create_csv
, load_csv
, add_contact
, search_contact
, update_contact
, and delete_contact
.
Here, we define functions to create a new CSV file with a header row, load the CSV file into a list of dictionaries, add a new contact to the CSV file, search for a contact by name, update an existing contact, and delete a contact.
def create_csv():
with open("contacts.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["Name", "Phone", "Email"])
def load_csv():
with open("contacts.csv", newline="") as file:
reader = csv.DictReader(file)
contacts = []
for row in reader:
contacts.append(row)
return contacts
def add_contact(name, phone, email):
with open("contacts.csv", "a", newline="") as file:
writer = csv.writer(file)
writer.writerow([name, phone, email])
def search_contact(contacts, name):
for contact in contacts:
if contact["Name"] == name:
return contact
return None
def update_contact(contacts, name, phone, email):
for contact in contacts:
if contact["Name"] == name:
contact["Phone"] = phone
contact["Email"] = email
return True
return False
def delete_contact(contacts, name):
for i, contact in enumerate(contacts):
if contact["Name"] == name:
del contacts[i]
return True
return False
Managing Contacts
In the main function of the program, we first check if the CSV file exists and create it if it doesn’t using the create_csv
function.
def main():
if not os.path.exists("contacts.csv"):
create_csv()
Next, we load the contacts from the CSV file using the load_csv
function and display a menu with options to add, search, update, and delete contacts.
def main():
if not os.path.exists("contacts.csv"):
create_csv()
contacts = load_csv()
while True:
print("Contact Book")
print("1. Add Contact")
print("2. Search Contact")
print("3. Update Contact")
print("4. Delete Contact")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == "1":
name = input("Enter name: ")
phone = input("Enter phone: ")
email = input("Enter email: ")
add_contact(name, phone, email)
print("Contact added successfully.")
elif choice == "2":
name = input("Enter name: ")
contact = search_contact(contacts, name)
if contact:
print("Name: ", contact["Name"])
print("Phone: ", contact["Phone"])
print("Email: ", contact["Email"])
else:
print("Contact not found.")
elif choice == "3":
name = input("Enter name: ")
phone = input("Enter new phone: ")
email = input("Enter new email: ")
if update_contact(contacts, name, phone, email):
print("Contact updated successfully.")
else:
print("Contact not found.")
elif choice == "4":
name = input("Enter name: ")
if delete_contact(contacts, name):
print("Contact deleted successfully.")
else:
print("Contact not found.")
elif choice == "5":
sys.exit()
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
Run the code
You can run this code by saving it in a Python file (e.g., contact_book.py
) and running it from the command line with the command python contact_book.py
. The program will create a CSV file to store contacts if one doesn’t exist, then display a menu with options to add, search, update, and delete contacts.
Conclusion of python project
In conclusion, the Contact Book project in Python is a simple but useful application for managing a list of contacts. The program allows the user to add, search, update, and delete contacts from a CSV file.
The code makes use of the csv module to read and write to the file, and includes error handling to prevent the program from crashing in case of invalid inputs.
This project demonstrates how to work with files and data structures in Python, and can be a good starting point for building more complex applications.
0 Comments