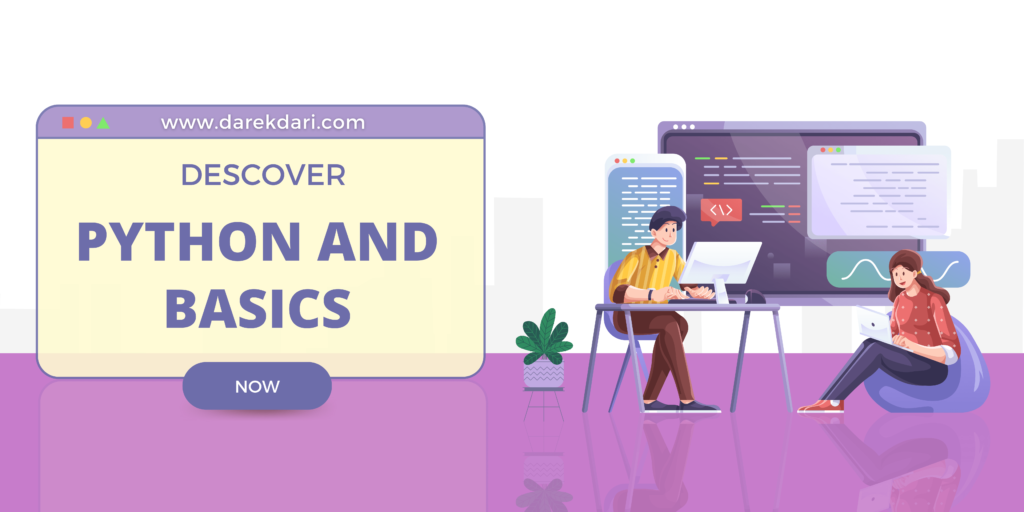
Python is a high-level, interpreted, general-purpose programming language with many uses, including web development, data analysis, machine learning, and more. It is very simple to learn. Python and its fundamentals will be discussed in this post.
Python array
The array is represented in Python using the array module. To create a homogeneous array, which requires that each member in the array be of the same data type, use the array module.
First of all, to use the array
module, you need to import it first:
import array
Once the array module is imported, you can create an array by using the array() function, which takes two arguments. The first argument specifies the data type of the elements, while the second argument provides the initial values of the elements.
Example:
import array
# create an array of integers
arr = array.array('i', [1, 2, 3, 4, 5])
# print the array
for i in arr:
print(i)
Using the append() and insert() functions, you can also add or remove elements from an array:
# append an element to the array
arr.append(6)
# insert an element at a specific position in the array
arr.insert(3, 7)
Just like in any other programming language, you can use the index of an element to retrieve it from an array in Python. The array’s length, n, is indicated by the indexes of the first and last elements, which are 0 and n-1, respectively.
# access the elements of the array
print(arr[0]) # Output: 1
print(arr[3]) # Output: 7
print(arr[-1]) # Output: 6 (accessing the last element using negative indexing)
An element in the array can also have its value changed by having a new value assigned to it:
# modify the value of an element
arr[2] = 8
Finally, you can get the length of an array using the len()
function:
# get the length of the array
print(len(arr)) # Output: 6
Python break
The break command in Python is utilized to immediately stop the execution of a loop. Whenever a break statement is encountered within a loop, such as a for or while loop, the loop is immediately terminated, and the statement that comes after the loop is executed instead.
Example:
i = 0
while i < 10:
print(i)
i += 1
if i == 5:
break
In the above code, we have initialized a variable
i
to 0 and used awhile
loop to print the value ofi
on each iteration. Inside the loop, we have incremented the value ofi
by 1 and added anif
statement to check ifi
is equal to 5. Ifi
is equal to 5, thebreak
statement is executed, which terminates the loop prematurely.
The output:
0
1
2
3
4
As you can see, the loop terminated when i
was equal to 5, and the program execution continued with the statement that follows the loop.
The break
statement can also be used inside a for
loop:
for i in range(10):
print(i)
if i == 5:
break
In the above code, the for loop is used to iterate over the range of integers from 0 to 9 repeatedly. An if statement is included in the loop’s code to check if the value of i is equal to 5, and the value of i is printed. If i equals 5, the break statement is executed, abruptly terminating the loop.
Output
0
1
2
3
4
5
As you can see, the loop ended when i equaled 5, and the sentence that follows the loop is what the computer did next.
When you want to end a loop early depending on a given circumstance, you frequently utilize the break statement, which is a strong tool for regulating program flow.
Python class: python and class
A class in Python serves as a guide for building objects with certain properties and actions. When defining a class, the class keyword is used, followed by the class name, and the definition is contained in a block of code that may also contain attributes and methods.
Define a class in python:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I'm {self.age} years old.")
A class called Person has been defined in the code above. It has the characteristics name and age, as well as the method say_hello(). A particular method known as the constructor, which is invoked when an object of the class is created, is the init() method. Utilizing the data supplied as inputs, it initializes the name and age aspects of the object.
The say_hello() function is a straightforward method that uses the name and age characteristics of the object to output a message to the terminal.
You can just call the class name followed by parentheses to construct an object of the Person class:
person1 = Person("John", 25)
In the code above, a Person class object was created and assigned to a variable called person1. The constructor of the class receives two arguments, “John” and 25, which it uses to initialize the name and age attributes of the object.
The dot notation can be used to access an object’s attributes and methods:
print(person1.name) # Output: John
print(person1.age) # Output: 25
person1.say_hello() # Output: Hello, my name is John and I'm 25 years old.
As seen here, the code above accesses the name and age attributes of the person1 object using the dot notation. Using the same approach, the object’s say_hello() method is also called.
Python and dataclass: python dataclass
In Python, a data class is a class primarily used to store data. While it resembles a regular class, it is designed solely to hold data and does not include business logic or complex methods.
The dataclass decorator, which is part of the dataclasses package made available in Python 3.7, can be used to build a data class.
Define python dataclass
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
In the code above, we have defined a data class named Person with the attributes name and age. We use annotations, which come after the attribute name and are separated by colons, to define the types of these attributes. When a class is created, the @dataclass decorator automatically generates several special methods based on the class’s properties, including init(), repr(), and eq().
Class calling
You can just call the class name followed by parentheses to construct an object of the Person class:
person1 = Person("John", 25)
In the code above, a Person class object was created and assigned to a variable called person1. The constructor of the class receives two arguments, “John” and 25, which it uses to initialize the name and age attributes of the object.
You can use the dot notation to obtain an object’s attributes:
print(person1.name) # Output: John
print(person1.age) # Output: 25
Utilizing a data class has the advantage of providing a clear and understandable approach to building basic classes that primarily serve as data storage units. The @dataclass decorator’s unique methods automate many typical operations, making the code required to create and operate objects of the class simpler.
Note that the dataclasses module is only available in Python 3.7 and later, so if you are using an earlier version of Python, you will need to define data classes manually.
Python datetime
The datetime module makes it possible to work with dates and times in Python. This module includes classes for working with date objects, time objects, and datetime objects, which combine date and time information.
Example
import datetime
# Create a date object
d = datetime.date(2023, 4, 19)
print(d) # Output: 2023-04-19
# Create a time object
t = datetime.time(12, 30, 15)
print(t) # Output: 12:30:15
# Create a datetime object
dt = datetime.datetime(2023, 4, 19, 12, 30, 15)
print(dt) # Output: 2023-04-19 12:30:15
# Get the current date and time
now = datetime.datetime.now()
print(now) # Output: 2023-04-19 13:45:23.491405
In the code above, we first import the datetime module. Then, we create a date object representing April 19, 2023, a time object representing 12:30:15, and a datetime object representing both the date and the time on April 19, 2023.
In addition, we use the datetime module to find the current date and time using the datetime.now() method, which provides a datetime object containing the information.
Once we have a datetime object, we can use characteristics like year, month, day, hour, minute, second, and microsecond to access each of its distinct parts. On datetime objects, we may also apply arithmetic operations to add, subtract, or compute differences between them.
Calculate the difference between two datetime
objects (python and datetime)
import datetime
dt1 = datetime.datetime(2023, 4, 19, 12, 30, 15)
dt2 = datetime.datetime(2023, 4, 18, 10, 15, 30)
delta = dt1 - dt2
print(delta) # Output: 1 day, 2:14:45
In the code above, first, we create two datetime objects that represent the various dates and times. Then, we obtain the timedelta object, which represents the difference between the two datetime objects, by subtracting dt2 from dt1. The resulting timedelta object indicates a period of time that is 1 day, 2 hours, 14 minutes, and 45 seconds.
Please leave a comment and share the blog if you liked it and it helps you. Thank you in advance.
1 Comment
binance · March 21, 2024 at 4:57 pm
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.