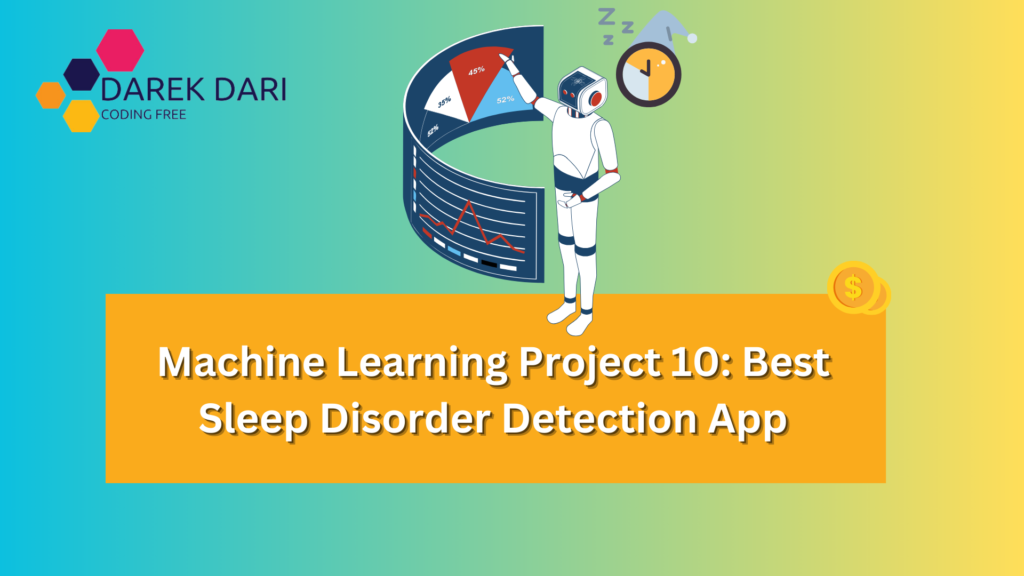
Table of Contents
Introduction to Sleep Disorder Detection
The main objective of this project is to develop a machine learning model that can accurately predict sleep disorders.
To achieve this, we will be using various input features such as age, gender, occupation, and sleep duration.
Also, check Machine Learning projects:
- Machine Learning Project 1: Honda Motor Stocks best Prices analysis
- Machine Learning Project 2: Diversity in Tech Companies Best EDA Analysis
- Machine Learning Project 3: Exploring Indian Cuisine Best Analysis
- Machine Learning Project 4: Exploring Video Game Data
- Machine Learning Project 5: Best Students Performance EDA
- Machine Learning Project 6: Obesity type Best EDA and classification
- Machine Learning Project 7: Best ChatGPT Reviews Analysis
Additionally, we have created an interactive web application using Streamlit, which will make it convenient for users to access these predictions. The workflow of this project involves data cleaning, analysis, model training, and deployment.
By following this workflow, users will be able to input their data and receive predictions for sleep disorders, along with helpful sleep tips.
About Our Database
This dataset is synthetic and contains 400 rows and 13 columns. It offers a detailed overview of sleep health and lifestyle factors. Here are the specifics and structure of the dataset:
Column Name | Description | Data Type |
---|---|---|
Person ID | An identifier for each individual. | Integer |
Gender | The gender of the person (Male/Female). | String |
Age | The age of the person in years. | Integer |
Occupation | The occupation or profession of the person. | String |
Sleep Duration (hours) | The number of hours the person sleeps per day. | Numeric |
Quality of Sleep (scale: 1-10) | A subjective rating of the quality of sleep, ranging from 1 to 10. | Integer |
Physical Activity Level (minutes/day) | The number of minutes the person engages in physical activity daily. | Integer |
Stress Level (scale: 1-10) | A subjective rating of the stress level experienced by the person, ranging from 1 to 10. | Integer |
BMI Category | The BMI category of the person (e.g., Underweight, Normal, Overweight). | String |
Blood Pressure (systolic/diastolic) | The blood pressure measurement of the person, indicated as systolic pressure over diastolic pressure. | String |
Heart Rate (bpm) | The resting heart rate of the person in beats per minute. | Integer |
Daily Steps | The number of steps the person takes per day. | Integer |
Sleep Disorder | The presence or absence of a sleep disorder in the person (None, Insomnia, Sleep Apnea). | String |
Additional Details for the Sleep Disorder
Column:
Category | Description |
---|---|
None | The individual does not exhibit any specific sleep disorder. |
Insomnia | The individual experiences difficulty falling asleep or staying asleep, leading to inadequate or poor-quality sleep. |
Sleep Apnea | The individual suffers from pauses in breathing during sleep, resulting in disrupted sleep patterns and potential health risks. |
This table summarizes the key elements and data types of the Sleep Health and Lifestyle Dataset, providing a clear overview of the data structure and its components.
Let’s Code And Use Machine Learning
Data Cleaning and Analysis
Data Import: Received the dataset on sleep disorders, filled with information on sleep patterns, demographics, and health data.
Dataset link: https://www.kaggle.com/datasets/uom190346a/sleep-health-and-lifestyle-dataset/data
Data Cleaning:
- Dealing with Missing Values: Noticed any missing data? Replaced them with either the average or the most common values, depending on the data type.
- Eliminating Duplicates: Spotted any duplicates? Removed them to maintain a clean dataset.
- Identifying and Handling Outliers: Identified any unusual data points? Checked if they impact the analysis and either corrected or removed them accordingly.
Exploratory Data Analysis (EDA)
- Summary Statistics: Analyzed the data to understand the distribution of variables.
- Data Visualizations: Incorporated various graphs such as histograms, bar charts, and box plots to explore the relationship between different features and sleep disorder categories.
Feature Engineering
To encode the categorical variables, I transformed gender, occupation, and BMI categories into numerical values using label encoding. It’s like introducing a new language to the computer.
For feature scaling, I employed MinMaxScaler to standardize the data. This ensures that everything is on an equal footing, resulting in smoother model performance.
Additionally, I applied some creativity and generated new features based on our existing knowledge of the data. It’s like discovering hidden treasures and extracting more information from what we already have.
Model Training
Sure, let me explain what happened. To begin with, I divided the cleaned dataset into two sets: one for training and the other for testing. I did this in an 80-20 ratio so that we could evaluate the model’s performance.
After that, I experimented with various machine learning models such as Decision Trees, Random Forests, and Gradient Boosting Machines (GBM). Eventually, I chose the Decision Tree model because it struck a nice balance between simplicity and accuracy.
Moving on, I trained the Decision Tree model using the training dataset. To enhance its performance even further, I fine-tuned the model’s hyperparameters through cross-validation.
This step involved making adjustments to the model to achieve optimal results. Once the model was fully trained, I assessed its performance on the test set using metrics like accuracy, precision, recall, and F1-score.
Additionally, I used confusion matrices to visualize how the model classified different items, providing a comprehensive understanding of its performance.
Streamlit App Development
Version 1: Alright, so here’s the lowdown. To start off, I created a Streamlit environment to develop a cool web app focused on data. Imagine it as a one-stop destination for interactive data-driven awesomeness.
After that, I got to work and designed the prediction interface. Envision a sleek interface where users can input details like age, gender, occupation, and sleep duration. Then, with just a simple click, the app generates predictions based on those inputs, along with personalized sleep tips.
But hold on, there’s more! I didn’t stop there. I also added another section in the app for all the interesting analytics and insights. We’re talking about interactive visualizations powered by Plotly, providing users with a deeper understanding of the data.
And the best part? I deployed the entire thing on Streamlit Cloud. Now, anyone with internet access can visit, enter their information, and receive expert sleep predictions right at their fingertips. It’s convenient, data-driven goodness, served up fresh and easy.
Web App and GitHub Links
- Web App Link: Click Here To Visit Streamlit Web App
- GitHub Repo: Click Here To Go To GitHub Repo
Sleep Disorder Detection Prediction
import pandas as pd
import warnings
warnings.filterwarnings('ignore')
# Load dataset
file_path = '/kaggle/input/sleep-health-and-lifestyle-dataset/Sleep_health_and_lifestyle_dataset.csv'
df = pd.read_csv(file_path)
# Display first few rows
df.head()
Data Cleaning
# Checking for missing values
df.isnull().sum()
# Displaying dataset information
df.info()
# Displaying descriptive statistics
df.describe()
# Filling missing values for 'Sleep Disorder'
df['Sleep Disorder'] = df['Sleep Disorder'].fillna('No Disorder')
# Splitting 'Blood Pressure' into 'SYSTOLIC' and 'DIASTOLIC'
df[['SYSTOLIC', 'DIASTOLIC']] = df['Blood Pressure'].str.split('/', expand=True).astype(float)
# Dropping unnecessary columns
data = df.drop(['Person ID', 'Blood Pressure'], axis=1)
data.head()
Data Analysis
import seaborn as sns
import matplotlib.pyplot as plt
import plotly.express as px
# Correlation matrix
corr = data.corr()
plt.figure(figsize=(10, 10))
sns.heatmap(corr, annot=True, cmap='coolwarm', fmt=".2f", linewidths=0.5)
plt.title('Correlation Matrix')
plt.show()
# Visualizations with Plotly
fig = px.histogram(data, x='Sleep Disorder', title='Distribution of Sleep Disorder', color='Sleep Disorder', template='plotly_dark')
fig.show()
fig = px.bar(data, x='Sleep Disorder', color='Gender', title='Gender-wise Sleep Disorder Distribution', template='plotly_dark')
fig.show()
# Additional visualizations...
Feature Engineering and Encoding
# Encoding categorical variables
from sklearn.preprocessing import LabelEncoder
label_encoder = LabelEncoder()
cat_columns = ['Occupation', 'BMI Category', 'Sleep Disorder', 'Gender']
for col in cat_columns:
data[col] = label_encoder.fit_transform(data[col])
# Balancing and scaling
from imblearn.over_sampling import SMOTE
from sklearn.preprocessing import MinMaxScaler
X = data.drop('Sleep Disorder', axis=1)
y = data['Sleep Disorder']
smote = SMOTE(random_state=1)
x_resampled, y_resampled = smote.fit_resample(X, y)
scaler = MinMaxScaler()
x_scaled = scaler.fit_transform(x_resampled)
Model Building
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
x_train, x_test, y_train, y_test = train_test_split(x_scaled, y_resampled, train_size=0.8, test_size=0.2, random_state=4)
# Training KNN model
model = KNeighborsClassifier(n_neighbors=3)
model.fit(x_train, y_train)
y_pred = model.predict(x_test)
# Evaluating model performance
accuracy = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred, average='weighted')
recall = recall_score(y_test, y_pred, average='weighted')
f1 = f1_score(y_test, y_pred, average='weighted')
print("Accuracy:", accuracy)
print("Precision:", precision)
print("Recall:", recall)
print("F1-score:", f1)
What Did We Use in the Code?
Let me break it down for you. First off, you loaded up your dataset, which was all about sleep health and lifestyle, into a pandas DataFrame. Then, you took a peek at the first few rows to get a feel for what you’re working with.
When it came to cleaning up your data, you did a few key things. You checked for missing values, got the lowdown on your dataset with info()
, and looked at some descriptive statistics.
Then, you tackled those missing values in the ‘Sleep Disorder’ column by filling them in with ‘No Disorder’. You also split up the ‘Blood Pressure’ column into systolic and diastolic values for better analysis. Finally, you dropped some columns that weren’t needed for your analysis.
Next up, you dove into some data analysis. You visualized correlations between different features using seaborn’s heatmap and explored the distribution of sleep disorders with Plotly. Plus, you checked out sleep disorder distributions by gender, adding some more insight to your analysis.
For feature engineering and encoding, you got down to business. You encoded categorical variables like ‘Occupation’, ‘BMI Category’, ‘Sleep Disorder’, and ‘Gender’ using label encoding to turn them into numerical form.
Then, you balanced and scaled your data to prep it for modeling, using techniques like SMOTE to handle imbalanced classes and MinMaxScaler for normalization.
Now, onto the fun part – building your model! You split your data into training and testing sets, with 80% for training and 20% for testing.
Then, you trained a K Nearest Neighbors (KNN) classifier on the training data and made predictions on the test data. After that, you evaluated your model’s performance using metrics like accuracy, precision, recall, and F1-score.
And that’s a wrap! You went from cleaning and analyzing your data to training and evaluating a machine learning model, all in one smooth process.
What is the best app to check sleep quality?
Ah, for keeping tabs on your sleep game, there’s a handful of apps that folks swear by. One popular pick is Sleep Cycle. It tracks your snooze patterns using your phone’s microphone or accelerometer, then wakes you up during your lightest sleep phase for a smoother morning wake-up call.
Another crowd favorite is Fitbit, especially if you’re already sporting one of their smartwatches or fitness trackers. It not only logs your z’s but also gives you insights into your overall health and activity levels.
If you’re into the whole mindfulness vibe, Headspace isn’t just about meditation anymore. They’ve got a nifty feature called “Sleepcasts” designed to ease you into dreamland with soothing bedtime stories and sounds.
And let’s not forget SleepScore. It uses sonar technology to monitor your sleep without needing to wear any gadgets. Just pop your phone on the nightstand, and it’ll do the rest.
So, take your pick! Whether you’re all about tracking your sleep cycles, want to dive into the nitty-gritty of your health data, or just need some calming bedtime vibes, there’s an app out there with your name on it.
Which sleep tracker is most accurate?
When it comes to pinpointing the most accurate sleep tracker, it’s a bit like trying to pick the perfect pillow—everyone’s got their own preference. That being said, a few names tend to pop up in the accuracy game more often than others.
Fitbit has garnered quite a reputation for its accuracy, thanks to its combination of heart rate monitoring, movement tracking, and sleep stage analysis. Users often find its sleep data aligns pretty closely with how they feel when they wake up in the morning.
Withings Sleep is another contender, particularly if you’re after something that doesn’t require you to wear anything on your wrist. It slips right under your mattress and uses advanced sensors to track your sleep cycles, heart rate, and snoring patterns.
If you’re a data geek looking for super detailed insights, Oura Ring might be your jam. This sleek little ring tracks your heart rate variability, body temperature, and movement to give you a comprehensive picture of your sleep quality.
For those who prefer a smartphone app, Sleep Cycle has won over many fans. Its clever use of sound analysis to track your sleep stages, combined with smart alarm features, makes it a top choice for some.
Remember, though, accuracy can vary depending on factors like how well the device fits your lifestyle and personal sleep habits. So, it might take a bit of trial and error to find the perfect match for you.
Is there an app that can tell if you have sleep apnea?
While many apps are available to monitor your sleep patterns and quality, they are not typically designed to diagnose sleep apnea. This serious medical condition usually requires a formal diagnosis from a healthcare provider.
Nevertheless, some apps might offer clues or information that could hint at the presence of sleep apnea. For instance, apps that track snoring or detect breathing interruptions during sleep could signal potential symptoms of sleep apnea.
These apps can be helpful for keeping track of your sleep habits and collecting data to share with a doctor or sleep specialist for further assessment.
It’s crucial to remember that while these apps can be informative, they should not replace professional medical advice or diagnosis.
If you suspect you have sleep apnea or any other sleep disorder, it’s important to seek guidance from a healthcare professional for a thorough evaluation and suitable treatment options.
What’s the best free sleep tracker app?
There are various free sleep tracker apps to choose from, each offering unique features. “Sleep Cycle” is a popular choice with its user-friendly interface and advanced algorithms.
“Pillow” provides detailed sleep analysis and syncs with Apple Health. “Sleep Tracker by Sleepmatic” is another great option for comprehensive sleep tracking.
To find the best app for you, consider your needs, read reviews, and check compatibility with your device. Whether you want basic monitoring or advanced features like snoring detection, there’s a free sleep tracker app out there for you.
What type of machine is used to study sleep patterns and diagnose sleep disorders?
A polysomnography (PSG) machine is frequently utilized to examine sleep patterns and identify sleep disorders.
This device records different physiological measurements while a person is asleep, including brain waves, eye movements, muscle activity, heart rate, and breathing patterns.
Sleep specialists can evaluate sleep quality, identify irregularities, and diagnose conditions like sleep apnea, insomnia, narcolepsy, and REM sleep behavior disorder by analyzing the data gathered from a PSG.
Can an app detect sleep apnea?
Yes, machine learning apps have shown promise in detecting sleep apnea. These apps utilize algorithms trained on large datasets containing sleep-related physiological signals, such as breathing patterns, heart rate variability, and movement during sleep.
By analyzing these signals, machine learning models can identify patterns associated with sleep apnea episodes.
However, it’s essential to note that while machine learning apps can provide valuable insights and preliminary screenings for sleep apnea, they are not a replacement for professional medical diagnosis.
If someone suspects they have sleep apnea or any other sleep disorder, it’s crucial to consult a healthcare professional for a proper evaluation and diagnosis.
Related searches
- Sleep detection machine learning python
- Sleep detection machine learning pdf
- Best sleep detection machine learning
- Free sleep detection machine learning
- sleep stage detection
- sleep stage classification
- slumbernet: deep learning classification of sleep stages using residual neural networks
- sleep stage classification dataset
Conclusion
This project showcases the complete journey of creating a machine learning model to detect sleep disorders, starting from cleaning and analyzing data to training the model and launching it as a Streamlit app.
With this interactive app, users can input their data and get predictions, as well as receive useful sleep tips.
0 Comments