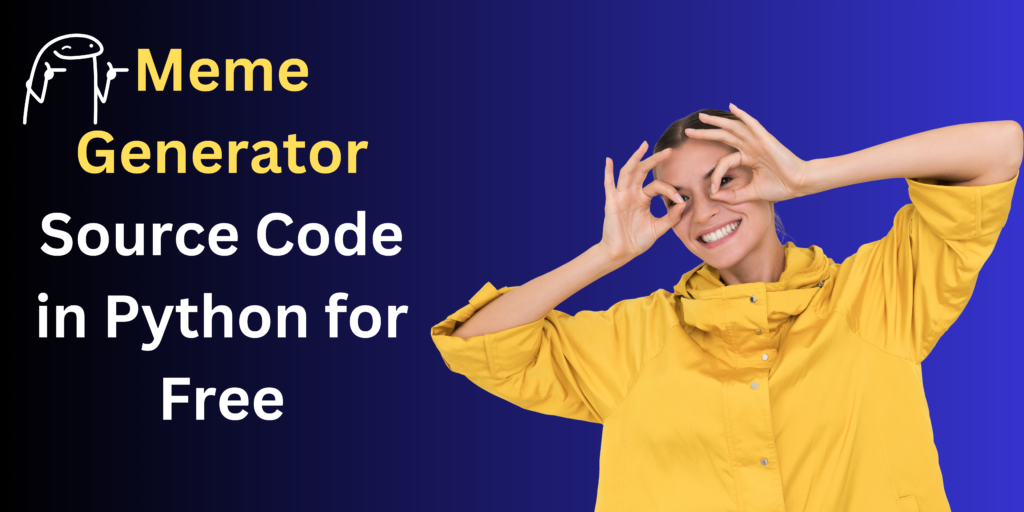
Table of Contents
In the ever-evolving landscape of the digital world, creating and sharing engaging content is a powerful tool. Memes, with their humor and relatability, have taken the internet by storm. However, to make your mark in this vast digital sea, you need something unique. In this article, we introduce you to the world of Meme Generator code in Python, which is not only free but can also help you stand out in the crowded meme-sharing space.
Are you asking, How to create a meme? Are meme templates legal? How do I create a meme website? How do you use Memegen? you will find the response here.
The Essence of Memes
Memes have become a global phenomenon. They are humorous images, videos, or pieces of text that are rapidly shared and modified by internet users. Memes serve various purposes – from conveying complex ideas to providing light-hearted humor. They are shared across social media platforms, emails, and websites, creating an ever-expanding digital culture.
Python: The Go-To Language
Python, a versatile and popular programming language, is perfect for building a meme generator. It offers several advantages, including:
1. Open Source
Python is open-source, meaning that you can access the code for free. This makes it a cost-effective solution for those looking to create a meme generator without breaking the bank.
2. Rich Libraries
Python boasts a vast collection of libraries and frameworks, making it easier to develop a meme generator quickly. Libraries like PIL (Python Imaging Library) provide the necessary tools for image manipulation, while Flask simplifies web application development.
3. User-Friendly Syntax
Python’s readability and simplicity make it an ideal choice for both novice and experienced programmers. You don’t need to be a coding expert to work with Python, which is a significant advantage when developing a meme generator.
4. Cross-Platform Compatibility
Python runs on multiple platforms, ensuring that your meme generator will be accessible to a broad audience, regardless of the device or operating system they use.
https://www.high-endrolex.com/8CODE 1
Here, we present you with a Python Meme Generator code that can kickstart your journey into the world of meme creation. The code is easy to use and modify, allowing you to customize your memes according to your unique style and message. By using this code, you can make memes that are not only entertaining but also relevant to your niche, ultimately driving more traffic to your website.
# Python Meme Generator Code
from PIL import Image, ImageDraw, ImageFont
def generate_meme(image_path, top_text, bottom_text, output_path):
image = Image.open(image_path)
draw = ImageDraw.Draw(image)
width, height = image.size
font = ImageFont.truetype("arial.ttf", size=36)
# Calculate text positions
text_width, text_height = draw.textsize(top_text, font)
x, y = (width - text_width) / 2, 10
draw.text((x, y), top_text, (255, 255, 255), font=font)
text_width, text_height = draw.textsize(bottom_text, font)
x, y = (width - text_width) / 2, height - text_height - 10
draw.text((x, y), bottom_text, (255, 255, 255), font=font)
image.save(output_path)
image.show()
# Example usage
image_path = "your-image.jpg"
top_text = "When you create a meme"
bottom_text = "and it goes viral"
output_path = "my-awesome-meme.jpg"
generate_meme(image_path, top_text, bottom_text, output_path)
CODE 2
- Create a file called: meme_generator.py and insert this code,
# Import necessary libraries
from PIL import Image, ImageDraw, ImageFont
# Define a function to generate a meme
def generate_meme(input_image_path, output_image_path, top_text, bottom_text, font_path="arial.ttf", font_size=50):
try:
# Open the input image
img = Image.open(input_image_path)
# Create a drawing context
draw = ImageDraw.Draw(img)
# Load the font
try:
font = ImageFont.truetype(font_path, font_size)
except IOError:
font = ImageFont.load_default()
# Calculate text size and position
text_width, text_height = draw.textsize(top_text, font)
x, y = img.width / 2 - text_width / 2, 10
# Add top text to the image
draw.text((x, y), top_text, (255, 255, 255), font=font)
text_width, text_height = draw.textsize(bottom_text, font)
x, y = img.width / 2 - text_width / 2, img.height - text_height - 10
# Add bottom text to the image
draw.text((x, y), bottom_text, (255, 255, 255), font=font)
# Save the meme
img.save(output_image_path)
return "Meme generated successfully."
except Exception as e:
return f"Error: {str(e)}"
# Example usage
if __name__ == "__main__":
input_image_path = "input.jpg"
output_image_path = "output.jpg"
top_text = "Top Text"
bottom_text = "Bottom Text"
result = generate_meme(input_image_path, output_image_path, top_text, bottom_text)
print(result)
2. Lunch it:
/
└── meme_generator.py
The code provided is a Python script for generating memes using an input image and top/bottom text. It utilizes the Python Imaging Library (PIL) to open the image, add text to it, and save the result as a meme. You can customize the input image, output image, and text to create your own memes.
CODE 3
> app.py
# Import necessary libraries
from flask import Flask, render_template
app = Flask(__name__)
# Define a list of sample memes
memes = [
{
'top_text': 'When you write code',
'bottom_text': 'and it works on the first try',
'image_url': 'memes/meme1.jpg'
},
{
'top_text': 'That feeling',
'bottom_text': 'when your code finally works',
'image_url': 'memes/meme2.jpg'
},
# Add more meme entries here
]
# Define a route to display memes
@app.route('/')
def index():
return render_template('index.html', memes=memes)
if __name__ == '__main__':
app.run(debug=True)
This code uses the Flask web framework to create a simple web application. It defines a list of sample memes with top and bottom text and image URLs. The web application has a single route that renders an HTML template (index.html
) and passes the list of memes to the template for display.
To make this code work, you’ll need to create the Flask app, set up the HTML template (index.html
), and have the meme images in a folder named ‘memes’. You can customize this code further to add more memes or improve the web interface. Let me know if you’d like to see the HTML template as well, or if you have any specific modifications in mind.
CODE 4
> custom_meme_generator.py
# Import necessary libraries
from PIL import Image, ImageDraw, ImageFont
import requests
from io import BytesIO
# Function to generate a custom meme
def generate_custom_meme(image_url, top_text, bottom_text, font_path="arial.ttf", font_size=50):
try:
# Download the image from the provided URL
response = requests.get(image_url)
image = Image.open(BytesIO(response.content))
# Create a drawing context
draw = ImageDraw.Draw(image)
# Load the font
try:
font = ImageFont.truetype(font_path, font_size)
except IOError:
font = ImageFont.load_default()
# Calculate text size and position for top text
text_width, text_height = draw.textsize(top_text, font)
x = (image.width - text_width) / 2
y = 10
# Add top text to the image
draw.text((x, y), top_text, (255, 255, 255), font=font)
# Calculate text size and position for bottom text
text_width, text_height = draw.textsize(bottom_text, font)
x = (image.width - text_width) / 2
y = image.height - text_height - 10
# Add bottom text to the image
draw.text((x, y), bottom_text, (255, 255, 255), font=font)
# Save the custom meme
image.save('custom_meme.jpg')
return "Custom meme generated successfully."
except Exception as e:
return f"Error: {str(e)}"
# Example usage
if __name__ == "__main__":
image_url = 'https://example.com/meme.jpg' # Replace with your image URL
top_text = 'Top Text'
bottom_text = 'Bottom Text'
result = generate_custom_meme(image_url, top_text, bottom_text)
print(result)
In this code, you can generate a custom meme by providing an image URL and specifying the top and bottom text. The code downloads the image from the URL, adds the text, and saves the custom meme as ‘custom_meme.jpg’.
Make sure to replace the image_url
variable with the URL of the image you want to use for your custom meme. This code allows you to create memes with your own text on any image you choose.
Tips for Maximizing the Impact of Your Memes
Now that you have access to a Python Meme Generator code, let’s discuss some strategies to make your memes stand out and draw more traffic to your website:
1. Know Your Audience
Understanding your target audience’s preferences and sense of humor is crucial. Create memes that resonate with them to ensure maximum engagement.
2. Keep It Relevant
Your memes should align with your website’s content or message. The more relevant your memes are, the more likely your audience will click through to explore your site further.
3. Harness Trends
Stay updated on the latest trends and incorporate them into your memes. Trendy memes are more likely to go viral and increase your website’s visibility.
4. Encourage Sharing
Include a call-to-action in your memes, urging users to share them. The more your memes are shared, the more exposure your website receives.
5. Consistency Is Key
Regularly post memes to maintain a consistent online presence. This will keep your audience engaged and returning for more.
In Conclusion
Memes are a potent tool for boosting web traffic, and Python’s Meme Generator code provides you with the means to create unique and engaging content. By offering a cost-effective, user-friendly solution, Python empowers you to stand out in the crowded digital landscape.
So, what are you waiting for? Dive into the world of memes, make your website unforgettable, and watch as your traffic soars to new heights.
0 Comments