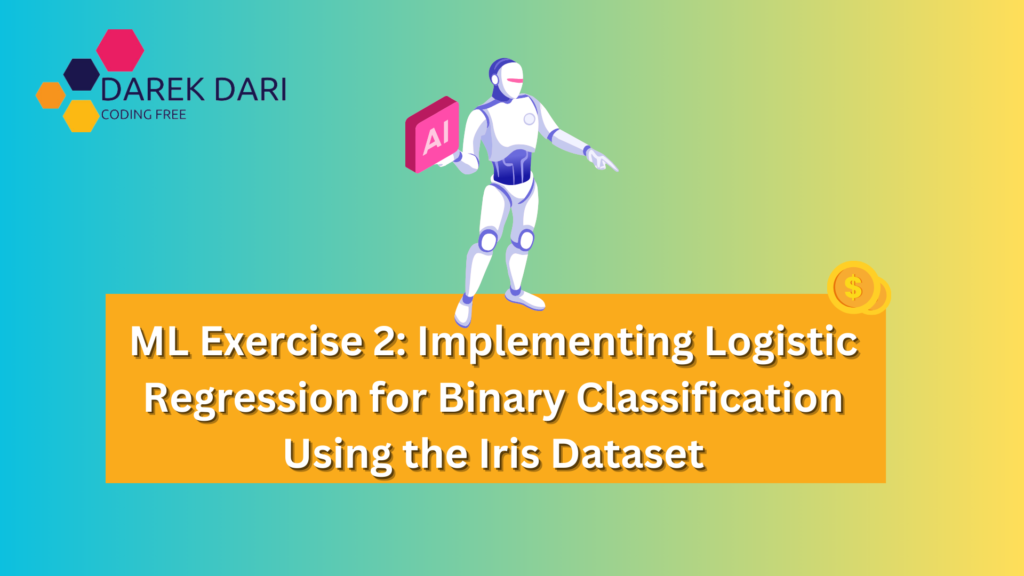
Table of Contents
Introduction
Logistic regression is a powerful algorithm commonly used for binary classification tasks in machine learning.
In this article, we explore how logistic regression can be practically implemented using Python, with a focus on the well-known Iris dataset.
This dataset is famous for its simplicity and effectiveness in demonstrating classification problems, containing information about iris flowers from three different species.
What is Logistic Regression ?
Logistic regression is a supervised learning algorithm specifically designed for binary classification tasks. It predicts the probability of a given input belonging to a certain class by utilizing the logistic function, also referred to as the sigmoid function.
Despite its name, logistic regression is primarily utilized for classification purposes rather than regression tasks.
Getting Started
Alright, let’s dive into the world of logistic regression with the classic Iris dataset! This blog will guide you through the process step by step. No need for fancy jargon; we’re keeping it simple and fun.
Load the Iris Dataset
First things first, we gotta load up our dataset. Imagine it’s like opening a treasure chest full of flowers, each with its own unique features.
import numpy as np
import pandas as pd
from sklearn.datasets import load_iris
The Iris dataset is like a goldmine for machine learning enthusiasts. It contains measurements of iris flowers, including their sepal length, sepal width, petal length, and petal width. These measurements act as the features we’ll use to classify the flowers.
Select the Relevant Classes
Now, let’s pick out the flowers we want to classify. We’re keeping it binary here, just like choosing between pizza and burgers for dinner.
iris = load_iris()
X = iris.data
y = iris.target
# Let's just grab the first two classes for simplicity's sake
X = X[y != 2]
y = y[y != 2]
In the Iris dataset, there are three classes of iris flowers: setosa, versicolor, and virginica. For our binary classification task, we’re simplifying things by only considering setosa and versicolor.
Split the Dataset
It’s time to divide our dataset into two parts: one for training our model and one for testing how well it performs.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Splitting the dataset helps us ensure that our model generalizes well to unseen data. We’ll use 80% of the data for training and 20% for testing.
Standardize Features
Think of standardizing features like putting all your ingredients in the same measuring cup before cooking. It ensures fairness and balance.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
Standardization ensures that each feature contributes equally to the learning process. It prevents features with larger scales from dominating the model during training.
Train the Logistic Regression Model
Alright, let’s train our model! Think of it as teaching a puppy new tricks – it takes patience and repetition.
from sklearn.linear_model import LogisticRegression
model = LogisticRegression()
model.fit(X_train, y_train)
During training, the logistic regression model learns the optimal weights for each feature to make accurate predictions. It’s like finding the right recipe for success!
Make Predictions
Time to put our model to the test! It’s like guessing which flavor of ice cream your friend will choose – but with flowers.
y_pred = model.predict(X_test)
Once trained, the model can predict the class labels of new data samples. We’ll use these predictions to evaluate how well our model performs.
Evaluate the Model
Alright, moment of truth! Let’s see how well our model did using accuracy as our measure. It’s like grading a test – we wanna see how many answers our model got right.
from sklearn.metrics import accuracy_score
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
By comparing the predicted labels to the actual labels in the test set, we can calculate the accuracy of our model. The higher the accuracy, the better our model is at classifying iris flowers.
What is logistic regression vs linear regression?
This table provides a quick comparison between linear regression and logistic regression, highlighting their key differences in terms of target variable, output, use cases, function, and typical applications.
Aspect | Linear Regression | Logistic Regression |
---|---|---|
Target Variable | Continuous | Categorical (Binary) |
Output | Predicts continuous values | Predicts probabilities between 0 and 1 |
Use Cases | Prediction of continuous outcomes (e.g., house prices) | Binary classification tasks (e.g., spam detection) |
Function | Fits a straight line to the data | Utilizes the logistic function to model probabilities |
Typical Application | Predicting house prices, stock prices | Predicting whether an email is spam or not |
When should logistic regression be used?
Logistic regression is often the preferred choice when you encounter a binary classification problem, where you need to categorize data into one of two classes. For example, if you’re trying to determine whether an email is spam or not, or if a patient has a specific disease, logistic regression is highly effective in these situations.
Additionally, logistic regression is useful when you want to estimate probabilities. Let’s say you need to assess the likelihood of a customer purchasing a product based on certain features. Logistic regression can provide you with probabilities, enabling you to make more informed decisions.
It’s important to consider the nature of your data as well. Logistic regression works best when the relationship between the features and the target variable can be represented by a linear decision boundary. So, if your data appears to follow a linear pattern, logistic regression is a reliable choice.
Interpretability is another significant advantage of logistic regression. The coefficients it generates are easily interpretable. For instance, you can confidently state that “for every unit increase in feature X, the odds of the target variable being true increase by a certain amount.” This makes it simpler to explain the model to others.
Logistic regression also performs well with small to medium-sized datasets. Even if your data isn’t massive, logistic regression can still provide meaningful results without overfitting, which can be a concern with more complex models.
Lastly, logistic regression is capable of handling situations where there is a high degree of variability or noise in the data. Even if the signal-to-noise ratio is low, logistic regression can still offer valuable insights.
Overall, logistic regression is a versatile and dependable tool for binary classification tasks, particularly when interpretability, simplicity, and moderate-sized datasets are important considerations.
What are the three types of logistic regression?
The three types of logistic regression are:
- Binary Logistic Regression: This is the most common type of logistic regression, where the target variable has only two possible outcomes, typically coded as 0 and 1. It’s used for binary classification tasks, such as predicting whether an email is spam or not, or whether a patient has a disease.
- Multinomial Logistic Regression: Also known as softmax regression, multinomial logistic regression is used when the target variable has three or more categories that are not ordered. It’s suitable for problems where there are more than two classes to predict, but the classes are not inherently ordered.
- Ordinal Logistic Regression: This type of logistic regression is used when the target variable has three or more ordered categories. It’s commonly employed in scenarios where the classes have a natural ordering, such as rating scales or levels of satisfaction. Ordinal logistic regression models the cumulative probabilities of the ordinal categories.
These three types of logistic regression cater to different types of classification problems, depending on the nature and structure of the target variable. Whether you’re dealing with binary outcomes, multiple unordered categories, or ordered categories, there’s a logistic regression variant to suit your needs.
What is the 3 parameter logistic regression?
The 3-parameter logistic regression, often abbreviated as 3PL regression, is a statistical model used primarily in psychometrics and educational measurement. It’s a variation of logistic regression specifically designed for items in tests or questionnaires where respondents provide binary responses (usually correct/incorrect or yes/no) to multiple-choice questions with three or more options.
In a 3PL regression model, each item is characterized by three parameters:
- Difficulty Parameter (b): This parameter represents the difficulty level of the item, indicating the level of proficiency or ability required for a respondent to correctly answer the item. Items with lower difficulty parameters are easier to answer, while those with higher difficulty parameters are more challenging.
- Discrimination Parameter (a): Also known as the discrimination index, this parameter measures how effectively the item discriminates between individuals with high ability levels and those with low ability levels. A high discrimination parameter indicates that the item effectively distinguishes between individuals of different abilities.
- Guessing Parameter (c): This parameter accounts for the probability of guessing the correct answer for an item, especially when the respondent has low ability or knowledge. It represents the likelihood of obtaining a correct response by guessing alone, independent of the respondent’s actual ability level.
The 3PL regression model is typically represented by the following equation:
[ P_i = c_i + (1 – c_i) \frac{1}{1 + e^{-(a_i (\theta – b_i))}} ]
Where:
- ( P_i ) is the probability of a correct response to item ( i ).
- ( \theta ) is the latent trait (e.g., ability or proficiency) of the respondent.
- ( a_i ), ( b_i ), and ( c_i ) are the discrimination, difficulty, and guessing parameters for item ( i ), respectively.
- ( e ) is the base of the natural logarithm.
The 3PL regression model is widely used in educational testing, particularly in computerized adaptive testing (CAT) systems, to estimate examinees’ abilities based on their responses to multiple-choice items.
It provides a flexible and effective framework for item response theory (IRT) analysis, allowing for the estimation of item and person parameters to improve the precision and fairness of assessments.
What is better than logistic regression?
Determining what can be considered “superior” to logistic regression depends on various factors such as your data’s characteristics, analysis objectives, and the trade-offs you’re willing to make. However, there are several alternative methods that may outperform logistic regression in specific scenarios:
- Support Vector Machines (SVM): SVM is skilled at creating both linear and non-linear decision boundaries, making it particularly useful in high-dimensional spaces and when classes are clearly separable.
- Random Forest: This ensemble learning technique generates multiple decision trees during training and then outputs the mode of the classes (classification) or the mean prediction (regression) of the individual trees. It is known for its robustness, scalability, and ability to handle large datasets with numerous features.
- Gradient Boosting Machines (GBM): GBM constructs a sequence of weak learners (usually decision trees) in a forward stage-wise manner. While it offers high predictive accuracy and flexibility, it may require more tuning compared to other algorithms.
- Neural Networks: Deep learning models, in particular, have gained popularity for their ability to identify intricate patterns and representations from data. They excel in tasks like image recognition and natural language processing, where large volumes of unstructured data are involved.
- XGBoost and LightGBM: These implementations of gradient boosting algorithms are optimized for efficiency and performance. They often outperform traditional gradient boosting methods like GBM and are widely used in data science competitions and real-world applications.
- K-Nearest Neighbors (KNN): KNN is a straightforward and intuitive classification algorithm that categorizes a data point based on the majority class of its nearest neighbors. While it is non-parametric and can handle complex decision boundaries, computational costs may be high with large datasets.
These alternative methods offer different strengths and weaknesses, so it’s important to consider your specific requirements and constraints when choosing the most suitable approach for your analysis.
What is the difference between multiple regression and logistic regression?
Here’s a table summarizing the differences between multiple regression and logistic regression:
Aspect | Multiple Regression | Logistic Regression |
---|---|---|
Outcome Variable | Continuous | Categorical (Binary) |
Modeling Approach | Models the relationship between independent variables and a continuous outcome using a linear equation. | Models the probability of belonging to a specific category using the logistic function. |
Output Interpretation | Coefficients represent the change in the mean value of the dependent variable for a one-unit change in the corresponding independent variable. | Coefficients represent the change in the log-odds of the outcome variable for a one-unit change in the corresponding independent variable. |
Examples | Predicting house prices based on features like square footage, number of bedrooms, and location. | Predicting whether a customer will churn based on customer demographics, usage patterns, and satisfaction scores. |
This table provides a concise overview of the differences between multiple regression and logistic regression, highlighting their distinct characteristics in terms of outcome variable, modeling approach, output interpretation, and examples of use cases.
Is XGBoost better than logistic regression?
Determining whether XGBoost is “better” than logistic regression depends on various factors such as the nature of the data, the complexity of the problem, and the specific goals of the analysis. Each algorithm has its own strengths and weaknesses, and the choice between them should be based on the particular requirements of the task at hand.
XGBoost:
- Strengths: XGBoost is a powerful ensemble learning algorithm known for its high predictive accuracy, scalability, and ability to handle complex relationships in the data. It performs well across a wide range of tasks and is particularly effective in competitions and real-world applications where achieving the highest possible accuracy is paramount.
- Weaknesses: XGBoost can be more computationally intensive and require more tuning compared to logistic regression. It may also be more prone to overfitting, especially with noisy or high-dimensional data.
Logistic Regression:
- Strengths: Logistic regression is a simple, interpretable, and computationally efficient algorithm that works well for binary classification tasks with linear decision boundaries. It’s particularly useful when interpretability is important or when dealing with small to medium-sized datasets.
- Weaknesses: Logistic regression may struggle with capturing complex relationships in the data and may not perform as well as more sophisticated algorithms like XGBoost in scenarios where the data is highly non-linear or contains interactions between features.
In general, if the problem involves binary classification and the relationship between the features and the target variable is relatively simple and linear, logistic regression may be a suitable choice.
However, if the goal is to achieve the highest possible predictive accuracy and the problem is more complex, XGBoost or other advanced machine learning algorithms may be preferred.
Ultimately, the choice between XGBoost and logistic regression should be based on empirical evaluation, considering factors such as performance metrics, computational resources, interpretability requirements, and the specific goals of the analysis.
Can logistic regression be multivariate?
Yes, logistic regression can indeed be multivariate. In the context of logistic regression, “multivariate” typically refers to having multiple predictor variables (also known as independent variables or features) rather than multiple outcome variables.
In a multivariate logistic regression model, there are multiple predictor variables that are used to predict the probability of a binary outcome.
Each predictor variable contributes to the prediction independently, and the model estimates the relationship between each predictor variable and the log-odds of the outcome.
For example, suppose we are trying to predict whether a patient has a particular disease based on several clinical features such as age, gender, blood pressure, and cholesterol level.
In this scenario, we would have multiple predictor variables (age, gender, blood pressure, cholesterol level) in the logistic regression model.
The logistic regression equation for a multivariate logistic regression model can be represented as follows:
[ P(Y=1|X) = \frac{1}{1 + e^{-(\beta_0 + \beta_1X_1 + \beta_2X_2 + \ldots + \beta_pX_p)}} ]
Where:
- ( P(Y=1|X) ) represents the probability of the outcome variable ( Y ) being 1 (e.g., having the disease) given the predictor variables ( X_1, X_2, \ldots, X_p ).
- ( \beta_0, \beta_1, \beta_2, \ldots, \beta_p ) are the coefficients (parameters) estimated by the logistic regression model.
- ( X_1, X_2, \ldots, X_p ) are the predictor variables.
- ( e ) is the base of the natural logarithm.
Each coefficient ( \beta_i ) represents the change in the log-odds of the outcome for a one-unit change in the corresponding predictor variable ( X_i ), holding all other variables constant.
In summary, logistic regression can indeed be multivariate, allowing for the inclusion of multiple predictor variables in the model to predict a binary outcome.
Conclusion
And there you have it, folks! We’ve successfully implemented logistic regression for binary classification using Python and the Iris dataset.
It’s like unlocking a new skill in your toolkit – the possibilities are endless! So go ahead, give it a try with your own datasets and see what fascinating insights you uncover. Happy coding!
0 Comments