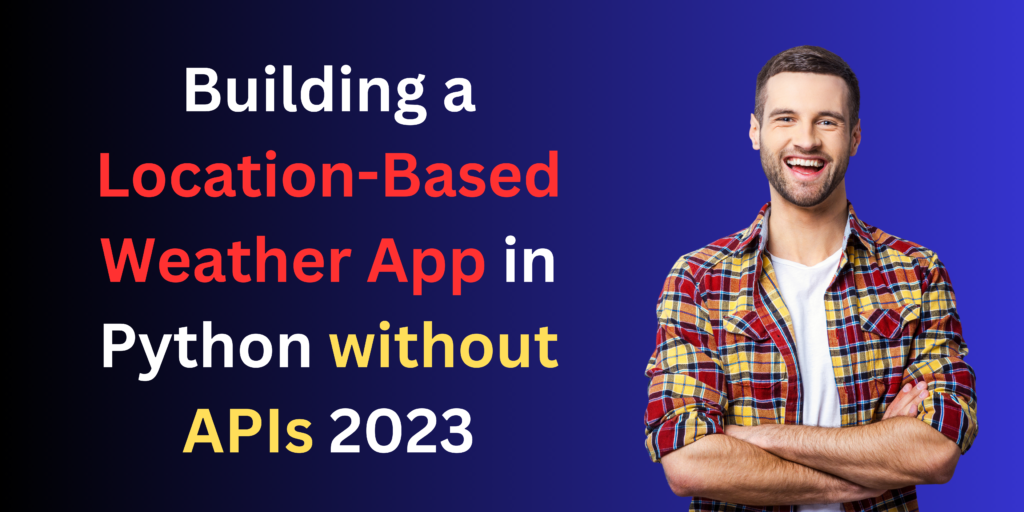
Table of Contents
Introduction to Weather App
Hey there, if you’re just getting started with coding, you’re in for a fun ride! In this article, we’ll show you how to create a Location-Based Weather App using Python. And guess what? We won’t be using any complicated external stuff, so it’s beginner-friendly all the way. Let’s dive in! This means complete control over the data and a deeper understanding of the mechanisms that power weather apps. Let’s dive in.
Tools and requirements
- Python
- Requests Library for making HTTP requests and fetching our data.
- Beautiful Soup is used in web scraping, parsing HTML, and extracting data.
- Tkinter to create a user-friendly interface.
- Geopy used for converting location names into coordinates.
Fetching Weather Data
We’ll begin by scraping weather data from a reliable source. One of the widely used websites for this purpose is the National Weather Service. We’ll need to identify the elements on the page that hold the data we want, such as temperature, humidity, and current conditions. With Beautiful Soup, this process becomes a breeze.
import requests
from bs4 import BeautifulSoup
def fetch_weather_data(location):
# Construct the URL for the desired location
url = f"https://forecast.weather.gov/MapClick.php?textField1={location}"
# Send an HTTP GET request
response = requests.get(url)
# Parse the HTML content of the page
soup = BeautifulSoup(response.content, 'html.parser')
# Extract the weather data
temperature = soup.find("p", class_="tombstone-container").text
humidity = soup.find("p", class_="period-name").find_next_sibling().text
conditions = soup.find("img")["alt"]
return temperature, humidity, conditions
Geocoding with Geopy
Our app’s main feature is location-based weather, so we’ll need to convert location names into latitude and longitude coordinates. Geopy makes this task a breeze. Let’s add this function:
from geopy.geocoders import Nominatim
def get_coordinates(location):
geolocator = Nominatim(user_agent="weather-app")
location = geolocator.geocode(location)
return location.latitude, location.longitude
Building the GUI
A user-friendly interface is crucial. Tkinter helps us create a simple yet effective GUI. We’ll add an entry field where users can input their location, a button to initiate the weather fetch, and a display area to show the results.
import tkinter as tk
def create_gui():
window = tk.Tk()
window.title("Location-Based Weather App")
label = tk.Label(window, text="Enter Location:")
label.pack()
entry = tk.Entry(window)
entry.pack()
button = tk.Button(window, text="Get Weather", command=lambda: display_weather(entry.get()))
button.pack()
display = tk.Label(window, text="")
display.pack()
window.mainloop()
Displaying Weather Data
Once we fetch the weather data, we need to present it to the user in a user-friendly format.
Display the temperature, humidity, and weather conditions, and consider adding weather icons for a visual touch.
def display_weather_data(temperature, humidity, conditions):
print("Weather Information:")
print(f"Temperature: {temperature}")
print(f"Humidity: {humidity}")
print(f"Conditions: {conditions}")
# You can add weather icons here based on conditions
if "rain" in conditions.lower():
print("Weather Icon: 🌧️")
elif "sun" in conditions.lower():
print("Weather Icon: ☀️")
else:
print("Weather Icon: 🌤️")
# Example usage:
temperature = "72°F"
humidity = "60%"
conditions = "Partly Cloudy"
display_weather_data(temperature, humidity, conditions)
Conclusion
Building a Location-Based Weather App in Python without relying on APIs is a challenging yet rewarding endeavor. It allows you to gain a deeper understanding of web scraping, data retrieval, and geocoding.
Moreover, it gives you full control over the data, making this project a perfect opportunity to expand your Python development skills. So, dive into the code, and may your weather app be as precise as your coding skills!
Happy coding, Love you, my lovely developers!
1 Comment
Machine Learning Project 1: Honda Motor Stocks Best Prices · May 23, 2024 at 6:41 pm
[…] Weather App […]