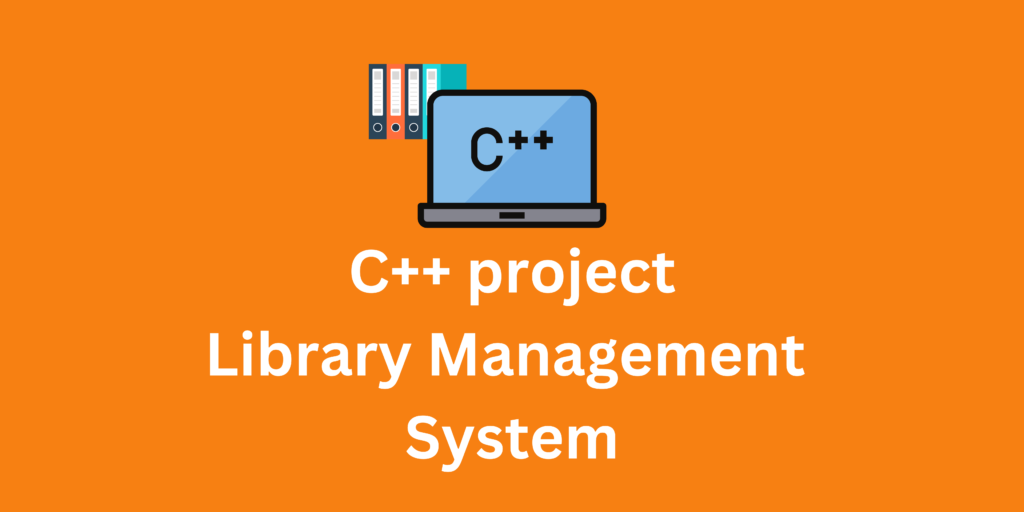
Create a library management system that allows library users to manage books. The system should allow users to add new books, search for books, borrow and return books, and view the available books.
Requirements:
- The system should have a user interface where users can interact with the program.
- Users should be able to add new books to the library by providing details such as the book title, author, ISBN, and publication year.
- Users should be able to search for books in the library based on various criteria such as title, author, or ISBN.
- The system should keep track of the availability of each book, indicating whether it is currently borrowed or available for borrowing.
- Users should be able to borrow books by providing their library card number or ID and the ISBN of the book.
- Users should be able to return books by providing the ISBN of the book they want to return.
- The system should maintain a list of borrowed books and provide the ability to display this list.
- Users should be able to view the details of a specific book, including its title, author, ISBN, publication year, and availability.
Code
#include <iostream>
#include <vector>
#include <string>
class Book {
private:
std::string title;
std::string author;
std::string isbn;
int publicationYear;
bool isAvailable;
public:
Book(const std::string& title, const std::string& author, const std::string& isbn, int publicationYear)
: title(title), author(author), isbn(isbn), publicationYear(publicationYear), isAvailable(true) {}
std::string getTitle() const {
return title;
}
std::string getAuthor() const {
return author;
}
std::string getISBN() const {
return isbn;
}
int getPublicationYear() const {
return publicationYear;
}
bool getAvailability() const {
return isAvailable;
}
void setAvailability(bool availability) {
isAvailable = availability;
}
};
class Library {
private:
std::vector<Book> books;
public:
void addBook(const Book& book) {
books.push_back(book);
}
void displayBooks() const {
std::cout << "Library Books:" << std::endl;
for (const auto& book : books) {
std::cout << "Title: " << book.getTitle() << std::endl;
std::cout << "Author: " << book.getAuthor() << std::endl;
std::cout << "ISBN: " << book.getISBN() << std::endl;
std::cout << "Publication Year: " << book.getPublicationYear() << std::endl;
std::cout << "Availability: " << (book.getAvailability() ? "Available" : "Borrowed") << std::endl;
std::cout << "---------------------" << std::endl;
}
}
Book* findBookByISBN(const std::string& isbn) {
for (auto& book : books) {
if (book.getISBN() == isbn) {
return &book;
}
}
return nullptr;
}
};
int main() {
Library library;
Book book1("The Great Gatsby", "F. Scott Fitzgerald", "9780743273565", 1925);
Book book2("To Kill a Mockingbird", "Harper Lee", "9780061120084", 1960);
Book book3("1984", "George Orwell", "9780451524935", 1949);
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
library.displayBooks();
std::string isbn;
std::cout << "Enter the ISBN of the book you want to borrow: ";
std::cin >> isbn;
Book* foundBook = library.findBookByISBN(isbn);
if (foundBook != nullptr) {
if (foundBook->getAvailability()) {
foundBook->setAvailability(false);
std::cout << "Book borrowed successfully!" << std::endl;
} else {
std::cout << "Book is not available for borrowing." << std::endl;
}
} else {
std::cout << "Book not found in the library." << std::endl;
}
return 0;
}
Optional Enhancements:
- Implement a feature to allow users to reserve books that are currently unavailable.
- Add a feature to generate reports, such as the most borrowed books or the books with the highest ratings.
- Implement a user authentication system to differentiate between librarians and regular users, allowing librarians to perform administrative tasks such as adding or removing books.
0 Comments