C++ projects with source code |
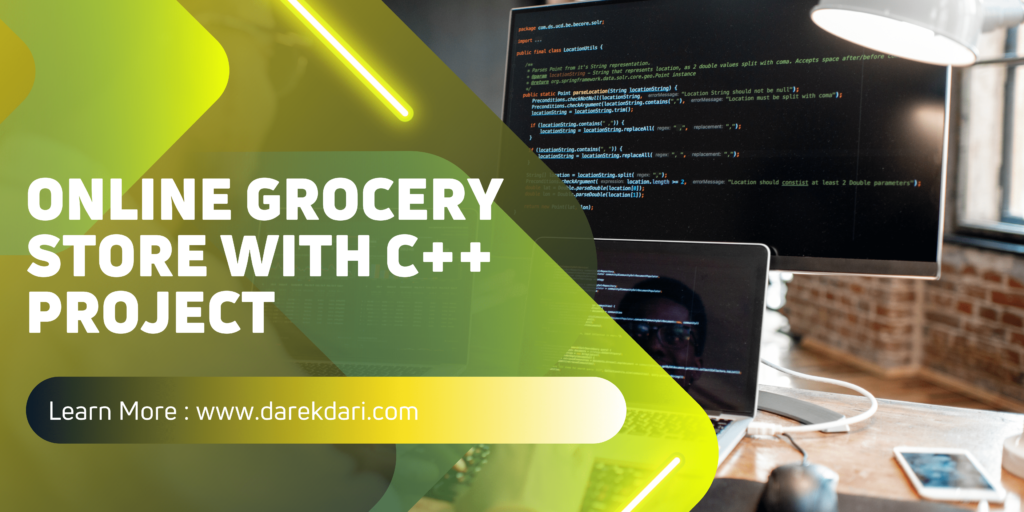
Table of Contents
Introduction to the C++ project (Online Grocery Store)
C++ project with source code: C++ can be used to build an online grocery store, however it’s not the best language for web development. However, by following these steps, you can create a console-based program that mimics an online grocery store:
- List the grocery products, their costs, and their availability.
- To enable customers to add products to their shopping carts, create a user interface.
- Put in place a payment mechanism to handle orders and produce receipts.
- Store client and order information in a database or file.
Here is a more thorough step-by-step tutorial on using C++ to build an online grocery store:

Steps for C++ project
Step 1: Define the terms “grocery items” and “price”
- Make a list of the groceries you need and their costs. This information can either be hardcoded or read from a file.
- To store the item data, you can use data structures like arrays, structs, or classes.
Step 2: Implement a user interface
- To enable customers to examine the available items and add them to their carts, provide a menu-driven user interface.
- In order to implement the user interface, loops and switch statements can be used.
- Make sure the software verifies user input to avoid mistakes.
Step 3: Implement a payment system
- Create a payment system that processes orders and generates receipts.
- You can use functions or classes to implement the payment system.
- Ensure that the payment system calculates the total cost of the order and deducts the appropriate amount from the customer’s balance.
Step 4: Store customer and order data
- Store customer and order data in a file or database.
- You can use file handling in C++ to read and write data to a file.
- Ensure that the program encrypts sensitive customer data to protect it from unauthorized access.
online grocery store grocery delivery grocery shopping online online grocery shopping grocery online shopping
Once you’ve completed these stages, you may test your application by simulating customer orders and seeing if the data storage and payment system function as intended. To make your program more comprehensive and user-friendly, you can also include more features like discounts, promotions, and client accounts.
c++ project ideas |
Remember those steps we talked about? First, we make a list of all the yummy stuff in our store and how much they cost.
Then, we make it so people can pick out what they want and put it in their cart. Next up, we figure out how they can pay for all their treats and get a receipt.
Lastly, we make sure we remember who bought what by keeping track of it all in our super secret database.
groceries online grocery online online grocery grocery delivery service groceries online delivery online grocery delivery
C++ project code: Online Grocery Store
This is a basic template for creating an online grocery store in C++. c project for beginners. However, please note that the code provided here is just a starting point, and you will need to customize it to fit your specific requirements and needs.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Product {
string name;
double price;
int quantity;
};
vector<Product> products = {
{"Apple", 2.99, 20},
{"Orange", 3.99, 15},
{"Banana", 1.99, 25},
{"Grapes", 5.99, 10},
{"Milk", 2.49, 30},
{"Eggs", 1.99, 40},
{"Bread", 3.49, 25}
};
struct CartItem {
string name;
double price;
int quantity;
};
vector<CartItem> cart;
void displayProducts() {
cout << "Welcome to our online grocery store!\n";
cout << "-------------------------------------\n";
cout << "Available Products:\n";
for (int i = 0; i < products.size(); i++) {
cout << i+1 << ". " << products[i].name << " - $" << products[i].price << " (" << products[i].quantity << " in stock)\n";
}
}
void addToCart(int index, int quantity) {
if (quantity > products[index].quantity) {
cout << "Sorry, we don't have enough items in stock.\n";
return;
}
cart.push_back({products[index].name, products[index].price, quantity});
products[index].quantity -= quantity;
cout << "Added " << quantity << " " << products[index].name << " to your cart.\n";
}
void displayCart() {
if (cart.empty()) {
cout << "Your cart is empty.\n";
return;
}
cout << "Your cart:\n";
double total = 0;
for (int i = 0; i < cart.size(); i++) {
cout << i+1 << ". " << cart[i].name << " - $" << cart[i].price << " (" << cart[i].quantity << ")\n";
total += cart[i].price * cart[i].quantity;
}
cout << "Total: $" << total << endl;
}
void checkout() {
if (cart.empty()) {
cout << "Your cart is empty.\n";
return;
}
cout << "Checkout:\n";
displayCart();
cout << "Total: $" << getTotal() << endl;
cout << "Enter your payment information:\n";
// TODO: Implement payment processing logic here
cout << "Thank you for shopping with us!\n";
cart.clear();
}
int main() {
while (true) {
cout << "\n1. Display Products\n";
cout << "2. Add to Cart\n";
cout << "3. View Cart\n";
cout << "4. Checkout\n";
cout << "5. Exit\n";
int choice;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
displayProducts();
break;
case 2: {
int index, quantity;
cout << "Enter the product index: ";
cin >> index;
index--;
cout << "Enter the quantity: ";
cin >> quantity;
addToCart(index, quantity);
break;
}
case 3:
displayCart();
break
case 4:
checkout();
break;
case 5:
return 0;
default:
cout << "Invalid choice.\n";
break;
}
}
return 0;
}
ebt online shopping online stores that accept ebt stores that accept ebt online grocery shop online online grocery shop
Code explanation C++ project code: Online Grocery Store
The program uses two vector data structures: the products
vector stores the available products with their prices and quantities, and the cart
vector stores the items that the user has added to their cart.
The displayProducts()
function simply loops through the products
vector and prints out each product’s name, price, and quantity in stock.
The addToCart()
function takes two arguments: the index of the product to add to the cart (which is decremented by 1 to match the vector indexing), and the quantity of the product to add.
If the requested quantity is greater than the available quantity of the product, a message is displayed indicating that the store doesn’t have enough items in stock. Otherwise, the product is added to the cart, and the quantity of the product in the products
vector is decremented accordingly.
The displayCart()
function loops through the cart
vector and prints out each item’s name, price, and quantity. It also calculates and prints out the total cost of the items in the cart.
c projects github and c++ projects github |
The checkout()
function displays the contents of the cart using the displayCart()
function, calculates the total cost using the getTotal()
function, and prompts the user to enter their payment information. This function could be expanded to include payment processing logic.
Now, we looked at this example code for our grocery store. It’s like a blueprint that shows us how to set things up.
We’ve got functions for showing what’s in our store, adding stuff to our cart, and checking out. Plus, we’ve got some cool tricks to keep track of everything.
groceries shipped buy groceries online who accepts ebt online food online shopping ebt online shopping free delivery grocery stores that accept ebt online what grocery stores accept ebt online
Learn more
Conclusion
Alright, wrapping up our talk about C++ projects and that online grocery store we’ve been working on, let’s sum it all up.
So, when we’re talking about C++, it’s like having a super cool toolbox for building awesome stuff. We talked about how we can use it to make our own online grocery store, even though it’s not the best for making websites.
But hey, we can still make a really cool store where people can shop for their favorite snacks and goodies!
But hey, remember, this code is just the beginning! You can change it up and add your own special touches to make it even cooler.
And if you ever get stuck or need some inspiration, there are lots of other kids out there sharing their own C++ projects online. You can check out their stuff and maybe even share your own ideas!
So, to wrap it all up, C++ is like a big adventure where you get to build awesome things like our online grocery store. It’s all about learning and having fun while you do it. So, keep exploring, keep coding, and who knows what amazing stuff you’ll create next!
1 Comment
registrácia na binance · April 6, 2024 at 7:04 pm
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.