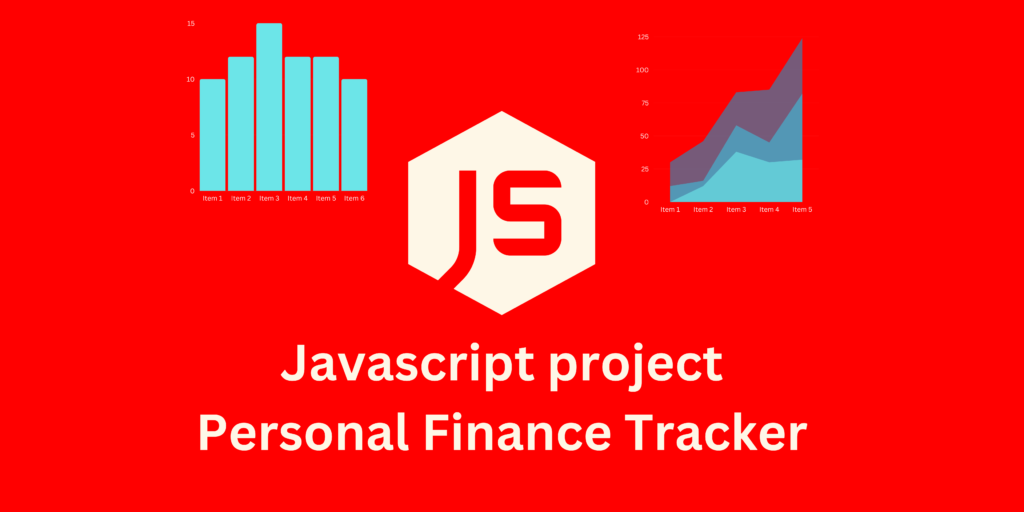
Table of Contents
Creating your own Personal Finance Tracker using JavaScript can be an empowering journey, allowing you to take control of your financial data. In this article, we’ll guide you through the steps to build your own finance tracking application. We’ll start with the basics and gradually add more advanced features to make it a robust tool.
Getting Started with personal finance tracker javascript project
Before diving into the code, ensure you have a basic understanding of HTML, CSS, and JavaScript. We won’t cover the absolute fundamentals here, but you should be comfortable with basic programming concepts.
HTML Structure
Let’s begin by setting up the HTML structure for our finance tracker. We’ll have a simple layout with input fields to add income, expenses, and a display area for the financial summary.
<!DOCTYPE html>
<html>
<head>
<title>Personal Finance Tracker</title>
</head>
<body>
<h1>Personal Finance Tracker</h1>
<h2>Income</h2>
<input type="number" id="incomeAmount">
<button onclick="addIncome()">Add Income</button>
<h2>Expenses</h2>
<input type="number" id="expenseAmount">
<button onclick="addExpense()">Add Expense</button>
<h2>Financial Summary</h2>
<div id="summary">
Total Income: $<span id="totalIncome">0</span><br>
Total Expenses: $<span id="totalExpenses">0</span><br>
Balance: $<span id="balance">0</span>
</div>
</body>
</html>
JavaScript Logic
Now, let’s add JavaScript to make our finance tracker functional. We’ll create functions to add income and expenses, update the financial summary, and handle calculations.
<script>
let totalIncome = 0;
let totalExpenses = 0;
function addIncome() {
const incomeAmount = parseFloat(document.getElementById('incomeAmount').value);
totalIncome += incomeAmount;
document.getElementById('totalIncome').textContent = totalIncome;
updateBalance();
}
function addExpense() {
const expenseAmount = parseFloat(document.getElementById('expenseAmount').value);
totalExpenses += expenseAmount;
document.getElementById('totalExpenses').textContent = totalExpenses;
updateBalance();
}
function updateBalance() {
const balance = totalIncome - totalExpenses;
document.getElementById('balance').textContent = balance;
}
</script>
Styling with CSS
We’ll also add some basic CSS to make the finance tracker visually appealing. Customize this as needed.
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
text-align: center;
}
h2 {
margin-top: 20px;
}
input, button {
margin: 10px;
}
#summary {
background-color: #f0f0f0;
padding: 10px;
border: 1px solid #ccc;
}
</style>
Enhancing Your Personal Finance Tracker
While the code above gives you a solid foundation for a Personal Finance Tracker, there are several ways you can enhance this project further:
- Data Persistence: Implement local storage or a backend database to save financial data securely.
- Categories: Allow users to categorize their income and expenses for better tracking. Categories like “Rent,” “Groceries,” and “Entertainment” can provide a detailed breakdown of expenses.
- Date Tracking: Add the ability to input and track transactions by date, making it easier to monitor your financial history.
- Charting: Visualize financial data using charts and graphs for a more intuitive experience. Chart.js is a popular library for creating interactive charts.
- Authentication: Consider adding user authentication for secure access to financial data. This ensures that your financial information remains private.
- Notifications: Implement notifications for important financial events, such as reaching a savings goal or exceeding a budget limit.
- Budget Planner: Extend the functionality to allow users to set and track budgets. Provide visual cues to indicate when a budget is close to being exceeded.
Remember that building your own personal finance tracker is a dynamic project. You can customize and expand it to meet your specific financial tracking needs. Have fun coding and mastering your finances!
What are the strategies should adopt bank for growth?
Banks can adopt various strategies for growth, including expanding product offerings and geographic reach, embracing technology, engaging in mergers and acquisitions, prioritizing risk management, and enhancing marketing and customer relationships. These strategies help attract new customers and increase revenue, contributing to the bank’s overall growth and success.
Conclusion
In summary, creating a Personal Finance Tracker with JavaScript offers a powerful tool for financial management. By following the provided steps and adding advanced features, you can tailor the project to your specific needs, fostering greater financial control and understanding through coding.
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments