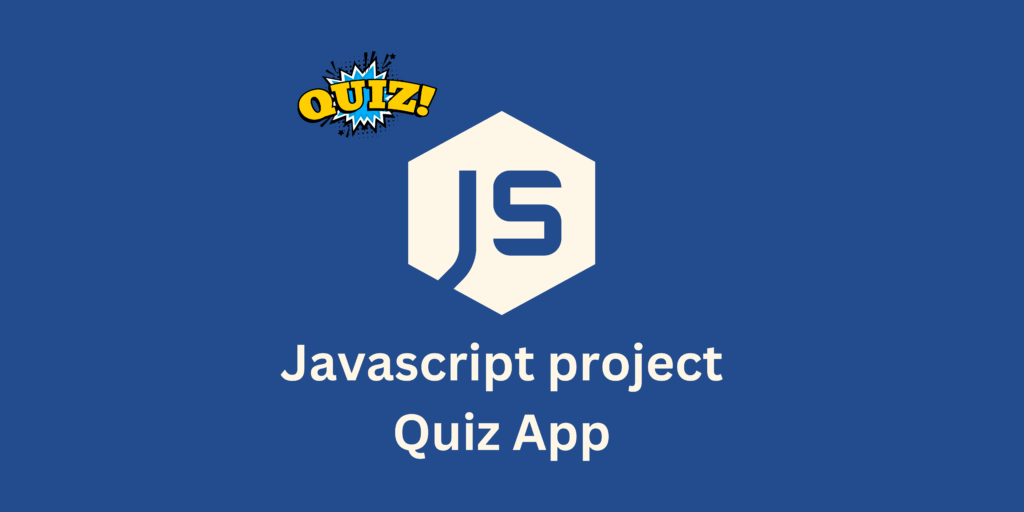
Javascript project: Quiz App: By putting users’ knowledge of various subjects to the test, a quiz app is a great tool for user engagement. Users can take quizzes on this app, and the results are displayed.
In this project, JavaScript will be used to build a quiz app. The user chooses an answer after the app displays a question with multiple choice options. The app will then determine whether the response is accurate and give the user feedback. Users can proceed through the quiz and view their results at the conclusion.
1.HTML
In the HTML, we create a container div with a class quiz-container
, and within that, we have a div with class question-container
and an empty unordered list with a class answer-container
. We also have two buttons, one to submit the answer and the other to move to the next question.
<div class="quiz-container">
<div class="question-container"></div>
<ul class="answer-container"></ul>
<button class="submit-btn">Submit Answer</button>
<button class="next-btn">Next Question</button>
</div>
2.CSS
In the CSS, we style the container and its contents, including the buttons, to be visually appealing.
.quiz-container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
background-color: #f7f7f7;
text-align: center;
}
.question-container {
margin-bottom: 20px;
}
.answer-container {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
margin-bottom: 20px;
}
label {
font-size: 1.2rem;
margin-bottom: 10px;
}
button {
background-color: #4286f4;
color: #fff;
font-size: 1.2rem;
padding: 10px 20px;
border-radius: 5px;
border: none;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #2267b4;
}
.hide {
display: none;
}
.result-container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
background-color: #f7f7f7;
text-align: center;
font-size: 1.2rem;
}
.reset-btn {
display: block;
margin: 20px auto 0;
padding: 10px 20px;
border-radius: 5px;
border: none;
background-color: #4286f4;
color: #fff;
font-size: 1.2rem;
cursor: pointer;
transition: background-color 0.3s ease;
}
.reset-btn:hover {
background-color: #2267b4;
}
3. JAVASCRIPT
In the JavaScript, we start by defining some variables:
quizContainer
: a reference to the container div.questionContainer
: a reference to the div that displays the question.answerContainer
: a reference to the unordered list that displays the answer choices.submitBtn
andnextBtn
: references to the submit and next buttons respectively.currentQuestion
: a variable that tracks the index of the current question.score
: a variable that tracks the user’s score.questions
: an array of objects that contain the questions, answer choices, and correct answers for the quiz.
Next, we define two functions:
showQuestion(question)
: this function takes a question object as an argument and displays the question and answer choices in the HTML. It also updates thecurrentQuestion
variable to reflect the new question.checkAnswer()
: this function checks whether the selected answer is correct and updates the score accordingly. It then displays feedback to the user indicating whether they were correct or not.
const quizContainer = document.querySelector('.quiz-container');
const questionContainer = document.querySelector('.question-container');
const answerContainer = document.querySelector('.answer-container');
const submitBtn = document.querySelector('.submit-btn');
const nextBtn = document.querySelector('.next-btn');
let currentQuestion = 0;
let score = 0;
const questions = [
{
question: 'What is the capital of France?',
answers: ['London', 'Paris', 'Madrid', 'Rome'],
correctAnswer: 'Paris'
},
{
question: 'What is the highest mountain in the world?',
answers: ['Mount Everest', 'K2', 'Makalu', 'Lhotse'],
correctAnswer: 'Mount Everest'
},
{
question: 'What is the largest planet in our solar system?',
answers: ['Mars', 'Venus', 'Jupiter', 'Saturn'],
correctAnswer: 'Jupiter'
}
];
function showQuestion(question) {
questionContainer.textContent = question.question;
answerContainer.innerHTML = '';
question.answers.forEach(answer => {
const li = document.createElement('li');
li.textContent = answer;
li.addEventListener('click', () => {
answerContainer.querySelectorAll('li').forEach(li => {
li.classList.remove('selected');
});
li.classList.add('selected');
});
answerContainer.appendChild(li);
});
currentQuestion++;
}
The checkAnswer()
function in a Quiz App using JavaScript is responsible for validating the user’s answer and displaying feedback to the user. Here’s an example implementation:
function checkAnswer() {
const selectedAnswer = document.querySelector('input[name="answer"]:checked');
if (!selectedAnswer) {
// If no answer is selected, display an error message
const feedback = document.querySelector('.feedback');
feedback.innerHTML = "Please select an answer";
feedback.style.color = "red";
} else {
// If an answer is selected, compare it to the correct answer
const userAnswer = selectedAnswer.value;
const correctAnswer = questions[currentQuestion].answer;
if (userAnswer === correctAnswer) {
// If the answer is correct, increment the score and display feedback
score++;
const feedback = document.querySelector('.feedback');
feedback.innerHTML = "Correct!";
feedback.style.color = "green";
} else {
// If the answer is incorrect, display feedback
const feedback = document.querySelector('.feedback');
feedback.innerHTML = "Sorry, that's incorrect";
feedback.style.color = "red";
}
// Disable answer options and show the next question button
const answerOptions = document.querySelectorAll('input[name="answer"]');
answerOptions.forEach(option => {
option.disabled = true;
});
nextBtn.classList.remove("hide");
submitBtn.disabled = true;
}
}
Learn more
In this implementation, the function starts by selecting the user’s answer from the radio buttons in the HTML using the querySelector()
method. If no answer is selected, an error message is displayed to the user. If an answer is selected, the function compares the user’s answer to the correct answer for the current question.
If the answer is correct, the score is incremented, and the feedback is displayed in green. If the answer is incorrect, feedback is displayed in red. The function also disables the answer options and shows the next question button while disabling the submit button. This ensures that the user cannot change their answer after submitting and progress through the quiz.
After the checkAnswer()
function, the next step in building a Quiz App using JavaScript is to define a function that displays the next question when the user clicks on the “Next” button.
function nextQuestion() {
// Reset feedback message
const feedback = document.querySelector('.feedback');
feedback.innerHTML = "";
// Enable answer options and hide the next question button
const answerOptions = document.querySelectorAll('input[name="answer"]');
answerOptions.forEach(option => {
option.disabled = false;
option.checked = false;
});
nextBtn.classList.add("hide");
submitBtn.disabled = false;
// Move to the next question
currentQuestion++;
if (currentQuestion < questions.length) {
// If there are more questions, display the next question
displayQuestion();
} else {
// If all questions are answered, show the final score
displayScore();
}
}
The next step in building a Quiz App using JavaScript is to define a function that displays the user’s final score once all the questions have been answered.
function displayScore() {
// Hide quiz questions and show score screen
quizContainer.classList.add("hide");
scoreContainer.classList.remove("hide");
// Display final score
const scorePercent = Math.round((score / questions.length) * 100);
const scoreText = `You scored ${score} out of ${questions.length}, which is ${scorePercent}%`;
const scoreElement = document.querySelector('.score');
scoreElement.innerHTML = scoreText;
}
The next step in building a Quiz App using JavaScript is to define a function that resets the quiz and allows the user to take it again.
function resetQuiz() {
// Reset quiz variables
currentQuestion = 0;
score = 0;
// Reset HTML elements
const answerOptions = document.querySelectorAll('input[name="answer"]');
answerOptions.forEach(option => {
option.disabled = false;
option.checked = false;
});
const feedback = document.querySelector('.feedback');
feedback.innerHTML = "";
submitBtn.disabled = false;
nextBtn.classList.add("hide");
// Show quiz questions and hide score screen
scoreContainer.classList.add("hide");
quizContainer.classList.remove("hide");
// Display first question
displayQuestion();
}
The next step in building a Quiz App using JavaScript is to add event listeners to the “Submit” and “Next” buttons so that the user can interact with the quiz.
// Add event listeners to buttons
submitBtn.addEventListener('click', checkAnswer);
nextBtn.addEventListener('click', nextQuestion);
resetBtn.addEventListener('click', resetQuiz);
The next step in building a Quiz App using JavaScript is to handle edge cases such as the user not answering a question, reaching the end of the quiz, and displaying appropriate feedback to the user.
function checkAnswer() {
// Check if an answer has been selected
const answerOptions = document.querySelectorAll('input[name="answer"]');
let answerSelected = false;
answerOptions.forEach(option => {
if (option.checked) {
answerSelected = true;
}
});
// If an answer has been selected, check if it's correct
if (answerSelected) {
const userAnswer = document.querySelector('input[name="answer"]:checked').value;
const correctAnswer = questions[currentQuestion].answer;
if (userAnswer === correctAnswer) {
feedback.innerHTML = "Correct!";
score++;
} else {
feedback.innerHTML = "Incorrect.";
}
// Disable answer options and show "Next" button
answerOptions.forEach(option => {
option.disabled = true;
});
submitBtn.disabled = true;
nextBtn.classList.remove("hide");
// If an answer has not been selected, display error message
} else {
feedback.innerHTML = "Please select an answer.";
}
}
function nextQuestion() {
// Check if there are more questions
if (currentQuestion < questions.length - 1) {
currentQuestion++;
displayQuestion();
feedback.innerHTML = "";
nextBtn.classList.add("hide");
submitBtn.disabled = false;
} else {
displayScore();
}
}
The next step in building a Quiz App using JavaScript is to display the user’s final score and allow them to retake the quiz.
function displayScore() {
// Hide quiz questions and feedback
quizContainer.classList.add("hide");
feedback.classList.add("hide");
// Display final score
resultContainer.classList.remove("hide");
resultContainer.innerHTML = `You scored ${score} out of ${questions.length}!`;
// Show "Reset" button
resetBtn.classList.remove("hide");
}
function resetQuiz() {
// Reset variables and UI
currentQuestion = 0;
score = 0;
feedback.innerHTML = "";
resultContainer.innerHTML = "";
quizContainer.classList.remove("hide");
feedback.classList.remove("hide");
resultContainer.classList.add("hide");
resetBtn.classList.add("hide");
// Display first question
displayQuestion();
}
0 Comments