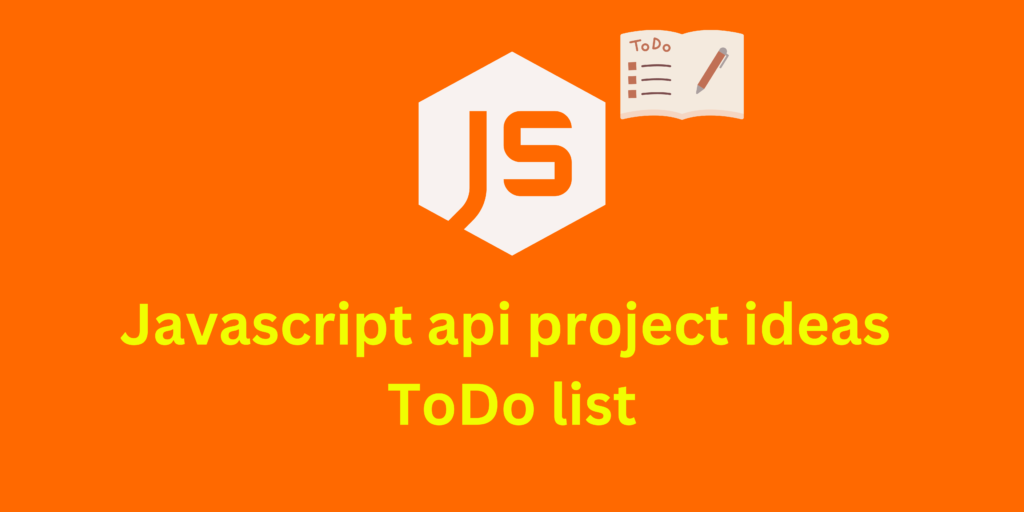
Javascript api project ideas: ToDo list: A web app that allows users to create and manage a to-do list. Users can add tasks, mark them as completed, and delete them as needed.
Description of Javascript api project ideas: ToDo list
A web app that allows users to create and manage a to-do list. Users can add tasks, mark them as completed, and delete them as needed.
HTML
<div class="container">
<h1>To-Do List</h1>
<form>
<input type="text" placeholder="Add task...">
<button type="submit">Add</button>
</form>
<ul class="list"></ul>
</div>
CSS
.container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
}
h1 {
text-align: center;
}
form {
display: flex;
margin-bottom: 20px;
}
input[type="text"] {
flex-grow: 1;
padding: 10px;
border: none;
}
button[type="submit"] {
padding: 10px;
border: none;
background-color: #3b8ad9;
color: #fff;
cursor: pointer;
}
ul {
list-style: none;
padding: 0;
}
li {
display: flex;
align-items: center;
margin-bottom: 10px;
}
.task {
flex-grow: 1;
margin-right: 10px;
}
.task.completed {
text-decoration: line-through;
}
Javascript
const form = document.querySelector('form');
const input = document.querySelector('input');
const ul = document.querySelector('ul');
form.addEventListener('submit', (event) => {
event.preventDefault();
if (input.value.trim() === '') {
return;
}
const li = document.createElement('li');
const taskSpan = document.createElement('span');
const deleteButton = document.createElement('button');
const completeButton = document.createElement('button');
taskSpan.textContent = input.value.trim();
deleteButton.textContent = 'Delete';
completeButton.textContent = 'Complete';
li.appendChild(taskSpan);
li.appendChild(deleteButton);
li.appendChild(completeButton);
ul.appendChild(li);
input.value = '';
});
ul.addEventListener('click', (event) => {
const target = event.target;
if (target.tagName === 'BUTTON') {
const li = target.parentElement;
if (target.textContent === 'Delete') {
ul.removeChild(li);
} else {
const taskSpan = li.querySelector('.task');
taskSpan.classList.toggle('completed');
}
}
});
This code uses event listeners to add functionality to the to-do list. The submit
event is used to add tasks to the list, and the click
event is used to mark tasks as completed and delete them.
0 Comments