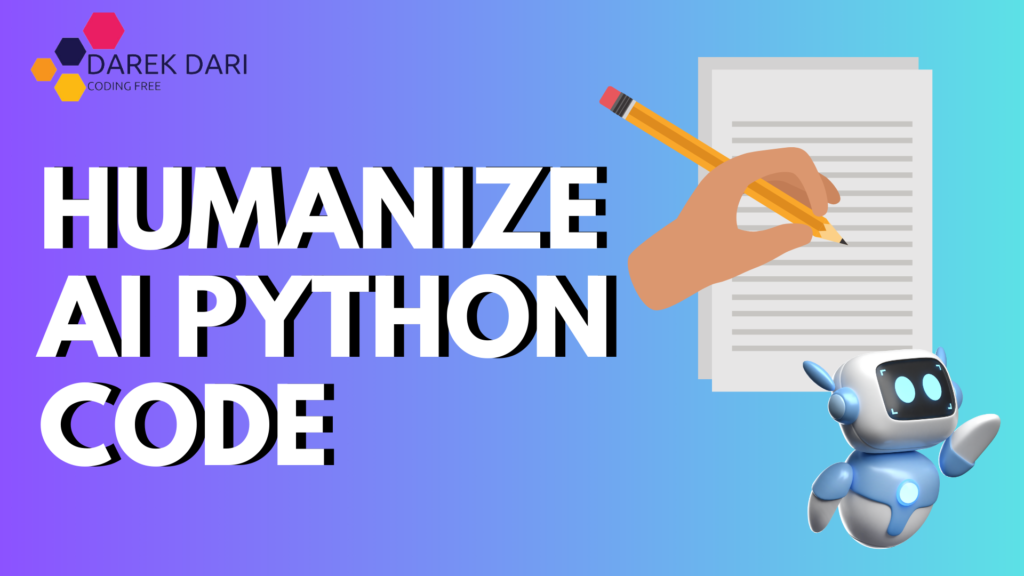
Table of Contents
Introduction to Humanize AI Python Code
When developing AI systems, one common goal is to make interactions more natural and engaging for users. To achieve this, developers can employ various techniques to Humanize AI Python Code, allowing the AI to respond in a more conversational and empathetic manner.
To “humanize” an AI model in Python and make its responses feel more natural and conversational, you can apply various strategies like adjusting the tone, adding variability, or using advanced NLP libraries.
By incorporating elements like variability in responses, realistic pauses, and sentiment analysis, you can enhance the user experience, making it feel more like interacting with a human rather than a machine. This guide explores different strategies to help you Humanize AI Python Code and create AI-driven solutions that are both effective and personable.
ai humanizer reddit
ai powered performance ads certification answers
ai to human converter free
ai to human text converter free
ai to human text converter reddit
ai to human text free
ai to humanize text converter
ai-powered performance ads certification answers
best beginner python projects
best humanizer tool reddit
Conversational Bot Example
One way to make an AI feel more human is by structuring responses in a friendly and conversational way.
import random
# Sample responses
greetings = ["Hello!", "Hi there!", "Hey, how can I assist you today?", "Greetings!"]
affirmations = ["Absolutely!", "Of course!", "Yes, I'd be happy to help!", "Sure, let's get that done."]
thanks = ["You're welcome!", "No problem at all!", "Happy to help!", "Glad I could assist!"]
def greet_user():
return random.choice(greetings)
def affirm():
return random.choice(affirmations)
def respond_to_thanks():
return random.choice(thanks)
# Conversation simulation
def humanize_interaction(user_input):
if 'hello' in user_input.lower():
return greet_user()
elif 'thank you' in user_input.lower() or 'thanks' in user_input.lower():
return respond_to_thanks()
elif 'can you' in user_input.lower() or 'help' in user_input.lower():
return affirm()
else:
return "I'm not sure I understand, but I'll do my best to help!"
# Simulating a conversation
user_input = "Hello"
print(humanize_interaction(user_input))
user_input = "Can you help me?"
print(humanize_interaction(user_input))
user_input = "Thank you!"
print(humanize_interaction(user_input))
best free humanizer reddit
best ai humanizer reddit
best ai to human text converter reddit
chat gpt humanizer
Using AI Language Models (like GPT-based models)
If you’re using a large language model like GPT, you can control the model’s tone by carefully crafting prompts.
from transformers import pipeline
# Create a conversational pipeline using a pre-trained model
generator = pipeline('text-generation', model='gpt-2')
# Example: prompting the model with a humanized tone
prompt = "You are a helpful assistant. A user asks: Can you help me organize my tasks?"
response = generator(prompt, max_length=100, num_return_sequences=1)
print(response[0]['generated_text'])
Adding Variability
Human conversations are often non-repetitive. You can introduce randomness in responses to make them feel more dynamic.
import random
responses = {
'greetings': ["Hi!", "Hello!", "Hey!", "Greetings!", "Hi there!"],
'affirmations': ["Of course!", "Sure!", "Absolutely!", "I'm happy to help!", "Yes, certainly!"],
'farewell': ["Goodbye!", "See you later!", "Take care!", "Have a great day!"]
}
def humanized_response(category):
return random.choice(responses[category])
print(humanized_response('greetings'))
print(humanized_response('affirmations'))
print(humanized_response('farewell'))
Adding Pauses for Realism
Humans don’t respond instantly, so adding small delays can make interactions feel more natural.
import time
def humanize_with_delay(text, delay=1.5):
time.sleep(delay) # Pause for realism
return text
print(humanize_with_delay("Thinking...", 2))
print(humanize_with_delay("Sure, I can help with that!"))
Emulating Human Error or Imperfection
You can simulate human-like typing delays or slight imperfections to make the AI feel less robotic.
def simulate_typing_delay(text):
for char in text:
print(char, end='', flush=True)
time.sleep(random.uniform(0.05, 0.15))
print()
simulate_typing_delay("Let me think for a moment...")
simulate_typing_delay("Okay, here's what I found!")
Handling Sentiment & Emotions
To respond based on the user’s sentiment, you can use sentiment analysis to detect the tone and adjust the AI’s reply accordingly.
from textblob import TextBlob
def analyze_sentiment(user_input):
blob = TextBlob(user_input)
sentiment = blob.sentiment.polarity
if sentiment > 0:
return "I'm glad you're feeling positive!"
elif sentiment < 0:
return "I'm sorry you're feeling down. Let me help!"
else:
return "I'm here to assist however you feel."
user_input = "I'm really upset about this!"
print(analyze_sentiment(user_input))
By combining these techniques, you can make AI interactions feel more human-like, fostering better engagement and understanding.
Conclusion
By applying techniques such as using conversational tones, adding variability to responses, simulating delays, and leveraging sentiment analysis, you can Humanize AI Python Code to make interactions feel more natural and human-like.
Whether using basic Python logic or advanced language models, the key is to introduce variability, empathy, and responsiveness. This approach will help Humanize AI Python Code, creating a more engaging and personable experience for users. Ultimately, with these strategies, your AI can feel less robotic and more like a helpful assistant, effectively helping you Humanize AI Python Code for more authentic user interactions.
0 Comments