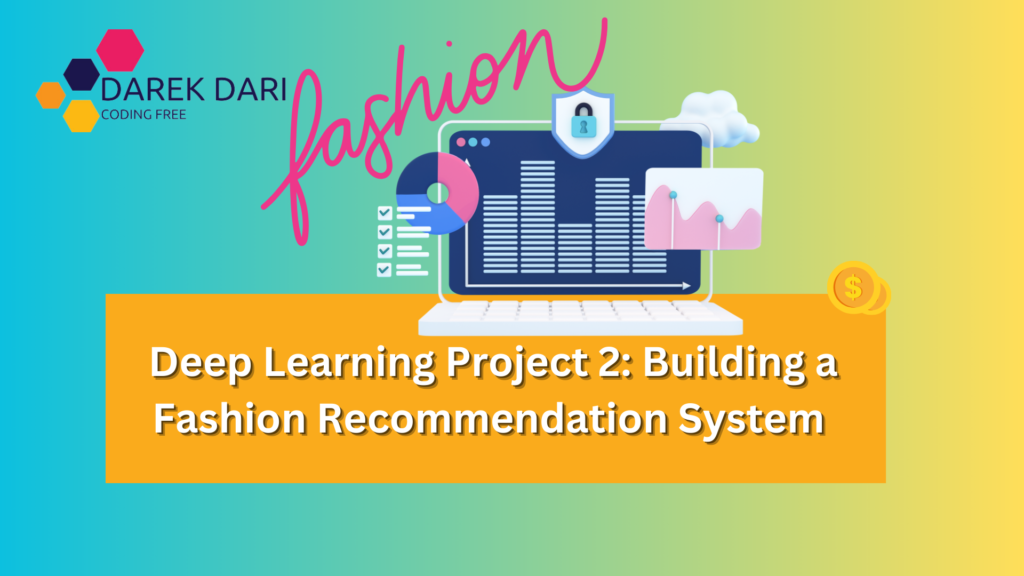
Table of Contents
Introduction to Fashion Recommendation System
In this blog, we’ll walk through the process of building a fashion recommendation system using a Convolutional Neural Network (CNN).
You can also check:
- Deep Learning Project 1: Real and AI-Generated Synthetic Images
- Deep Learning Project 2: Building a Fashion Recommendation System
Specifically, we’ll use the VGG16 model pre-trained on ImageNet to extract features from fashion images and recommend similar items based on those features. Here’s a step-by-step guide on how to achieve this.
What is the aim of the fashion recommendation system?
Fashion recommendation systems strive to offer personalized fashion suggestions to users by considering their preferences, past behavior, and current trends.
These systems analyze user data and fashion trends to assist users in finding new clothing items, accessories, and styles that align with their taste and requirements.
Ultimately, this enhances their shopping experience and boosts sales for retailers.
Detailed Breakdown of the Aim
Objective | Description | Example |
---|---|---|
Personalization | Customize recommendations based on individual user data, including past behavior and preferences. | Suggesting a user who likes casual wear a new line of comfortable jeans and t-shirts. |
Increase Engagement | Maintain user interest by continuously offering items they are likely to find appealing. | Showing new arrivals in the user’s favorite category (e.g., athletic wear) regularly. |
Boost Sales | Enhance sales by targeting recommendations to user preferences, increasing the likelihood of purchase. | Recommending accessories that complement a dress a user has shown interest in. |
Inventory Management | Promote products that need to be sold to balance stock levels, reducing overstock or understock situations. | Highlighting winter coats on sale as the season ends to clear out stock. |
Trend Awareness | Keep users informed about the latest fashion trends and new products. | Featuring a trending new sneaker line prominently for users interested in footwear. |
Customer Retention | Improve customer loyalty by providing a consistently satisfying shopping experience. | Regularly suggesting items that fit the user’s evolving style based on recent purchases. |
Cross-Selling and Up-Selling | Recommend related or higher-end products to enhance the shopping cart value. | Suggesting a designer handbag to go with the high-end dress a user is considering. |
Data Utilization | Continuously refine recommendation algorithms using collected data and user feedback. | Using machine learning to improve accuracy of suggestions based on user interaction data. |
User Trust and Loyalty | Build a trustworthy relationship with users by delivering consistent, high-quality, and relevant recommendations. | Offering consistent quality in recommendations that meet user expectations and preferences. |
Focusing on these goals can greatly improve the user experience, boost sales, and foster lasting customer loyalty in a fashion recommendation system.
Step 1: Assemble a Diverse Dataset
First, we need a dataset of fashion items. For this example, we have a collection of women’s fashion images stored in a directory. Let’s list the files to see a preview of our dataset.
import os
directory_path = '/kaggle/input/womens-fashion-image-dataset/women fashion'
files = os.listdir(directory_path)
print(files[:10])
Output:
['long, intricately designed dress with full sleeves.jpg',
'royal blue off-shoulder dress.jpg',
'bright red, form-fitting, strapless dress with a high slit on one side revealing part of the leg.jpg',
'high-waisted white trousers paired with a structured, corset-style bodice featuring mesh.jpg',
'classic black slip dress with a midi length.jpg',
'anarkali suit with lavender in color with intricate white patterns throughout the fabric.jpg',
'anarkali suit. It consists of a turquoise skirt with detailed golden embroidery, a multicolored blouse with floral patterns, and an orange dupatta with lace borders.jpg',
'tight-fitting, off-the-shoulder white dress.webp',
'red dress with a pattern of small white flowers.jpg',
'strapless, sequined dress that sparkles with multiple colors.jpg']
Step 2: Display an Image
To understand the characteristics of our dataset, let’s load and display an image using the PIL library.
from PIL import Image
import matplotlib.pyplot as plt
# Function to load and display an image
def display_image(file_path):
image = Image.open(file_path)
plt.imshow(image)
plt.axis('off')
plt.show()
# Display the first image to understand its characteristics
first_image_path = os.path.join(directory_path, files[-2])
display_image(first_image_path)
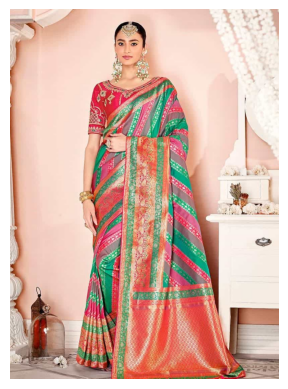
Step 3: Preprocess Images
We need to ensure that all images are in a consistent format (JPEG, PNG) and resolution. We’ll load the images, resize them to 224×224 pixels, and preprocess them to fit the input requirements of the VGG16 model.
import glob
import os
# Directory path containing your images
image_directory = '/kaggle/input/womens-fashion-image-dataset/women fashion'
# List all image files with the specified extensions
image_paths_list = [file for file in glob.glob(os.path.join(image_directory, '*.*')) if file.endswith(('.jpg', '.png', '.jpeg', '.webp'))]
# Print the list of image file paths
print(image_paths_list[:10])
Step 4: Load the Pre-trained VGG16 Model
We’ll use the VGG16 model pre-trained on the ImageNet dataset. This model is powerful for feature extraction from images.
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.vgg16 import VGG16, preprocess_input
from tensorflow.keras.models import Model
import numpy as np
# Load the VGG16 model pre-trained on ImageNet
base_model = VGG16(weights='imagenet', include_top=False)
model = Model(inputs=base_model.input, outputs=base_model.output)
Step 5: Extract Features from Images
We preprocess each image, pass it through the VGG16 model, and extract features. These features will be used to compare and recommend similar items.
def preprocess_image(img_path):
img = image.load_img(img_path, target_size=(224, 224))
img_array = image.img_to_array(img)
img_array_expanded = np.expand_dims(img_array, axis=0)
return preprocess_input(img_array_expanded)
def extract_features(model, preprocessed_img):
features = model.predict(preprocessed_img)
flattened_features = features.flatten()
normalized_features = flattened_features / np.linalg.norm(flattened_features)
return normalized_features
all_features = []
all_image_names = []
for img_path in image_paths_list:
preprocessed_img = preprocess_image(img_path)
features = extract_features(model, preprocessed_img)
all_features.append(features)
all_image_names.append(os.path.basename(img_path))
Step 6: Measure Similarity and Recommend Items
We define a metric for measuring the similarity between feature vectors. Here, we use cosine similarity. Based on this metric, we rank the dataset images and recommend the top N items that are most similar to the input image.
from scipy.spatial import distance
def recommend_similar_items(feature_vectors, input_feature_vector, top_n=5):
similarities = [1 - distance.cosine(input_feature_vector, fv) for fv in feature_vectors]
sorted_indices = np.argsort(similarities)[::-1]
return sorted_indices[:top_n]
# Example: Recommend top 5 items similar to the first image in the dataset
input_feature_vector = all_features[0]
recommended_indices = recommend_similar_items(all_features, input_feature_vector)
print("Recommended items:")
for index in recommended_indices:
print(all_image_names[index])
Output:
Recommended items:
bright red, form-fitting, strapless dress with a high slit on one side revealing part of the leg.jpg
bright red, sequined dress with thin shoulder straps.jpg
long, flowing, pink dress with a sparkly texture.jpg
strapless red midi dress with a mermaid silhouette.jpg
vibrant blue and a neutral tone adorned with colorful floral patterns.jpg
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 282ms/step

What is Fashion Recommendation System?
Fashion recommendation systems use machine learning or artificial intelligence to suggest clothing items to users based on factors like user preferences, browsing history, purchase history, and visual similarity.
The goal is to improve the shopping experience by providing personalized recommendations, helping users discover products they’ll love and likely buy.
Key Components of a Fashion Recommendation System
- Data Gathering: The system acquires data from a variety of sources including user interactions (clicks, purchases, likes), product catalogs, and user profiles.
- Feature Extraction: In visual recommendation systems, features are derived from images through methods like Convolutional Neural Networks (CNNs). Textual features can also be obtained from product descriptions.
- Similarity Assessment: The system evaluates the similarity between different items using methods like cosine similarity, Euclidean distance, or more advanced techniques such as neural embeddings.
- Recommendation Techniques: Utilizing the extracted features and similarity measures, the system employs algorithms to suggest items. Common strategies include collaborative filtering, content-based filtering, and hybrid approaches.
- Personalization: Recommendations are customized for individual users by taking into account their unique preferences and behaviors.
Types of Fashion Recommendation Systems
- Content-Based Filtering suggests items that are comparable to the ones the user has previously liked or viewed, focusing on features like visual attributes, text descriptions, or metadata.
- Collaborative Filtering suggests items based on the behavior of users with similar tastes, either by finding users with similar preferences or items that are commonly liked together.
- Hybrid Systems combine content-based and collaborative filtering methods to benefit from the strengths of both approaches.
- Visual Search and Similarity use image recognition and computer vision techniques to recommend visually similar items.
Examples and Applications
- E-commerce Platforms: Online platforms such as Amazon, ASOS, and Zalando utilize recommendation systems to provide product suggestions tailored to user activity and preferences.
- Fashion Retail Apps: Mobile applications frequently employ visual similarity and user preferences to offer outfit, accessory, and other fashion item recommendations.
- Social Media: Platforms like Instagram and Pinterest suggest fashion items and trends by analyzing user interactions and visual content.
What is CNN?
CNN is short for Convolutional Neural Network, a deep learning model created for handling structured grid data like images.
CNNs excel at tasks such as image recognition, image classification, and object detection by capturing spatial hierarchies within images.
Key Components of CNN
Component | Description |
---|---|
Convolutional Layers | Apply filters to detect features like edges, textures, or shapes in the image. |
Pooling Layers | Down-sample the feature maps to reduce dimensions and computation load. |
Fully Connected Layers | Perform high-level reasoning by combining detected features to make final predictions. |
Activation Functions | Introduce non-linearity to enable the network to learn complex patterns. |
How CNN Works?
In a CNN, the input is typically a raw image represented as a matrix of pixel values. For color images, this matrix consists of three channels – red, green, and blue.
The input image is processed using small filters (kernels) of sizes like 3×3 or 5×5. These filters slide over the image, performing element-wise multiplications and summing up the results to generate feature maps.
Following the convolution operation, an activation function (such as ReLU) is applied to introduce non-linearity.
Pooling layers help reduce the spatial dimensions of the feature maps. For example, a 2×2 Max Pooling operation selects the maximum value from each 2×2 block, effectively reducing the size of the feature map by a factor of 2.
After going through multiple convolutional and pooling layers, the feature maps are flattened into a single vector.
The flattened vector is then passed through one or more fully connected layers. These layers perform the final classification or regression tasks.
The last layer produces the output, which can be class probabilities (for classification tasks) or other predicted values.
Example of a CNN Architecture
Consider the architecture of a simple CNN designed for image classification:
Layer | Description | Output Shape |
---|---|---|
Input Layer | 64x64x3 image (for a 64×64 RGB image) | 64x64x3 |
Convolutional Layer 1 | 32 filters of size 3×3, stride 1, padding same | 64x64x32 |
Activation Function | ReLU | 64x64x32 |
Pooling Layer 1 | Max Pooling with 2×2 window | 32x32x32 |
Convolutional Layer 2 | 64 filters of size 3×3, stride 1, padding same | 32x32x64 |
Activation Function | ReLU | 32x32x64 |
Pooling Layer 2 | Max Pooling with 2×2 window | 16x16x64 |
Flattening Layer | Flattening the feature maps | 16384 (16x16x64) |
Fully Connected Layer 1 | 128 neurons | 128 |
Activation Function | ReLU | 128 |
Fully Connected Layer 2 | 10 neurons (for 10 classes) | 10 |
Activation Function | Softmax (for classification) | 10 |
Example of Fashion Recommendation System
To illustrate, let’s build a basic fashion recommendation system using a pre-trained Convolutional Neural Network (CNN) model like VGG16 to extract features from fashion images and recommend similar items.
- Dataset: Collect a dataset of fashion images, ensuring a variety of styles and categories.
- Preprocess Images: Resize images to a consistent format and resolution (e.g., 224×224 pixels for VGG16).
- Load Pre-trained Model: Use a model like VGG16 pre-trained on ImageNet to extract deep features from the images.
- Extract Features: Pass the preprocessed images through the model to obtain feature vectors.
- Measure Similarity: Use a metric like cosine similarity to measure the similarity between feature vectors.
- Recommend Items: Based on the similarity scores, recommend the top N most similar items.
Here’s a simplified Python implementation to demonstrate these steps:
import os
from PIL import Image
import numpy as np
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.vgg16 import VGG16, preprocess_input
from tensorflow.keras.models import Model
from scipy.spatial import distance
import matplotlib.pyplot as plt
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet', include_top=False)
model = Model(inputs=base_model.input, outputs=base_model.output)
# Function to preprocess and extract features from an image
def preprocess_and_extract_features(img_path):
img = image.load_img(img_path, target_size=(224, 224))
img_array = image.img_to_array(img)
img_array_expanded = np.expand_dims(img_array, axis=0)
img_preprocessed = preprocess_input(img_array_expanded)
features = model.predict(img_preprocessed)
return features.flatten() / np.linalg.norm(features.flatten())
# Directory containing fashion images
image_dir = '/path/to/fashion/images'
image_paths = [os.path.join(image_dir, fname) for fname in os.listdir(image_dir)]
# Extract features for all images
features_list = [preprocess_and_extract_features(img_path) for img_path in image_paths]
# Function to recommend similar items
def recommend_similar_items(input_features, all_features, top_n=5):
similarities = [1 - distance.cosine(input_features, features) for features in all_features]
return np.argsort(similarities)[::-1][:top_n]
# Example usage
input_img_path = image_paths[0]
input_features = preprocess_and_extract_features(input_img_path)
recommended_indices = recommend_similar_items(input_features, features_list)
# Display recommended images
for idx in recommended_indices:
plt.imshow(Image.open(image_paths[idx]))
plt.axis('off')
plt.show()
In this instance, VGG16 is employed to extract features from images and cosine similarity is utilized to suggest similar items.
By incorporating advanced models, extra data sources, and personalized algorithms, this simple method can be upgraded to develop a strong fashion recommendation system.
What is the smart clothing recommendation system?
An innovative Smart Clothing Recommendation System utilizes cutting-edge technologies such as artificial intelligence (AI), machine learning (ML), and computer vision to offer tailored fashion tips and outfit ideas.
This system aims to improve user satisfaction by suggesting apparel that aligns with the user’s taste, body shape, and the latest fashion trends. Let’s delve deeper into this concept with detailed insights and practical examples.
Benefits of the fashion recommendation system
Objective | Description | Example |
---|---|---|
Personalized Shopping Experience | Tailor recommendations to individual user preferences, enhancing the shopping experience. | Suggesting a curated selection of outfits based on a user’s style profile and past purchases. |
Increased Sales | Boost sales by providing relevant product suggestions that users are more likely to purchase. | Recommending accessories that complement a dress a user has added to their cart. |
Customer Retention | Enhance customer loyalty by consistently offering relevant and high-quality recommendations. | Sending personalized style boxes to subscribers, keeping them engaged with new fashion items. |
Trend Adaptation | Keep users informed about the latest fashion trends, encouraging timely purchases. | Featuring the latest trends in streetwear for users interested in that style. |
Inventory Management | Help retailers manage inventory by promoting items that need to be sold. | Highlighting end-of-season sales to clear out remaining stock efficiently. |
The Smart Clothing Recommendation System is a game-changer in the fashion retail sector, utilizing AI, machine learning, and computer vision to provide a tailored and interactive shopping journey.
Through analyzing user preferences and utilizing advanced algorithms, these systems boost customer satisfaction, increase sales, and optimize inventory management for retailers.
What is an AI driven fashion recommender system?
Fashion recommendation systems rely on a range of tools and frameworks to provide personalized suggestions and improve the shopping experience.
Deep learning frameworks like TensorFlow and Keras are commonly used for tasks such as image recognition, which is essential for analyzing fashion items.
PyTorch, another deep learning framework, is preferred for its flexibility and user-friendly nature, particularly in research settings.
When it comes to traditional machine learning tasks like collaborative filtering and content-based filtering, developers often rely on Scikit-Learn, a widely used Python library.
This library offers efficient implementations of various algorithms for data preprocessing, modeling, and evaluation.
Computer vision plays a vital role in fashion recommendation systems by enabling the analysis of images to identify clothing items, extract features, and provide virtual try-on experiences.
OpenCV, a powerful library for image processing and computer vision tasks, is commonly used in this context.
NLP plays a crucial role in comprehending user reviews, product descriptions, and other textual data. Prominent NLP libraries like NLTK (Natural Language Toolkit) and SpaCy aid in tasks such as text tokenization, part-of-speech tagging, and named entity recognition.
Task | Tools and Frameworks |
---|---|
Deep Learning | TensorFlow, Keras, PyTorch |
Machine Learning | Scikit-Learn |
Computer Vision | OpenCV |
Natural Language Processing | NLTK, SpaCy |
These libraries empower developers to build advanced fashion recommender systems that provide personalized recommendations based on individual preferences and style.
Conclusion
In our blog, we’ve developed a fashion recommendation system by utilizing a pre-trained VGG16 model for feature extraction.
We’ve covered loading and preprocessing images, extracting features, and utilizing these features to suggest similar items. This method harnesses the capabilities of deep learning to establish a reliable recommendation system for fashion items.
To improve this system further, you can delve into more advanced models, fine-tune the pre-trained model with a fashion-specific dataset, or include extra features like textual descriptions and user preferences.
2 Comments
Deep Learning Project 1: Best Generated Synthetic Images · June 5, 2024 at 11:37 am
[…] Deep Learning Project 2: Building a Fashion Recommendation System […]
Best Dropout And Strides Models Deep Learning Exercise 1 · June 5, 2024 at 12:11 pm
[…] Deep Learning Project 2: Building a Fashion Recommendation System […]