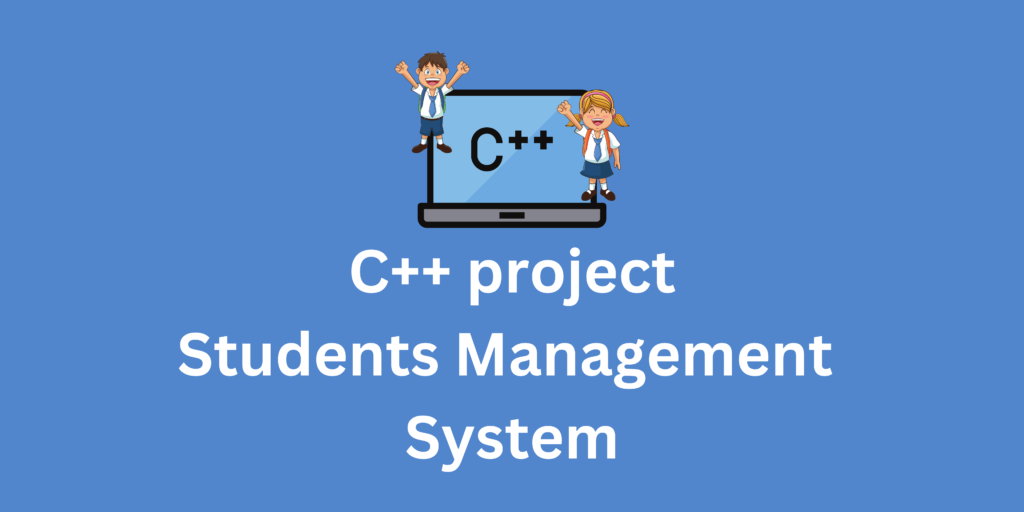
Create a student management system to store and manage student information in a school or educational institution. The system should allow users to add students, see student details, search for students, and execute basic activities such as updating and deleting student records.
Requirements
- The system should have a user interface where users can interact with the program.
- Users should be able to add new students by providing details such as student ID, name, age, grade, and contact information.
- Users should be able to display a list of all students in the system, including their details.
- The system should allow users to search for students based on various criteria, such as student ID, name, or grade.
- Users should be able to update student information, such as their contact details or grade.
- The system should provide the option to delete a student record from the system.
- Implement basic input v
Code
#include <iostream>
#include <vector>
#include <string>
class Student {
private:
std::string studentID;
std::string name;
int age;
int grade;
std::string contact;
public:
Student(const std::string& studentID, const std::string& name, int age, int grade, const std::string& contact)
: studentID(studentID), name(name), age(age), grade(grade), contact(contact) {}
std::string getStudentID() const {
return studentID;
}
std::string getName() const {
return name;
}
int getAge() const {
return age;
}
int getGrade() const {
return grade;
}
std::string getContact() const {
return contact;
}
void displayDetails() const {
std::cout << "Student ID: " << studentID << std::endl;
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
std::cout << "Grade: " << grade << std::endl;
std::cout << "Contact: " << contact << std::endl;
}
};
class StudentManagementSystem {
private:
std::vector<Student> students;
public:
void addStudent(const Student& student) {
students.push_back(student);
}
void displayAllStudents() const {
std::cout << "Student List:" << std::endl;
for (const auto& student : students) {
std::cout << "---------------------" << std::endl;
student.displayDetails();
std::cout << "---------------------" << std::endl;
}
}
Student* findStudentByID(const std::string& studentID) {
for (auto& student : students) {
if (student.getStudentID() == studentID) {
return &student;
}
}
return nullptr;
}
void updateStudent(Student* student) {
std::cout << "Enter updated contact details: ";
std::cin.ignore();
std::getline(std::cin, student->getContact());
std::cout << "Student details updated successfully!" << std::endl;
}
void deleteStudent(Student* student) {
students.erase(std::remove(students.begin(), students.end(), *student), students.end());
std::cout << "Student record deleted successfully!" << std::endl;
}
};
int main() {
StudentManagementSystem sms;
Student student1("001", "John Doe", 18, 12, "john.doe@example.com");
Student student2("002", "Jane Smith", 17, 11, "jane.smith@example.com");
Student student3("003", "Robert Johnson", 16, 10, "robert.johnson@example.com");
sms.addStudent(student1);
sms.addStudent(student2);
sms.addStudent(student3);
sms.displayAllStudents();
std::string studentID;
std::cout << "Enter the student ID of the student you want to update: ";
std::cin >> studentID;
Student* foundStudent = sms.findStudentByID(studentID);
if (foundStudent != nullptr) {
sms.updateStudent(foundStudent);
} else {
std::cout << "Student not found in the system." << std::endl;
}
std::cout << "Enter the student ID of the student you want to delete: ";
std::cin >> studentID;
foundStudent = sms.findStudentByID(studentID);
if (foundStudent != nullptr) {
sms.deleteStudent(foundStudent);
} else {
std::cout << "Student not found in the system." << std::endl;
}
return 0;
}
Optional Enhancements
- Add a feature to calculate and display statistics, such as the average age or grade of all students.
- Implement a feature to generate reports, such as a list of students in a specific grade or with a specific age range.
- Include a feature to sort the student records based on different criteria, such as student ID or name.
- Implement a user authentication system to differentiate between administrators and regular users, allowing administrators to perform administrative tasks such as adding or deleting student records.
1 Comment
código de indicac~ao da binance · April 15, 2024 at 11:34 pm
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.