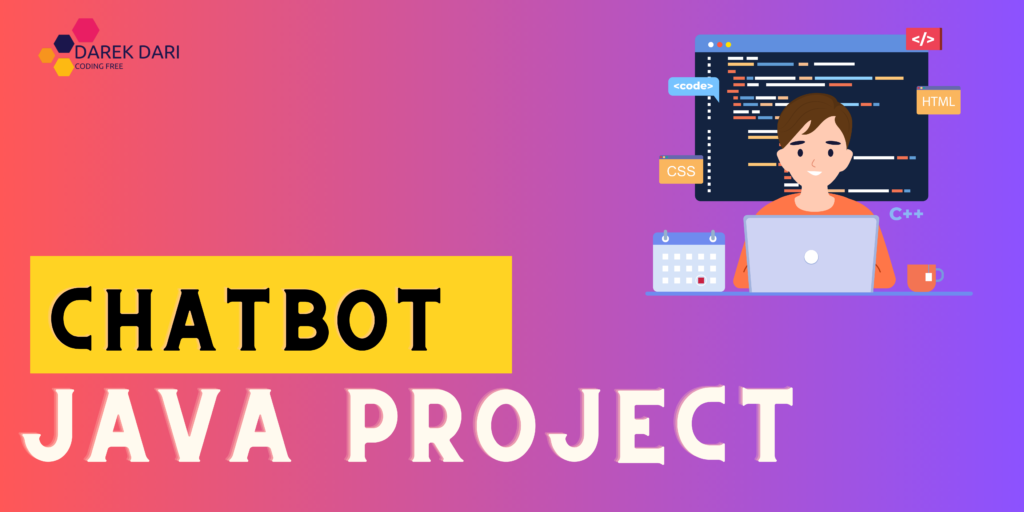
Table of Contents
Hey there, fellow explorer! Today, we’re delving into the fascinating world of chatbots and learning how to create one using Java. This journey promises to be both enlightening and enjoyable. By the end of this guide, you’ll have your very own chatbot, ready to engage in conversations with you.
Understanding Chatbot in Java
First and foremost, let’s ensure we’re on the same page regarding what chatbots are. A chatbot is essentially a computer program designed to engage in conversations with users, emulating the interaction you might have with a virtual friend. This versatility makes chatbots valuable for a wide range of applications.
Why Choose Java?
For this exciting venture, we’re opting for Java, a widely-used programming language renowned for its simplicity and flexibility. If you’re new to Java, fear not – we’ll break it down into easy-to-follow steps.
Setting the Stage for Chatbot in Java
To create our chatbot, we’ll start by coding a Java class aptly named “Chatbot.” This class serves as the heart of our chatbot’s functionality. Let’s dissect the code step by step:
import java.util.Scanner;
public class Chatbot {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Hello! I'm your chatbot. What's your name?");
String userName = scanner.nextLine();
System.out.println("Nice to meet you, " + userName + "! How can I assist you today?");
while (true) {
System.out.print(userName + ": ");
String userMessage = scanner.nextLine();
if (userMessage.equalsIgnoreCase("bye")) {
System.out.println("Chatbot: Goodbye, " + userName + "! Have a great day!");
break;
} else {
String chatbotResponse = generateResponse(userMessage);
System.out.println("Chatbot: " + chatbotResponse);
}
}
}
public static String generateResponse(String userMessage) {
return "I'm just a basic chatbot. I don't understand complex questions.";
}
}
Explain the code
- We start by importing the
Scanner
class, which allows us to take user input from the console. - Inside the
main
method, we create aScanner
object namedscanner
to read user input. - We greet the user with a welcoming message and ask for their name. The user’s name is stored in the
userName
variable. - After greeting the user, we enter a loop using
while (true)
. This loop continues until the user types “bye.” - Inside the loop, we prompt the user for input using
System.out.print(userName + ": ")
to show the user’s name as a prefix to their input. - We read the user’s message using
scanner.nextLine()
and store it in theuserMessage
variable. - We check if the user’s message is “bye” (case-insensitive), and if so, we print a goodbye message and exit the loop.
- If the user’s message is not “bye,” we call the
generateResponse
method, passing the user’s message as an argument. This method is responsible for generating the chatbot’s response. - The chatbot’s response is then printed, prefixed with “Chatbot:.”
- The
generateResponse
method, as defined in the code, always returns the same response: “I’m just a basic chatbot. I don’t understand complex questions.”
In summary, this code creates a simple chatbot that engages in a conversation with the user, responding to their input. The chatbot continues to chat until the user types “bye,” at which point the chatbot bids the user farewell. Feel free to customize the generateResponse
method to make the chatbot respond to a variety of user messages.
FAQ
Have questions? Here are some frequently asked questions:
Q1: What is a chatbot? A chatbot is a computer program designed to engage in conversations with users, simulating human interaction.
Q2: Why choose Java for creating a chatbot? Java is chosen for its simplicity and flexibility, making it a suitable language for chatbot development.
Q3: How can I customize my chatbot’s responses? You can customize responses by editing the generateResponse
method, incorporating if statements, connecting to external services, or generating random responses.
Q4: Are there limitations to what a chatbot can do? Chatbots can be as simple or as complex as you design them. Their capabilities depend on your programming and integration with external services.
Q5: Can I integrate my chatbot with other applications or services? A: Yes, chatbots can be integrated with various applications and services through APIs, allowing them to perform a wide range of tasks.
Q6: What are the potential applications for chatbots? Chatbots are used in customer service, virtual assistants, e-commerce, healthcare, and many other fields where automated interactions are beneficial.
Feel free to ask more questions and explore the world of chatbot development further.
Happy coding! 🤖🚀
2 Comments
Best Medium E-commerce Platform Java Source Code 2024 · May 20, 2024 at 9:32 pm
[…] Chatbot: Let’s explore the captivating realm of chatbots and discover the art of crafting one using Java. This adventure guarantees to be both enlightening and pleasurable. Once you complete this guide, you’ll have your very own chatbot, all set to engage in delightful conversations with you. […]
Best Medium Morse Code Translator Java Source Code 2024 · May 20, 2024 at 10:01 pm
[…] Chatbot: Let’s explore the captivating realm of chatbots and discover the art of crafting one using Java. This adventure guarantees to be both enlightening and pleasurable. Once you complete this guide, you’ll have your very own chatbot, all set to engage in delightful conversations with you. […]