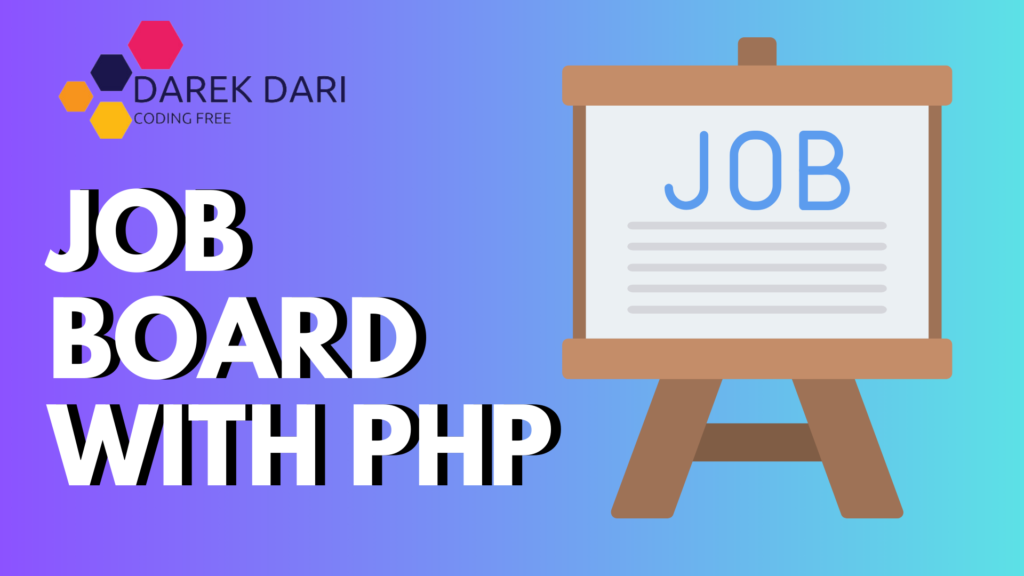
Table of Contents
Introduction to Job Board with PHP
Hey there, tech enthusiasts! đ If you’ve ever dreamed of building your own job board but didnât know where to start, you’re in the right place.
PHP Projects
- Quiz Application in PHP
- PHP Image Gallery Project Source Code
- Best 200 Questions Game in PHP
- Bulletin Board PHP Project
- Content Management System (CMS) PhP Project
- Event Management System Project in PHP
- Personal Blog with PHP
- Chatbot in PHP
- URL Shortenee in php
- Creating Best PHP MVC Framework from Scratch
- Recipe Sharing Platform with PHP
- Job Board with PHP
Today, weâll walk through creating a basic job board using PHP and MySQL. By the end, youâll have a functioning site where users can view job listings and apply for jobs. Letâs dive in!
php exercises
php lab exercises
php mvc folder structure best practices
php mvc framework from scratch
php mvc from scratch
php mvc project
php photo database
php photo gallery
php practice exercises
php project source code
php projects source code
php projects with source code github
php realtime chat
Let’s Start Coding
Step 1: Set Up Your Database
First things first, we need to create a database to store our job listings and applications. Letâs get our hands dirty with some SQL!
- Create Your Database
Open your MySQL management tool (like phpMyAdmin) and create a new database. Weâll call itjob_board
. Simple enough, right? - Create Tables
Next, weâll set up two tables: one for job listings and one for applications. Hereâs the SQL youâll need:
USE job_board;
CREATE TABLE jobs (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
description TEXT NOT NULL,
company VARCHAR(255) NOT NULL,
location VARCHAR(255),
date_posted TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE applications (
id INT AUTO_INCREMENT PRIMARY KEY,
job_id INT,
applicant_name VARCHAR(255),
applicant_email VARCHAR(255),
cover_letter TEXT,
date_applied TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (job_id) REFERENCES jobs(id)
);
This sets up our jobs
table to store job details and an applications
table to track who applied for each job.
Step 2: Create Your PHP Files
Now, letâs build out the functionality with PHP. Weâll create three main files: one to connect to the database, one to display jobs, and one to handle job applications.
2.1. Database Connection (db.php
)
Create a file called db.php
to handle our database connection. This file will be our gateway to the database.
<?php
$host = 'localhost'; // Your database host
$db = 'job_board'; // The database name we created
$user = 'root'; // Your database username
$pass = ''; // Your database password
// Create connection
$conn = new mysqli($host, $user, $pass, $db);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
2.2. Display Jobs (index.php
)
Now letâs create index.php
, which will display our job listings. This page will pull job data from the database and show it to visitors.
<?php
include 'db.php';
// Fetch jobs
$sql = "SELECT * FROM jobs ORDER BY date_posted DESC";
$result = $conn->query($sql);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Job Board</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Welcome to the Job Board!</h1>
<ul>
<?php
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "<li>";
echo "<h2>" . $row['title'] . "</h2>";
echo "<p>" . $row['description'] . "</p>";
echo "<p><strong>Company:</strong> " . $row['company'] . "</p>";
echo "<p><strong>Location:</strong> " . $row['location'] . "</p>";
echo "<a href='apply.php?job_id=" . $row['id'] . "'>Apply Now</a>";
echo "</li>";
}
} else {
echo "<li>No jobs available right now.</li>";
}
?>
</ul>
</body>
</html>
<?php
$conn->close();
?>
Explanation:
- This code connects to the database and fetches job listings.
- It then displays these jobs in a simple, clean list format.
- Each job has an “Apply Now” link that directs users to the application page.
2.3. Apply for a Job (apply.php
)
Finally, we need a way for users to apply for jobs. Create a file called apply.php
for the application process.
<?php
include 'db.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$job_id = $_POST['job_id'];
$applicant_name = $_POST['applicant_name'];
$applicant_email = $_POST['applicant_email'];
$cover_letter = $_POST['cover_letter'];
// Insert application
$sql = "INSERT INTO applications (job_id, applicant_name, applicant_email, cover_letter) VALUES (?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("isss", $job_id, $applicant_name, $applicant_email, $cover_letter);
if ($stmt->execute()) {
echo "Thank you for applying!";
} else {
echo "Oops! Something went wrong: " . $stmt->error;
}
$stmt->close();
$conn->close();
} else {
$job_id = $_GET['job_id'];
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Apply for Job</title>
</head>
<body>
<h1>Apply for This Job</h1>
<form action="apply.php" method="POST">
<input type="hidden" name="job_id" value="<?php echo htmlspecialchars($job_id); ?>">
<label for="applicant_name">Your Name:</label>
<input type="text" id="applicant_name" name="applicant_name" required><br>
<label for="applicant_email">Your Email:</label>
<input type="email" id="applicant_email" name="applicant_email" required><br>
<label for="cover_letter">Cover Letter:</label>
<textarea id="cover_letter" name="cover_letter" required></textarea><br>
<input type="submit" value="Submit Application">
</form>
</body>
</html>
<?php
}
?>
Explanation:
- When the form is submitted, it sends the application data to the database.
- If the form is accessed directly (without submission), it shows the application form with fields for name, email, and cover letter.
- The job ID is passed as a hidden field to link the application to the specific job.
list of job boards
job board examples
example of job boards
examples of job boards
job board example
php mvc project source code
php photo database
php photo gallery
php project source code
php projects source code
php projects with source code
php real time application
php real time chat application
php realtime chat
Step 3: Add Some Style (styles.css
)
To make your job board look good, weâll add some basic styling. Create a file called styles.css
for this.
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
text-align: center;
}
ul {
list-style-type: none;
padding: 0;
}
li {
border: 1px solid #ddd;
padding: 10px;
margin-bottom: 10px;
}
h2 {
margin: 0;
}
a {
display: inline-block;
margin-top: 10px;
color: #007BFF;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
form {
max-width: 600px;
margin: auto;
}
label {
display: block;
margin-top: 10px;
}
input, textarea {
width: 100%;
padding: 8px;
margin-top: 5px;
box-sizing: border-box;
}
input[type="submit"] {
margin-top: 10px;
background-color: #007BFF;
color: white;
border: none;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #0056b3;
}
Explanation
- This CSS file gives a clean and modern look to our job board.
- It styles the job listings and application form for a better user experience.
How to get a job in PHP?
Are you excited to jump into the PHP world and land that dream developer job? Great decision! Letâs simplify it into enjoyable, manageable steps.
Start by getting familiar with PHP basics. Master the fundamentals such as syntax, loops, and functions. Once you feel comfortable, explore advanced topics like object-oriented programming and database manipulation. Experiment with popular PHP frameworks like Laravel or Symfonyâtheyâll be your trusty sidekicks!
Next, apply your skills by creating something youâre proud ofâwhether itâs a personal website, a blog, or a small e-commerce site. Contributing to open source projects is like being part of a supportive tech community. Consider freelancing or interning to gain real-world experience.
Now, enhance your professional profile. Develop a standout resume highlighting your skills and projects, and showcase your work in a portfolio. Donât forget to update your LinkedIn profileâitâs your online tech resume for networking and making connections.
jobs php
jobs in php
php jobs
jobs for php
php job
job for php
job for php developer
photo gallery php
php exercises
php exercises and solutions
php exercises and solutions pdf
php exercises online
php lab exercises
php mvc framework from scratch
php mvc from scratch
When itâs time to apply for jobs, check out job boards and company websites. Prepare for interviews by practicing common questions and solving coding challenges. Customize your resume and cover letter for each position to make a strong impression.
Stay updated on the latest trends in the tech world by following PHP updates and engaging with tech communities. Keep learning new skills and consider getting certified to boost your resume.
With these tips, youâre ready to excel in the PHP industry. Best of luck on your job search! đ If you need more guidance or just want to chat, feel free to reach out!
PHP Job Board Code Template
PHP Job Board Code on GitHub
Free PHP Job Board Code
Example PHP Job Board Code
Free Download of Job Portal PHP Script
Job Board Script
PHP Source Code for Job Portals
Job Portal - Laravel Job Board Script
Wrapping Up
Congratulations! đ Youâve just built a basic job board using PHP and MySQL. Now, you have a functional site where users can browse job listings and apply for jobs.
Feel free to expand on this foundation with more features like job categories, user authentication, and advanced search capabilities. Keep experimenting and happy coding!
Got questions or need further help? Drop a comment below, and Iâll be happy to assist. đ
job in php developer
job php developer
jobs for php developer
php careers
0 Comments