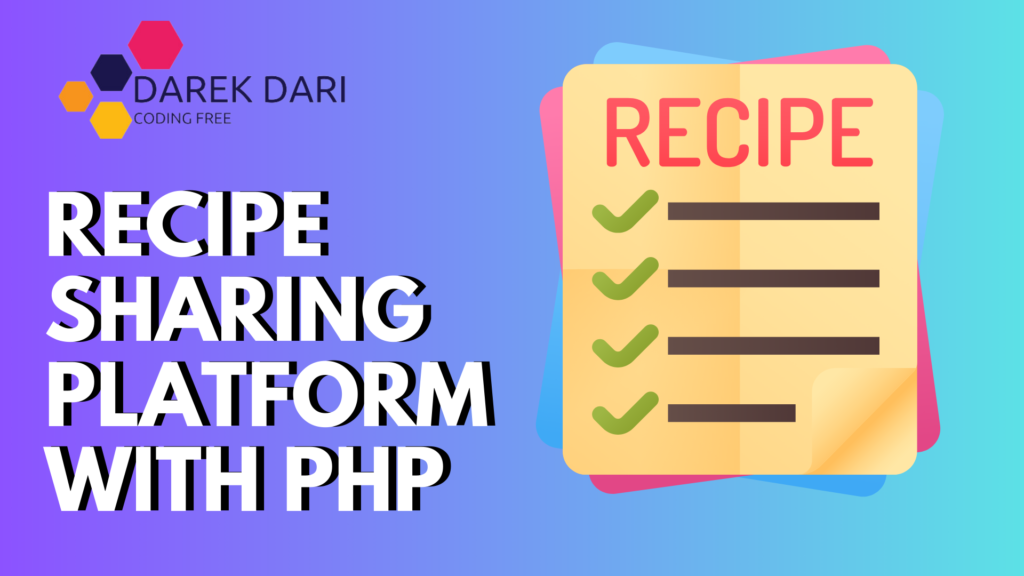
Table of Contents
Introduction To Recipe Sharing Platform
Welcome to the world of coding your very own recipe sharing platform! Imagine a site where food enthusiasts can share their favorite recipes, rate them, and leave comments.
PHP Projects
- Quiz Application in PHP
- PHP Image Gallery Project Source Code
- Best 200 Questions Game in PHP
- Bulletin Board PHP Project
- Content Management System (CMS) PhP Project
- Event Management System Project in PHP
- Personal Blog with PHP
- Chatbot in PHP
- URL Shortenee in php
- Creating Best PHP MVC Framework from Scratch
- Recipe Sharing Platform with PHP
- Job Board with PHP
Excited? Let’s dive into this tasty project and break it down step-by-step.
Setting the Table: Database Setup
Before we cook up the platform, we need to set up our database. This is where all our recipes, user data, ratings, and comments will be stored. Start by creating a database named recipe_platform
. Inside this database, you’ll need to define several tables to manage different types of data.
First, we have the users
table, which will store information about our platform’s users. Each user will have a unique ID, username, password, and email. The recipes
table will hold the details of the recipes, including the title, ingredients, and instructions, along with a link to the user who posted it.
Next, we need a ratings
table to track how users rate recipes, and a comments
table for users to leave their thoughts and feedback. The SQL script below sets up these tables:
-- Create the database
CREATE DATABASE recipe_platform;
-- Use the database
USE recipe_platform;
-- Users table
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL,
password VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL
);
-- Recipes table
CREATE TABLE recipes (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
ingredients TEXT NOT NULL,
instructions TEXT NOT NULL,
user_id INT,
date_posted TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id)
);
-- Ratings table
CREATE TABLE ratings (
id INT AUTO_INCREMENT PRIMARY KEY,
recipe_id INT,
user_id INT,
rating INT CHECK(rating BETWEEN 1 AND 5),
FOREIGN KEY (recipe_id) REFERENCES recipes(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
-- Comments table
CREATE TABLE comments (
id INT AUTO_INCREMENT PRIMARY KEY,
recipe_id INT,
user_id INT,
comment TEXT,
date_commented TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (recipe_id) REFERENCES recipes(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
This script sets up everything we need to manage users, recipes, ratings, and comments efficiently.
php mvc project source code
php photo database
php photo gallery
php project source code
php projects source code
php projects with source code
php real time application
php real time chat application
php realtime chat
User Registration and Login: Getting Started
Now that we have our database ready, it’s time to create the functionality for user registration and login. This is crucial for personalizing the experience and managing user interactions.
User Registration
To allow new users to sign up, we need a registration form where they can enter their details. When a user registers, their information is saved in the users
table. Here’s how you can set up the register.php
script:
<?php
include 'db.php'; // Include database connection
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = $_POST['username'];
$password = password_hash($_POST['password'], PASSWORD_DEFAULT); // Hash the password
$email = $_POST['email'];
// Insert user into the database
$sql = "INSERT INTO users (username, password, email) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sss", $username, $password, $email);
if ($stmt->execute()) {
echo "Registration successful!";
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Register</title>
</head>
<body>
<h1>Register</h1>
<form action="register.php" method="POST">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br>
<input type="submit" value="Register">
</form>
</body>
</html>
This script hashes the user’s password for security and saves their information into the database.
User Login
Next, let’s create a login form that authenticates users. When users log in, their credentials are checked against the stored data, and if they match, they’re granted access. Here’s how to set up login.php
:
<?php
include 'db.php'; // Include database connection
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = $_POST['username'];
$password = $_POST['password'];
// Fetch user from the database
$sql = "SELECT id, password FROM users WHERE username = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $username);
$stmt->execute();
$stmt->store_result();
if ($stmt->num_rows > 0) {
$stmt->bind_result($user_id, $hashed_password);
$stmt->fetch();
if (password_verify($password, $hashed_password)) {
$_SESSION['user_id'] = $user_id;
echo "Login successful!";
} else {
echo "Invalid password.";
}
} else {
echo "User not found.";
}
$stmt->close();
$conn->close();
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="login.php" method="POST">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required><br>
<input type="submit" value="Login">
</form>
</body>
</html>
This script verifies user credentials and starts a session if the login is successful.
Cooking Up Recipes: Adding and Viewing Recipes
With user registration and login sorted, it’s time to focus on recipe management. Users should be able to add new recipes and view existing ones.
Adding Recipes
To let users share their favorite recipes, create a form where they can enter details about their dish. The add_recipe.php
script handles recipe submissions and stores them in the recipes
table:
<?php
include 'db.php'; // Include database connection
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_SESSION['user_id'])) {
$title = $_POST['title'];
$ingredients = $_POST['ingredients'];
$instructions = $_POST['instructions'];
$user_id = $_SESSION['user_id'];
// Insert recipe into the database
$sql = "INSERT INTO recipes (title, ingredients, instructions, user_id) VALUES (?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sssi", $title, $ingredients, $instructions, $user_id);
if ($stmt->execute()) {
echo "Recipe added successfully!";
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Add Recipe</title>
</head>
<body>
<h1>Add a New Recipe</h1>
<form action="add_recipe.php" method="POST">
<label for="title">Recipe Title:</label>
<input type="text" id="title" name="title" required><br>
<label for="ingredients">Ingredients:</label>
<textarea id="ingredients" name="ingredients" required></textarea><br>
<label for="instructions">Instructions:</label>
<textarea id="instructions" name="instructions" required></textarea><br>
<input type="submit" value="Add Recipe">
</form>
</body>
</html>
This form allows users to submit their recipes, which are then saved in the database.
photo gallery php
php exercises
php exercises and solutions
php exercises and solutions pdf
php exercises online
php lab exercises
php mvc framework from scratch
php mvc from scratch
Viewing Recipes
To let users view recipes, create a page that displays a recipe’s details based on its ID. The view_recipe.php
script fetches and shows the recipe’s title, ingredients, and instructions:
<?php
include 'db.php'; // Include database connection
$recipe_id = $_GET['id'];
// Fetch recipe details
$sql = "SELECT * FROM recipes WHERE id = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("i", $recipe_id);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
$recipe = $result->fetch_assoc();
echo "<h1>" . htmlspecialchars($recipe['title']) . "</h1>";
echo "<h2>Ingredients</h2>";
echo "<p>" . htmlspecialchars($recipe['ingredients']) . "</p>";
echo "<h2>Instructions</h2>";
echo "<p>" . htmlspecialchars($recipe['instructions']) . "</p>";
} else {
echo "Recipe not
found.";
}
$stmt->close();
$conn->close();
?>
This script retrieves and displays the details of a recipe, providing a rich user experience.
Spicing Things Up: Ratings and Comments
Ratings and comments add flavor to our recipe-sharing platform by allowing users to provide feedback and share their thoughts.
Rating Recipes
Allow users to rate recipes from 1 to 5 stars with the rate_recipe.php
script. Here’s how it works:
<?php
include 'db.php'; // Include database connection
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_SESSION['user_id'])) {
$recipe_id = $_POST['recipe_id'];
$rating = $_POST['rating'];
$user_id = $_SESSION['user_id'];
// Insert rating into the database
$sql = "INSERT INTO ratings (recipe_id, user_id, rating) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("iii", $recipe_id, $user_id, $rating);
if ($stmt->execute()) {
echo "Rating submitted successfully!";
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Rate Recipe</title>
</head>
<body>
<h1>Rate This Recipe</h1>
<form action="rate_recipe.php" method="POST">
<input type="hidden" name="recipe_id" value="<?php echo htmlspecialchars($_GET['id']); ?>">
<label for="rating">Rating (1 to 5):</label>
<input type="number" id="rating" name="rating" min="1" max="5" required><br>
<input type="submit" value="Submit Rating">
</form>
</body>
</html>
This script allows users to submit their ratings for recipes.
Commenting on Recipes
Users can also comment on recipes using the comment_recipe.php
script. Here’s how to set it up:
<?php
include 'db.php'; // Include database connection
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_SESSION['user_id'])) {
$recipe_id = $_POST['recipe_id'];
$comment = $_POST['comment'];
$user_id = $_SESSION['user_id'];
// Insert comment into the database
$sql = "INSERT INTO comments (recipe_id, user_id, comment) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("iis", $recipe_id, $user_id, $comment);
if ($stmt->execute()) {
echo "Comment posted successfully!";
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Comment on Recipe</title>
</head>
<body>
<h1>Comment on This Recipe</h1>
<form action="comment_recipe.php" method="POST">
<input type="hidden" name="recipe_id" value="<?php echo htmlspecialchars($_GET['id']); ?>">
<label for="comment">Your Comment:</label>
<textarea id="comment" name="comment" required></textarea><br>
<input type="submit" value="Submit Comment">
</form>
</body>
</html>
This script enables users to leave comments on recipes, enriching the interaction on your platform.
php exercises and solutions
php exercises and solutions pdf
php projects with source code
php source code projects
Adding Some Flavor: Styling Your Platform
To make your recipe-sharing platform visually appealing, add some styling with CSS. Here’s a simple stylesheet to give your site a polished look:
styles.css
body {
font-family: Arial, sans-serif;
margin: 20px;
padding: 20px;
}
h1, h2 {
color: #333;
}
form {
margin-top: 20px;
}
input[type="text"], input[type="password"], input[type="email"], textarea {
width: 100%;
padding: 10px;
margin: 10px 0;
border: 1px solid #ddd;
border-radius: 4px;
}
input[type="submit"] {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #45a049;
}
This CSS file styles forms and buttons, making your platform user-friendly and attractive.
Wrapping Up
Congratulations! You’ve now got the basic ingredients to build a fantastic recipe-sharing platform. With user registration, recipe management, ratings, comments, and some stylish design, you’re well on your way to creating a platform that food lovers will flock to. Happy coding and bon appétit!
0 Comments