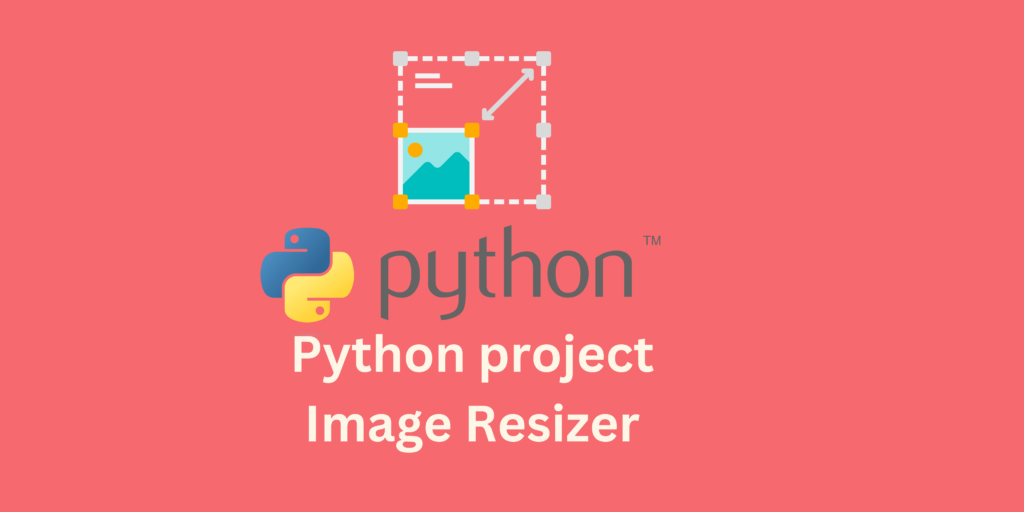
A Python project called the Image Resizer project uses the Pillow library to resize images to a given dimension. The software outputs a resized image with the specified width and height after reading an input image.
The program also has command-line arguments for specifying the desired width and height as well as the input and output files.
Steps of Python Project
Importing Modules
First, we import the required modules – argparse
and Pillow
.
import argparse
from PIL import Image
Parsing Command-Line Arguments
Next, we define a function called get_args
that uses the argparse
module to parse the command-line arguments.
Here, we create an argument parser object and add four arguments: input, output, width, and height, which stand for the desired image width and height, respectively.
Additionally, we define the width and height arguments’ data types as integers.
Then, after parsing the arguments, we return the outcome.
def get_args():
parser = argparse.ArgumentParser(description="Resize an image to a specified dimension.")
parser.add_argument("input", help="input image file path")
parser.add_argument("output", help="output image file path")
parser.add_argument("width", type=int, help="desired image width")
parser.add_argument("height", type=int, help="desired image height")
args = parser.parse_args()
return args
Resizing the Image
To obtain the command-line arguments, we first call the get_args function in the program’s main function. After opening the input image file with the Pillow library’s Image module, we resize the image to the desired width and height. The resized image is then saved to the output file path.
def main():
args = get_args()
with Image.open(args.input) as im:
resized_im = im.resize((args.width, args.height))
resized_im.save(args.output)
print(f"Resized image saved to {args.output}.")
Running the Program
if __name__ == "__main__":
main()
We can also run the program from the command line using the following syntax:
python image_resizer.py input_image.jpg output_image.jpg 800 600
This resizes the input_image.jpg
to a width of 800 pixels and a height of 600 pixels and saves it as output_image.jpg
.
Conclusion of the python project
In conclusion, the Python Image Resizer project shows how to resize images using the Pillow library and how to parse command-line arguments using the argparse module.
This project can be made even better by incorporating more sophisticated image processing methods like cropping, rotation, and filtering, as well as support for simultaneously resizing multiple images.
0 Comments