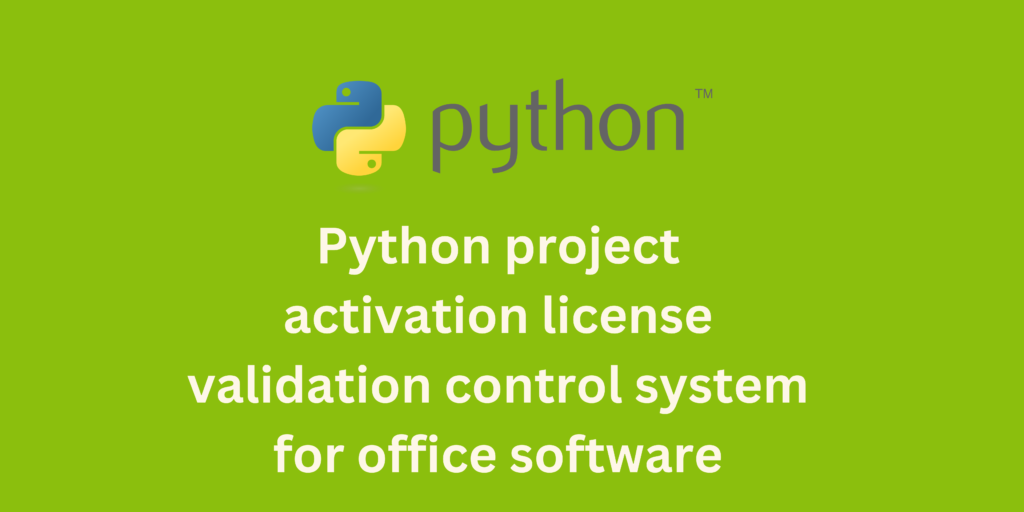
To create an activation license validation control system for office software in Python, you will need to follow these general steps:
- Generate unique license keys for your users. You can use a library like
uuid
orhashlib
to create unique license keys based on user information and expiration dates. - Store the license keys and user information in a database or file. You can use a lightweight database like SQLite or a text file to store the license keys and associated user information.
- Create a user interface to allow users to enter their license key and activate the software. This can be a simple text input field or a more complex dialog with multiple steps.
- When a user enters their license key, validate it against the stored license keys in your database or file. You can use a library like
sqlite3
orcsv
to read the license keys from your storage. - If the license key is valid, activate the software for the user. This can involve updating a configuration file or storing a flag in memory.
- If the license key is not valid, display an error message and prevent the user from using the software.
import sqlite3
import uuid
# Step 1: Generate a unique license key for a user
user_info = {'name': 'John Doe', 'email': 'johndoe@example.com', 'expiration_date': '2023-12-31'}
license_key = str(uuid.uuid4())
# Step 2: Store the license key and user information in a SQLite database
conn = sqlite3.connect('licenses.db')
c = conn.cursor()
c.execute('CREATE TABLE IF NOT EXISTS licenses (license_key TEXT, name TEXT, email TEXT, expiration_date TEXT)')
c.execute('INSERT INTO licenses VALUES (?, ?, ?, ?)', (license_key, user_info['name'], user_info['email'], user_info['expiration_date']))
conn.commit()
conn.close()
# Step 3: Create a user interface to allow users to enter their license key and activate the software
user_input = input('Enter your license key: ')
# Step 4: Validate the license key against the stored license keys in the SQLite database
conn = sqlite3.connect('licenses.db')
c = conn.cursor()
c.execute('SELECT * FROM licenses WHERE license_key=?', (user_input,))
result = c.fetchone()
if result:
print('License key is valid.')
# Step 5: Activate the software for the user
# Do something here to activate the software, e.g. update a configuration file or set a flag in memory
else:
print('Invalid license key.')
# Step 6: Display an error message and prevent the user from using the software
This is a simplified example and that you may need to modify it to fit your specific use case.
Additional details
- Security: It’s important to ensure that your license validation system is secure and can’t be easily bypassed or tampered with. One way to increase security is to use encryption or hashing to protect the license keys and user information stored in your database or file. Additionally, you may want to consider using a public-key cryptography system to ensure that only legitimate license keys can activate your software.
- Expiration dates: You can add an expiration date to each license key to limit the amount of time that the software can be used. This can help prevent users from sharing their license key with others or using the software after their license has expired.
- Activation limits: You may want to limit the number of times that a license key can be used to activate the software. This can help prevent users from sharing their license key with others or using the software on multiple machines.
- Revocation: In the event that a license key is stolen or used fraudulently, you may need to revoke the key to prevent further use. To do this, you can add a revocation flag to the license key in your database or file and check for this flag during validation.
- User feedback: It’s important to provide clear feedback to users when they enter a license key. This can include messages indicating whether the key is valid or invalid, why the key may not be valid (e.g. expired or revoked), and instructions on how to resolve any issues.
- Error handling: It’s important to handle errors gracefully in your activation license validation control system. This can include catching exceptions and providing meaningful error messages to users when something goes wrong.
- Logging: You may want to log activation attempts and license key validation events to help with troubleshooting and auditing.
import sqlite3
import uuid
from hashlib import sha256
from datetime import datetime
DATABASE_FILE = 'licenses.db'
HASH_SECRET = 'mysecretkey'
def generate_license_key(user_info):
"""Generate a license key based on user information and expiration date."""
key_data = f"{user_info['name']}{user_info['email']}{user_info['expiration_date']}{HASH_SECRET}"
return str(uuid.UUID(sha256(key_data.encode()).hexdigest()))
def store_license_key(license_key, user_info):
"""Store the license key and user information in a SQLite database."""
conn = sqlite3.connect(DATABASE_FILE)
c = conn.cursor()
c.execute('CREATE TABLE IF NOT EXISTS licenses (license_key TEXT, name TEXT, email TEXT, expiration_date TEXT, activated INTEGER DEFAULT 0, revoked INTEGER DEFAULT 0)')
c.execute('INSERT INTO licenses VALUES (?, ?, ?, ?, ?, ?)', (license_key, user_info['name'], user_info['email'], user_info['expiration_date'], 0, 0))
conn.commit()
conn.close()
def activate_license(license_key):
"""Activate the software for the user associated with the license key."""
conn = sqlite3.connect(DATABASE_FILE)
c = conn.cursor()
c.execute('UPDATE licenses SET activated=1 WHERE license_key=?', (license_key,))
conn.commit()
conn.close()
def revoke_license(license_key):
"""Revoke the license key to prevent further use."""
conn = sqlite3.connect(DATABASE_FILE)
c = conn.cursor()
c.execute('UPDATE licenses SET revoked=1 WHERE license_key=?', (license_key,))
conn.commit()
conn.close()
def is_license_key_valid(license_key):
"""Check if the license key is valid and not expired or revoked."""
conn = sqlite3.connect(DATABASE_FILE)
c = conn.cursor()
c.execute('SELECT * FROM licenses WHERE license_key=? AND activated=1 AND revoked=0', (license_key,))
result = c.fetchone()
conn.close()
if result:
expiration_date = datetime.strptime(result[3], '%Y-%m-%d').date()
if expiration_date >= datetime.now().date():
return True
return False
def activate_office():
"""Activate the office software using a valid license key."""
user_input = input('Enter your license key: ')
if is_license_key_valid(user_input):
activate_license(user_input)
print('Activation successful.')
else:
print('Invalid or expired license key.')
if __name__ == '__main__':
user_info = {'name': 'John Doe', 'email': 'johndoe@example.com', 'expiration_date': '2023-12-31'}
license_key = generate_license_key(user_info)
store_license_key(license_key, user_info)
activate_office()
0 Comments