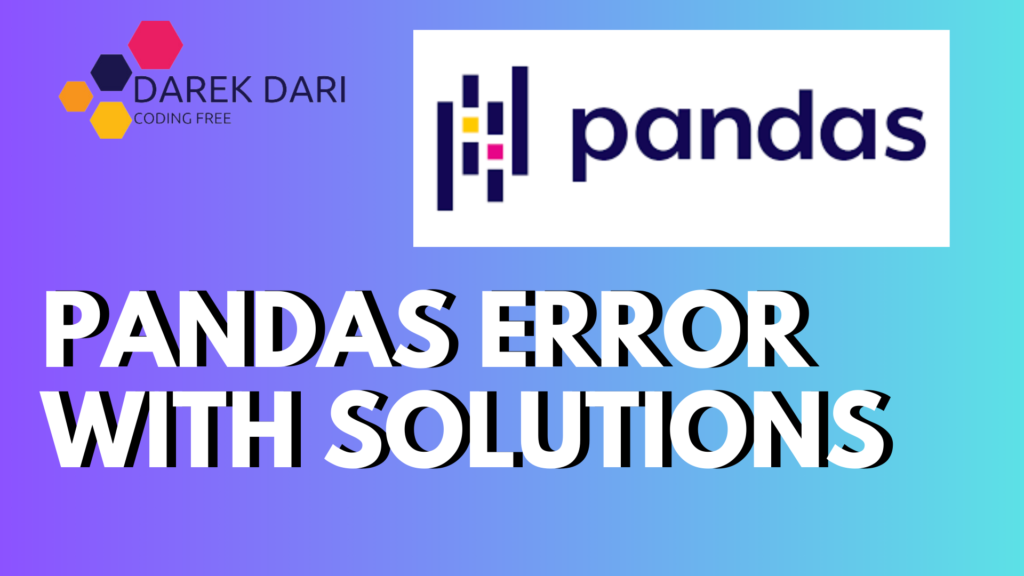
Table of Contents
Introduction to Pandas Error
Hello, data lovers! 😄
Exploring data analysis with Pandas can bring great rewards, but it does come with its own set of challenges. Whether you’re a seasoned expert or just getting started, you may have encountered some tricky issues.
But don’t worry! In this guide, we will delve into the 7 most common errors in Pandas and provide you with step-by-step solutions.
pandas
pandas py
pandas dataframe
pandas dataframe python
python pandas
pandas csv
pandas read excel
pandas docs
dataframe python
dataframe
From handling infinite values and silencing annoying warnings to comparing DataFrames and Series, we will present practical fixes to keep your data organized and your code running smoothly.
We will also address common pitfalls such as AbstractMethodError, AttributeConflictWarning, and ChainedAssignmentError to ensure you are well-prepared to tackle any obstacles that may arise.
Are you ready to transform these errors into learning opportunities? Let’s dive in and conquer these Pandas challenges together! 🚀.
Fill Infinity With 0 Pandas
Hey there! 😄
Got a DataFrame in Pandas and spotted some pesky infinite values? No worries, I’ve got you covered! Let’s walk through how to replace those infinite values with 0, step by step.
Import Pandas and NumPy:
First things first, make sure you have Pandas and NumPy installed. If not, you can easily install them using:
pip install pandas numpy
Create a Sample DataFrame:
Let’s create a DataFrame with some infinite values to play around with.
Replace Infinite Values:
Now, we’ll use the replace
method to swap out those infinite values for a nice, clean 0.
Check Out Your Updated DataFrame:
Finally, we’ll print the DataFrame to see the magic!
Here’s the full code:
import pandas as pd
import numpy as np
# Step 1: Import Pandas and NumPy
# You got this! Just a quick import.
# Step 2: Create a Sample DataFrame
data = {
'Column1': [1, 2, np.inf, 4],
'Column2': [5, np.inf, 7, 8],
'Column3': [9, 10, 11, -np.inf]
}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Step 3: Replace Infinite Values
# Time to say goodbye to infinity! 🌌
df.replace([np.inf, -np.inf], 0, inplace=True)
# Step 4: Check Out Your Updated DataFrame
print("\nDataFrame after replacing infinite values with 0:")
print(df)
pandas cheat sheet
pandas logo
data frame python
dataframe in python
pandas documentation
pandas doc
pandas document
panda documentation
What’s Happening Here?
Import Libraries:
We start by importing Pandas and NumPy.
import pandas as pd
import numpy as np
Create a Sample DataFrame:
We create a DataFrame df
that includes some infinite values. This will help us see the changes clearly.
data = {
'Column1': [1, 2, np.inf, 4],
'Column2': [5, np.inf, 7, 8],
'Column3': [9, 10, 11, -np.inf]
}
df = pd.DataFrame(data)
Replace Infinite Values:
Here’s where the magic happens! Using the replace
method, we swap all np.inf
and -np.inf
values with 0. The inplace=True
argument makes sure our DataFrame is updated directly.
df.replace([np.inf, -np.inf], 0, inplace=True)
Print the Updated DataFrame:
Finally, we print the updated DataFrame to see our clean, infinity-free data.
print("\nDataFrame after replacing infinite values with 0:")
print(df)
And voila! Your DataFrame is now free from infinite values, replaced with friendly zeros. 🎉
Here’s what it looks like:
Original DataFrame:
Column1 Column2 Column3
0 1.0 5.0 9.0
1 2.0 inf 10.0
2 inf 7.0 11.0
3 4.0 8.0 -inf
DataFrame after replacing infinite values with 0:
Column1 Column2 Column3
0 1.0 5.0 9.0
1 2.0 0.0 10.0
2 0.0 7.0 11.0
3 4.0 8.0 0.0
Hope this helps you keep your data tidy and ready for analysis.
install pandas
pandas install
pip install pandas
install pandas pip
python pandas install
pandas python install
python install pandas
Pandas Suppress Warnings
If you’re getting overwhelmed by warnings while working with Pandas, don’t worry—I’ve got a simple fix for you. Let’s dive into how you can suppress those warnings and keep your console neat.
First, make sure you have Pandas installed. You’ll also need the warnings
library, which is included with Python. Here’s the code to suppress warnings:
import pandas as pd
import warnings
# Suppress all warnings
warnings.filterwarnings("ignore")
# Example: Create a DataFrame and perform an operation that might trigger warnings
data = {
'Column1': [1, 2, 3, 4],
'Column2': [5, 6, 7, 8]
}
df = pd.DataFrame(data)
# Perform operations that might generate warnings (just an example)
df['Column3'] = df['Column1'] / 0 # This will normally generate a division by zero warning
print("DataFrame with suppressed warnings:")
print(df)
We bring in Pandas and the warnings library to manage warnings.
We utilize warnings.filterwarnings(“ignore”) to suppress all warnings. This will stop any warning messages from popping up.
We generate a DataFrame and execute an action that would typically cause a warning (such as dividing by zero). Because we’ve suppressed warnings, no warning messages will be displayed.
And that’s all there is to it! Your DataFrame operations will no longer be bothered by those pesky warning messages. 🎉.
python for data analysis
data analysis with python book
pandas pandas
what is pandas python
python for data science
pandas tutorial
tutorial on pandas
tutorial pandas
pandas python tutorial
Pandas Error1: pandas.testing.assert_frame_equal
When you’re working with DataFrames in Pandas and want to ensure they are equal, pandas.testing.assert_frame_equal
is a handy tool. It’s used for comparing two DataFrames to verify they are identical, which is particularly useful for testing.
Here’s a friendly guide on how to use assert_frame_equal
:
How to Use pandas.testing.assert_frame_equal
- Import Pandas and Testing Module:
First, make sure you have Pandas installed. Import both Pandas and thetesting
module. - Create DataFrames:
Prepare the DataFrames you want to compare. - Use
assert_frame_equal
:
Use theassert_frame_equal
function to check if the DataFrames are the same.
Here’s a complete example:
import pandas as pd
import pandas.testing as pdt
# Create sample DataFrames
df1 = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6]
})
df2 = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6]
})
# Use assert_frame_equal to check if the DataFrames are the same
try:
pdt.assert_frame_equal(df1, df2)
print("The DataFrames are equal!")
except AssertionError:
print("The DataFrames are not equal.")
What’s Happening Here?
- Import Libraries:
You start by importing Pandas and thetesting
module.
import pandas as pd
import pandas.testing as pdt
- Create DataFrames:
Prepare the DataFrames you want to compare.
df1 = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6]
})
df2 = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6]
})
- Compare DataFrames:
Usepandas.testing.assert_frame_equal
to compare the DataFrames. If they are the same, it will print “The DataFrames are equal!” If they differ, it will raise anAssertionError
and print “The DataFrames are not equal.”
try:
pdt.assert_frame_equal(df1, df2)
print("The DataFrames are equal!")
except AssertionError:
print("The DataFrames are not equal.")
pandas in python tutorial
pandas python tutorials
pandas tutorial python
pandas example
pandas python examples
python pandas example
pandas python example
Customizing the Comparison
assert_frame_equal
also allows for customization. You can use parameters like check_dtype
to control whether to check the data types of the DataFrames, or check_like
to check if the DataFrames have the same data regardless of the index or column order.
Here’s a quick example with parameters:
pdt.assert_frame_equal(df1, df2, check_dtype=False, check_like=True)
check_dtype=False
means data types will not be checked.check_like=True
means the order of rows and columns won’t matter.
And that’s it! With assert_frame_equal
, you can easily compare DataFrames and ensure they match exactly or with your specified criteria.
Pandas Error 2: pandas.testing.assert_series_equal
When you’re dealing with Pandas Series and need to verify that they are identical, pandas.testing.assert_series_equal
is the tool for the job. It’s perfect for testing and ensuring that two Series are exactly the same.
Here’s a friendly guide on how to use assert_series_equal
:
How to Use pandas.testing.assert_series_equal
- Import Pandas and Testing Module:
Make sure Pandas is installed, and import both Pandas and thetesting
module. - Create Series:
Prepare the Series you want to compare. - Use
assert_series_equal
:
Use theassert_series_equal
function to check if the Series are the same.
Here’s a complete example:
import pandas as pd
import pandas.testing as pdt
# Create sample Series
s1 = pd.Series([1, 2, 3, 4], name='A')
s2 = pd.Series([1, 2, 3, 4], name='A')
# Use assert_series_equal to check if the Series are the same
try:
pdt.assert_series_equal(s1, s2)
print("The Series are equal!")
except AssertionError:
print("The Series are not equal.")
What’s Happening Here?
- Import Libraries:
Import Pandas and thetesting
module to use the comparison function.
import pandas as pd
import pandas.testing as pdt
- Create Series:
Define the Series you want to compare.
s1 = pd.Series([1, 2, 3, 4], name='A')
s2 = pd.Series([1, 2, 3, 4], name='A')
- Compare Series:
Usepandas.testing.assert_series_equal
to compare the Series. If they are identical, it will print “The Series are equal!” If they differ, it will raise anAssertionError
and print “The Series are not equal.”
try:
pdt.assert_series_equal(s1, s2)
print("The Series are equal!")
except AssertionError:
print("The Series are not equal.")
pandas excel
read csv pandas
pandas read xlsx
read xlsx in python
python read csv pandas
read csv in python pandas
read csv python pandas
pandas python read csv
pandas excel sheet
Customizing the Comparison
assert_series_equal
also offers customization options. You can use parameters like check_dtype
to control whether to check the data types, or check_index_type
to check if the index types match.
Here’s a quick example with parameters:
pdt.assert_series_equal(s1, s2, check_dtype=True, check_index_type='equiv')
check_dtype=True
means data types will be checked.check_index_type='equiv'
means the index type will be compared in a way that allows for different index types with the same labels.
With assert_series_equal
, you can easily ensure that two Series are identical or compare them with your specified criteria.
Pandas Error 3: pandas.testing.assert_index_equal
When you need to verify that two Pandas Index objects are identical, pandas.testing.assert_index_equal
is the tool for the job. It’s particularly useful in testing scenarios where you need to ensure that Indexes match exactly.
Here’s a friendly guide on how to use assert_index_equal
:
How to Use pandas.testing.assert_index_equal
- Import Pandas and Testing Module:
Ensure you have Pandas installed, and import both Pandas and thetesting
module. - Create Index Objects:
Define the Index objects you want to compare. - Use
assert_index_equal
:
Use theassert_index_equal
function to check if the Indexes are the same.
Here’s a complete example:
import pandas as pd
import pandas.testing as pdt
# Create sample Index objects
index1 = pd.Index([1, 2, 3, 4], name='index')
index2 = pd.Index([1, 2, 3, 4], name='index')
# Use assert_index_equal to check if the Indexes are the same
try:
pdt.assert_index_equal(index1, index2)
print("The Indexes are equal!")
except AssertionError:
print("The Indexes are not equal.")
What’s Happening Here?
- Import Libraries:
Start by importing Pandas and thetesting
module for comparison functions.
import pandas as pd
import pandas.testing as pdt
- Create Index Objects:
Define the Index objects you want to compare.
index1 = pd.Index([1, 2, 3, 4], name='index')
index2 = pd.Index([1, 2, 3, 4], name='index')
- Compare Indexes:
Usepandas.testing.assert_index_equal
to compare the Index objects. If they are identical, it will print “The Indexes are equal!” If they differ, it will raise anAssertionError
and print “The Indexes are not equal.”
try:
pdt.assert_index_equal(index1, index2)
print("The Indexes are equal!")
except AssertionError:
print("The Indexes are not equal.")
Customizing the Comparison
assert_index_equal
also provides options to customize the comparison. For instance, you can use parameters like check_dtype
to control whether to check the data types, or check_index_type
to specify how to compare the index types.
Here’s an example with parameters:
pdt.assert_index_equal(index1, index2, check_dtype=True, check_index_type='equiv')
check_dtype=True
ensures that the data types of the Index objects are checked.check_index_type='equiv'
allows comparison of Index objects with different types but the same labels.
With assert_index_equal
, you can easily ensure that two Index objects match exactly or according to your specified criteria.
pandas book
pandas function
pandas functions
pandas python documentation
python pandas documentation
pandas source
pandas source code
pandas library documentation
pandas library doc
pandas python book
python pandas books
Pandas Error 3: pandas.testing.assert_extension_array_equal
If you need to compare two ExtensionArrays in Pandas, pandas.testing.assert_extension_array_equal
is your go-to function. First, make sure you’ve imported Pandas and the testing module:
import pandas as pd
import pandas.testing as pdt
Next, create the ExtensionArrays you want to compare:
ext_arr1 = pd.array([1, 2, 3, 4], dtype="Int64")
ext_arr2 = pd.array([1, 2, 3, 4], dtype="Int64")
To compare the arrays, use assert_extension_array_equal
:
try:
pdt.assert_extension_array_equal(ext_arr1, ext_arr2)
print("The Extension Arrays are equal!")
except AssertionError:
print("The Extension Arrays are not equal.")
You can also customize the comparison with parameters like check_dtype=True
to ensure data types are compared. For instance:
pdt.assert_extension_array_equal(ext_arr1, ext_arr2, check_dtype=True)
This function helps you verify that your ExtensionArrays are identical, making it perfect for testing and data consistency checks.
pandas github
panda github
github pandas
github panda
pandas repo
Pandas Error 4: pandas.errors.AbstractMethodError
An AbstractMethodError
in Pandas indicates that a required method hasn’t been implemented in your custom class or extension. This is common when dealing with custom extension types or advanced features.
For instance, if you’re creating a custom extension array and forget to implement required methods, you might see this error. Here’s how you can handle it:
Example of the Error
Suppose you create a custom extension array but forget to implement the __getitem__
method:
from pandas.api.extensions import ExtensionArray
class MyArray(ExtensionArray):
# Missing implementation for __getitem__
pass
You’ll need to implement the missing methods. Here’s how you can fix it:
Correct Implementation
Make sure to implement all required abstract methods:
from pandas.api.extensions import ExtensionArray
class MyArray(ExtensionArray):
def __getitem__(self, item):
# Example implementation
return self._data[item]
def __len__(self):
# Example implementation
return len(self._data)
# Implement other required methods as needed
def __init__(self, data):
self._data = data
In this example, the __getitem__
and __len__
methods are provided, which were missing in the initial implementation.
Check the error message to see which methods are required and ensure they are implemented in your custom class. For further details, refer to the Pandas documentation to understand the necessary methods for your extensions.
pandas dataframe cheat sheet
panda dataframe cheat sheet
pandas python cheat sheet
pandas functions cheat sheet
pandas commands cheat sheet
pandas basics cheat sheet
Pandas Error 5: pandas.errors.AttributeConflictWarning
The AttributeConflictWarning
in Pandas is a warning that occurs when there is a conflict between an attribute of a DataFrame or Series and a method name, often due to overlapping names.
hen you see an AttributeConflictWarning
, it typically means that there’s a naming conflict between an attribute and a method in a Pandas DataFrame or Series. This often happens when a user-defined attribute or method name overlaps with existing names in Pandas.
Example of the Warning
Suppose you have a DataFrame and accidentally name an attribute with the same name as an existing method:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3]})
# Define an attribute with the same name as an existing method
df.head = "This is a conflict"
In this case, you might see a warning because head
is a method of the DataFrame, and assigning a new value to df.head
conflicts with the existing method.
How to Resolve
To resolve this warning, ensure that your attribute names do not conflict with existing methods or attributes. Here’s how to fix the issue:
- Use Unique Names: Choose names for attributes that don’t overlap with existing method names in Pandas.
- Avoid Overwriting Methods: Be cautious not to overwrite existing methods or attributes.
Example Fix
Rename your custom attribute to avoid conflicts:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3]})
# Define an attribute with a unique name
df.custom_attribute = "This is a unique name"
By using unique names for attributes, you prevent naming conflicts and avoid AttributeConflictWarning
. This helps keep your code clean and avoids unintended issues with Pandas functionality.
pandas data analysis cheat sheet
pandas python functions
python pandas methods
pandas functions python
common pandas functions
Pandas Error 6: pandas.errors.CategoricalConversionWarning
The CategoricalConversionWarning
in Pandas occurs when you try to convert a non-categorical data type to a categorical type and the conversion is not straightforward. This warning is commonly seen when converting between types or when data doesn’t fit neatly into categories.
What is CategoricalConversionWarning
?
This warning is triggered when Pandas detects that a conversion to or from a categorical type may not be as intended. It usually occurs if you’re trying to convert data that doesn’t fit well into categories or if there’s a potential issue with how categories are being handled.
Example of the Warning
You might encounter this warning when converting a DataFrame column to a categorical type with unexpected values:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': ['apple', 'banana', 'cherry', 'apple', 'banana']})
# Convert column to categorical type
df['A'] = df['A'].astype('category')
# Change the order of categories which might trigger a warning
df['A'] = df['A'].cat.set_categories(['banana', 'apple', 'cherry'], ordered=True)
How to Resolve
To handle the CategoricalConversionWarning
, you should:
- Check Data Consistency: Ensure the data you’re converting fits the categorical structure you’re applying.
- Specify Categories Explicitly: When setting categories, make sure they match the values in your data. This avoids mismatches that can trigger warnings.
- Handle Missing Values: Make sure that any NaN or missing values are handled appropriately before conversion.
Example Fix
Here’s how you might adjust the code to avoid the warning:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': ['apple', 'banana', 'cherry', 'apple', 'banana']})
# Convert column to categorical type with specified categories
df['A'] = pd.Categorical(df['A'], categories=['apple', 'banana', 'cherry'])
# Check the DataFrame
print(df)
By ensuring that your categories align with your data and handling any potential issues proactively, you can avoid CategoricalConversionWarning
and work with categorical data more effectively.
pandas python package
pandas python logo
python panda read csv
python pandas load csv
install panda python
pandas data analysis cheat sheet
pandas library functions
Pandas Error 7: pandas.errors.ChainedAssignmentError
The ChainedAssignmentError
in Pandas is a warning that occurs when you modify a DataFrame or Series in a way that might lead to unintended side effects. This typically happens when you’re using chained indexing, which can result in ambiguous or unreliable results.
What is ChainedAssignmentError
?
This warning is triggered when you perform operations that involve chaining indexing or assignment, which can lead to unpredictable behavior. It’s a way to signal that Pandas isn’t sure whether you’re modifying a view or a copy of the data, which might cause bugs in your code.
Example of the Warning
You might see this warning if you try to set a value in a DataFrame using chained indexing:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Attempt to modify a value using chained indexing
df[df['A'] > 1]['B'] = 10
This will trigger a ChainedAssignmentError
because it’s unclear whether you’re modifying the original DataFrame or a temporary view of it.
How to Resolve
To avoid ChainedAssignmentError
, use the following strategies:
- Use
.loc
for Assignment: Always use.loc
to access and modify data, which makes it clear whether you are working with a view or a copy. - Avoid Chained Indexing: Break down complex operations into simpler steps to avoid ambiguity.
- Ensure Data Consistency: Make sure you’re modifying the DataFrame or Series in a way that avoids unintended side effects.
Example Fix
Here’s how to modify the DataFrame correctly using .loc
:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Use .loc for safe assignment
df.loc[df['A'] > 1, 'B'] = 10
In this corrected version, df.loc[df['A'] > 1, 'B']
ensures that you’re directly modifying the DataFrame rather than a temporary view, preventing the ChainedAssignmentError
.
To avoid ChainedAssignmentError
, always use .loc
for assignments and be cautious with chained indexing. This approach helps ensure that your modifications are clear and reliable.
pandas all functions
python package pandas
excel in python pandas
pandas dataframe github
Conclusion
In conclusion, becoming proficient in Pandas requires not only grasping its robust data manipulation capabilities but also effectively managing common errors that may occur. This guide has covered the top 7 Pandas errors and provided detailed solutions:
- Replacing Infinity with 0 – Eliminate troublesome infinite values to maintain tidy and organized data.
- Silencing Warnings – Keep your command line clutter-free by muting unnecessary warning messages.
- Validating DataFrames with assert_frame_equal – Ensure precise matching of your DataFrames.
- Validating Series with assert_series_equal – Confirm the identity or specific criteria of your Series.
- Validating Indexes with assert_index_equal – Guarantee equality and consistency of your Index objects.
- Validating Extension Arrays with assert_extension_array_equal – Verify the sameness of your ExtensionArrays.
- Resolving Errors such as AbstractMethodError, AttributeConflictWarning, and ChainedAssignmentError – Address common Pandas errors and warnings to prevent issues and ensure seamless data processing.
Armed with these techniques, you are well-prepared to tackle diverse obstacles and maintain the smooth operation of your data analysis.
Remember, effective error management not only promotes clean code but also enhances your confidence in utilizing Pandas. Happy analyzing, and may your data always remain flawless! 🎉.
0 Comments