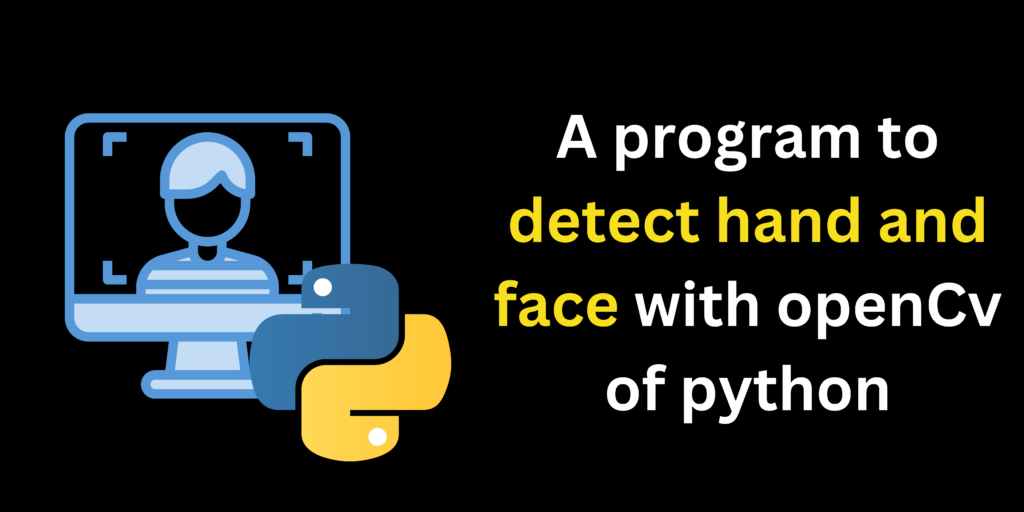
Introduction
A program to detect hand and face with openCv of python. OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library that can be used to develop real-time computer vision applications. In Python, OpenCV provides a number of functions that make it easy to detect faces and hands in images and video streams.
detect hand and face with openCv
To get started with face and hand detection using OpenCV in Python, you will need to install the OpenCV library using pip. You can do this by opening a terminal window and entering the following command:
pip install opencv-python
Once you have installed OpenCV, you can create a Python script to detect faces and hands in an image or video stream. Here is an example program that demonstrates how to detect faces and hands using OpenCV in Python:
import cv2 # Load the cascade for detecting faces and hands face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') hand_cascade = cv2.CascadeClassifier('hand.xml') # Open the camera cap = cv2.VideoCapture(0) while True: # Read the frame from the camera ret, frame = cap.read() # Convert the frame to grayscale gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Detect faces faces = face_cascade.detectMultiScale(gray, 1.3, 5) # Draw rectangles around each face for (x,y,w,h) in faces: cv2.rectangle(frame,(x,y),(x+w,y+h),(255,0,0),2) # Detect hands hands = hand_cascade.detectMultiScale(gray, 1.3, 5) # Draw rectangles around each hand for (x,y,w,h) in hands: cv2.rectangle(frame,(x,y),(x+w,y+h),(0,255,0),2) # Display the resulting frame cv2.imshow('frame',frame) # Exit if the user presses 'q' if cv2.waitKey(1) & 0xFF == ord('q'): break # Release the capture and close the window cap.release() cv2.destroyAllWindows()
Learn more
Conclusion
To sum up, OpenCV in Python has robust features for detecting faces and hands in images and videos. It uses sophisticated computer vision algorithms such as Haar cascades and HOG to precisely identify and follow facial features and hand movements. This technology has diverse applications, including security systems, gesture-based interfaces, and virtual reality environments. Moreover, OpenCV is user-friendly and comes with extensive documentation, making it a great choice for developing computer vision applications in Python.
0 Comments