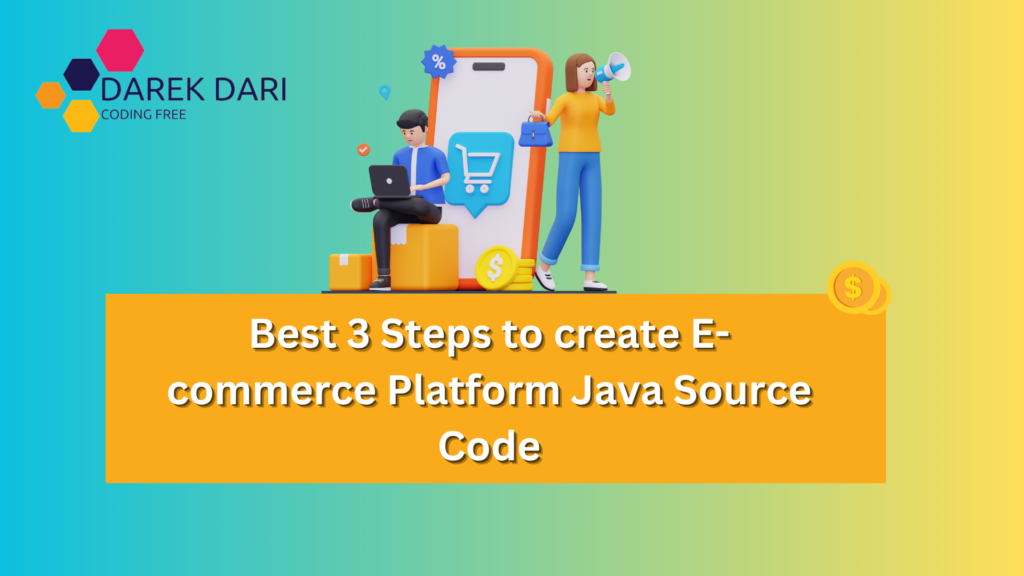
Table of Contents
Introduction
Hey there, aspiring e-commerce guru! 🌟 Are you excited to explore the world of creating your very own online store using Java? Get ready for a thrilling and straightforward journey ahead. Here’s the scoop. We have a trio of fantastic steps lined up to help you launch your e-commerce platform.
First things first – let’s delve into user authentication. Every store needs a way to identify its customers, right? That’s exactly what we’re focusing on here. You’ll establish a system that allows users to register, log in, and safeguard their information.
Think of it as the gatekeeper at a trendy club, only granting access to the right individuals. 💪
Next on the agenda – the product catalog. This is where the enchantment unfolds. You’ll devise a method to showcase all the amazing products you have on offer.
Envision a virtual aisle where customers can explore, search, and marvel at all the fantastic items. It needs to be well-organized, with categories, prices, and descriptions, so customers can easily find what they’re looking for.
e-commerce code
java e-commerce project source code
java e commerce project source code github
Last but not least – we’re venturing into the shopping cart. This is where customers gather all their essentials before proceeding to click that delightful “checkout” button.
You’ll need to program it to remember their selections, calculate totals, and guide them smoothly through the purchasing process. Visualize a little basket that accompanies them, gathering treasures along the way.
There you have it, champ! Three straightforward steps to set your e-commerce platform in motion. Ready to kick off your coding journey? Let’s get started! 🚀.
E-commerce Platform Java Source Code
Code 1: User Authentication
public class UserAuthentication {
public boolean authenticateUser(String username, String password) {
// Authentication logic here
return true; // Placeholder for actual authentication process
}
}
Explaining Code 1: User Authentication
Alright, let’s break down the User Authentication code. This is the part where we make sure only the right people can get into your e-commerce platform. Here’s a simple Java class to get you started:
public class UserAuthentication {
public boolean authenticateUser(String username, String password) {
// Authentication logic here
return true; // Placeholder for actual authentication process
}
}
What’s Happening Here?
1. Class Definition
public class UserAuthentication
: This line defines a public class namedUserAuthentication
. A class in Java is like a blueprint for creating objects. In this case, it’s a blueprint for user authentication objects.
2. Method Declaration
public boolean authenticateUser(String username, String password)
: This line declares a public method namedauthenticateUser
. It’s a method that returns a boolean value (true or false) and takes two parameters:username
andpassword
. These parameters represent the user’s login credentials.
3. Placeholder for Authentication Logic
// Authentication logic here
: This is a comment indicating where you’d put the actual code to check if the username and password are correct. This could involve checking a database or an external service to see if the credentials match.
4. Return Statement
return true;
: This line is a placeholder that always returnstrue
. In a real application, this would returntrue
if the credentials are valid andfalse
otherwise.
java project topics list
ecommerce project examples
ecommerce java project
ecommerce java project github
What You’d Do in Real Life
In a real-world scenario, the authenticateUser
method would include code to:
- Connect to a database.
- Query the database to find the user with the provided username.
- Compare the provided password with the stored password (usually hashed) for that user.
- Return
true
if the credentials match, otherwise returnfalse
.
For example, the method might look something like this with actual logic:
public boolean authenticateUser(String username, String password) {
// Fetch user data from database
User user = database.getUserByUsername(username);
// Check if user exists and passwords match
if (user != null && user.getPassword().equals(hashPassword(password))) {
return true;
}
return false;
}
This simple class is the starting point for handling user logins. You’d expand it to include real authentication logic, making your e-commerce platform secure and reliable. Now, you’re ready to build the first brick of your e-commerce empire! 🚀
Code 2: Product Catalog
import java.util.HashMap;
import java.util.Map;
public class ProductCatalog {
private Map<String, Double> products = new HashMap<>();
public void addProduct(String productName, double price) {
products.put(productName, price);
}
public double getProductPrice(String productName) {
return products.getOrDefault(productName, 0.0);
}
}
Explaining Code 2: Product Catalog
Let’s dive into the Product Catalog code. This part of your e-commerce platform is where you manage all the items you’re selling. Here’s a simple Java class to handle your product catalog:
import java.util.HashMap;
import java.util.Map;
public class ProductCatalog {
private Map<String, Double> products = new HashMap<>();
public void addProduct(String productName, double price) {
products.put(productName, price);
}
public double getProductPrice(String productName) {
return products.getOrDefault(productName, 0.0);
}
}
What’s Happening Here?
1. Import Statements
import java.util.HashMap;
import java.util.Map;
These lines import theHashMap
andMap
classes from the Java Collections Framework. AHashMap
is a collection that stores items in key-value pairs.
2. Class Definition
public class ProductCatalog
: This line defines a public class namedProductCatalog
. This class will manage the products in your catalog.
e commerce project in java source code
e commerce java project github
e-commerce project examples
e-commerce java project description for resume
3. Instance Variable
private Map<String, Double> products = new HashMap<>();
: This line creates aHashMap
to store the products. The keys areString
(product names), and the values areDouble
(product prices).
4. Method to Add Products
public void addProduct(String productName, double price)
: This method allows you to add a product to the catalog. It takes two parameters:productName
(aString
) andprice
(adouble
).products.put(productName, price);
: This line adds the product to theproducts
map, with the product name as the key and the price as the value.
5. Method to Get Product Price
public double getProductPrice(String productName)
: This method allows you to retrieve the price of a product. It takes one parameter:productName
(aString
).return products.getOrDefault(productName, 0.0);
: This line fetches the price of the product from theproducts
map. If the product isn’t found, it returns0.0
by default.
What You’d Do in Real Life
In a real-world application, you’d likely add more functionality to the ProductCatalog
class, such as:
- Methods to update product prices.
- Methods to remove products.
- More sophisticated error handling and validation.
- Integration with a database to persist product data.
Here’s an example with an additional method to update a product’s price:
public void updateProductPrice(String productName, double newPrice) {
if (products.containsKey(productName)) {
products.put(productName, newPrice);
}
}
e commerce java project github
e-commerce java tutorial
e commerce java project free download
java e-commerce open source projects
This simple class is the backbone of your product catalog, allowing you to add and retrieve product prices easily. It’s straightforward but essential for managing the items in your store. Now your customers can browse and see the prices of all the cool stuff you’re selling. Onward to building your e-commerce dream! 🚀
Code 3: Shopping Cart
import java.util.ArrayList;
import java.util.List;
public class ShoppingCart {
private List<String> items = new ArrayList<>();
public void addItem(String itemName) {
items.add(itemName);
}
public void removeItem(String itemName) {
items.remove(itemName);
}
public List<String> getItems() {
return items;
}
public void clearCart() {
items.clear();
}
}
Explaining Code 2: Product Catalog
This code defines a class named ShoppingCart
in Java that simulates a shopping cart system. Here’s a breakdown of the code:
List<String> items
: This line declares a private member variable nameditems
of typeList<String>
. This variable will hold the list of items in the shopping cart.addItem(String itemName)
: This method adds an item to the shopping cart. It takes a string argumentitemName
which represents the name of the item to be added. The method uses theadd
method of theitems
list to add theitemName
to the list.removeItem(String itemName)
: This method removes an item from the shopping cart. It takes a string argumentitemName
which represents the name of the item to be removed. The method first checks if theitemName
exists in theitems
list using thein
operator. If the item exists, theremove
method of theitems
list is called to remove the item.getItems() List<String>
: This method returns a list of all the items currently in the shopping cart. It simply returns theitems
list.clearCart()
: This method clears all the items from the shopping cart. It calls theclear
method of theitems
list to remove all items.
Here’s an example of how to use the ShoppingCart
class:
ShoppingCart cart = new ShoppingCart();
// Add some items to the cart
cart.addItem("Apples");
cart.addItem("Bread");
cart.addItem("Milk");
// Get the items in the cart
List<String> items = cart.getItems();
System.out.println("Items in the cart:", items);
// Remove an item from the cart
cart.removeItem("Bread");
// Get the items in the cart again
items = cart.getItems();
System.out.println("Items in the cart after removal:", items);
// Clear the cart
cart.clearCart();
// Get the items in the cart (should be empty now)
items = cart.getItems();
System.out.println("Items in the cart after clearing:", items);
This code will output the following:
Items in the cart: [Apples, Bread, Milk]
Items in the cart after removal: [Apples, Milk]
Items in the cart after clearing: []
As you can see, the
ShoppingCart
class provides a simple way to simulate a shopping cart system in Java. It allows you to add, remove, and get a list of all the items in the cart.
java based e commerce platform
e-commerce platform companies
e-commerce platform project
How to make an e-commerce website in Java?
To create a comprehensive e-commerce website using Java, you will need to focus on various elements. Here is a basic guide to help you kickstart your project:
1. Backend Development (Java):
- Server-side Framework: Utilize frameworks like Spring Boot to streamline the process of developing web applications. These frameworks handle essential tasks such as managing database connections, ensuring security, and routing requests.
- Database: Choose a reliable database system like MySQL or PostgreSQL to store crucial information such as product details, user data, and order history.
- Java Classes: Develop classes to represent your data model (Product, User, Order) and establish methods for database interaction (adding products, managing users, processing orders).
2. Shopping Cart Functionality (Covered in previous example):
- Implement a
ShoppingCart
class, similar to the one discussed earlier, to facilitate adding, removing, and retrieving items as users browse and proceed to checkout.
3. Security:
- Ensure secure user authentication (login/registration) by incorporating password hashing for enhanced protection.
- Opt for secure payment gateways like Stripe or PayPal to handle transactions securely, without storing sensitive credit card details on your server.
4. Frontend Development (HTML, CSS, JavaScript):
- Craft the user interface using HTML, CSS, and JavaScript. This includes designing product pages, a shopping cart view, checkout process, and user account management sections.
- Leverage frameworks like Bootstrap to create responsive layouts that adapt well to various devices.
Learning Resources:
Here are some valuable resources to assist you in building your e-commerce website with Java:
- Spring Boot Tutorial: https://start.spring.io/
- Java Database Connectivity (JDBC): https://docs.oracle.com/javase/tutorial/jdbc/basics/index.html
- E-commerce Website with Java and Spring Boot (YouTube): https://www.youtube.com/watch?v=U5TdEJnJU88 (Remember, this might use older libraries or practices, so check for updates)
Here’s a brief overview, but keep in mind that each step will require more in-depth attention. If you’re new to this, think about utilizing pre-existing e-commerce platforms instead of creating one from the ground up, as it demands a lot of time and expertise in development.
Remember, this is just the beginning. As you progress, you’ll come across more detailed features and obstacles. However, this should provide you with a guide to kickstart your e-commerce website development using Java!
How do I create an eCommerce platform?
Here’s a condensed version:
- Choose your niche and revenue strategy.
- Decide on platform (like Shopify) or custom coding.
- Ensure essential features like secure logins, product listings, cart, payments, and easy checkout.
- Prioritize user-friendly design, mobile compatibility, and security.
- Launch, promote, and continuously enhance your online store!
This roadmap is a basic guide to kickstart your e-commerce dream!
What are the 3 most important things that you can do to make your e-commerce site visible on the Web?
Here are three key strategies to boost the visibility of your e-commerce site on the web:
- Search Engine Optimization (SEO): Think of SEO as shining bright neon signs for online shoppers. Optimize your website by incorporating relevant keywords that people use to search for products like yours. Make sure to naturally include these keywords in your product descriptions, titles, and website content. Tools like Google Search Console can assist you in this process.
- Social Media Marketing: People spend a significant amount of time on social media platforms. Take advantage of this by actively promoting your products and engaging with potential customers on platforms such as Facebook, Instagram, or TikTok (depending on your target audience). You can also consider running targeted ads or collaborating with influencers to expand your reach to a wider audience.
- Paid Advertising: Consider utilizing pay-per-click (PPC) advertising on platforms like Google Ads. This allows you to specifically target relevant searches with tailored ads, driving traffic directly to your product pages. Start with a small budget and closely monitor your results to ensure the best return on investment.
e commerce website using java
e commerce website using java and mysql
how to build an ecommerce website using java
How do I create an eCommerce website from scratch code?
Absolutely, here’s the information on building an e-commerce website with a comparison table included:
Building an E-commerce Website: Options Beyond Coding
While coding from scratch offers maximum control, other approaches can simplify the process:
Approach | Pros | Cons |
---|---|---|
E-commerce Platforms (Shopify, Wix eCommerce, WooCommerce) | * User-friendly interface with drag-and-drop features. * Pre-built functionalities like product listings, shopping carts, and payment gateways. * Easy to set up and manage. | * Limited customization compared to custom development. * Subscription or transaction fees may apply. * Might not be suitable for highly specialized needs. |
Open-Source E-commerce Solutions (Magento, PrestaShop) | * More flexibility and customization than pre-built platforms. * Free to download and use. | * Steeper learning curve for setup and maintenance. * Requires hosting and server management expertise. * May need additional development for specific functionalities. |
CMS with E-commerce Plugins (WordPress + WooCommerce, Drupal + Drupal Commerce) | * Flexibility in website design and content management. * Basic e-commerce functionalities with plugins. | * Requires some technical knowledge for plugins. * Might need additional plugins for advanced features. * Security updates for both CMS and plugin need monitoring. |
Headless E-commerce | * Maximum flexibility and customization. * Choice of front-end framework and back-end API/CMS. | * Requires significant development expertise and budget. * More complex to manage and maintain. |
Choosing the Right Approach
The best approach depends on your specific needs:
- Technical Skills: If you’re not comfortable with coding, consider platforms or CMS with plugins.
- Budget: Platforms and plugins often have lower upfront costs, while custom development and headless e-commerce require more investment.
- Website Complexity & Customization: Simpler stores might do well with platforms, while complex needs might require custom development or headless solutions.
Remember:
- Start with a clear plan: Define your needs and target audience.
- Prioritize core features: Focus on functionalities essential for your store.
- Focus on user experience: Make your website user-friendly and mobile-responsive.
- Consider future growth: Choose an approach that scales with your needs.
By evaluating your options and considering these factors, you can choose the best way to build your e-commerce website and start selling online!
ecommerce website using java spring boot
e commerce website in java
e commerce application java code
e commerce project in java source code
Related searches
- e commerce project in java source code
- online shopping project source code in java pdf
- e commerce website project with source code
- spring boot e-commerce project github
- e-commerce projects examples
- shopping-cart github
- e commerce project using spring boot + react js
- java projects with source code
Conclusion
And that’s a wrap, everyone! 🎉 By incorporating user authentication, a product catalog, and a shopping cart, you’re ready to kickstart your very own e-commerce platform. You hold the key to success, and your online store is just a few lines of Java source code away from becoming a reality.
Remember, the real magic unfolds when you bring all these elements together. Your users will appreciate the seamless experience, and you’ll be thrilled to witness your creation come to life. So, dive into coding, and witness your e-commerce aspirations soar. 🚀
Keep forging ahead, keep coding, and remember to enjoy the journey. You’ve got what it takes! 🌟
0 Comments