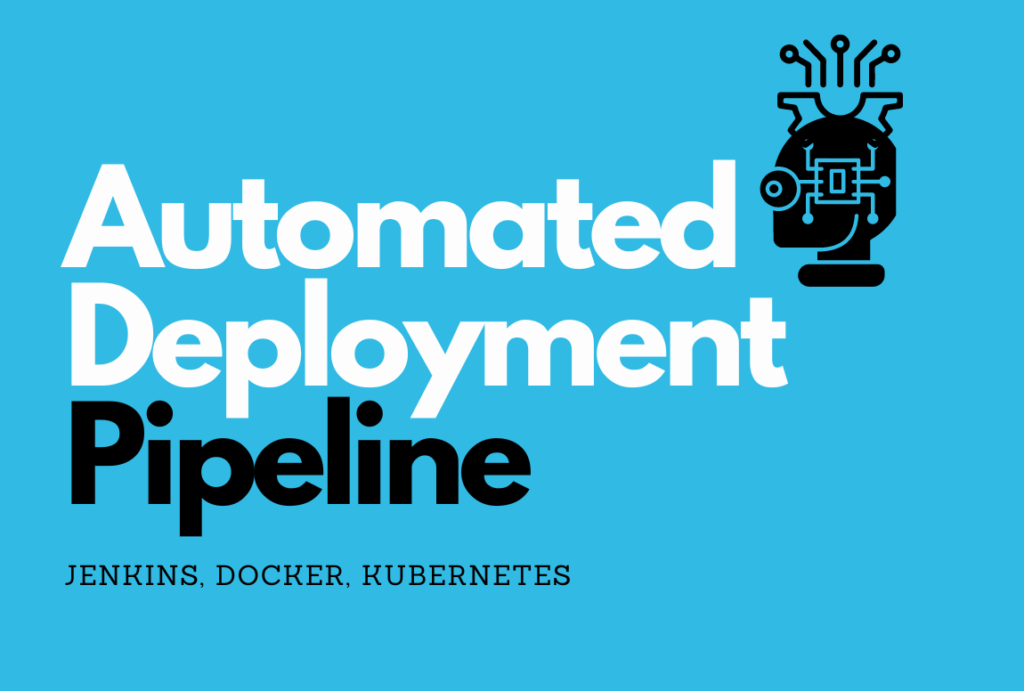
Table of Contents
Introduction
In today’s fast-paced software development world, it is essential to be able to deploy applications quickly and reliably. Automated Deployment Pipelines play a vital role in simplifying the process of building, testing, and deploying code. They ensure that software changes are implemented efficiently and with minimal need for manual intervention. In this article, we will guide you through the steps of creating an Automated Deployment Pipeline using well-known tools such as Jenkins, Docker, and Kubernetes.
The Elements of the Automated Deployment Pipeline
Code Repository
Start by storing your application code in a version control system like GitHub. This offers a centralized and structured approach to managing your codebase.
Jenkinsfile (Pipeline Script)
Jenkins, a popular automation server, enables you to define your deployment pipeline using code. In the Jenkinsfile, you can define stages to carry out different tasks such as code checkout, building, creating Docker images, and deploying to Kubernetes.
// Example Jenkinsfile
pipeline {
// Stages of the deployment pipeline
stages {
stage('Checkout') {
// Code checkout from the source code repository
steps {
checkout scm
}
}
stage('Build') {
// Build the application using Maven
steps {
script {
sh 'mvn clean package'
}
}
}
stage('Docker Build') {
// Build Docker image
steps {
script {
sh 'docker build -t your-image-name .'
}
}
}
stage('Docker Push') {
// Push Docker image to Docker Hub
steps {
script {
// Docker Hub credentials are used for authentication
withCredentials([usernamePassword(credentialsId: 'dockerhub-credentials', usernameVariable: 'DOCKER_USERNAME', passwordVariable: 'DOCKER_PASSWORD')]) {
sh 'docker login -u $DOCKER_USERNAME -p $DOCKER_PASSWORD'
sh 'docker push your-image-name'
}
}
}
}
stage('Deploy to Kubernetes') {
// Deploy the application to Kubernetes cluster
steps {
script {
sh 'kubectl apply -f kubernetes-deployment.yaml'
}
}
}
}
}
Dockerfile
- The Dockerfile is responsible for setting up the environment in which your application runs. It outlines the steps to package your application into a Docker image.
# Example Dockerfile
FROM openjdk:11-jre-slim
COPY target/your-app.jar /app/your-app.jar
CMD ["java", "-jar", "/app/your-app.jar"]
Kubernetes Deployment YAML
- The YAML file for Kubernetes Deployment outlines the specific instructions for deploying your application in a Kubernetes cluster.
# Example kubernetes-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: your-app
spec:
replicas: 3
selector:
matchLabels:
app: your-app
template:
metadata:
labels:
app: your-app
spec:
containers:
- name: your-app
image: your-image-name:latest
ports:
- containerPort: 8080
---
apiVersion: v1
kind: Service
metadata:
name: your-app-service
spec:
selector:
app: your-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: LoadBalancer
Jenkins Configuration
To configure Jenkins, you need to set it up to use the Jenkinsfile from your source code repository. Additionally, make sure to set up Jenkins credentials for Docker Hub so that you can push Docker images.
How do I automate Kubernetes deployment using Jenkins?
Yo, fam, let’s talk about automating Kubernetes deployment using Jenkins. I know you’re all about that hustle, trying to make your life easier with some automation magic. Well, buckle up ’cause we’re about to dive into it.
Setting Up Automation with Jenkins:
Step 1: Configure Jenkins:
First things first, you gotta get Jenkins up and running. Install that bad boy and set up your pipelines like a boss. Jenkins is gonna be your ride or die when it comes to automating your Kubernetes deployments.
Step 2: Integrate with Kubernetes:
Next up, you gotta establish a connection between Jenkins and Kubernetes. It’s like introducing your two besties at a party. You want them to vibe together seamlessly. Set up your Kubernetes credentials in Jenkins so it can talk to your clusters like they’re old pals.
Step 3: Write Your Pipeline Script:
Now comes the fun part. You gotta write your pipeline script in Jenkins to automate the deployment process. Think of it like giving Jenkins a to-do list, and it’s gonna handle the rest like a champ. Define your stages, specify your containers, and let Jenkins work its magic.
Step 4: Test and Deploy:
Last but not least, you gotta test your pipeline to make sure everything’s running smooth like butter. Ain’t nobody got time for janky deployments. Once you’re confident everything’s golden, sit back and watch Jenkins do its thing. Automatic Kubernetes deployments, baby!
So, is automating Kubernetes deployment possible with Jenkins? You bet your bottom dollar it is. With the right setup and a little bit of know-how, you can have Jenkins handling your deployments like a pro. So, what are you waiting for? Get out there and automate like there’s no tomorrow!
How to automate Docker build and auto deploy using Jenkins?
Alright, fam, let’s talk about automating Docker build and auto deploy using Jenkins. I know you’re tired of manually building and deploying your Docker containers like it’s the Stone Age. Well, fear not, ’cause Jenkins is here to save the day.
Getting Started with Docker and Jenkins
Step 1: Set Up Jenkins
First things first, you gotta get Jenkins set up on your system. Install it, configure it, and get it ready to rock and roll. Jenkins is gonna be your wingman on this automation journey, so treat it right.
Step 2: Integrate with Docker
Next up, you gotta integrate Jenkins with Docker. It’s like introducing two long-lost friends who were meant to be together. Configure your Jenkins pipeline to pull your Docker images, build them, and push them to your registry like a boss.
Step 3: Write Your Pipeline Script
Now comes the fun part: writing your pipeline script in Jenkins. Think of it like giving Jenkins a recipe for your Docker containers, and it’s gonna follow it to a T. Define your stages, specify your Dockerfile, and let Jenkins do its thing.
Step 4: Sit Back and Relax
Once you’ve got everything set up, it’s time to kick back and relax. Jenkins is gonna handle the heavy lifting from here on out. It’ll automatically build and deploy your Docker containers whenever you push a new commit. Talk about efficiency!
So, is automatic deployment possible in Jenkins? You better believe it. With Jenkins by your side, you can automate just about anything, including Docker builds and deployments. So why waste time doing things manually when you can let Jenkins do the work for you? Automate like a pro and watch your productivity soar.
How do I establish a connection between Jenkins and Kubernetes?
Aight, fam, let’s talk about establishing a connection between Jenkins and Kubernetes. You’re all about that automation life, and you know that having Jenkins and Kubernetes working together is the key to success. So, let’s break it down real quick.
Connecting Jenkins and Kubernetes
Step 1: Set Up Kubernetes Credentials
First things first, you gotta set up your Kubernetes credentials in Jenkins. It’s like giving Jenkins the keys to the kingdom so it can talk to your Kubernetes clusters without any hiccups. Configure your credentials with the appropriate permissions, and you’re good to go.
Step 2: Install Kubernetes Plugin
Next up, you gotta install the Kubernetes plugin for Jenkins. This plugin is gonna be your go-to tool for interacting with your Kubernetes clusters from Jenkins. It’ll handle all the heavy lifting when it comes to spinning up pods, deploying containers, and managing resources.
Step 3: Configure Jenkins Pipeline
Now comes the fun part: configuring your Jenkins pipeline to work with Kubernetes. You gotta specify which Kubernetes cluster you wanna target, define your deployment strategy, and set up any additional parameters you need. Once you’ve got everything dialed in, Jenkins will handle the rest like a pro.
Step 4: Test Your Connection
Last but not least, you gotta test your connection to make sure everything’s running smoothly. Run a few test builds, deploy some test containers, and make sure Jenkins is able to communicate with your Kubernetes clusters without any issues. If everything checks out, you’re good to go!
So, how do you establish a connection between Jenkins and Kubernetes? With a little bit of configuration and some know-how, you can have Jenkins and Kubernetes working together like peanut butter and jelly. So why wait? Get out there and start automating like a boss! π
Automated Deployment Pipeline 2024: Jenkins, Docker, Kubernetes, and GitHub
Yo, fam, let’s talk about the future of automated deployment pipelines in 2024. We’re living in a fast-paced world where every second counts, and ain’t nobody got time to be messing around with manual deployments. That’s where Jenkins, Docker, Kubernetes, and GitHub come into play.
The Ultimate Combo: Jenkins, Docker, Kubernetes, and GitHub:
Listen up, ’cause I’m about to drop some knowledge on you. In 2024, automated deployment pipelines are the name of the game, and Jenkins is the captain of the ship. With Jenkins leading the charge, you can set up a killer pipeline that’ll have your code flying from GitHub to your Kubernetes server faster than you can say “automation.”
But hold up, we can’t forget about Docker and Kubernetes. These two are like the dynamic duo of containerization and orchestration, and when you pair them with Jenkins, it’s like adding rocket fuel to your deployment pipeline. Docker containers make it easy to package up your code and dependencies, while Kubernetes handles the heavy lifting of scaling and managing your applications.
And let’s not overlook GitHub. As the home base for your code, GitHub plays a crucial role in your automated deployment pipeline. With Jenkins pulling code from GitHub, running tests, building Docker images, and deploying to Kubernetes, you’ve got yourself a seamless workflow that’s as smooth as butter.
The Power of Integration:
Now, you might be wondering how all these pieces fit together. Well, my friend, that’s the beauty of it. Jenkins orchestrates the entire process, pulling code from GitHub, kicking off builds in Docker, and deploying to Kubernetes with just a few lines of code. It’s like watching a symphony orchestra, with Jenkins as the conductor, Docker and Kubernetes as the musicians, and GitHub as the sheet music.
But don’t just take my word for it. Let’s break it down step by step with a killer example.
Jenkins-Kubernetes Pipeline Example with GitHub Integration
Alright, fam, it’s time to dive into a real-world example of a Jenkins-Kubernetes pipeline with GitHub integration. Strap in ’cause we’re about to take your deployment game to the next level.
Step-by-Step Breakdown:
- Setup Jenkins Pipeline: First things first, you gotta set up your Jenkins pipeline to pull code from GitHub. Configure your Jenkinsfile with the necessary stages for building, testing, and deploying your application.
docker run --name myjenkins-container -p 8080:8080 -p 50000:50000 -v /var/jenkins_home jenkins
- Integrate Docker: Next up, integrate Docker into your pipeline. Use Jenkins to build Docker images from your code and push them to your Docker registry.
- Connect with Kubernetes: Now comes the fun part: connecting Jenkins with Kubernetes. Configure Jenkins to deploy your Docker images to your Kubernetes clusters using Kubernetes manifests.
- GitHub Integration: Last but not least, don’t forget about GitHub. Make sure your Jenkins pipeline is triggered automatically whenever you push new code to your GitHub repository.
And there you have it, folks: a seamless Jenkins-Kubernetes pipeline example with GitHub integration. With Jenkins, Docker, Kubernetes, and GitHub working together in perfect harmony, you can automate your deployments like a pro. So why wait? Dive in and start building your own automated deployment pipeline today! π
Create a simple HelloWorld Application in NodeJs
Alright, fam, let’s spice things up and create a simple HelloWorld application in NodeJs. Get ready to flex those coding muscles and spread some cheer with your very own Hello World app!
Step 1: Set Up Your Workspace
First things first, we gotta create a new folder to house our masterpiece. Whip out your coding cape and make a folder called “jenkins-deploy-nodejs” on your local system. This is gonna be the home base for our epic adventure.
Step 2: Install Dependencies
Now it’s time to get our hands dirty with some code. In your freshly minted “jenkins-deploy-nodejs” folder, fire up your terminal and run this command:
npm install express nodemon
This will install the magic ingredients we need to make our Hello World dreams come true.
Step 3: Write Some Code
Alright, now for the fun part. Let’s create an “index.js” file in our folder and fill it with some code that’ll make the world smile:
var express = require('express');
var app = express();
app.get('/', function(req, res){
res.send("Welcome to the Hello World App!");
});
app.listen(3000, () => {
console.log("App is running on port 3000")
});
module.exports = app;
Step 4: Level Up Your Package.json
Open up your “package.json” file and add this line to the “scripts” object:
"start": "nodemon index.js"
This will let us run our app with just a simple command. Easy peasy!
Step 5: Test It Out
Alright, moment of truth. Let’s fire up our app and see if it’s ready to rock and roll. Run this command in your terminal:
npm run start
And boom, there it is! Your very own Hello World app, ready to spread joy to the masses. Give yourself a pat on the back, ’cause you just made magic happen. π
Creating Docker File:
Alright, fam, now that we’ve got our NodeJs Hello World app all set up and looking fly, it’s time to take things to the next level with Docker. We’re gonna containerize this bad boy and make it even easier to deploy. Let’s dive in!
Step 1: Dockerizing Your App
First things first, let’s create a Dockerfile and give our app a cozy new home:
FROM node:latest
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "index.js"]
This Dockerfile is like the blueprint for our container. It tells Docker how to build our image and what commands to run when it’s fired up.
Step 2: Kubernetes Manifest Files
Now that we’ve got our Docker image sorted, it’s time to get Kubernetes in on the action. We’re gonna create some manifest files to tell Kubernetes how to deploy and manage our app.
Deployment Manifest (deployment.yml)
apiVersion: apps/v1
kind: Deployment
metadata:
name: helloworld-app
spec:
replicas: 2
selector:
matchLabels:
app: helloworld-app
template:
metadata:
labels:
app: helloworld-app
spec:
containers:
- name: helloworld-app
image: <your_docker_hub_image_url>
imagePullPolicy: Always
ports:
- containerPort: 3000
Service Manifest (service.yml)
apiVersion: v1
kind: Service
metadata:
name: node-app-service
spec:
selector:
app: helloworld-app
ports:
- protocol: TCP
port: 80
targetPort: 3000
nodePort: 31258 /* will allocate a port from a range (default: 30000-32767)*/
type: NodePort
Step 3: Jenkins Pipeline
And now for the grand finale, let’s tie it all together with a Jenkins pipeline. We’re gonna automate the whole shebang, from building our Docker image to deploying it to Kubernetes like a boss.
Jenkins Pipeline (Jenkinsfile)
pipeline {
agent any
stages {
stage('Git CheckOut') {
steps {
cleanWs()
checkout scmGit(branches: [[name: '*/main']], extensions: [], userRemoteConfigs: [[credentialsId: '<your_github_credential_id>', url: '<your_github_repository_url>']])
}
}
stage('Build docker image') {
steps {
script {
sh 'docker build -t helloworld/node-app:latest .'
}
}
}
stage('Push image to Docker Hub') {
steps {
script {
withCredentials([string(credentialsId: '<your_dockerhub_credential_id>', variable: 'dockerpwd')]) {
sh 'docker login -u <your_dockerhub_username> -p ${dockerpwd}'
}
sh 'docker push helloworld/node-app:latest'
}
}
}
stage('Deploy to k8s') {
steps {
script {
kubeconfig(credentialsId: 'mykubeconfig', serverUrl: 'https://172.31.54.73:8443') {
sh 'kubectl apply -f deployment.yml'
sh 'kubectl rollout restart -f deployment.yml'
sh 'kubectl apply -f service.yml'
}
}
}
}
}
}
And there you have it, folks! A fully automated deployment pipeline using Jenkins, Docker, Kubernetes, and GitHub. Sit back, relax, and watch the magic happen as your app goes from code to production in no time flat. π
Deployment Pipeline
Git CheckOut Stage
In this groovy stage of our Jenkins Pipeline, we’re diving headfirst into the GitHub repository universe. We’ll grab hold of the repository URL you’ve provided and pull down all the juicy files waiting for us π.
Build Docker Image Stage
Time to roll up our sleeves and get down to Docker business! This stage is where the magic happens. We’ll use the provided Dockerfile to craft our masterpiece, tagging it with the name helloworld/node-app:latest. Get ready to witness some containerization wizardry! π³
Push Image to Docker Hub Stage
Next up, we’re taking our freshly minted Docker image and giving it a one-way ticket to the Docker Hub registry. This stage is all about sharing the love and making our image available to the world. Get those virtual passports ready, ’cause we’re going global! π
Deploy to k8s Stage
And now, it’s time to unleash our creation upon the Kubernetes kingdom! This stage is where the rubber meets the road as we apply the Kubernetes deployment YAML file, paving the way for our Docker image to take its rightful place in the cluster. It’s deployment day, baby! π
Testing Your Application
Once the pipeline has worked its magic and reached a successful status, it’s time to put your application to the test. Head on over to your GitHub repository and ensure all the files are pushed and ready to roll. Then, swing by your Jenkins pipeline to bask in the glory of success.
If all looks good, hop over to your AWS EC2 instance console, grab the public IP address of your Minikube-installed instance, and paste it into your browser alongside port 31258. Sit back, relax, and behold the fruits of your labor as your application springs to life before your very eyes! π
Conclusion
By integrating Jenkins, Docker, and Kubernetes into an Automated Deployment Pipeline, you can achieve a strong and efficient continuous deployment process. This pipeline automates important stages of the software delivery lifecycle, allowing for faster release cycles, improved collaboration, and increased productivity within your development team. It’s important to customize the pipeline to meet your specific requirements and continuously improve it to optimize the deployment process. With this foundation, you’ll be well on your way to embracing DevOps principles and practices in your software development workflow.
0 Comments