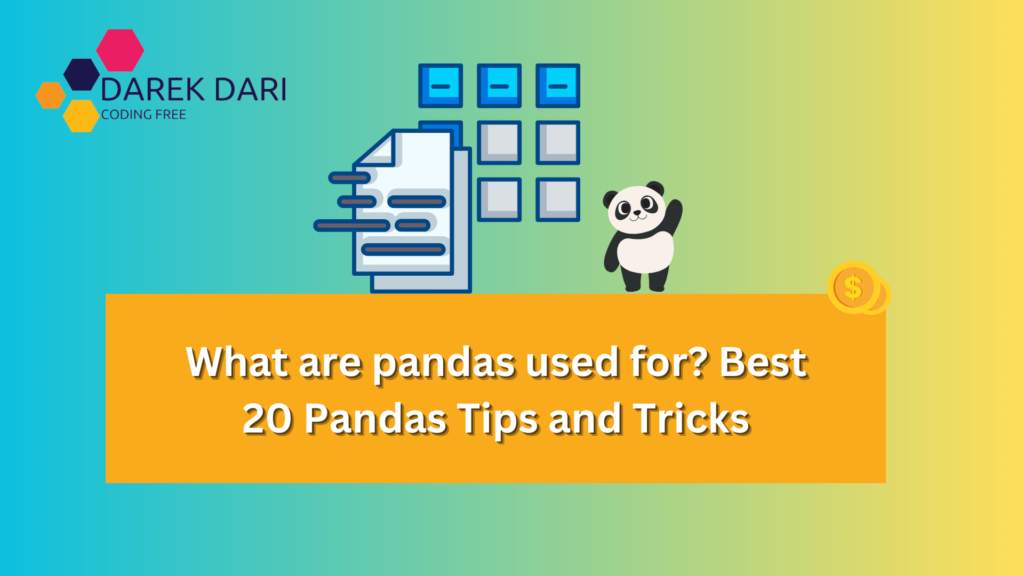
Table of Contents
Introduction
Welcome to the realm of Pandas, where data manipulation meets expertise! Let’s dive into the world of Pandas, a Python library that is transforming the way we manage and analyze data.
Also, check:
- Lesson 1: What are pandas used for? Pandas Tips and Tricks
- Lesson 2: What does pandas DataFrame mean?
- Lesson 3: Charts and Diagrams in Colab with Matplotlib
- Lesson 4: Interactive Forms in Google Colab with Python vs. R
- Lesson 5: Working with Local Files, Google Drive, Google Sheets, and Google Cloud Storage in Google Colab
What is Pandas?
Pandas is not your average library—it’s a game-changer in the field of data science and analysis. Short for “Panel Data,” Pandas offers a powerful toolkit for data manipulation, exploration, and analysis, making it essential for anyone dealing with structured data.
Your Data Swiss Army Knife
Think of Pandas as your go-to Swiss Army knife for all things data-related. Whether you’re loading datasets, cleaning up messy data, performing intricate transformations, or analyzing patterns, Pandas has all the tools you need with its array of functions and methods.
Pandas Key Features
Pandas introduces two main data structures: DataFrame and Series. A DataFrame acts like a supercharged spreadsheet, enabling you to store and manipulate data in a tabular format, while a Series represents a single column of data with built-in indexing.
With Pandas, you have the flexibility to manipulate your data in any way you desire. Whether you need to filter rows, add new columns, or summarize data, Pandas simplifies the process with its user-friendly syntax and robust capabilities.
Why Pandas?
So, why opt for Pandas over other tools? Its seamless integration with Python makes it a top choice for Python enthusiasts. Moreover, its speed and efficiency make it well-suited for handling large datasets effortlessly.
Pandas isn’t just for data scientists—it’s for anyone looking to extract insights from data. From business analysts to researchers to hobbyists, Pandas equips users to derive valuable insights and make well-informed decisions.
What are pandas used for?
So, you might have heard about this thing called Pandas in the world of Python. What’s it all about? Well, imagine you’ve got a bunch of data lying around, maybe in a spreadsheet or a database. Pandas swoops in like a superhero to help you make sense of it all.
Cleaning Up the Mess
One of the coolest things about Pandas is how it can handle messy data. You know, missing values, weird outliers, all that jazz. It’s like having a magical broom that sweeps away the junk and leaves you with nice, tidy data.
Let’s Go Exploring
Once your data is all spick and span, Pandas helps you go on an adventure of exploration. You can peek into your dataset, check out the stats, and even spot trends or patterns hiding in the numbers. It’s like being an explorer in the wild world of data.
Bend it, Twist it, Shape it
Pandas is like Play-Doh for data. You can twist and turn your dataset any which way you like. Merge it with another dataset, reshape it into something new, or group it together for some serious analysis. The possibilities are endless!
Getting Visual
Okay, so Pandas doesn’t do fancy graphics on its own, but it’s best buddies with Matplotlib and Seaborn, which are like the artists of the data world. Together, they can turn your boring numbers into beautiful charts and graphs that tell a story.
In and Out, Shake it All About
Importing data from different sources? Exporting your analysis for others to see? Pandas has your back. It can read and write data in all sorts of formats, from CSV to Excel to JSON. It’s like the Swiss Army knife of data handling.
Bottom Line
Pandas is the go-to tool for anyone wrangling data in Python. Whether you’re a data scientist, analyst, or just a curious soul digging into some numbers, Pandas is your loyal companion on the data journey.
Creating a DataFrame from Lists
Code:
import pandas as pd
last_names = ['Connor', 'Connor', 'Reese']
first_names = ['Sarah', 'John', 'Kyle']
df = pd.DataFrame({
'first_name': first_names,
'last_name': last_names,
})
df
Explanation:
- Importing Pandas: We start by importing the pandas library, which is essential for creating and manipulating data structures like DataFrames.
- Creating Lists: We have two lists,
last_names
andfirst_names
, containing last and first names, respectively. - Creating DataFrame: Using these lists, we create a DataFrame with two columns: ‘first_name’ and ‘last_name’. The
pd.DataFrame
function takes a dictionary where the keys are column names and the values are the lists. - Displaying DataFrame: Simply typing
df
at the end outputs the DataFrame.
Renaming Columns
Code:
import pandas as pd
df = pd.DataFrame({
'Year': [2016, 2015, 2014, 2013, 2012],
'Top Animal': ['Giant panda', 'Chicken', 'Pig', 'Turkey', 'Dog']
})
df.rename(columns={
'Year': 'Calendar Year',
'Top Animal': 'Favorite Animal',
}, inplace=True)
df
Explanation:
- Creating DataFrame: We create a DataFrame with columns ‘Year’ and ‘Top Animal’.
- Renaming Columns: The
rename
method is used to change column names. Thecolumns
parameter takes a dictionary where the keys are old names and the values are new names. Theinplace=True
parameter makes the changes directly to the DataFrame.
Querying with Regex
Code:
import pandas as pd
df = pd.DataFrame({
'first_name': ['Sarah', 'John', 'Kyle', 'Joe'],
'last_name': ['Connor', 'Connor', 'Reese', 'Bonnot'],
})
df[df.last_name.str.match('.*onno.*')]
Explanation:
- Creating DataFrame: Another DataFrame is created with ‘first_name’ and ‘last_name’ columns.
- Regex Query: The
str.match
method is used to find rows where ‘last_name’ matches a regex pattern. Here,.*onno.*
looks for any string containing ‘onno’.
Querying by Variable Value
Code:
import pandas as pd
df = pd.DataFrame({
'first_name': ['Sarah', 'John', 'Kyle'],
'last_name': ['Connor', 'Connor', 'Reese'],
})
foo = 'Connor'
df.query('last_name == @foo')
Explanation:
- Creating DataFrame: We create a DataFrame with first and last names.
- Variable Value Query: We set a variable
foo
to ‘Connor’ and usedf.query
to filter rows where ‘last_name’ equals the value offoo
. The@
symbol is used to reference the variable in the query string.
Variable as Column Name
Code:
import pandas as pd
df = pd.DataFrame({
'first_name': ['Sarah', 'John', 'Kyle'],
'last_name': ['Connor', 'Connor', 'Reese'],
})
column_name = 'first_name'
df.query(f"`{column_name}` == 'John'")
Explanation:
- Creating DataFrame: Same DataFrame creation as before.
- Dynamic Column Name Query: We set
column_name
to ‘first_name’ and use it in a query to filter rows where the ‘first_name’ column equals ‘John’. The{}
braces are used for string interpolation.
Query by Timestamp
Code:
import pandas as pd
df = pd.DataFrame({
'time': ['2022-09-14 00:52:00-07:00', '2022-09-14 00:52:30-07:00',
'2022-09-14 01:52:30-07:00'],
'letter': ['A', 'B', 'C'],
})
df['time'] = pd.to_datetime(df.time)
df.query('time >= "2022-09-14 00:52:30-07:00"')
Explanation:
- Creating DataFrame: This DataFrame has ‘time’ and ‘letter’ columns.
- Converting to Datetime: The ‘time’ column is converted to datetime format using
pd.to_datetime
. - Timestamp Query: We filter rows where the ‘time’ column is greater than or equal to a specific timestamp.
Filtering by Time Range
Code:
import pandas as pd
df = pd.DataFrame({
'time': ['2022-09-14 00:52:00-07:00', '2022-09-14 00:52:30-07:00',
'2022-09-14 01:52:30-07:00'],
'letter': ['A', 'B', 'C'],
})
df['time'] = pd.to_datetime(df.time)
begin_ts = '2022-09-14 00:52:00-07:00'
end_ts = '2022-09-14 00:54:00-07:00'
df.query('@begin_ts <= time < @end_ts')
Explanation:
- Creating DataFrame: Same as before.
- Time Range Query: We define
begin_ts
andend_ts
as the start and end timestamps and use them in a query to filter rows within this range.
Filtering by DatetimeIndex with .loc[]
Code:
import pandas as pd
df = pd.DataFrame({
'time': ['2022-09-14 00:52:00-07:00', '2022-09-14 00:52:30-07:00',
'2022-09-14 01:52:30-07:00'],
'letter': ['A', 'B', 'C'],
})
df['time'] = pd.to_datetime(df.time)
df.set_index('time', inplace=True)
df.loc['2022-09-14':'2022-09-14 00:53']
Explanation:
- Creating DataFrame: Same as before.
- Setting Index: We set the ‘time’ column as the index using
set_index
. - DatetimeIndex Query: Using
.loc[]
, we filter rows by specifying a time range.
Filtering by TimeDelta
Code:
import pandas as pd
df = pd.DataFrame({
'time': ['2022-09-14 00:52:00-07:00', '2022-09-14 00:52:30-07:00',
'2022-09-14 01:52:30-07:00'],
'letter': ['A', 'B', 'C'],
})
df['time'] = pd.to_datetime(df.time)
def rows_in_time_range(df, time_column, start_ts_str, timedelta_str):
start_ts = pd.Timestamp(start_ts_str).tz_localize('US/Pacific')
end_ts = start_ts + pd.to_timedelta(timedelta_str)
return df.query("@start_ts <= {0} < @end_ts".format(time_column))
rows_in_time_range(df, 'time', '2022-09-14 00:00', '52 minutes 31 seconds')
Explanation:
- Creating DataFrame: Same as before.
- TimeDelta Query Function: This function filters rows within a specific time range calculated using
start_ts_str
andtimedelta_str
.
Describing Timestamp Values
Code:
import pandas as pd
df = pd.DataFrame({
'time': ['2022-09-14 00:52:00-07:00', '2022-09-14 00:52:30-07:00',
'2022-09-14 01:52:30-07:00'],
'letter': ['A', 'B', 'C'],
})
df['time'] = pd.to_datetime(df.time)
df['time'].describe(datetime_is_numeric=True)
Explanation:
- Creating DataFrame: Same as before.
- Descriptive Statistics: The
describe
method withdatetime_is_numeric=True
provides descriptive statistics for the ‘time’ column, treating it as numeric.
Exploding a Dictionary Column
Code:
import pandas as pd
df = pd.DataFrame({
'date': ['2022-09-14', '2022-09-15', '2022-09-16'],
'letter': ['A', 'B', 'C'],
'dict' : [{ 'fruit': 'apple', 'weather': 'aces'},
{ 'fruit': 'banana', 'weather': 'bad'},
{ 'fruit': 'cantaloupe', 'weather': 'cloudy'}],
})
pd.concat([df.drop(['dict'], axis=1), df['dict'].apply(pd.Series)], axis=1)
Explanation:
- Creating DataFrame: This DataFrame includes a column with dictionary
- Column with Dictionaries: The DataFrame includes a ‘dict’ column where each cell is a dictionary.
- Exploding the Dictionary: Using
df['dict'].apply(pd.Series)
converts the dictionaries into separate columns.pd.concat
is then used to concatenate these new columns back to the original DataFrame, dropping the original ‘dict’ column.
Extracting Values with Regex
Code:
import pandas as pd
df = pd.DataFrame({
'request': ['GET /index.html?baz=3', 'GET /foo.html?bar=1'],
})
df['request'].str.extract('GET /([^?]+)\?', expand=True)
Explanation:
- Creating DataFrame: A DataFrame with a ‘request’ column containing HTTP request strings.
- Regex Extraction: The
str.extract
method uses a regex pattern to extract parts of the string. Here,GET /([^?]+)\?
extracts the path of the request (everything between ‘GET /’ and ‘?’).
Convert String to Timestamp
Code:
import pandas as pd
pd.Timestamp('9/27/22').tz_localize('US/Pacific')
Explanation:
- String to Timestamp (Date Only): Converts a date string to a timestamp and localizes it to the ‘US/Pacific’ timezone.
Code (Including Time):
import pandas as pd
pd.Timestamp('9/27/22 06:59').tz_localize('US/Pacific')
Explanation:
- String to Timestamp (Including Time): Converts a date and time string to a timestamp and localizes it to the ‘US/Pacific’ timezone.
Creating a TimeDelta
Code:
import pandas as pd
pd.to_timedelta(1, unit='h')
Explanation:
- Basic TimeDelta: Creates a
Timedelta
object representing 1 hour.
Code (More Complex):
import pandas as pd
pd.Timedelta(days=2)
Explanation:
- Days TimeDelta: Creates a
Timedelta
object representing 2 days.
Code (Even More Complex):
import pandas as pd
pd.Timedelta('2 days 2 hours 15 minutes 30 seconds')
Explanation:
- Detailed TimeDelta: Creates a
Timedelta
object from a string representing 2 days, 2 hours, 15 minutes, and 30 seconds.
Replacing NaN Values
Code:
import numpy as np
import pandas as pd
df = pd.DataFrame({
'dogs': [5, 10, np.nan, 7],
})
df['dogs'].replace(np.nan, 0, regex=True)
Explanation:
- Creating DataFrame: A DataFrame with a ‘dogs’ column containing some NaN values.
- Replacing NaN: The
replace
method replaces NaN values with 0. Theregex=True
parameter is not necessary here, but it doesn’t affect the outcome in this case.
Dropping Duplicate Rows
Code:
import pandas as pd
df = pd.DataFrame({
'first_name': ['Sarah', 'John', 'Kyle', 'Joe'],
'last_name': ['Connor', 'Connor', 'Reese', 'Bonnot'],
})
df.set_index('last_name', inplace=True)
df.loc[~df.index.duplicated(), :]
Explanation:
- Creating DataFrame: A DataFrame with ‘first_name’ and ‘last_name’ columns.
- Setting Index: The ‘last_name’ column is set as the index using
set_index
. - Dropping Duplicates:
~df.index.duplicated()
creates a boolean mask whereFalse
corresponds to duplicated index values. The.loc[]
method uses this mask to select only unique rows.
With these explanations, you should have a solid understanding of each code snippet and how to use these techniques in your data analysis projects.
What is pandas and NumPy in Python?
Pandas and NumPy: The Dynamic Duo of Data
So, you’re diving into Python and everyone’s raving about Pandas and NumPy. What’s the deal with these two?
NumPy: The Brains Behind the Numbers
NumPy is like the foundation of the entire Python data world. It stands for “Numerical Python,” and it’s all about crunching numbers, working with arrays, and delving into the world of math. Think of it as the strong backbone that makes everything else work seamlessly.
With NumPy, you can perform some pretty impressive number magic. Need to do complex math operations on arrays? NumPy is your go-to. Dealing with multi-dimensional data? NumPy has got your back.
Pandas: The Data Whisperer
Now, let’s talk about Pandas. This library is all about mastering data like a pro. It’s like the wizard of data manipulation, transforming messy datasets into gold.
Pandas builds upon the foundation of NumPy, adding even more power to the mix. It introduces two key players: DataFrame and Series. A DataFrame is like a fancy table where you can store and analyze your data, while a Series is like a single column of that table.
With Pandas, you’re in control of your data. Need to slice it, dice it, filter it, or group it? Pandas has your back with a whole bag of tricks.
Why They’re a Perfect Match
So, why do Pandas and NumPy go together like peanut butter and jelly? Well, Pandas heavily relies on NumPy behind the scenes. A lot of Pandas’ data wizardry is powered by NumPy’s efficient array processing.
Moreover, they complement each other seamlessly. You can effortlessly switch between Pandas DataFrames and NumPy arrays, combining their strengths to tackle any data challenge.
Are pandas easy to learn?
Ease of Learning | Description |
---|---|
Beginner-Friendly | Pandas may seem daunting initially, particularly for those new to Python or data manipulation. However, numerous beginner-friendly tutorials and resources are available to facilitate the learning process. |
Python Knowledge | While not mandatory, having a basic understanding of Python syntax and data structures can significantly ease the learning curve. Familiarity with Python allows learners to grasp Pandas concepts more readily and apply them effectively. |
Practice | Like mastering any skill, practice is essential for proficiency. Beginners can start with straightforward tasks such as loading datasets, manipulating dataframes, and performing basic statistical analyses. As competence grows, learners can tackle more complex operations and scenarios. |
Documentation | Pandas offers comprehensive documentation complete with numerous examples and explanations. Utilizing this resource allows learners to delve into Pandas functionalities, understand their intricacies, and apply them confidently in real-world scenarios. |
Community Support | The Python community is renowned for its helpfulness and inclusivity. Should learners encounter obstacles or have queries, various forums and communities provide platforms for seeking guidance and assistance from experienced users, fostering a supportive and collaborative learning environment. |
Although Pandas can be difficult at first, there are plenty of resources available for beginners, a helpful Python community, and chances for hands-on learning.
By staying committed and persistent, anyone interested in data can easily become proficient in Pandas.
Is pandas a library or package?
Pandas is an incredible library in Python. In the Python world, the terms “library” and “package” are often used interchangeably to describe a group of modules or functions that enhance the capabilities of Python.
These libraries/packages consist of reusable code that serves specific purposes like data manipulation, mathematical operations, web development, and more.
Pandas, in particular, is an exceptional open-source library for data analysis and manipulation in Python. It offers high-level data structures like DataFrame and Series, along with a vast array of tools for cleaning, exploring, manipulating, and analyzing data.
To sum it up, while both “library” and “package” can be used to describe collections of code in Python, “library” is the more commonly used term when referring to Pandas.
Conclusion
To sum up, Pandas is an incredibly useful library for data analysis and manipulation in Python. Although it may seem challenging at first, there are plenty of resources available to help beginners understand its fundamentals. As you gain more experience with Python, you’ll find that learning Pandas becomes easier.
It’s important to practice regularly and start with simple tasks before moving on to more complex operations. This approach allows you to gradually build your skills and confidence over time.
The Python community is also very supportive and offers many opportunities for collaboration and assistance.
If you ever need guidance or advice, you can rely on the community to help you out. Additionally, Pandas’ integration with other Python libraries and tools makes it even more versatile and useful for various data-related tasks and projects.
Although Pandas may appear intimidating initially, it is a foundational library in the Python data ecosystem. With its extensive capabilities and strong community support, investing time in mastering Pandas is definitely worthwhile if you want to excel in data analysis and manipulation.
6 Comments
Lesson 2: What Does Pandas DataFrame Mean? Best Tricks · May 21, 2024 at 8:57 pm
[…] I advice you to read Lesson 1: What are pandas used for? Pandas Tips and Tricks before delving in the lesson […]
Lesson 3: Best Matplotlib Charts And Diagrams In Colab · May 22, 2024 at 11:08 am
[…] Lesson 1: What are pandas used for? Pandas Tips and Tricks […]
Lesson 4: Best Forms In Google Colab With Python Vs R · May 22, 2024 at 11:47 am
[…] Lesson 1: What are pandas used for? Pandas Tips and Tricks […]
Lesson 5: Best Guide Local Files, Drive, Sheets, And Cloud · May 22, 2024 at 12:20 pm
[…] Lesson 1: What are pandas used for? Pandas Tips and Tricks […]
Lesson 6: Best BigQuery With Pandas Guide · May 22, 2024 at 9:26 pm
[…] Lesson 1: What are pandas used for? Pandas Tips and Tricks […]
Machine Learning Project 1: Honda Motor Stocks Best Prices · May 23, 2024 at 6:38 pm
[…] Lesson 1: What are pandas used for? Pandas Tips and Tricks […]