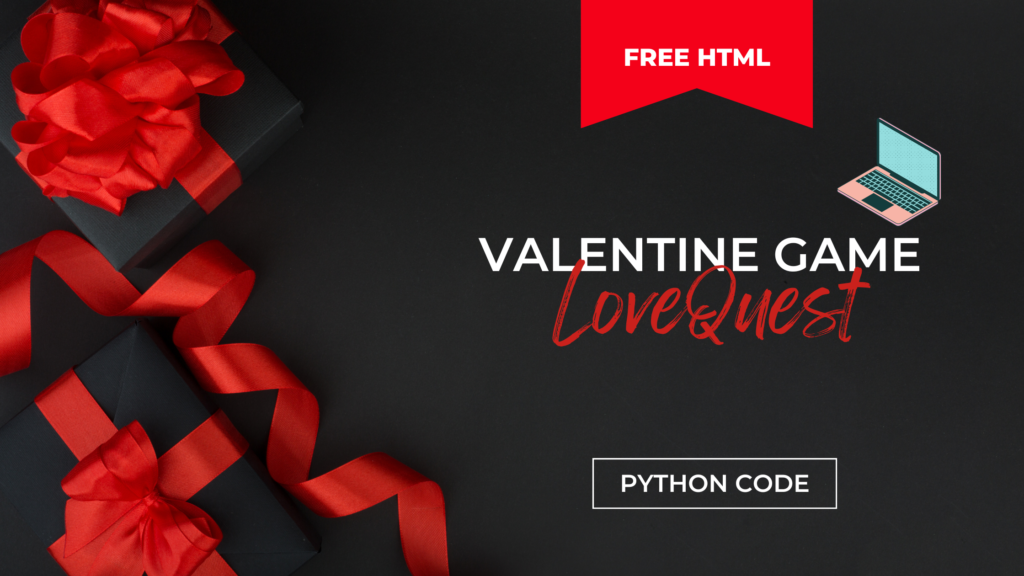
Table of Contents
Now get a Valentine Game 2024 with Source Code for Free. LoveQuest goes beyond being just a web application; it’s a charming and enchanting experience designed for couples who want to infuse their relationship with a sprinkle of magic. Drawing inspiration from the captivating essence of Valentine’s Day, LoveQuest invites you to embark on a delightful journey filled with sweet messages, romantic gestures, and heartfelt notes—all within the cozy confines of your web browser.
Welcome to LoveQuest!
The adventure of LoveQuest begins with a warm and inviting greeting on the landing page. A visually stunning interface entices users to embark on their romantic escapade. By clicking the “Start LoveQuest” button, you’ll set off on a voyage brimming with love-themed questions, heartwarming messages, and special tasks that will deepen the bond with your beloved.
Valentine Game Python Code
import time
def display_intro():
print("************************************")
print(" Welcome to LoveQuest: A Romantic Adventure!")
print("************************************\n")
print("Answer the questions correctly and complete special tasks to reveal heartfelt messages for your sweetheart.\n")
def ask_question(question, correct_answer, romantic_message):
print(f"\n💖 {question}")
user_answer = input("Your answer: ").lower()
if user_answer == correct_answer:
print("Correct! 💕\n")
return romantic_message
else:
print("Incorrect. Try again! 💔\n")
return ""
def perform_task(task, reward):
print(f"\n🌟 {task}")
input("Press Enter when completed...")
print(f"Task completed! {reward}\n")
return reward
def play_valentines_day_game():
questions = [
("What's the sweetest nickname you call each other? ", "sweetheart", "You'll always be my sweetest sweetheart."),
("Where did you go on your first date? ", "moonlit park", "Our first date was like a scene from a moonlit park, magical and unforgettable."),
("What's your favorite romantic movie to watch together? ", "the notebook", "Our love story is even more beautiful than 'The Notebook'."),
("What's your favorite love song? ", "unchained melody", "Our love is like an 'Unchained Melody,' timeless and full of passion."),
("What's your dream romantic getaway destination? ", "paris", "Just like Paris, our love is filled with romance and enchantment."),
]
romantic_messages = []
# Additional tasks
tasks = [
("Write a sweet note to your sweetheart and read it aloud.", "Your words are as beautiful as your heart."),
("Send a virtual hug to your sweetheart right now.", "Even across the distance, I feel your warmth."),
]
for question, correct_answer, message in questions:
romantic_message = ask_question(question, correct_answer, message)
romantic_messages.append(romantic_message)
print("\nCongratulations! You answered all the questions correctly.")
print("\nHere are the cute romantic messages for your sweetheart:")
for message in romantic_messages:
time.sleep(1)
print(f"\n❤️ {message}")
print("\nNow, let's complete some special tasks to make this day even more memorable!\n")
for task, reward in tasks:
perform_task(task, reward)
# Run the game
display_intro()
play_valentines_day_game()
Valentine Game HTML Code
This is the HTML code for the landing page, which can be found in the snippet labeled “A simple index.html.” It consists of a brief introduction and a button to start the LoveQuest.
from flask import Flask, render_template, request
app = Flask(__name__)
questions = [
("What's the sweetest nickname you call each other?", "sweetheart", "You'll always be my sweetest sweetheart."),
("Where did you go on your first date?", "moonlit park", "Our first date was like a scene from a moonlit park, magical and unforgettable."),
("What's your favorite romantic movie to watch together?", "the notebook", "Our love story is even more beautiful than 'The Notebook'."),
("What's your favorite love song?", "unchained melody", "Our love is like an 'Unchained Melody,' timeless and full of passion."),
("What's your dream romantic getaway destination?", "paris", "Just like Paris, our love is filled with romance and enchantment."),
]
tasks = [
("Write a sweet note to your sweetheart and read it aloud.", "Your words are as beautiful as your heart."),
("Send a virtual hug to your sweetheart right now.", "Even across the distance, I feel your warmth."),
]
romantic_messages = []
written_notes = []
@app.route('/')
def index():
return render_template('index.html')
@app.route('/quiz', methods=['GET', 'POST'])
def quiz():
if request.method == 'POST':
user_answer = request.form['answer'].lower()
current_question = questions[len(romantic_messages)]
if user_answer == current_question[1]:
romantic_messages.append(current_question[2])
return render_template('quiz.html', questions=questions, romantic_messages=romantic_messages)
@app.route('/tasks', methods=['GET', 'POST'])
def tasks_page():
if request.method == 'POST':
task_index = int(request.form['task_index'])
task, reward = tasks[task_index]
note = f"Task: {task}\nReward: {reward}"
written_notes.append(note)
return render_template('tasks.html', tasks=tasks, written_notes=written_notes)
if __name__ == '__main__':
app.run(debug=True)
Before running this code, ensure that you have Flask installed (pip install flask).
To proceed, create a folder called “templates” in the same directory as your Python script. Inside this folder, create three HTML files: index.html, quiz.html, and tasks.html. Feel free to customize these HTML files to your liking.
Here’s the index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>LoveQuest</title>
</head>
<body>
<h1>Welcome to LoveQuest: A Romantic Adventure!</h1>
<p>Click the button below to start your romantic journey.</p>
<a href="/quiz" class="button">Start LoveQuest</a>
</body>
</html>
Here is the quiz.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>LoveQuest Quiz</title>
</head>
<body>
<h1>LoveQuest Quiz</h1>
<form method="post" action="/quiz">
{% for question in questions %}
<p>{{ question[0] }}</p>
<input type="text" name="answer" required>
<br>
{% endfor %}
<input type="submit" value="Next">
</form>
{% for message in romantic_messages %}
<p>{{ message }}</p>
{% endfor %}
</body>
</html>
Now tasks.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>LoveQuest Tasks</title>
</head>
<body>
<h1>LoveQuest Tasks</h1>
<form method="post" action="/tasks">
{% for i, task in enumerate(tasks) %}
<p>{{ task[0] }}</p>
<input type="hidden" name="task_index" value="{{ i }}">
<input type="submit" value="Complete Task">
<br>
{% endfor %}
</form>
<h2>Your Sweet Notes:</h2>
{% for note in written_notes %}
<p>{{ note }}</p>
{% endfor %}
</body>
</html>
Added Romance with Unique Assignments
LoveQuest goes beyond mere inquiries by offering unique assignments that are sure to create lasting memories. Pen heartfelt messages to your beloved, send virtual embraces, and partake in activities that deepen the emotional bond between you and your partner. Every completed task yields a written note, serving as a cherished memento of the love and dedication poured into your relationship.
Your Sweet Memories with Valentine Game
It’s easy to embark on a LoveQuest! Just head over to the LoveQuest web application, begin your romantic journey, and dive into a world filled with love, laughter, and unforgettable moments. The application is designed to be user-friendly, ensuring that anyone who wants to strengthen their bond with their partner can easily do so.
Are you ready to embark on a Valentine Game ?
It’s as easy as visiting the Valentine Game web application and beginning your romantic journey. Get ready to be swept away in a world filled with love, laughter, and unforgettable moments. This user-friendly application is perfect for anyone looking to strengthen their bond with their special someone.
Conclusion
Valentine Game LoveQuest is not just a game, it’s an experience that turns ordinary moments into extraordinary memories. Take a break from the ordinary and dive into the enchanting world of Valentine Game LoveQuest. Let the magic of romance unfold as you answer questions, complete tasks, and write heartfelt notes. Your romantic adventure awaits.
Get a Valentine Game 2024 with Source Code for Free.
Start your Valentine Game LoveQuest today!
0 Comments