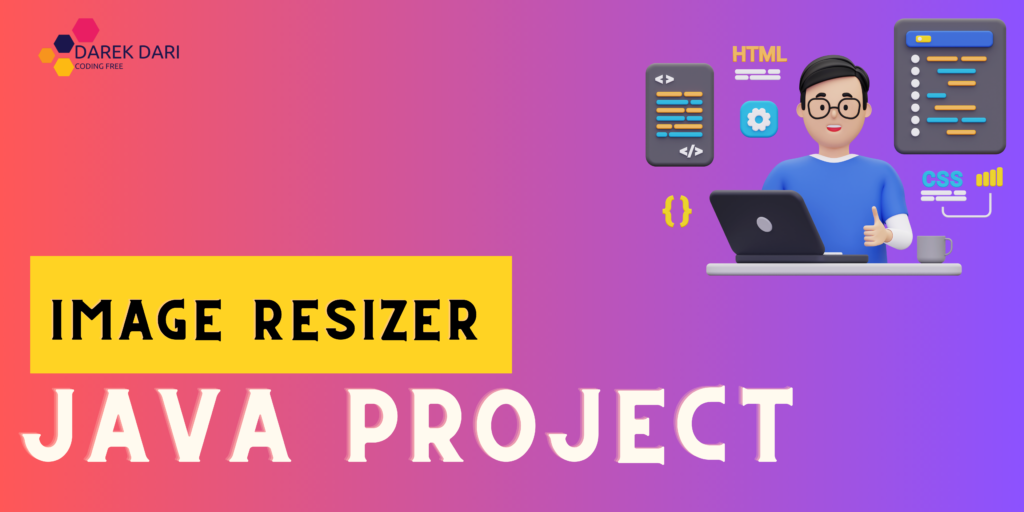
Table of Contents
Hey there, you want to make an image resizer in java?
I’ve got something interesting to share with you today. You know how sometimes you need to resize images when working on projects or applications? Well, I’ve got your back! I’ve discovered three fantastic methods to image resizer in java, and I’m going to break them down for you.
Solution 1: Using Java ImageIO
Let’s start with the basics. Solution 1 relies on Java’s built-in ImageIO library. Think of it as your trusty Swiss Army knife for image manipulation. Here’s how it works: You have an image you want to resize, and this method allows you to do it effortlessly. You load the image, specify the new dimensions you want, and voila – the image is resized! It’s like magic.
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageResizerUsingImageIO {
public static void main(String[] args) {
try {
File inputFile = new File("input.jpg");
BufferedImage originalImage = ImageIO.read(inputFile);
int newWidth = 300;
int newHeight = 200;
BufferedImage resizedImage = new BufferedImage(newWidth, newHeight, originalImage.getType());
Graphics2D graphics = resizedImage.createGraphics();
graphics.drawImage originalImage, 0, 0, newWidth, newHeight, null);
graphics.dispose();
File outputFile = new File("output_using_imageio.jpg");
ImageIO.write(resizedImage, "jpg", outputFile);
System.out.println("Image resized and saved to 'output_using_imageio.jpg'");
} catch (IOException e) {
e.printStackTrace();
}
}
}
It’s the go-to solution for a quick and simple image resizer in java job.
Solution 2: Using Java Thumbnails
Now, let’s kick it up a notch. Solution 2 involves using the Java Thumbnails class from the Java 2D API. This method is like having a professional photographer retouching your pictures. It offers more control and helps you maintain the image’s quality.
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
public class ImageResizerUsingThumbnails {
public static void main(String[] args) {
try {
File inputFile = new File("input.jpg");
BufferedImage originalImage = ImageIO.read(inputFile);
int newWidth = 300;
int newHeight = 200;
BufferedImage resizedImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics2D = resizedImage.createGraphics();
graphics2D.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
graphics2D.drawImage(originalImage, 0, 0, newWidth, newHeight, null);
graphics2D.dispose();
File outputFile = new File("output_using_thumbnails.jpg");
ImageIO.write(resizedImage, "jpg", outputFile);
System.println("Image resized and saved to 'output_using_thumbnails.jpg'");
} catch (IOException e) {
e.printStackTrace();
}
}
}
This method is all about giving you control and ensuring top-notch quality. It’s like being the director of your own photo shoot.
Solution 3: Using a Third-Party Library (imgscalr)
Now, if you’re all about efficiency and ease, Solution 3 is where it’s at. It’s like having a personal assistant for your images. This method leverages the power of the imgscalr library, which simplifies the entire image resizing process.
import org.imgscalr.Scalr;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class ImageResizerUsingImgscalr {
public static void main(String[] args) {
try {
File inputFile = new File("input.jpg");
BufferedImage originalImage = ImageIO.read(inputFile);
int newWidth = 300;
int newHeight = 200;
BufferedImage resizedImage = Scalr.resize(originalImage, Scalr.Method.ULTRA_QUALITY, Scalr.Mode.FIT_EXACT, newWidth, newHeight);
File outputFile = new File("output_using_imgscalr.jpg");
ImageIO.write(resizedImage, "jpg", outputFile);
System.out.println("Image resized and saved to 'output_using_imgscalr.jpg'");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Solution 3 is like having a magical tool that does all the hard work for you. Just feed it your image (image resizer in java) and specify the size you want, and it will take care of the rest.
Choosing the Right Solution
Now, you might wonder, which one should you pick? Well, it all depends on what you need for your project. If you’re looking for a quick and straightforward solution, go for Solution 1. It’s like your instant fix. If you’re a perfectionist and need to have full control over the image quality, Solution 2 is the way to go. It’s like having a professional photo studio at your disposal. And if you’re all about saving time and effort, Solution 3 is your best friend. It’s like having a helpful assistant doing the work for you.
In a nutshell, these three methods provide different tools for image resizing in Java, each catering to various development needs. It’s like having a versatile set of tools in your toolbox, and you can pick the one that fits your project’s needs the best.
How to transform an image in Java?
Transforming an image can involve various operations, such as rotation, cropping, or flipping. For this, you can use the AffineTransform
class in Java. Here’s a basic example of how to rotate an image:
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageTransformer {
public static void transformImage(File inputFile, File outputFile, double rotationAngle) {
try {
BufferedImage originalImage = ImageIO.read(inputFile);
int width = originalImage.getWidth();
int height = originalImage.getHeight();
BufferedImage transformedImage = new BufferedImage(width, height, originalImage.getType());
Graphics2D graphics = transformedImage.createGraphics();
AffineTransform transform = new AffineTransform();
transform.rotate(Math.toRadians(rotationAngle), width / 2, height / 2);
graphics.setTransform(transform);
graphics.drawImage(originalImage, 0, 0, null);
graphics.dispose();
ImageIO.write(transformedImage, "jpg", outputFile);
System.out.println("Image transformed and saved to " + outputFile.getName());
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
File inputFile = new File("input.jpg");
File outputFile = new File("output.jpg");
double rotationAngle = 90; // Rotate 90 degrees
transformImage(inputFile, outputFile, rotationAngle);
}
}
This code rotates the image by 90 degrees, but you can apply other transformations as needed.
How to scale down BufferedImage in Java?
To scale down a BufferedImage
in Java, you can use the Scalr
library. First, you need to include this library in your project (image resizer in java). You can download it from its GitHub repository here. Once you have the library, you can use it like this:
import org.imgscalr.Scalr;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class BufferedImageScaler {
public static void scaleImage(File inputFile, File outputFile, int newWidth, int newHeight) {
try {
BufferedImage originalImage = ImageIO.read(inputFile);
BufferedImage scaledImage = Scalr.resize(originalImage, newWidth, newHeight);
ImageIO.write(scaledImage, "jpg", outputFile);
System.out.println("Image scaled down and saved to " + outputFile.getName());
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
File inputFile = new File("input.jpg");
File outputFile = new File("output.jpg");
int newWidth = 300;
int newHeight = 200;
scaleImage(inputFile, outputFile, newWidth, newHeight);
}
}
How to resize image size?
The process of changing the image resizer in java typically involves resizing, which we discussed in detail in the first section. However, if you want to adjust the size without a specific width and height, you can maintain the aspect ratio while resizing:
public class ImageResizer {
public static void resizeImageMaintainingAspectRatio(File inputFile, File outputFile, int maxSize) {
try {
BufferedImage originalImage = ImageIO.read(inputFile);
int width = originalImage.getWidth();
int height = originalImage.getHeight();
int newWidth;
int newHeight;
if (width > height) {
newWidth = maxSize;
newHeight = (maxSize * height) / width;
} else {
newHeight = maxSize;
newWidth = (maxSize * width) / height;
}
BufferedImage resizedImage = new BufferedImage(newWidth, newHeight, originalImage.getType());
Graphics2D graphics = resizedImage.createGraphics();
graphics.drawImage(originalImage, 0, 0, newWidth, newHeight, null);
graphics.dispose();
ImageIO.write(resizedImage, "jpg", outputFile);
System.out.println("Image resized while maintaining aspect ratio and saved to " + outputFile.getName());
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
File inputFile = new File("input.jpg");
File outputFile = new File("output.jpg");
int maxSize = 300;
resizeImageMaintainingAspectRatio(inputFile, outputFile, maxSize);
}
}
In this example, we maintain the aspect ratio while image resizer in java to fit within a maximum size (maxSize
).
I hope these explanations and examples help you with image resizer in java and transformations in Java. If you have any more questions or need further assistance, feel free to ask!
FAQs (Frequently Asked Questions)
- Why should I use Java ImageIO for image resizing?
- It’s the simplest and native way to resize images without the need for extra tools. It’s like using the most basic but effective tool from your toolkit.
- How can I ensure the best quality for my resized images with Java Thumbnails?
- Java Thumbnails allows you to fine-tune the quality of your resized images for top-notch results. It’s like having a professional photographer retouching your photos.
- What makes imgscalr a great choice for image resizing?
- Imgscalr is like having a magical tool that simplifies image resizer in java. It’s super efficient and does most of the work for you.
- Can I mix and match these methods in a single project for different tasks?
- Absolutely! You can use these methods based on what your project (image resizer in java) needs at any given moment. It’s like having a diverse set of tools in your toolbox for different tasks.
- Where can I find the imgscalr library for Solution 3?
- You can grab the imgscalr library from this GitHub repository.
For more details and extra resources on image resizer in java, just dive into the documentation and guides available online.
Conclusion for image resizer in Java
In conclusion, Java offers several versatile methods for image resizing and transformation, making it a powerful choice for image resizer in Java. Developers can tailor their solutions to specific project requirements, ensuring that images are manipulated with precision. Whether you need a quick and straightforward resizing using Java ImageIO, precise control over image quality with Java Thumbnails, or efficient scaling with the imgscalr library, Java provides the tools you need to get the job done as an image resizer in Java.
Additionally, we explored how to transform images using the AffineTransform class for operations like rotation and how to scale down a BufferedImage using the Scalr library. These methods offer flexibility and adaptability, making Java a robust language for image manipulation and serving as a reliable image resizer in Java.
Remember that the choice of method depends on your project’s unique needs. Choose the one that aligns best with your objectives, and don’t hesitate to experiment with these techniques to achieve the desired results as an image resizer in Java.
Whether you’re a Java developer looking to enhance your image processing capabilities or simply someone interested in image manipulation, the possibilities are within your reach with the right image resizer in Java. With the knowledge gained from this article, you can confidently tackle image resizing and transformations in Java to create an effective image resizer in Java. If you have more questions or need further assistance, don’t hesitate to explore online resources and communities. Happy coding!
0 Comments