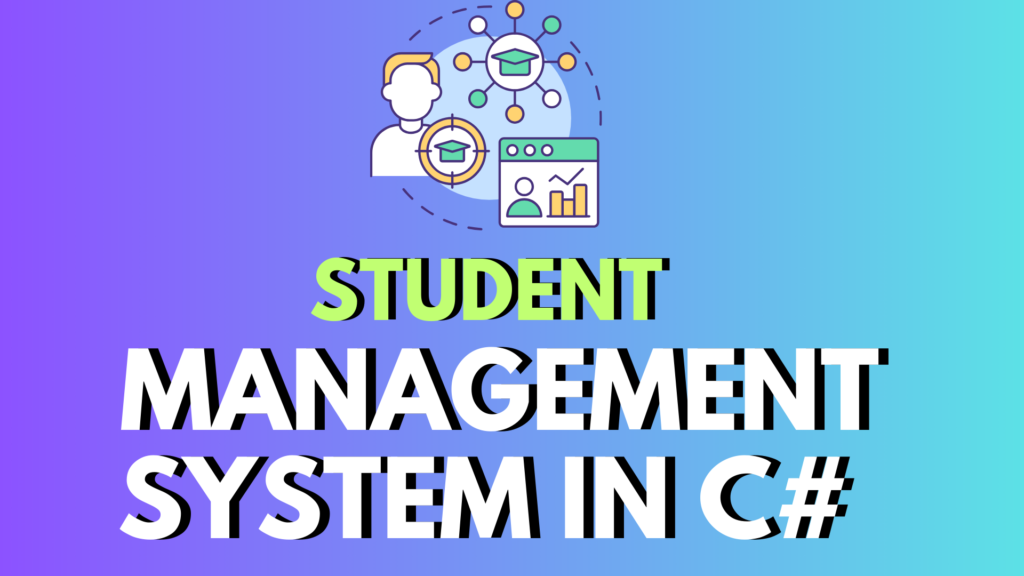
Introduction
Managing student records efficiently is a crucial task for educational institutions. A Student Management System (SMS) streamlines administrative processes, tracks student information, and automates essential operations. If you are looking to build a Student Management System in C#, this guide will walk you through the process, provide source code examples, and explore best practices.
Whether you’re a beginner or an experienced developer, this article will help you understand the fundamentals of an SMS project in C# with hands-on coding examples and GitHub resources.
What is a Student Management System?
A Student Management System (SMS) is a software application designed to manage student-related data, including:
- Student registration and profile management
- Attendance tracking
- Exam and grading system
- Fee management
- Course and schedule management
- Reporting and analytics
By developing an SMS in C#, institutions can automate many tasks, reducing manual workload and improving efficiency.
Why Use C# for a Student Management System?
C# is a powerful, object-oriented programming language that provides robustness, scalability, and security for desktop and web applications. Here’s why C# is ideal for SMS development:
- Strong integration with .NET Framework and ASP.NET for web-based applications.
- Rich library support for database connectivity and UI design.
- Cross-platform capabilities using .NET Core for wider usability.
- High performance and security for enterprise-level applications.
Key Features of a Student Management System in C#
A well-built C# Student Management System should include the following features:
1. User Authentication & Role Management
- Admin, teacher, student logins
- Secure authentication using ASP.NET Identity
2. Student Record Management
- Add, update, delete student details
- Store personal and academic information
3. Course & Attendance Management
- Assign students to courses
- Mark and track attendance records
4. Grade & Exam Management
- Enter and manage exam scores
- Generate grade reports
5. Fee Management System
- Track student payments
- Generate invoices and fee receipts
6. Reporting & Dashboard
- View insights on student performance and attendance
- Generate PDF or Excel reports
Student Management System C# Example (With Code)
Here’s a simple C# student management system project with Windows Forms (WinForms) and SQL Server.
Step 1: Setting Up the Project
- Open Visual Studio and create a Windows Forms App (.NET Framework) project.
- Add a SQL Server Database for storing student records.
- Install Entity Framework for database operations.
Step 2: Database Schema (SQL Server)
CREATE TABLE Students (
StudentID INT PRIMARY KEY IDENTITY(1,1),
Name NVARCHAR(100),
Age INT,
Email NVARCHAR(100),
Course NVARCHAR(100)
);
Step 3: Connecting C# to SQL Database
using System;
using System.Data.SqlClient;
class DatabaseConnection {
static string connectionString = "your_connection_string_here";
public static void AddStudent(string name, int age, string email, string course) {
using (SqlConnection conn = new SqlConnection(connectionString)) {
string query = "INSERT INTO Students (Name, Age, Email, Course) VALUES (@Name, @Age, @Email, @Course)";
SqlCommand cmd = new SqlCommand(query, conn);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Age", age);
cmd.Parameters.AddWithValue("@Email", email);
cmd.Parameters.AddWithValue("@Course", course);
conn.Open();
cmd.ExecuteNonQuery();
}
}
}
Step 4: Creating a Simple UI with WinForms
Use Windows Forms Designer to create a form with textboxes and buttons for adding students.
private void btnSave_Click(object sender, EventArgs e) {
string name = txtName.Text;
int age = Convert.ToInt32(txtAge.Text);
string email = txtEmail.Text;
string course = txtCourse.Text;
DatabaseConnection.AddStudent(name, age, email, course);
MessageBox.Show("Student added successfully!");
}
Frequently Asked Questions
1. How to Create a Student Management System in C# from Scratch?
Provide a step-by-step guide, including project setup, database integration, and feature implementation.
2. Where Can I Find a Student Management System Project in C# with Source Code?
3. What Are Some Examples of Student Management Systems in C#?
- Console-Based Application: GitHub Repository
- Windows Forms Application: GitHub Repository
4. How to Implement CRUD Operations in C#?
Provide detailed code snippets demonstrating Create, Read, Update, and Delete operations.
5. Are There Any Video Tutorials for Building a Student Management System in C#?
Conclusion
Building a Student Management System in C# is a rewarding project that enhances both technical skills and software development knowledge. Whether you’re looking for a C# Student Management System tutorial or a fully functional project with source code, this guide provides all the essential details to get started.
Next Steps:
✔ Explore GitHub for more C# Student Management System projects ✔ Implement additional features like email notifications, dashboards, or cloud storage ✔ Share your project online to get feedback and improve!
Have any questions? Let’s discuss in the comments!
- Student Management System C# example
- Student Management System C# tutorial
- Student Management System C# GitHub
- C# Student Information System project source code
- How to create a Student Management System in C#
- Student Management System project in C# with database
0 Comments